I’ve been looking for a basic tutorial like this for quite some time, but I haven’t found one. I eventually figured out how to set up everything on my own, so I thought I could share it here. I’m not going to go over the benefits or anything with Typescript in this tutoria, but I am going to also show you how to additionally get set up with Eslint and Prettier as well, since it’s the way I think you should do it. But it comes later, so don’t worry about it now.
我一直在寻找像这样的基本教程,但是没有找到。 我最终想出了如何自行设置所有内容的方法,因此我想可以在这里共享它。 我不会在这个托里亚语中讨论使用Typescript带来的好处或任何其他问题,但是我还将向您展示如何另外使用Eslint和Prettier进行设置,因为这是我认为应该采用的方法。 但它来得晚,所以现在不用担心。
第1部分:使用Typescript设置Express (Part 1: Setting up Express with Typescript)
1.安装依赖项 (1. Install dependencies)
npm install express && npm install --save-dev typescript nodemon @types/node @types/express
2.添加package.json脚本和主字段 (2. Add package.json scripts and main field)
After installing the typescript package as a dev dependency, update your package.json script block with these 3 commands. Also, we need to set our main file to be the output of the code Typescript compiles for us, by setting the main field accordingly.
在将typescript软件包安装为dev依赖项之后,请使用这3个命令更新package.json脚本块。 另外,我们需要通过相应地设置主字段,将主文件设置为Typescript为我们编译的代码的输出。
"main": "dist/index.js",
"scripts": {
"build": "tsc",
"start": "tsc & node .",
"dev": "tsc -w & nodemon .",
}
3.添加一个tsconfig.json文件 (3. Add a tsconfig.json file)
One last step, make sure you create a tsconfig.json file in the root of your project. If you don’t create this Typescript won’t know what options to use and how to properly type your project.
最后一步,请确保您在项目的根目录中创建了tsconfig.json文件。 如果您不创建此Typescript,则不会知道要使用哪些选项以及如何正确键入项目。
{
"compilerOptions": {
"module": "commonjs",
"esModuleInterop": true,
"target": "es6",
"noImplicitAny": true,
"moduleResolution": "node",
"sourceMap": true,
"outDir": "dist",
"baseUrl": ".",
"paths": {
"*": ["node_modules/*"]
}
},
"include": ["src/**/*"]
}
4.创建主服务器文件 (4. Create main server file)
Now, your project should have support for using Typescript. Create the main file inside of a src folder that’s going to be the center of the project.
现在,您的项目应该支持使用Typescript。 在src文件夹中创建主文件,该文件夹将成为项目的中心。
mkdir src && touch src/index.ts
Add the following code to index.ts
将以下代码添加到index.ts
import express from 'express'
const app = express()
const port = 5000app.get('/', (_, res) => {
res.status(200).send()
})app.listen(port, () => console.log(`Running on port ${port}`))
5.运行服务器 (5. Run server)
Now you should simply be able to start your project by running the following command inside the root of the project. This will run Typescript in watch-mode, and by using nodemon, enable auto restarting of our server.
现在,您只需在项目根目录内运行以下命令即可启动您的项目。 这将以监视模式运行Typescript,并通过使用nodemon启用服务器的自动重启。
npm run dev
第2部分:设置Eslint和更漂亮 (Part 2: Setting up Eslint and Prettier)
Now that we have Express set up properly with Typescript, we might also want linting through Eslint, and code formatting with Prettier. Traditionally this has been a bit of a pain in the rear to set up properly, but just follow along and you’ll see it’s not that difficult. We’re going to start off by installing Eslint and Prettier, along with a couple of plugins to bind everything together.
现在我们已经使用Typescript正确设置了Express,我们可能还希望通过Eslint进行整理,并使用Prettier进行代码格式化。 传统上,正确设置后部会有些痛苦,但是只要坚持下去,您就会发现这并不困难。 我们将从安装Eslint和Prettier以及几个将所有内容绑定在一起的插件开始。
1.安装其他依赖项 (1. Install additional dependencies)
npm install --save-dev eslint prettier eslint-config-prettier eslint-plugin-prettier @typescript-eslint/parser @typescript-eslint/eslint-plugin
2.设置Eslint (2. Set up Eslint)
Run the Eslint configuration wizard by running the global eslint command through npx like this. This will ask you a couple of questions, and then create an initial configuration file in the root of your project.
通过像这样通过npx运行global eslint命令来运行Eslint配置向导。 这将问您几个问题,然后在项目的根目录中创建一个初始配置文件。
npx eslint --init
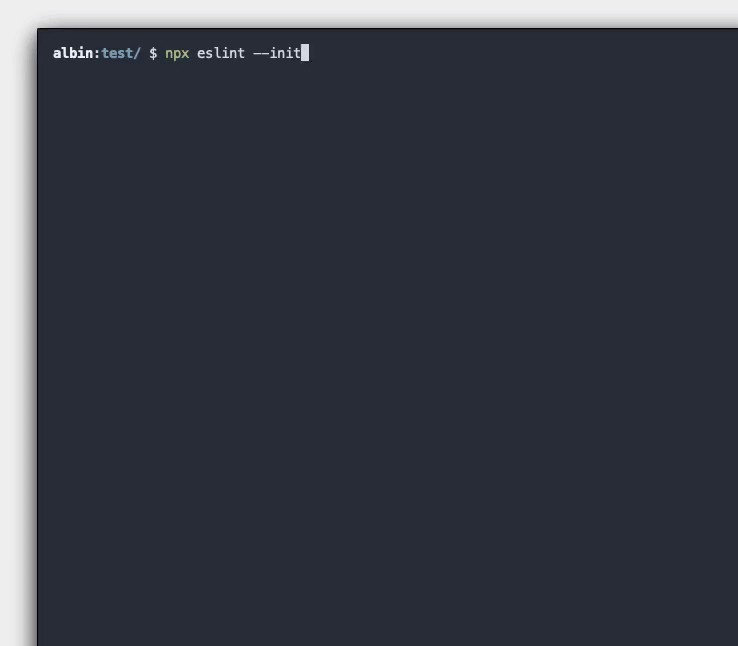
3.更新Eslint配置文件 (3. Update Eslint configuration file)
Now you need to update the Eslint configuration file that got generated from the previous commadnd (The file is going to be called .eslintrc), to both use the Typescript and Prettier plugins we installed.
现在,您需要更新从上一个命令生成的Eslint配置文件(该文件将称为.eslintrc ),以使用我们安装的Typescript和Prettier插件。
/* eslint-disable */
module.exports = {
env: {
browser: true,
es2020: true,
},
extends: ['eslint:recommended', 'plugin:@typescript-eslint/recommended'],
parser: '@typescript-eslint/parser',
parserOptions: {
ecmaVersion: 11,
sourceType: 'module',
},
plugins: ['@typescript-eslint', 'prettier'],
rules: { 'prettier/prettier': [
1,
{
trailingComma: 'es5',
singleQuote: true,
semi: false,
},
], ...require('eslint-config-prettier').rules,
...require('eslint-config-prettier/@typescript-eslint').rules,
},
}
4.瞧! (4. Voila!)
If you have done everything correctly, you should now for example get a Eslint warning if you use double quotes instead of single quotes — since that’s what we specified in our Eslint configuration file under the Prettier plugin section.
如果您已正确完成所有操作,则现在应使用双引号而不是单引号来得到Eslint警告,因为这是我们在Prettier插件部分的Eslint配置文件中指定的。
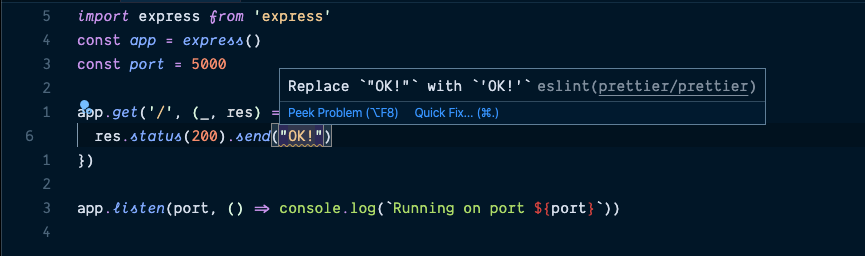
而已! (That’s it!)
You have now properly set up Express, Typescript, Eslint and Prettier without using any third part generator or templates that comes with a bunch of overhead. In the future, you will now have an example project that you can refer to when setting up new ones. Even better, throw it up on a source control provider and choose to save it as template.
现在,您已经正确设置了Express,Typescript,Eslint和Prettier,而无需使用任何第三方生成器或模板,而这会带来大量开销。 将来,您将有一个示例项目,可以在设置新项目时参考该项目。 更好的是,将其放在源代码控制提供程序上,然后选择将其另存为模板。
I hope this guide has been useful to you, and that everything went smoothly. If you have any questions or feedback whatsoever, don’t hesitate to leave a comment below, or reach out to me personally, and I’ll try to help you out.
希望本指南对您有所帮助,并且一切顺利。 如果您有任何疑问或反馈,请随时在下面发表评论,或亲自与我联系,我会尽力帮助您。
翻译自: https://levelup.gitconnected.com/how-to-properly-set-up-express-with-typescript-1b52570677c9