javascript指南
JavaScript基础 (JavaScript Fundamentals)
介绍 (Introduction)
Arrays are a structure common to all programming languages so knowing what they are and having a firm grasp on what you’re able to accomplish with Arrays will take you a long way in your journey as a software developer. The code examples I share in this post will be in JavaScript but the concepts are common among all languages. What you learn here can easily be translated to any other language you work with.
数组是所有编程语言所共有的结构,因此了解它们的含义并牢牢掌握使用数组能够完成的工作,将带给您作为软件开发人员的漫长旅程。 我在这篇文章中分享的代码示例将使用JavaScript,但是概念在所有语言中都是通用的。 您在这里学到的内容可以轻松转换为您使用的其他任何语言。
In this post I’ll be covering how to perform the create, read update and delete operations using arrays, some common functions that come with the Array prototype and also how to implement them.
在本文中,我将介绍如何使用数组执行创建,读取更新和删除操作,数组原型附带的一些常用功能以及如何实现它们。
什么是数组 (What is an Array)
Before we jump into the juicy bits of Arrays, lets quickly gloss over what they are. Arrays
在我们进入多汁的数组之前,让我们快速了解一下它们是什么。 数组
- are a fundamental data type in JavaScript 是JavaScript中的基本数据类型
are an ordered collection of values called elements that are stored at and accessed via an index
是称为元素的值的有序集合,这些值存储在索引中并通过索引进行访问
are untyped, meaning that the elements of an array could be of different types. This allows us to create complex arrays such as an array of objects or even an array of arrays (multidimensional arrays)
是无类型的 ,这意味着数组的元素可以是不同的类型。 这使我们可以创建复杂的数组,例如对象数组或什至数组数组(多维数组)
- can have elements that are constants or expressions 可以具有常量或表达式元素
have a property called length that tells you the number of elements in the array
有一个名为length的属性,它告诉您数组中元素的数量
inherit properties from
Array.prototype
that includes a wide variety useful functions that can be called from arrays orarray-like
objects从
Array.prototype
继承属性,该属性包括可以从数组或array-like
对象中调用的各种有用函数
使用数组进行CRUD操作 (CRUD operations using Arrays)
If you’re not familiar with the term CRUD it stands for Create, Read, Update and Delete. In this section we’ll go through each one of these operations and cover different ways you can perform each one.
如果您不熟悉CRUD,则代表创建,读取,更新和删除。 在本节中,我们将逐一介绍这些操作,并介绍执行每个操作的不同方法。
创建数组 (Creating Arrays)
There are several ways you can create an Array but the most common ways are by using
有几种创建数组的方法,但是最常见的方法是使用
- the Array literal syntax 数组文字语法
the Array constructor i.e.
new Array()
Array构造函数,即
new Array()
Lets take a look at each one with examples
让我们看一下每个例子
数组文字 (Array literal)
The array literal is the most common way of creating an array. It uses the square brackets as a notion of a container followed by comma separated values inside the square brackets. The following examples show how to use the array literal syntax and how arrays are untyped i.e. can contain elements of different types.
数组文字是创建数组的最常用方法。 它使用方括号作为容器的概念,后跟方括号内的逗号分隔值。 以下示例说明如何使用数组文字语法以及如何取消数组的类型化,即可以包含不同类型的元素。
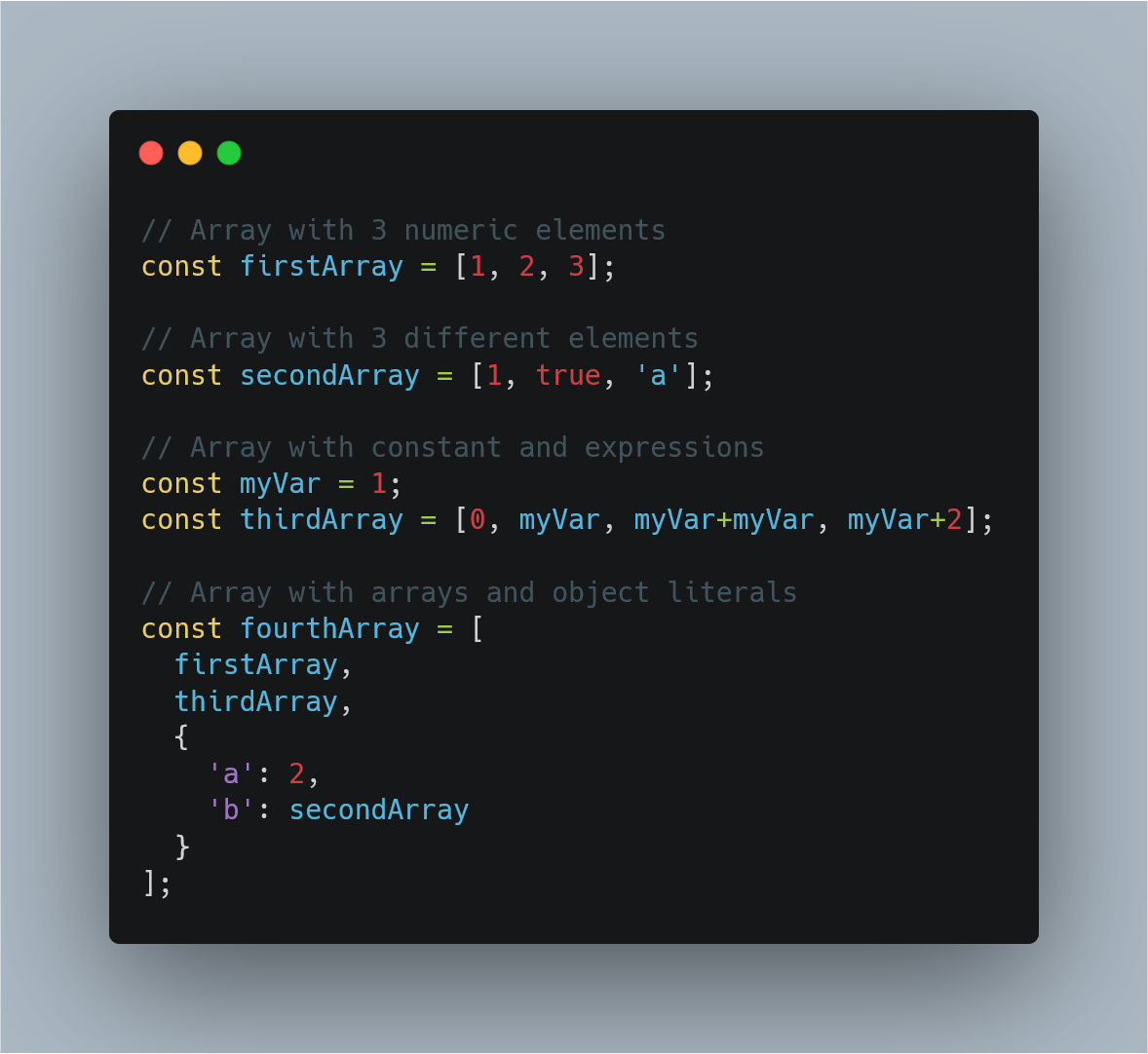
数组构造函数 (Array constructor)
Another way to create an array is through the Array constructor.
创建数组的另一种方法是通过Array构造函数。
const myArray = new Array();
Using the Array constructor, as shown above, is the same as creating an array with the array literal syntax. i.e.
如上所示,使用Array构造函数与使用数组文字语法创建数组相同。 即
// The following two lines behave exactly the same way i.e. both create an empty arraysconst myArray = new Array();
const myOtherArray = [];
The array constructor, however, is able to receive arguments that allow it to behave in different ways depending on the number and type of arguments passed to it.
但是,数组构造函数能够接收参数,使其根据传递给它的参数的数量和类型以不同的方式运行。
You can pass a single numeric argument which creates an array of the specified length. This option is mostly used when you know how many elements you’ll be placing in the array
您可以传递一个数字参数,该参数创建指定长度的数组。 当您知道要在数组中放置多少个元素时,通常使用此选项
const myArray = new Array(5);
Note: If you want to define the array with a specified size, as shown above, the argument passed must be a numeric value. Any other type would be considered as the first element that’ll be placed in the array.
注意: 如果要用指定的大小定义数组,如上所示,则传递的参数 必须 是数字值。 任何其他类型都将被视为放置在数组中的第一个元素。
- Or you can pass two or more arguments or a non-numeric argument to place the values inside the array. This works the same way as shown in the array literal examples. 或者,您可以传递两个或多个参数或一个非数字参数,以将值放置在数组中。 这与数组文字示例中显示的方式相同。
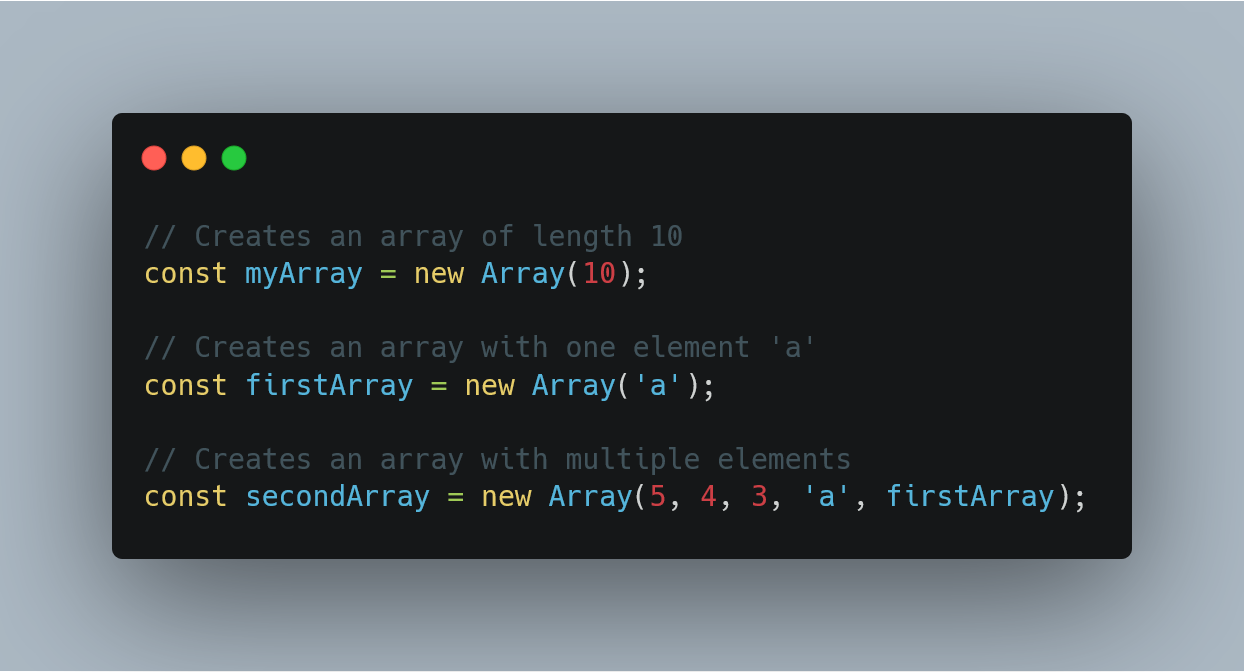
As stated earlier, these two ways are the most common ways of creating arrays that you’ll see and use 99% of the time. There are a few other ways but we won’t dive deep into how they work. They are
如前所述,这两种方法是创建数组的最常见方法,您将在99%的时间中看到并使用它们。 还有其他几种方法,但我们不会深入研究它们的工作方式。 他们是
the spread operator
const someArray = […someOtherArray]
散布运算符
const someArray = […someOtherArray]
the static method
Array.of()
静态方法
Array.of()
and the static method
Array.from()
和静态方法
Array.from()
读取,写入和更新阵列中的数据 (Reading, Writing and Updating data in an Array)
Reading, writing and updating element values in an array is just as simple as creating them. You can perform all three operations by using the square bracket syntax []
. Lets take a look at a few examples for each operation
读取,写入和更新数组中的元素值就像创建它们一样简单。 您可以使用方括号语法[]
来执行全部三个操作。 让我们看一下每个操作的一些示例
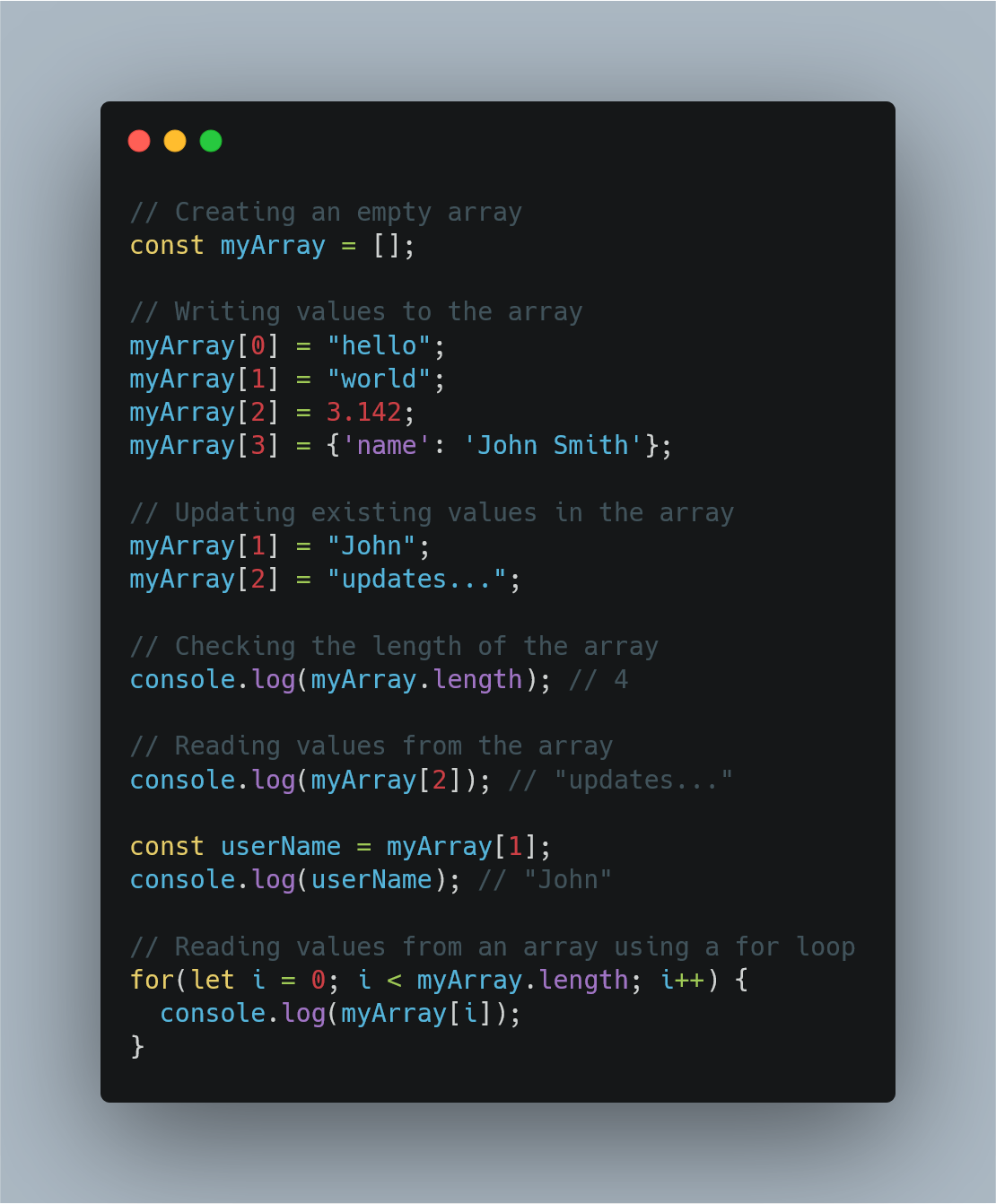
There are a few things to note here
这里有几件事要注意
- Array indexes start from 0 数组索引从0开始
- We’re placing values in the Array using specific indexes 我们使用特定索引在数组中放置值
Every time we add a value to the array its
length
property is updated每次我们向数组添加值时,其
length
属性都会更新- Using an existing index changes/updates the value of that index 使用现有索引更改/更新该索引的值
Note: JavaScript arrays don’t have an “out of bounds” error. When you try to access an index that doesn’t exist, you don’t get an error; you simply get undefined
.
注意: JavaScript数组没有“越界”错误。 当您尝试访问不存在的索引时,不会出现错误; 您只是 undefined
。
从阵列中删除 (Deleting from an Array)
This one’s pretty simple and straight forward. You can delete array elements with the delete
operator. For the delete operator you need to tell the index of the element you want to delete so it’ll be something like this:
这很简单直接。 您可以使用delete
运算符删除数组元素。 对于delete运算符,您需要告诉您要删除的元素的索引 ,这样它将类似于以下内容:
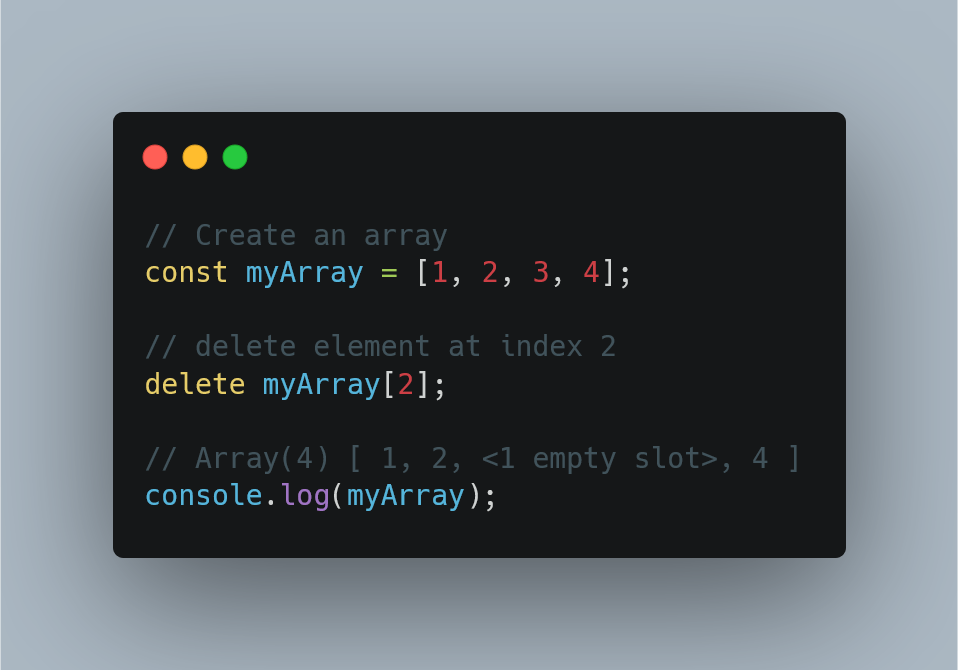
Note: When deleting an element from an array with the delete
operator it makes the array sparse
. This means that the length
property of the array remains the same as it was before the deletion. Making the array sparse also means that the elements of the array do not get shifted over to fill the gap.
注意: 使用 delete
运算符 从数组中删除元素时, 会使数组变 sparse
。 这意味着 数组 的 length
属性保持与删除前相同。 使数组稀疏还意味着数组的元素 不会 移位以填补空白。
数组类中的方法 (Methods from the Array Class)
In the previous section we took a look at some of the basic operations that you can perform with Arrays. Now we’ll be diving into some of the useful methods that are available in the Array class and taking a look at how some of these are implemented internally as well. These methods range from iterating over the array to inserting, removing and finding elements from the array. The list of methods in this post is by no means a full list of the methods that are at your disposal, instead it’s a list of some that I find that are the most commonly used methods.
在上一节中,我们介绍了可以对数组执行的一些基本操作。 现在,我们将深入研究Array类中可用的一些有用方法,并了解其中的一些内部实现方式。 这些方法的范围从遍历数组到从数组中插入,删除和查找元素。 这篇文章中的方法列表绝不是您可以使用的方法的完整列表,而是一些我认为是最常用的方法的列表。
forEach()
方法 (The forEach()
Method)
The forEach()
method loops through the given array and invokes the function you specify over each element of the array. Here’s an example.
forEach()
方法循环遍历给定的数组,并在数组的每个元素上调用您指定的函数。 这是一个例子。
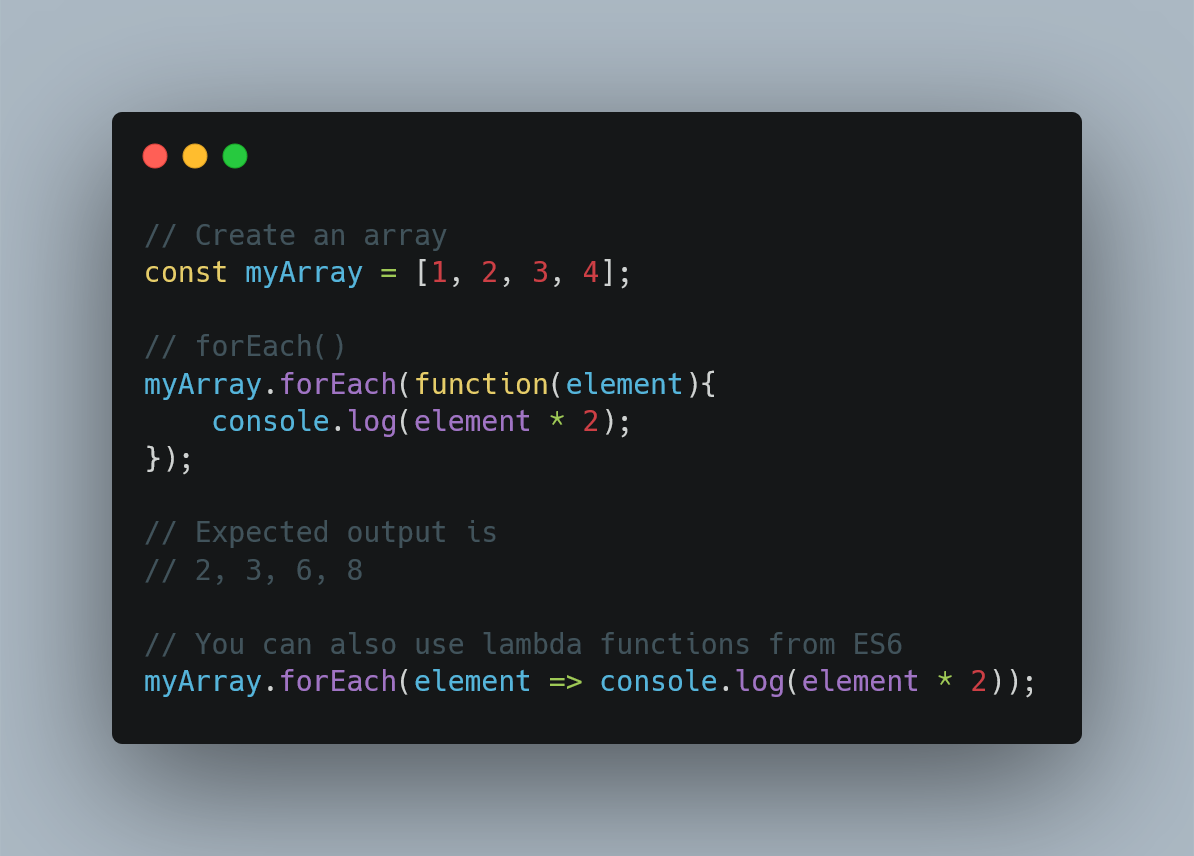
Note: The callback function you pass to the forEach()
method can take 3 arguments. First is the current element, second is the current index and the third is the array itself. More often you’ll only see the first two arguments being used.
注意: 传递给 forEach()
方法 的回调函数 可以使用3个参数。 第一个是当前元素,第二个是当前索引,第三个是数组本身。 通常,您只会看到正在使用的前两个参数。
One thing to keep in mind for this method is that it doesn’t allow you to terminate the loop before all elements are iterated over and that’s because of how the method itself is implemented.
该方法要记住的一件事是,它不允许您在所有元素都被迭代之前终止循环,这是因为方法本身是如何实现的。
Lets take a look one level deeper to see how this method is implemented.
让我们更深入地了解一下如何实现此方法。
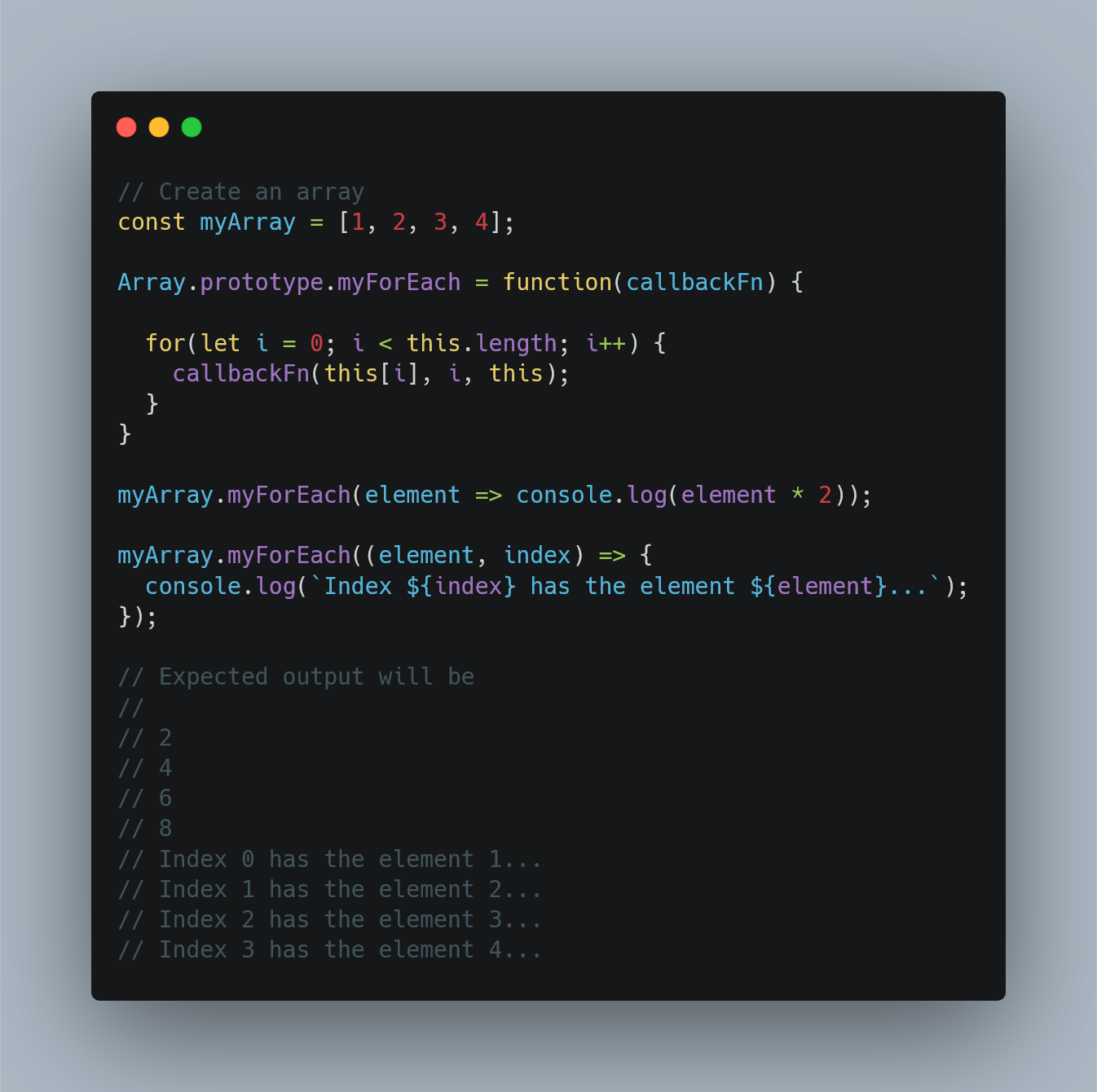
forEach()
method in JavaScript
forEach()
方法的示例
In the example above I created the myForEach()
method inside Array.prototype and it accepts a callback function. The usage of this new method is exactly the same as the regular forEach()
method and you can see that inside the method there’s no way of breaking the loop.
在上面的示例中,我在Array.prototype内创建了myForEach()
方法,该方法接受回调函数。 此新方法的用法与常规的forEach()
方法完全相同,并且您可以看到该方法内部没有中断循环的方法。
map()方法 (The map() Method)
The map()
method is similar to the forEach()
method in that it passes each element of the array to the callback function you pass to it. This time, the callback function you define should return a value. The map()
method then returns an array of the values your callback function returns.
map()
方法类似于forEach()
方法,因为它将数组的每个元素传递给您传递给它的回调函数。 这次,您定义的回调函数应返回一个值。 然后, map()
方法返回您的回调函数返回的值的数组。
Note: The map()
method does not modify the existing array but instead returns a brand new array that holds the modified values. Also if the original array is sparse, your function will not be called for the missing elements, but the returned array will be sparse in the same way as the original array.
注意: map()
方法不修改现有的阵列而是返回保持所述修改后的值一个全新的阵列。 同样,如果原始数组是稀疏的,则不会为缺少的元素调用函数,但是返回的数组将以与原始数组相同的方式稀疏。
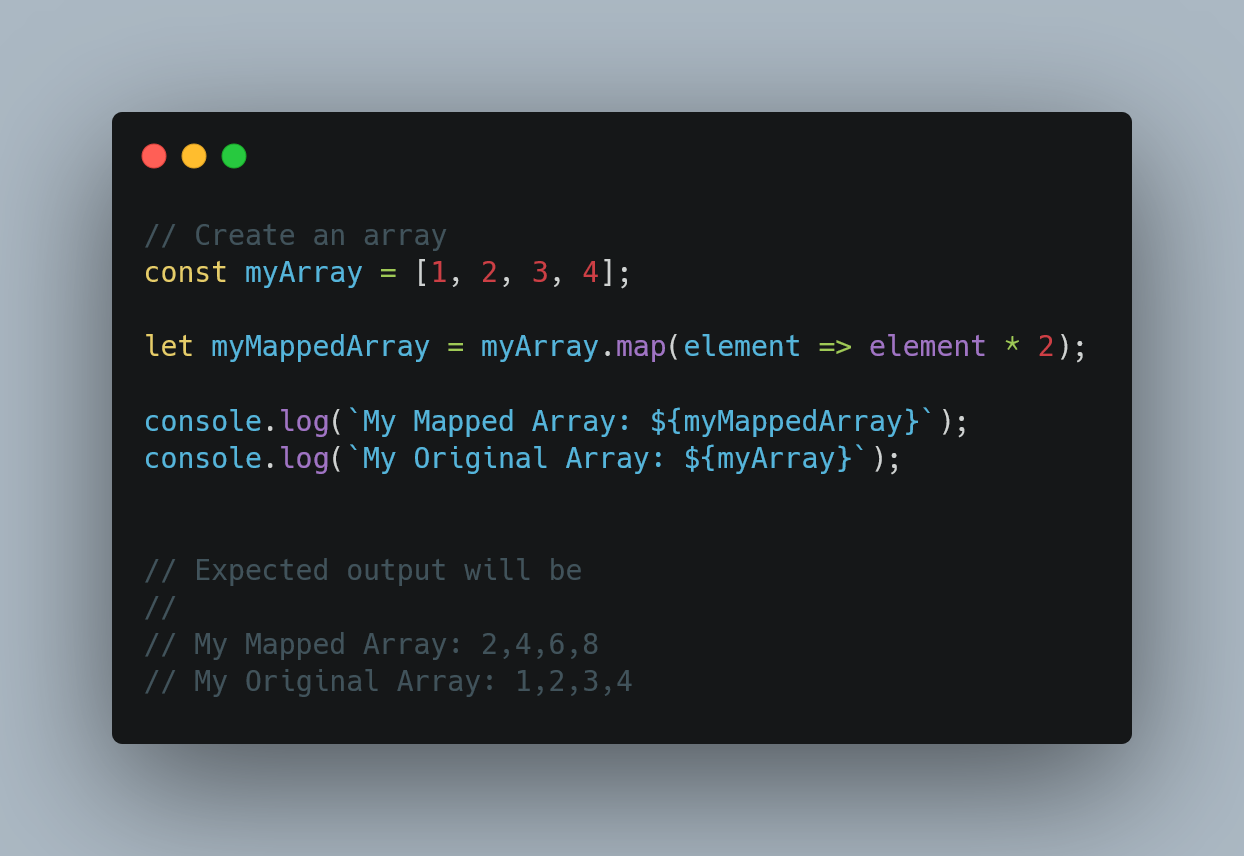
The internals of the map()
method are very similar to the ones of the forEach()
method but now the difference is that the values that your callback function returns are being stored in another array internally. Once the map()
method is done iterating over the values of your array, the new array with the modified values is returned. Here’s what it would look like internally.
map()
方法的内部与forEach()
方法的内部非常相似,但是现在的区别是回调函数返回的值在内部存储在另一个数组中。 一旦map()
方法完成了对数组值的迭代,将返回带有修改后值的新数组。 这是内部的样子。
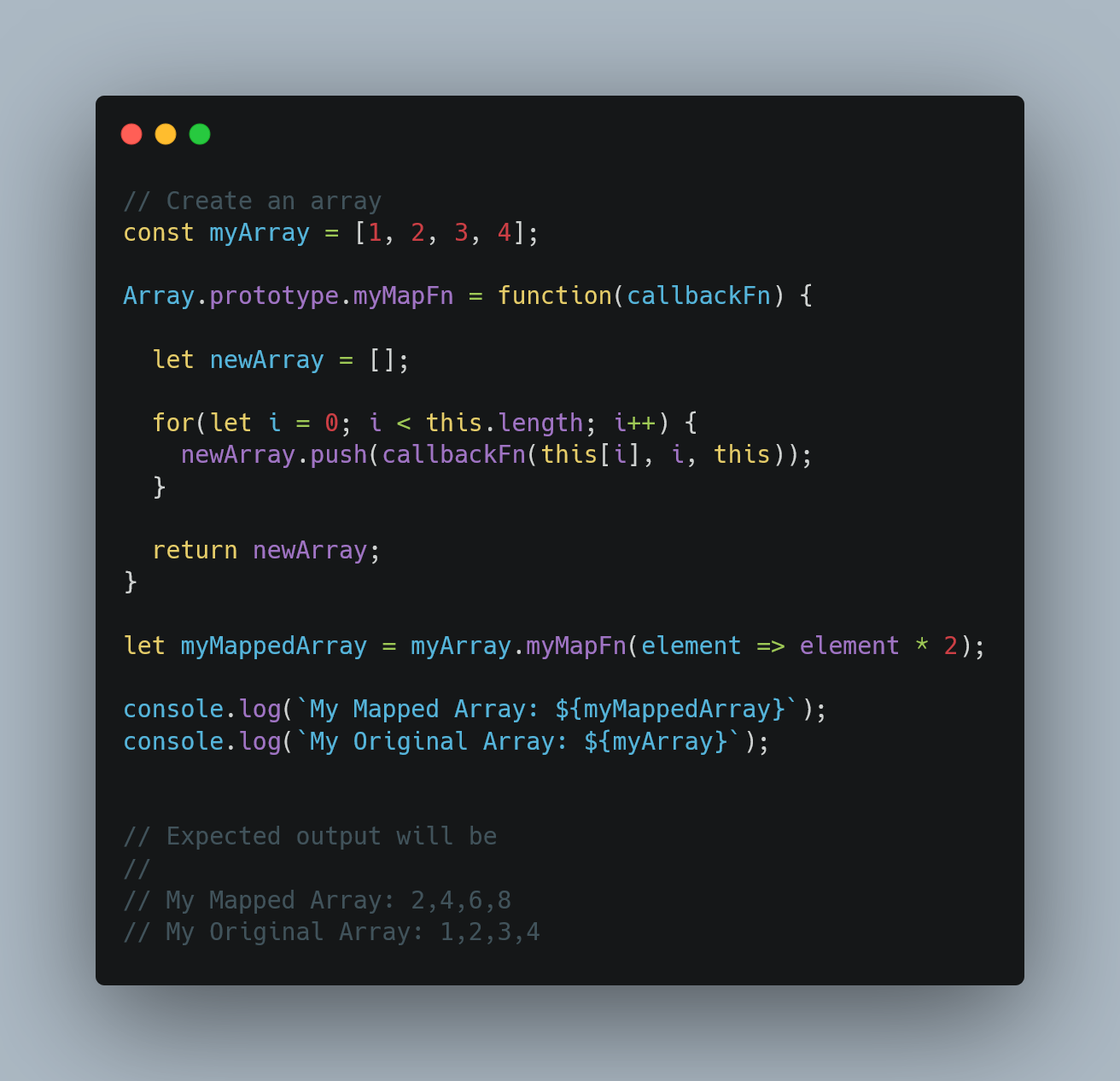
map()
method in JavaScript
map()
方法的示例
filter()方法 (The filter() Method)
Much like the map()
method the filter()
method also returns an array. The difference between the array returned by the filter()
method is that it’s a subset of the original array. Like the forEach()
and map()
methods, the filter()
method also takes a callback function. This callback function should be a predicate
i.e. should return a value that’s true
or false
. If the condition equates to true then the element that’s passed to the predicate is added to the subset array that’ll be the return value of the filter()
method.
与map()
方法非常相似, filter()
方法也返回一个数组。 filter()
方法返回的数组之间的区别是它是原始数组的子集。 与forEach()
和map()
方法一样, filter()
方法也采用回调函数。 此回调函数应为predicate
即应返回true
或false
的值。 如果条件等于true,则将传递给谓词的元素添加到子集数组中,该子集数组将成为filter()
方法的返回值。
Note: The filter() method does not modify the original array but instead returns a new array that’s a subset of the original.
注意: filter()方法不会修改原始数组,而是返回一个新数组,该数组是原始数组的子集。
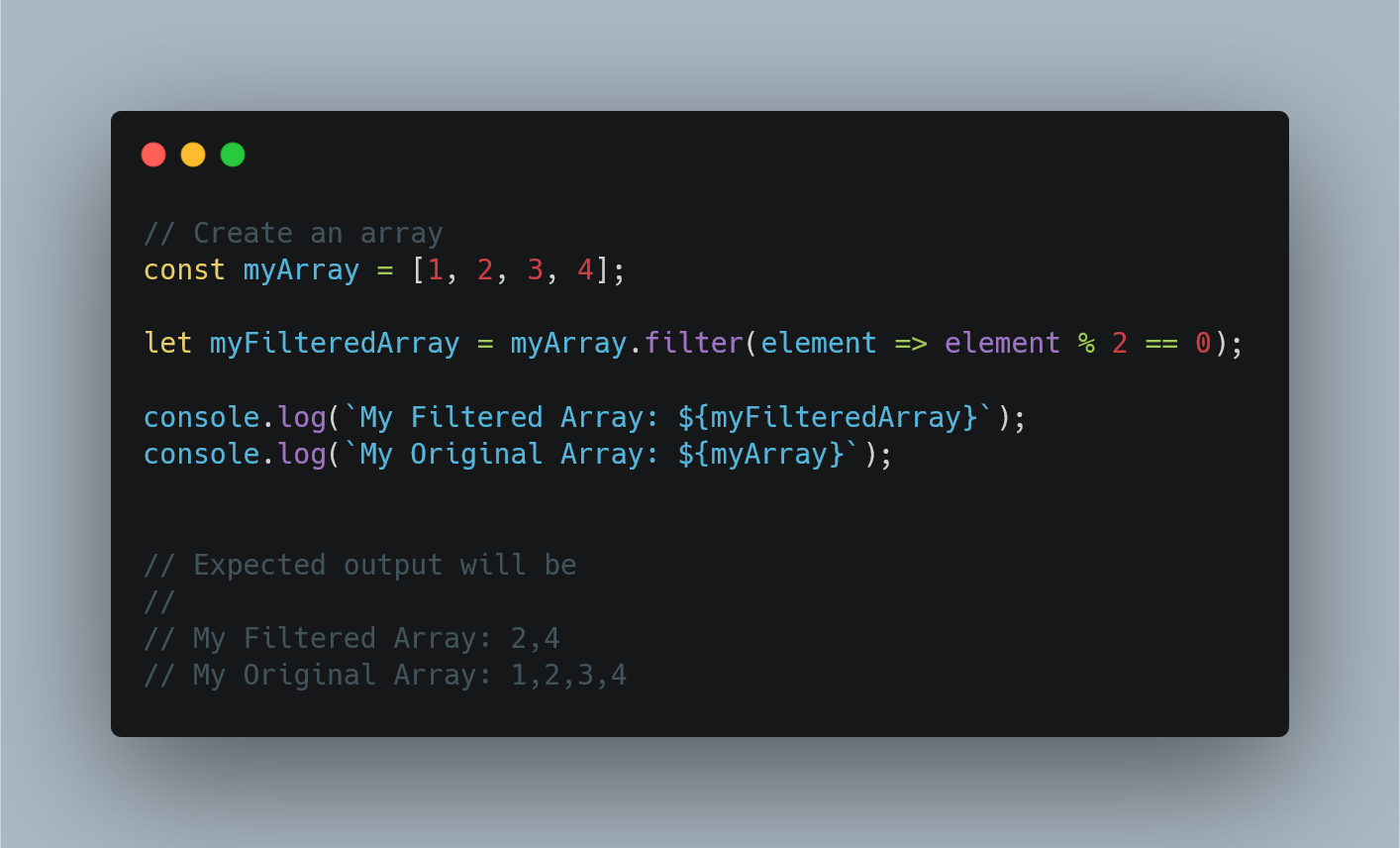
Now, the internals of the filter() method are very similar to that of the map() method. The difference is that there’s a condition based on which the element gets added to the internal array that represents a subset of the original array. Here’s something what it would look like.
现在,filter()方法的内部与map()方法的内部非常相似。 不同之处在于存在一个条件,根据该条件元素可以添加到表示原始数组的子集的内部数组中。 这是它的样子。
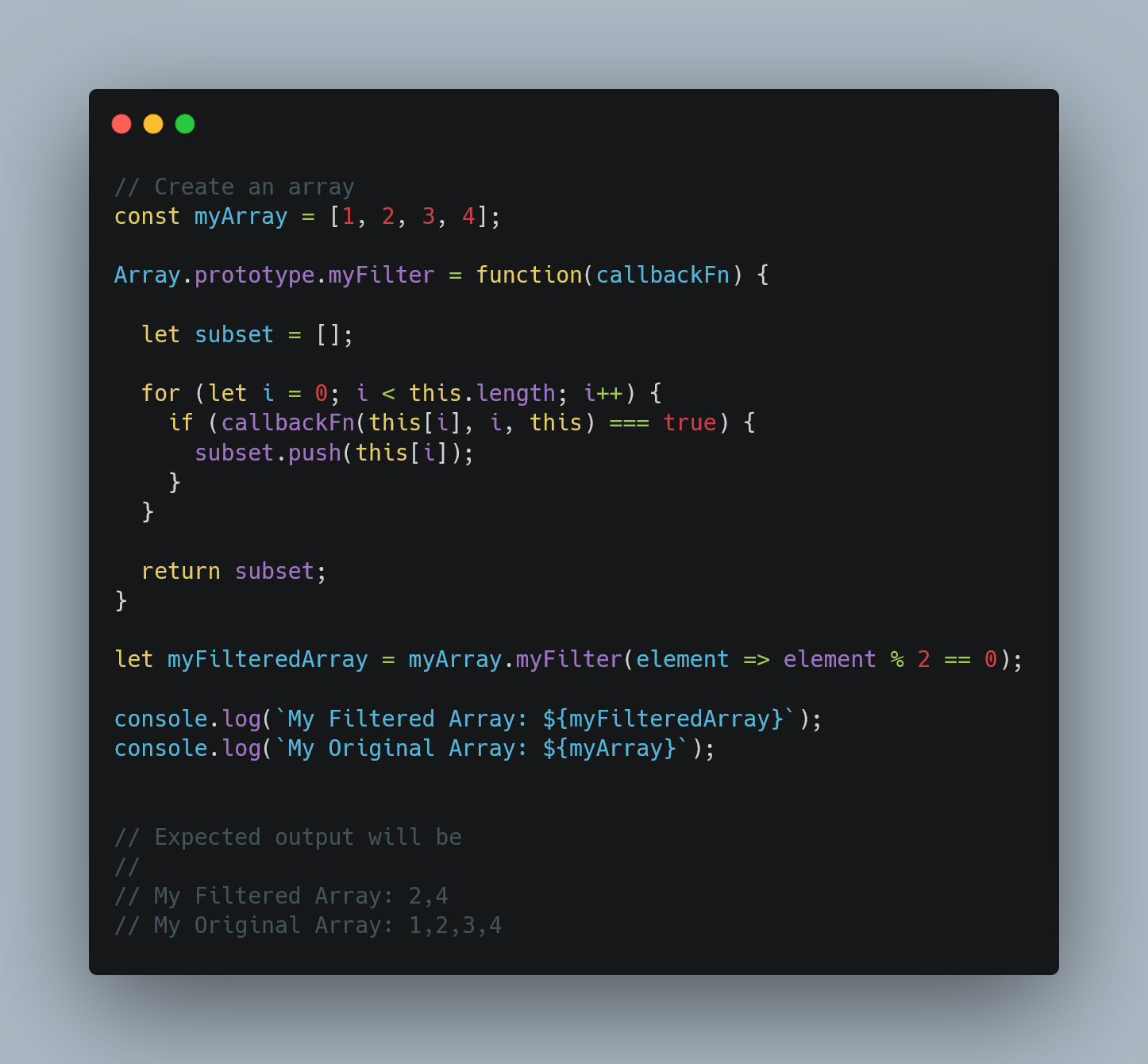
filter()
method in JavaScript
filter()
方法的示例
reduce()方法 (The reduce() Method)
The reduce()
method is used to combine the elements of the array using the function you pass in to produce a single value. The method takes two parameters. The first one is the function you want to use that performs the reduction operation and the second (optional) one is the starting value to use.
reduce()
方法用于使用传入的函数合并数组的元素以生成单个值。 该方法有两个参数。 第一个是要使用的执行归约运算的函数,第二个(可选)是要使用的起始值。
When you call the reduce()
method without the second parameter i.e. without an initial value to start from, it uses the first element of the array as the initial value and the second element of the array is used as the current value.
当您在没有第二个参数(即没有初始值reduce()
情况下调用reduce()
方法时,它将使用数组的第一个元素作为初始值,而将数组的第二个元素用作当前值。
The callback function you pass to the reduce()
method is a bit different from the ones you pass in the previous methods discussed. For the reduce()
method, the first argument of the callback function is the accumulated value and the second, third and fourth parameters are the element, index and array respectively. More often than not you’ll only be using the first two values i.e. the accumulated value and the current element being processed.
您传递给reduce()
方法的回调函数与您在前面讨论的方法中传递的回调函数有些不同。 对于reduce()
方法,回调函数的第一个参数是累加值,第二,第三和第四个参数分别是元素,索引和数组。 通常,您只会使用前两个值,即累计值和正在处理的当前元素。
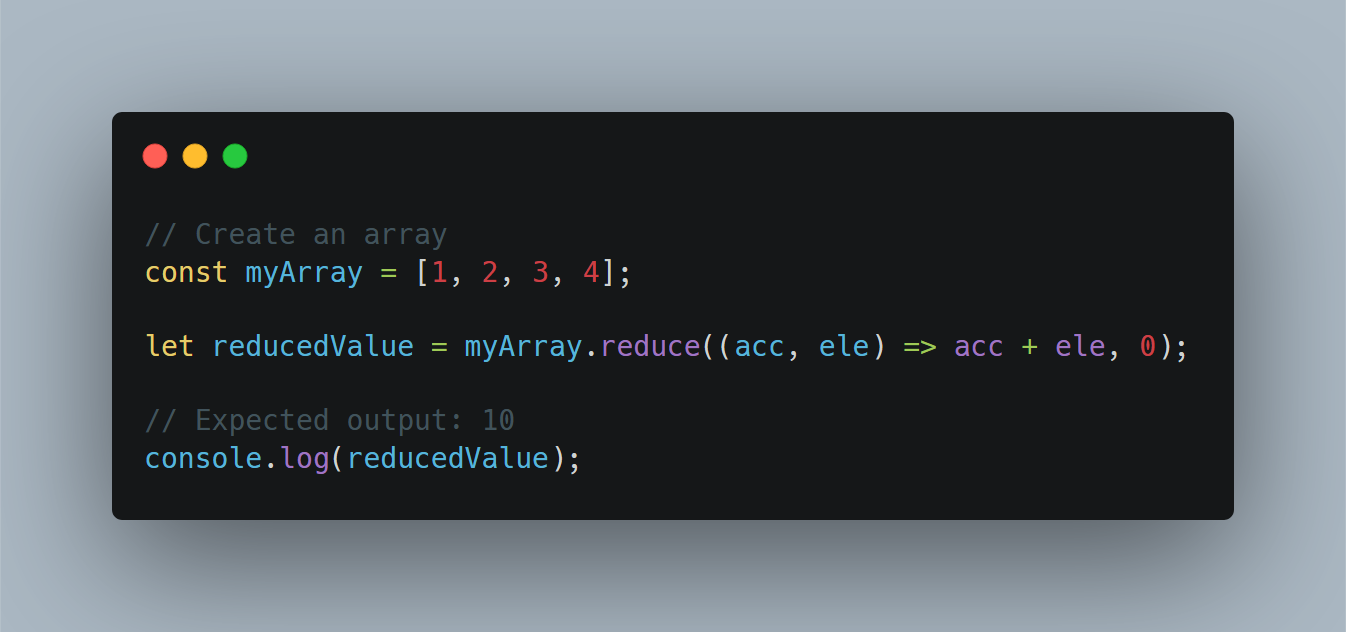
Lets step through how this works.
让我们逐步了解它的工作原理。
In the first call acc is 0
(because we passed in 0 as the initial value) and ele is 1
(because it’s the first element of the array) so it computes 0+1=1
and returns 1. Then acc is 1
and ele is 2
so it computes 1+2=3
. After that acc is 3
and ele is 3
so it computes 3+3=6
. Finally, acc is 6
and ele is 4
so it computes 6+4=10
. At this point there are no more values to accumulate i.e. no more elements in the array so the return value for the reduce()
method becomes 10.
在第一次调用中, acc is 0
(因为我们传入了0作为初始值),而ele is 1
(因为它是数组的第一个元素),因此它计算0+1=1
并返回1。然后acc is 1
而ele is 2
因此它计算1+2=3
。 之后, acc is 3
, ele is 3
因此它计算3+3=6
。 最后, acc is 6
, ele is 4
所以它计算6+4=10
。 此时,没有更多的值可累积,即数组中没有更多的元素,因此reduce()
方法的返回值变为10。
Lets dive a bit deeper and take a look at how this reduce()
method can be implemented.
让我们再深入一点,看看如何实现reduce()
方法。
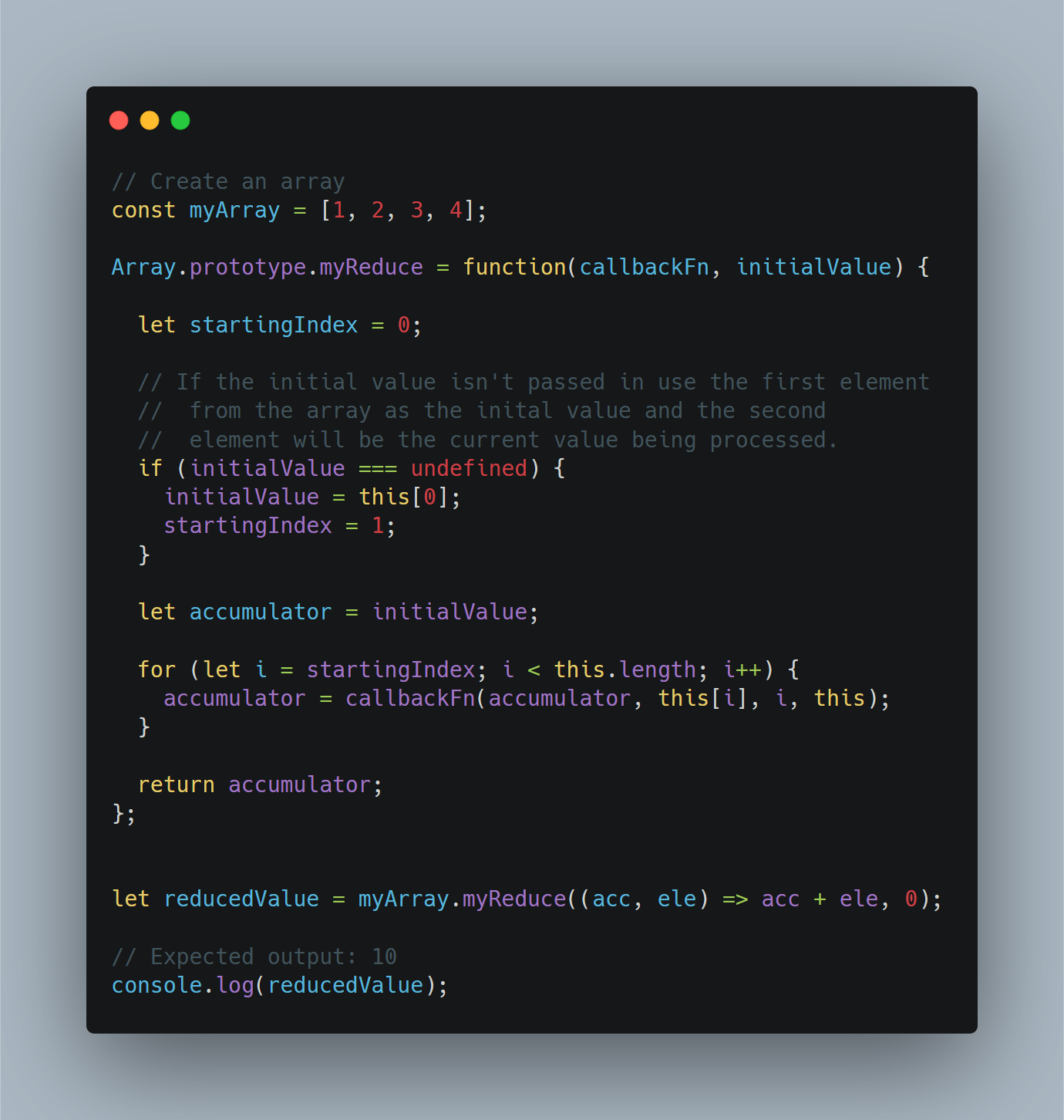
redu
ce() method in JavaScript
redu
ce()方法的示例
push()和pop()方法 (The push() and pop() Methods)
These two methods allow you to use arrays like Stacks. Stacks follow the LIFO protocol which means Last In First Out. The push()
method inserts one or more values to the end of the array and returns the new length. Whereas the pop()
method returns the last value of the array, decreases the length and deletes that last value from the array.
这两种方法使您可以使用堆栈之类的数组。 堆栈遵循LIFO协议,这意味着后进先出。 push()
方法将一个或多个值插入数组的末尾并返回新的长度。 pop()
方法返回数组的最后一个值,减小长度并从数组中删除该最后一个值。
Note: Both of these methods modify the array in place and don’t create a copy of it like what we saw in map()
and filter()
.
注意: 这两种方法都可以在适当的位置修改数组,并且不会像在 map()
和 filter()
看到的那样创建数组的副本 。
Lets take a look at how each one works and then have a look at how each one might be implemented internally.
让我们看一下每个人的工作方式,然后看看每个人如何在内部实现。
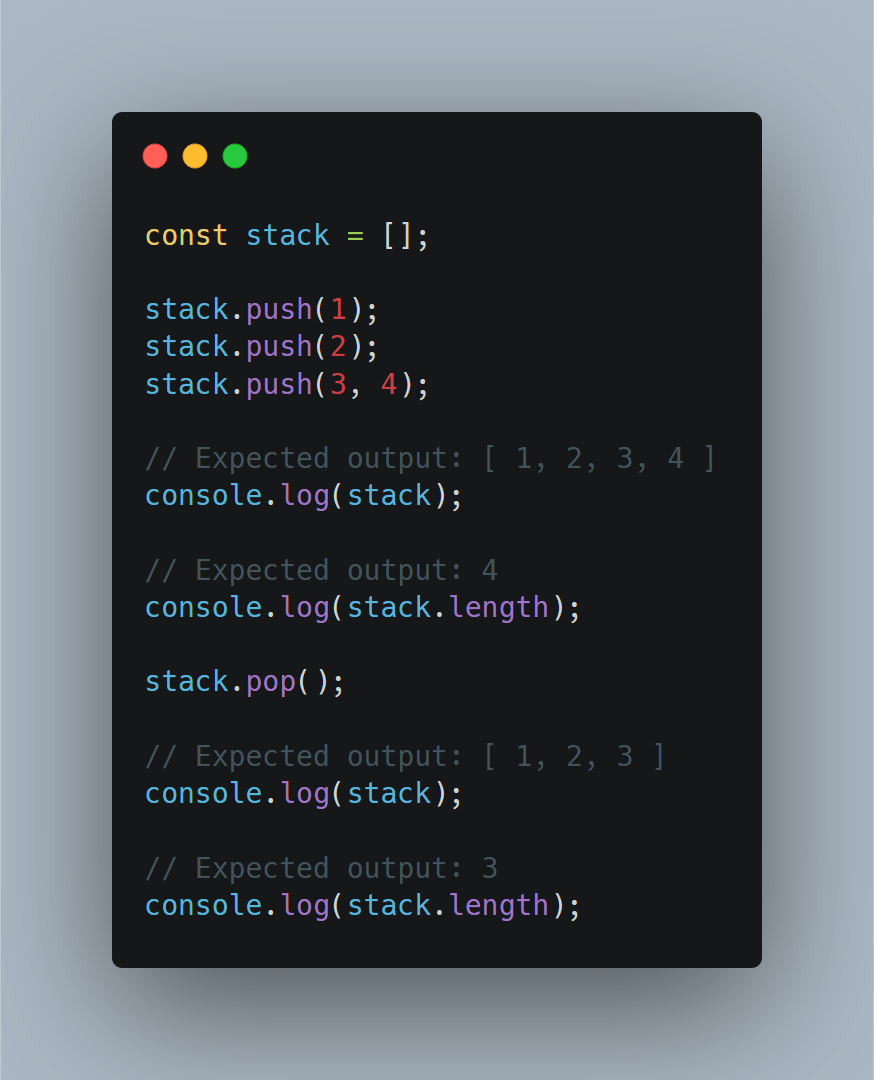
Lets now take a look at how these may be implemented internally.
现在让我们看一下如何在内部实现这些功能。
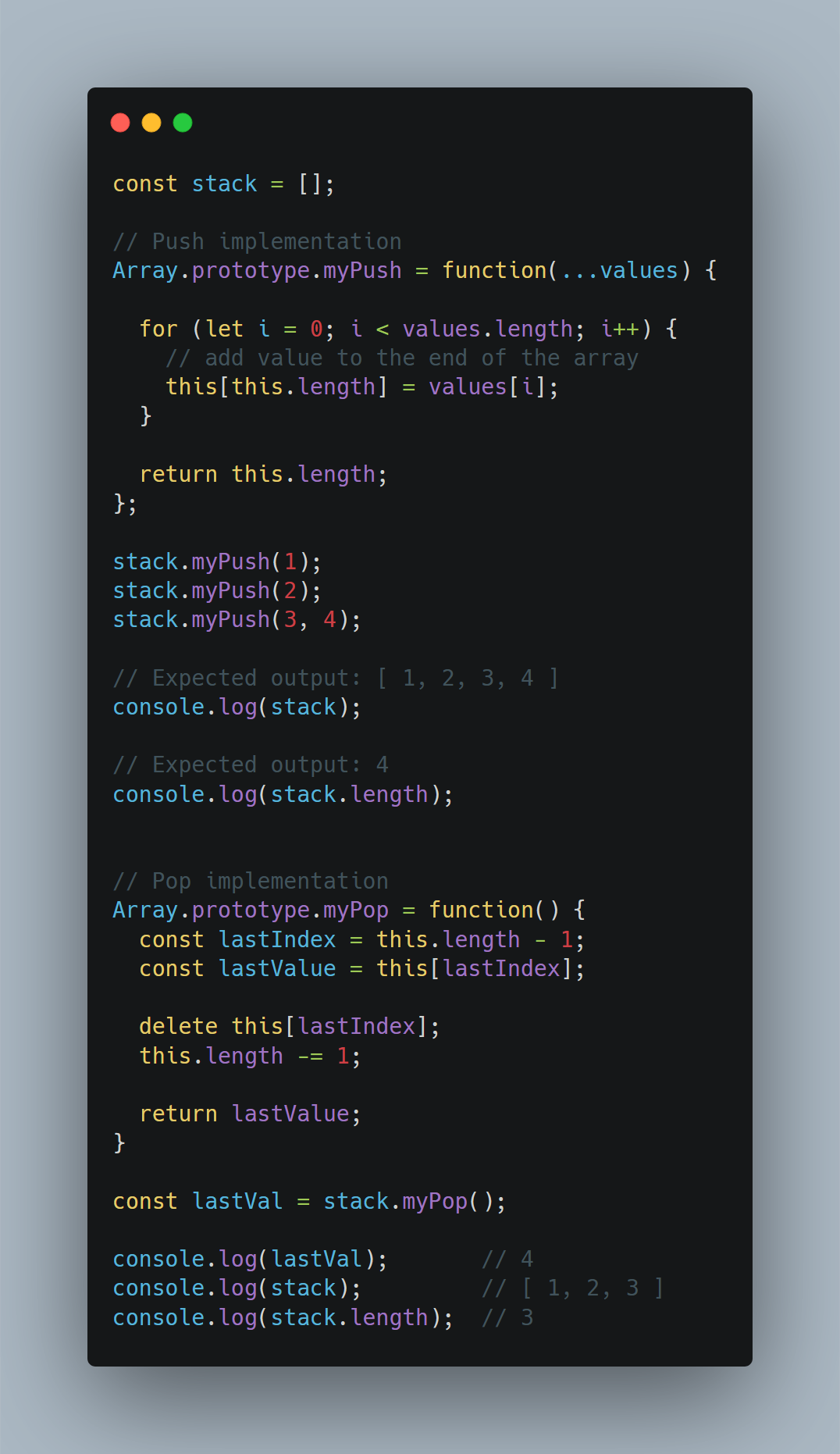
结论 (Conclusion)
This, by no means, is an exhaustive list of the methods that are available in the Array class but it is a list of the most used methods. In this post we looked at some basic CRUD operations that you can perform with Arrays and we also dove a bit deeper in to some of the most commonly used methods from the Array class and how they’re implemented. Keep in mind that the Array is a data structure that’s common to all programming languages so what you learned here can be applied to other languages as well.
这绝不是Array类中可用方法的详尽列表,但它是最常用方法的列表。 在本文中,我们研究了一些可以使用Arrays执行的基本CRUD操作,并且还深入研究了Array类中一些最常用的方法以及它们的实现方式。 请记住,数组是所有编程语言都通用的数据结构,因此您在这里学到的知识也可以应用于其他语言。
Well, that’s all for now. Until next time, peace ✌️
好吧,现在就这些。 直到下次,和平peace️
翻译自: https://medium.com/weekly-webtips/a-modest-guide-to-javascript-arrays-502b5a708acc
javascript指南