相邻颜色不得重复
The name of the problem I picked from LeetCode for this week is “Remove All Adjacent Duplicates In String”.
吨他的名字,我从采摘的问题本文给出了本周是“删除所有相邻重复在字符串”。
Given a string S
of lowercase letters, a duplicate removal consists of choosing two adjacent and equal letters, and removing them.
给定一个 小写字母 的字符串 S
,重复删除包括选择两个相邻且相等的字母,然后将其删除。
We repeatedly make duplicate removals on S until we no longer can.
我们反复对S进行重复删除,直到无法删除为止。
Return the final string after all such duplicate removals have been made. It is guaranteed the answer is unique.
完成所有此类重复删除后,返回最终字符串。 保证答案是唯一的。
Example:Input: "abbaca"Output: "ca"Explanation:
For example, in "abbaca" we could remove "bb" since the letters are adjacent and equal, and this is the only possible move. The result of this move is that the string is "aaca", of which only "aa" is possible, so the final string is "ca".
Below is another example showing how to process while working on this problem:
下面是另一个示例,显示了解决此问题时如何进行处理:

The giving string in the example is ‘mppmhkkl’
and the answer is ‘hl’
. First, the duplicate ‘pp’
is deleted, then ‘mm’
is removed, and finally ‘kk’
is deleted to get the result. The string left after removing all of the adjacent duplicates is ‘hl’
. Note that you only delete two at a time.
示例中的给定字符串为'mppmhkkl'
,答案为'hl'
。 首先,删除重复的'pp'
,然后删除'mm'
,最后删除'kk'
以获得结果。 删除所有相邻重复项后剩下的字符串是'hl'
。 请注意,一次只能删除两个。
我JavaScript解决方案 (My Solution in JavaScript)
My approach was to use a stack data structure to solve this problem. I will follow these steps to implement it:
我的方法是使用堆栈数据结构来解决此问题。 我将按照以下步骤实施它:
- Create an empty stack array. 创建一个空的堆栈数组。
- Iterate through the input string. 遍历输入字符串。
- Check at each iteration if the top (last) element of the stack is equal to the current element of the input string. 在每次迭代时检查堆栈的顶部(最后)元素是否等于输入字符串的当前元素。
- If true, remove the top element of the stack. 如果为true,则删除堆栈的顶部元素。
- If not, add the current element of the string on top of the stack. 如果不是,请将字符串的当前元素添加到堆栈顶部。
- Return all elements of the stack as a string after joining them. 加入堆栈后,将所有元素作为字符串返回。
Let’s see the implementation of the code in JavaScript to get a better understanding:
让我们看一下JavaScript中的代码实现,以更好地理解:
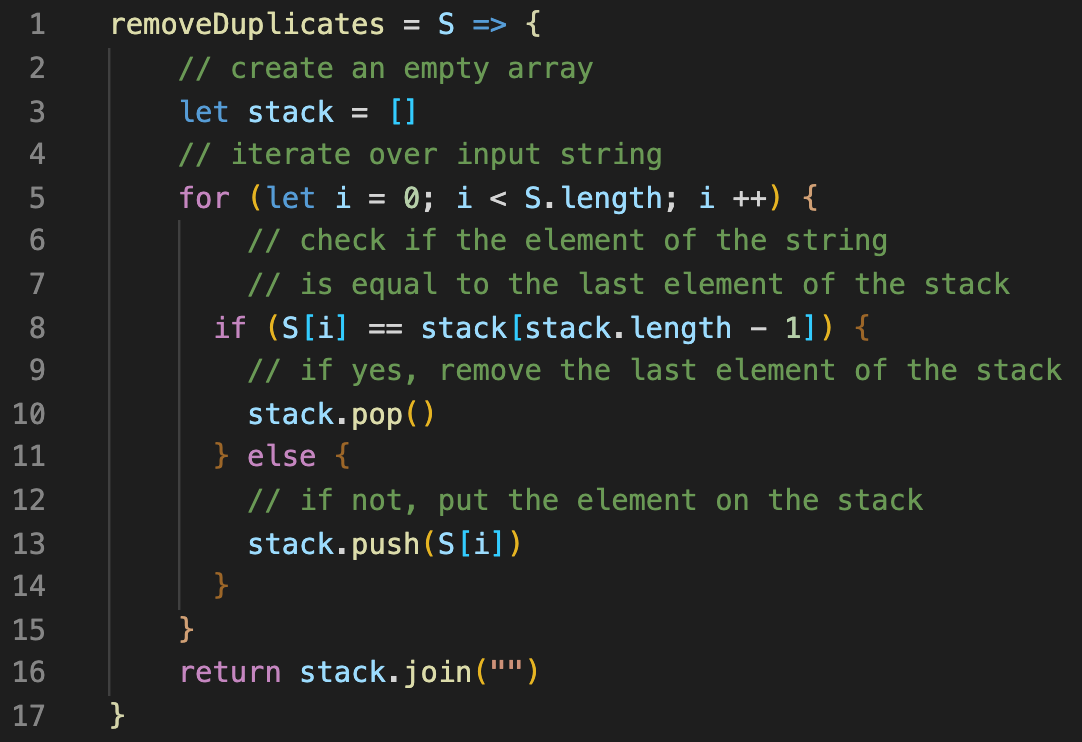
If the current element of the string is the same as the last element of the stack, pop the last element of the stack, otherwise, add it to the stack as you see in the above code. The algorithm keeps removing adjacent duplicates until no more duplicates exist in the result. Finally, join all of the items in the stack and return it as a string.
如果字符串的当前元素与堆栈的最后一个元素相同,请弹出堆栈的最后一个元素,否则,如上面的代码所示,将其添加到堆栈中。 该算法会不断删除相邻的重复项,直到结果中不再存在重复项为止。 最后,将堆栈中的所有项目连接起来,并将其作为字符串返回。
我在Ruby中的解决方案 (My Solution in Ruby)
Here is how I solved the same problem in Ruby with the same approach:
这是我使用相同方法解决Ruby中相同问题的方法:
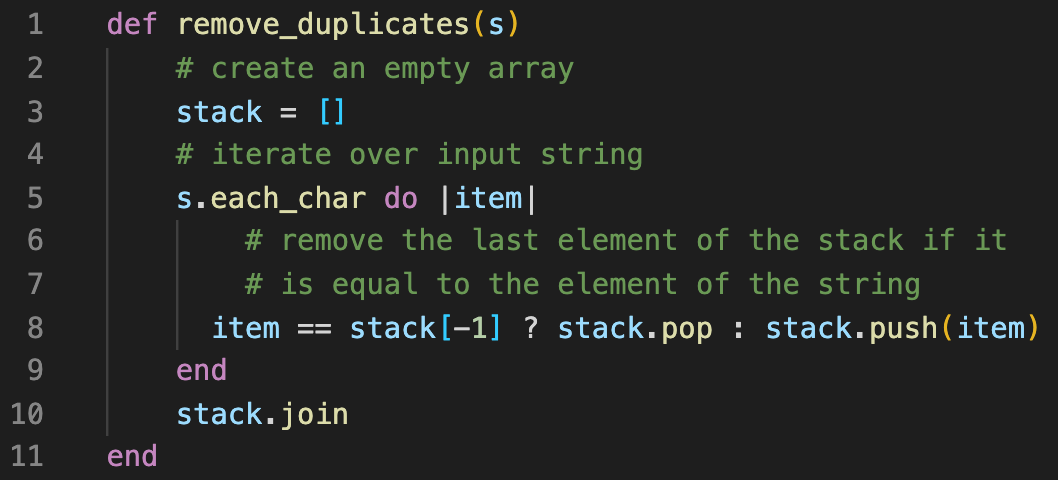
Again, iterate over the given string and check by using a stack if the current element is equal to the top of the stack. If yes, delete the last element from the stack to remove the two duplicate characters. If it does not equal, push it to the top of the stack.
同样,遍历给定的字符串,并使用堆栈检查当前元素是否等于堆栈的顶部。 如果是,请从堆栈中删除最后一个元素以删除两个重复的字符。 如果不相等,则将其推入堆栈的顶部。
时空复杂性 (Time and Space Complexity)
Since we are iterating through each element of the given string only once by using a stack, the time complexity is O(n) where n
is the length of the string and the space complexity is also O(n).
由于我们使用堆栈仅迭代给定字符串的每个元素一次, 因此 时间复杂度为O(n) ,其中n
是字符串的长度, 空间复杂度也为O(n) 。
结论 (Conclusion)
Using two-pointer or recursion are the other alternative ways to solve this algorithm, although I only shared my approach using a stack. I found this solution simple and efficient, I hope you also like it. Thank you for reading my post!
使用二指针或递归是解决此算法的其他替代方法,尽管我只使用堆栈来共享方法。 我发现此解决方案简单有效,希望您也喜欢它。 感谢您阅读我的帖子!
翻译自: https://medium.com/swlh/remove-adjacent-duplicates-problem-5b9ac4abe87f
相邻颜色不得重复