angular react
Reactive forms are an alternative to creating forms in Angular. Most of the heavy lifting for creating reactive forms happens in the component class instead of the template (HTML file).
React性表单是在Angular中创建表单的替代方法。 创建React形式的大部分繁重工作都发生在组件类中,而不是模板(HTML文件)中。
One of the big benefits of using reactive forms is that it makes testing easier. The reason for this is that all the code related to your form lives in the component class. Given this, you don’t need to access the template on your tests to test your form. Instead, you can simply write a unit test.
使用React形式的最大好处之一是它使测试更加容易。 原因是与表单相关的所有代码都存在于组件类中。 鉴于此,您无需访问测试中的模板即可测试表单。 相反,您可以简单地编写一个单元测试。
Module setup
模块设置
The first step to implement a reactive form will be to import ReactiveFormsModule
to your application’s module. This module can be imported from @angular/forms
.
实施React式表单的第一步是将ReactiveFormsModule
导入应用程序的模块。 可以从@angular/forms
导入此模块。
For simplicity purposes, as part of this example, I’ll be writing all the form related code inside the AppComponent
. The following code snippet shows how to include the ReactiveFormsModule
for you to start creating your first reactive form.
为了简单起见,作为本示例的一部分,我将在AppComponent
编写所有与表单相关的代码。 以下代码段显示了如何包括ReactiveFormsModule
以便您开始创建第一个React形式。
创建React形式 (Creating a reactive form)
Before we start, there are two important concepts we need to go over: form groups and form controls.
在开始之前,我们需要回顾两个重要的概念: 表单组和表单控件 。
Each control in your form will be of type FormControl
. A form control could represent something like a user's first name, date of birth, or country of birth. From a user's perspective, these could be input fields, text areas, or dropdown lists. Form controls keep track of the value, validations, and events in a control. A FormGroup
contains a group of form controls.
表单中的每个控件都将为FormControl
类型。 表单控件可以表示用户的名字,出生日期或出生国家。 从用户的角度来看,这些可以是输入字段,文本区域或下拉列表。 表单控件跟踪控件中的值,验证和事件。 一个FormGroup
包含一组表单控件。
For you to add controls to your form, you will first instantiate an object of type FormGroup
. When building reactive forms, for you to to create your form, the first step is to create a FormGroup
.
为了向表单添加控件,您将首先实例化FormGroup
类型的对象。 在构建React式表单时,要创建表单,第一步是创建FormGroup
。
Let’s start by creating the form group. Once you’ve done this, you will need to pass in as a parameter an object where:
让我们从创建表单组开始。 完成此操作后,您需要将以下对象作为参数传递:
- The keys define your control’s names 键定义控件的名称
The values will be objects of type
FormControl
这些值将是
FormControl
类型的对象
In this example, if we have a username and password, you will create an object with keys of username
and password
. As values, you will be providing, for now, new form controls.
在此示例中,如果我们具有用户名和密码,则将创建一个具有username
和password
键的对象。 作为值,您现在将提供新的表单控件。
As your forms become more complex, there is a simpler way of building them by using the FormBuilder
class. For this, you can start by injecting the FormBuilder
class and calling the group
method.
随着表单变得越来越复杂,使用FormBuilder
类可以更简单地构建它们。 为此,您可以首先注入FormBuilder
类并调用group
方法。
As a parameter to the group
method, you will pass in an object where
作为group
方法的参数,您将传入一个对象,其中
- The keys define your control’s names 键定义控件的名称
- The values, for now, are empty arrays 目前的值是空数组
So far we are done with the component class. Let’s go over what needs to be done in the template.
到目前为止,我们已经完成了组件类。 让我们回顾一下模板中需要完成的工作。
From your template, you can go ahead and wrap your input fields that represent your form controls in a form
tag. Your next step should be to add the formGroup
directive to your form tag. As a value to the formGroup
directive, pass in the form group you just instantiated on the component class.
您可以从模板继续,将代表表单控件的输入字段包装在form
标签中。 下一步应该是将formGroup
指令添加到表单标签中。 作为formGroup
指令的值,传入刚在组件类上实例化的表单组。
Finally, for each one of your controls, add a formControlName
directive. As a value, you will pass in a string with the name your form controls that you used in the component class.
最后,为每个控件添加一个formControlName
指令。 作为值,您将传入一个字符串,该字符串的名称应与您在组件类中使用的表单控件相同。
表单控件默认值 (Form control default values)
If for some reason, you need to provide default values to your form controls, you can pass these as the first value to the empty arrays on your form controls. If you were to want to set helloworld
as the default username in your control, you will need to do the following.
如果由于某种原因需要向表单控件提供默认值,则可以将这些默认值作为第一个值传递给表单控件上的空数组。 如果要在控件中将helloworld
设置为默认用户名,则需要执行以下操作。
设置表单控件的默认值 (Setting default values to form controls)
Sometimes you are going to need to set default values to your forms. This might be because you have an edit form where the user is going to edit some type of information. The methods `setValue` and `patchValue` will let you update values on your forms. As an argument, you will need to pass in an object. The keys for this object will be the form control names. The values will be the values for each one of your form controls.
有时您需要为表单设置默认值。 这可能是因为您有一个编辑表单,用户将在其中编辑某种类型的信息。 setValue和patchValue方法可以让您更新表单上的值。 作为参数,您将需要传递一个对象。 该对象的键将是表单控件名称。 这些值将是每个表单控件的值。
If you want to set only a specific form control, you might want to call patchValue
. The patchValue
method lets you update one or more fields from your form. Take as an example a form that contains a username
and password
form controls. In this case, if you want to set only a default value for the username
you could do the following.
如果只想设置特定的表单控件,则可能要调用patchValue
。 patchValue
方法使您可以更新表单中的一个或多个字段。 以包含username
和password
表单控件的表单为例。 在这种情况下,如果只想为username
设置默认值,则可以执行以下操作。
this.form.patchValue({ username: 'username' });
On the other hand, the setValue
method will need you to pass in an object with all the controls for your form. In other words, if you try to only set the username
form control, this will result in a runtime error like this one: Must supply a value for form control with name: ‘password’.
另一方面, setValue
方法将需要您传入具有表单所有控件的对象。 换句话说,如果您尝试仅设置username
表单控件,则将导致如下运行时错误: 必须为名称为'password'的表单控件提供一个值 。
This is an example of the correct usage of the setValue
method for this form.
这是此表单的setValue
方法正确用法的一个示例。
this.form.setValue({
username: 'username',
password: 'password'
});
表格提交 (Form submission)
We’ve created so far a form, but we can’t do that much with it until you submit it. The next step will be to add a submit button and retrieve the values for every control.
到目前为止,我们已经创建了一个表单,但是直到您提交它之后,我们才能做很多事情。 下一步将添加一个提交按钮,并检索每个控件的值。
To add the submit button, add a button
element with a type of submit
in the template. After you've done this, you are going to want to add an ngSubmit
handler on your form
tag. As an argument to this handler, you will call a submitForm
function you will be creating in your component.
要添加提交按钮,请在模板中添加带有submit
类型的button
元素。 完成此操作后,您将要在form
标签上添加ngSubmit
处理程序。 作为此处理程序的参数,您将调用要在组件中创建的submitForm
函数。
In your component class, you will now implement the submitForm
function. It is important to mention that you can give any name to this function.
现在,在您的组件类中,将实现submitForm
函数。 重要的是要提到您可以给该函数起任何名字。
Inside this function, you are going to retrieve the value of your form controls by calling this.form.getRawValue()
.
在此函数内,您将通过调用this.form.getRawValue()
来检索表单控件的值。
The getRawValue
method call will return an object where the key is the name of the control and the value is the form control's value. As an example, if for username the user entered helloworld
and password
for the password, this object will look like this:
调用getRawValue
方法将返回一个对象,其中键是控件的名称,而值是窗体控件的值。 例如,如果用户为用户名输入helloworld
并输入password
作为密码,则该对象将如下所示:
{
username: "helloworld",
password: "password"
}
验证表单控件 (Validating form controls)
Validators are functions that let you add validations to each one of your form controls. These validations can be something as simple as making a field on your form required or verifying the user entered valid e-mail address.
验证程序是使您可以向每个窗体控件添加验证的功能。 这些验证可以很简单,例如在表单上必填字段或验证用户输入的有效电子邮件地址即可。
While you can create your validators, Angular provides a few validators out of the box. These are a few of the validators provided by Angular:
虽然可以创建验证器,但是Angular提供了一些开箱即用的验证器。 以下是Angular提供的一些验证器:
RequiredValidator
- Validates that a form control has a value and marks it as requiredRequiredValidator
验证表单控件是否具有值并将其标记为必需EmailValidator
- Validates that a form control has a valid e-mail addressEmailValidator
验证表单控件具有有效的电子邮件地址MinLengthValidator
- Validates that the length of the text in a form control is greater than or equal to the valueMinLengthValidator
验证表单控件中文本的长度大于或等于该值MaxLengthValidator
- Validates that the length of the text in a form control is less than or equal to the valueMaxLengthValidator
验证表单控件中文本的长度小于或等于该值MaxValidator
- Validates that a number in a form control is less than or equal to the valueMaxValidator
验证表单控件中的数字是否小于或等于该值MinValidator
- Validates that a number in a form control is larger than the min or equal to the valueMinValidator
验证表单控件中的数字是否大于最小值或等于该值PatternValidator
- Validates that text in a form control matches a specific regular expressionPatternValidator
验证表单控件中的文本是否与特定的正则表达式匹配
For you to add a validator, you will need to add it as part of the form control’s initialization. All validators offered by Angular can be imported from the Validators
class in @angular/forms
.
为了添加验证器,您需要将其添加为表单控件初始化的一部分。 可以从@angular/forms
Angular @angular/forms
的Validators
类导入Angular提供的所有验证器。
Let’s say we are going to make the username
and password
fields to be required. For this, we'll be using the RequiredValidator
, and we'll be adding Validators.required
to both controls as a second value to the form control’s array.
假设我们将要设置username
和password
字段。 为此,我们将使用RequiredValidator
,并将Validators.required
添加到两个控件中,作为表单控件数组的第二个值。
If you want to add multiple validators to your form control, you’ll need to pass them inside an array as the second value to the form control’s value.
如果要向表单控件添加多个验证器,则需要将它们作为数组控件的第二个值传递到数组中。
定制验证器 (Custom validators)
As you have seen, Angular provides a pretty good set of default validators for your forms. The big question is, what if you need your own validator. Good news, this is something pretty simple to do.
如您所见,Angular为您的表单提供了相当不错的一组默认验证器。 最大的问题是,如果您需要自己的验证器,该怎么办。 好消息,这很简单。
Let’s say you want to have a validator that validates that a password is not the word password
. For you to create your custom validator, you will have to create a function that verifies this. As an argument, you will take in an object of type FormControl
. Next, you will get the value from your form control and return an error if one exists.
假设您要使用一个验证程序来验证密码不是单词password
。 为了创建自定义验证器,您将必须创建一个验证此函数的函数。 作为参数,您将采用FormControl
类型的对象。 接下来,您将从表单控件中获取值,如果存在则返回错误。
The next step will be for you to consume this custom validator on your form control. For you to do this, you will just add it to your list of validators when building the FormGroup
.
下一步将是您在表单控件上使用此自定义验证器。 为此,您只需要在构建FormGroup
时将其添加到验证器列表中FormGroup
。
验证表格有效 (Verify a form is valid)
So far you have a form control and have added a validator to it. The next step will be to verify that the form is valid by the time a user clicks on the submit button.
到目前为止,您已经有了一个表单控件,并向其中添加了一个验证器。 下一步将是在用户单击提交按钮时验证表单是否有效。
It is quite simple to do this, you only need to get the value from the valid
property inside your form group. In this case, inside your submitForm
method, you can do a conditional statement where you verify if this.form.valid
is true, then you move forward and do some other type of action with this data.
这样做非常简单,您只需要从表单组内的valid
属性中获取值即可。 在这种情况下,可以在您的submitForm
方法内部执行条件语句,以验证this.form.valid
是否为true,然后继续操作并对该数据执行其他某种类型的操作。
禁用提交按钮 (Disabling submit button)
Depending on your application’s requirements, under certain conditions, you might want to disable the submit button of your form.
根据您的应用程序要求,在某些情况下,您可能需要禁用表单的“提交”按钮。
A typical use case for this is that you want to disable the submit button when the form is not in a valid state. For this, you can add the disabled
attribute to your submit button. As a value, you will pass in !form.valid
to disable it only when the form is not valid.
一个典型的用例是您要在表单处于无效状态时禁用提交按钮。 为此,您可以将disabled
属性添加到您的提交按钮。 作为一个值,您将传递!form.valid
以仅在表单无效时将其禁用。
显示错误 (Displaying errors)
For you to display errors on the template, you will need to get the form control from the form first. For you to do this, on your template you need to call form.get('formControlName')
, where formControlName
is the name of your control.
为了在模板上显示错误,您需要首先从表单获取表单控件。 为此,您需要在模板上调用form.get('formControlName')
,其中formControlName
是控件的名称。
In this case, we’ll be adding this validation for the username
control. After doing this, you can call the hasError
method, where you will pass in the name of the error. Whenever you use Validators.required
, the error name will be required
. The hasError
method will return a boolean value.
在这种情况下,我们将为username
控件添加此验证。 完成此操作后,您可以调用hasError
方法,您将在其中传递错误的名称。 每当您使用Validators.required
,都将required
错误名称。 hasError
方法将返回一个布尔值。
Given this, you can use the *ngIf
structural directive in your template to determine if you want to display an error message or not.
鉴于此,您可以在模板中使用*ngIf
结构指令来确定是否要显示错误消息。
表格状态 (Form States)
Angular forms and their controls can have different states such as:- Pristine: User has not changed the values of the form control- Dirty: Opposite of pristine- Touched: User has visited the form control and exited it- Untouched: Opposite of touched
角度表单及其控件可以具有不同的状态,例如:- 原始 :用户未更改表单控件的值- 脏 :原始控件的对面-已触摸 :用户访问了表单控件并退出了它- 未 触摸 :已触摸的对面
These states help you enhance the way your forms behave. As an example, you wouldn’t want to show an error message if the username has not entered by the user.
这些状态有助于您增强表单的行为方式。 例如,如果用户未输入用户名,则不想显示错误消息。
One option is that you might consider displaying an error when the user clicks on the submit button without doing anything to the form. Additionally, you might want to only show the error message if the user touched the form control.
一种选择是,当用户单击“提交”按钮而不对表单进行任何操作时,您可能会考虑显示错误。 此外,您可能只想在用户触摸表单控件时显示错误消息。
The reason for this is that it might be annoying for your users to land on a form full of errors. By default when the form loads, it might not have a username. A friendlier approach will be to display the error only if the user has interacted with the form controls.
这样做的原因是,您的用户使用充满错误的表单可能会很烦人。 默认情况下,加载表单时,它可能没有用户名。 一种更友好的方法是仅在用户与表单控件进行交互时才显示错误。
For you to retrieve the state of your form controls, you’ll need to first get your form control usingform.get('formControlName')
. After doing this, you can call any of the following boolean properties on your form control.
为了检索表单控件的状态,您需要首先使用form.get('formControlName')
获得表单控件。 完成此操作后,您可以在窗体控件上调用以下任何布尔属性。
dirty
dirty
pristine
pristine
touched
touched
untouched
untouched
Let’s go over a few scenarios to get a better idea of how form states work in Angular.
让我们来看一些场景,以更好地了解表单状态在Angular中的工作方式。
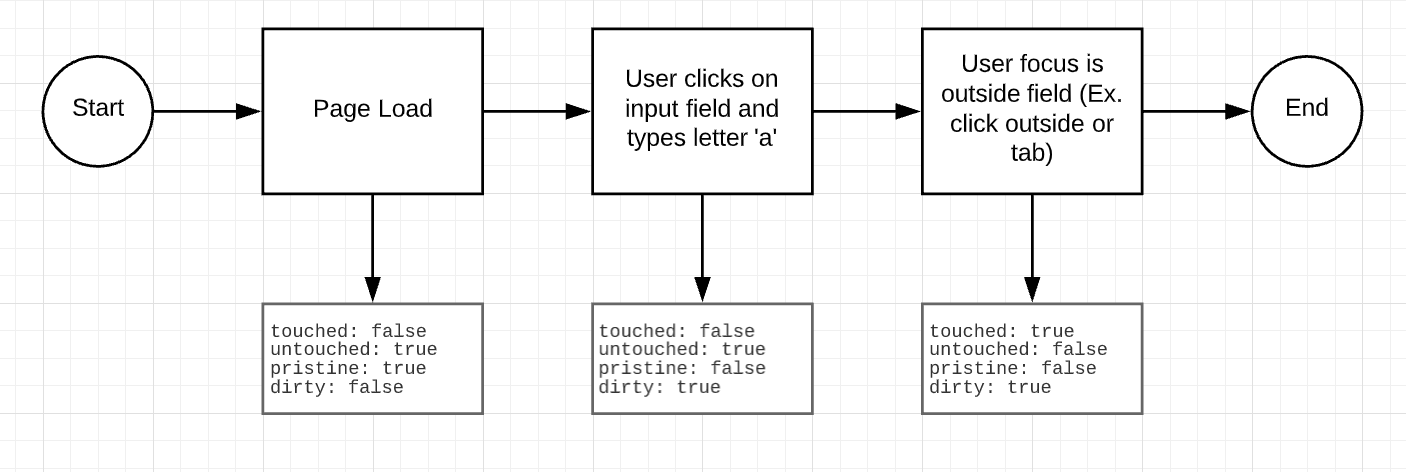
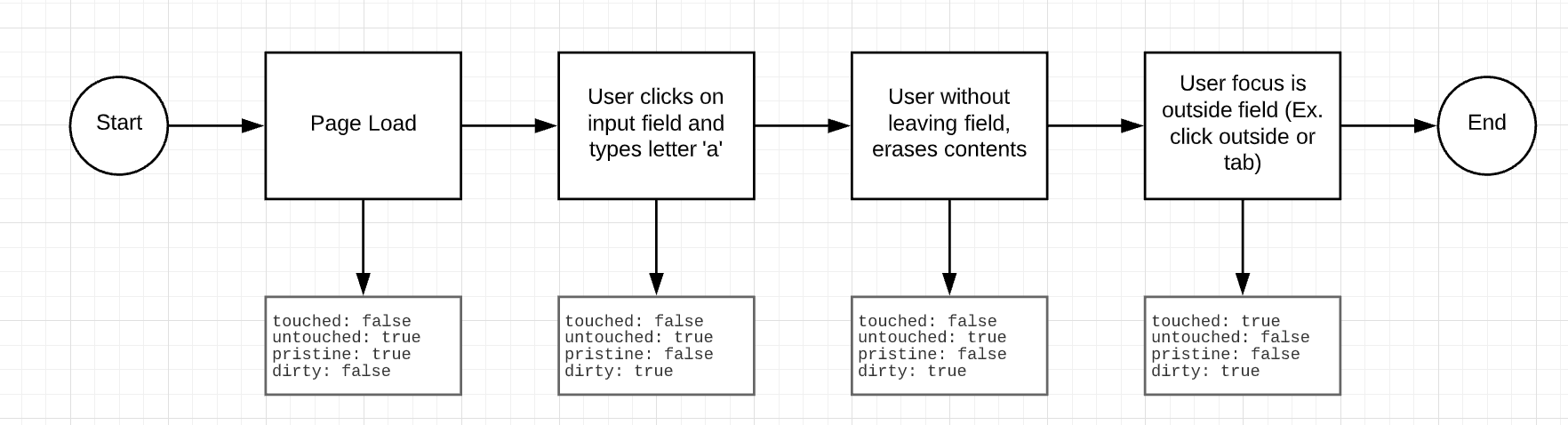
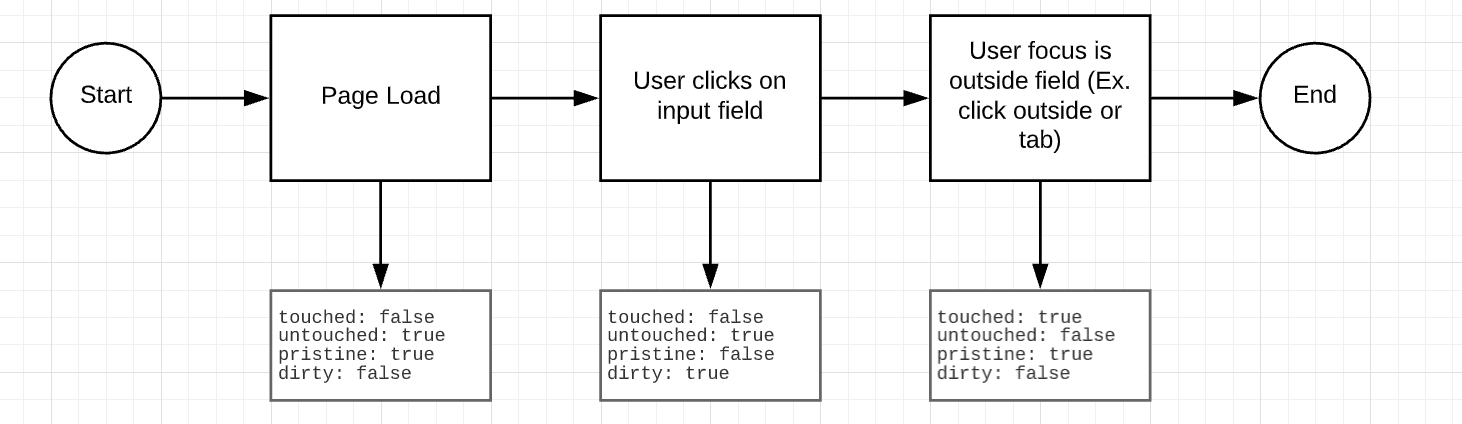
As you can see in the following example, we added an *ngIf
where we conditionally display the error message. If the error exists and if the form control is dirty, then we show an error message to the user.
如下面的示例所示,我们在条件显示错误消息的位置添加了*ngIf
。 如果错误存在并且表单控件脏了,那么我们会向用户显示错误消息。
I hope this post helps you get a better idea of how to get started with Angular Reactive Forms. Enjoy!
我希望这篇文章可以帮助您更好地了解如何开始使用Angular Reactive Forms。 请享用!
普通英语JavaScript (JavaScript In Plain English)
Enjoyed this article? If so, get more similar content by subscribing to Decoded, our YouTube channel!
喜欢这篇文章吗? 如果是这样,请订阅我们的YouTube频道解码,以获得更多类似的内容!
angular react