钢结构节点板计算小程序
One of the challenges when creating your first few Node based applications is determining where to put the files your code resides in. It is a challenge because there are very few “do it this way” statements. Instead there are recommendations and guidelines, or the tutorials do it a certain way without explaining why.
创建最初的几个基于Node的应用程序时面临的挑战之一就是确定将代码驻留在何处。这是一个挑战,因为很少有“以这种方式执行”语句。 取而代之的是提供建议和指南,或者教程以某种方式执行此操作而不解释原因。
The thing with Node is that it actually favours the software design patterns and design principles. Applying these patterns and principals to our own applications is a great idea as well. I have found that when I stray from the proven principles (where they would be applicable) my code tends to get “ugly” fast (more complex, harder to work with, harder to maintain, etc.)
Node的好处是实际上它偏爱软件设计模式和设计原则 。 将这些模式和主体应用于我们自己的应用程序也是一个好主意。 我发现,当我偏离行之有效的原则(适用于这些原则)时,我的代码往往变得“丑陋”(更复杂,更难以使用,更难以维护等)。
The modules you choose to use may suggest a method for organising the files too. For example, using Express to create a non-trivial application almost always ends up with a “routes” folder. Using Knex.JS often results in a “models” and “migrations” folders. These modules can work in harmony with the common design patterns precisely because they are extensively used and improved.
您选择使用的模块也可能会建议一种组织文件的方法。 例如,使用Express创建非平凡的应用程序几乎总是以“ routes”文件夹结尾。 使用Knex.JS通常会导致一个“模型”和“迁移”文件夹。 这些模块可以被广泛使用和改进,因此可以与常见的设计模式协调一致地工作。
When we are starting out the only “real” folder we need is a project directory. This is where we put the package.json
file and any other files for our application. We often see the base structure created with commands something like this:
当我们启动唯一的“真实”文件夹时,我们需要的是项目目录。 这是我们将package.json
文件和其他文件放入应用程序的位置。 我们经常看到用以下命令创建的基本结构:
mkdir myProject
cd myProject
npm init -y
Convention comes into play here too. Most Node projects will have a src
directory right beside the project.json
file. A lot of libraries and tools expect this, but Node itself does not say this is absolutely required. Convention can be a good thing — it brings a common understanding to the table allowing easier communication of ideas. In some cases it can make “magic” happen, but only if done a certain way. But conventions can sometimes clash with application requirements or even other modules that are needed.
约定在这里也起作用。 大多数Node项目将在project.json
文件旁边有一个src
目录。 许多库和工具都期望这样做,但是Node本身并没有说这是绝对必要的。 约定可能是一件好事–它使大家有了共识,可以更轻松地交流思想。 在某些情况下,它可以使“魔术”发生,但前提是一定要这样做。 但是约定有时可能会与应用程序需求甚至其他所需的模块发生冲突。
“The nice thing about standards is that you have so many to choose from” — Andrew Tanenbaum
“关于标准的好处是您可以选择的太多了” — Andrew Tanenbaum
With Node we work with modules a good deal. A module requires other modules as needed. The required module is either an installed module — require('express')
Or it is a path within our system, identified when the first character is a dot require('../models/widget.js')
The path to the desired module is relative to the current module. Where needed, the builtin path module can be used to ensure a proper path is used (and cross platform as well).
使用Node,我们可以处理很多模块。 一个模块根据需要需要其他模块。 必需的模块可以是已安装的模块require('../models/widget.js')
require('express')
或它是我们系统内的路径,当第一个字符是点时被标识require('../models/widget.js')
所需路径模块相对于当前模块。 如果需要,可以使用内置路径模块来确保使用正确的路径(以及跨平台)。
const path = require('path')
const WidgetModel = require(path.resolve(__dirname, '../models/widget.js'))
Because we specify the path to our module, the directory structure we choose is pretty much whatever we’d like. To avoid chaos though we apply the design principles and some common sense to suggest a reasonable directory structure that makes sense.
因为我们指定了模块的路径,所以我们选择的目录结构几乎是我们想要的。 为了避免混乱,我们采用了设计原则和一些常识来建议合理的目录结构。
For instance, when I create an Express based API that needs data access models, I might end up with a structure like this:
例如,当我创建一个需要数据访问模型的基于Express的API时,最终可能会得到如下结构:
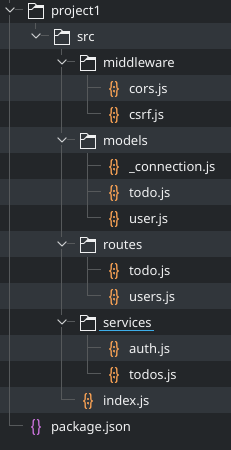
Here I have a routes and middleware folder to store some Express specific modules. Then I have a models directory to store my data access files. And finally I have the services directory to store my business logic. The phrase “services” may go by other names — “logic”, “business_intelligence”, etc. But “services” seems like a good collector phrase and has good adoption by the industry.
在这里,我有一个路由和中间件文件夹,用于存储一些Express特定模块。 然后,我有一个models目录来存储我的数据访问文件。 最后,我有了services目录来存储我的业务逻辑。 “服务”一词可能有其他名称-“逻辑”,“ business_intelligence”等。但是“服务”似乎是一个很好的收集器,并在业界得到了广泛采用。
The key here is to match your directory structure to the needs of your application. You know those needs best, so you’ll probably choose something meaningful. Applying design principles when possible can help define the needed structure as well. And finally, defining the common application layers may also assist in determining what folders are needed.
这里的关键是使目录结构与应用程序需求相匹配。 您最了解这些需求,因此您可能会选择一些有意义的东西。 尽可能应用设计原则也可以帮助定义所需的结构。 最后,定义公用应用程序层还可以帮助确定需要哪些文件夹。
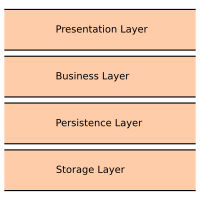
After you have built a few applications the directory structure you need will be come just one of those things you do without really thinking about it. As you work with other projects or frameworks, you’ll notice some conventions or recommendations that just make sense and you’ll adopt it where you can. (For example, my data access models were influenced by the Laravel model system. But that is a different topic.)
在构建了几个应用程序之后,您所需要的目录结构将成为您要做的其中一件事,而无需真正考虑它。 在处理其他项目或框架时,您会发现一些有意义的约定或建议,并会尽可能采用它。 (例如,我的数据访问模型受到Laravel模型系统的影响。但这是一个不同的主题。)
This has been a high level discussion, but here is hoping it helps you out some. Feel free to drop me a note if I can clarify/correct anything.
这是一个高级别的讨论,但是希望它能对您有所帮助。 如果我可以澄清/更正任何内容,请随时给我留言。
普通英语JavaScript (JavaScript In Plain English)
Enjoyed this article? If so, get more similar content by subscribing to Decoded, our YouTube channel!
喜欢这篇文章吗? 如果是这样,请订阅我们的YouTube频道解码,以获得更多类似的内容!
翻译自: https://medium.com/javascript-in-plain-english/node-application-directory-structure-dfe1ae97891d
钢结构节点板计算小程序