重点 (Top highlight)
As we all know, JavaScript has been changing rapidly. With the new ES2020, there are many awesome features introduced that you might want to checkout. To be honest, you can write code in many different ways. They might achieve the same goal, but some are shorter and much clearer. You can use some small tricks to make your code cleaner and clearer. Here is a list of useful tricks for JavaScript developers that will definitely help you one day.
众所周知,JavaScript一直在Swift变化。 借助新的ES2020,引入了许多很棒的功能,您可能想检出这些功能。 老实说,您可以用许多不同的方式编写代码。 他们可能会达到相同的目标,但其中一些会更短,更清晰。 您可以使用一些小技巧来使代码更清晰。 这里列出了一些对JavaScript开发人员有用的技巧,这些技巧一定会对您有所帮助。
方法参数验证 (Method Parameter Validation)
JavaScript allows you to set default values for your parameters. Using this, we can implement a neat trick to validate your method parameters.
JavaScript允许您设置参数的默认值。 使用此方法,我们可以实施一个巧妙的技巧来验证您的方法参数。
const isRequired = () => { throw new Error('param is required'); };const print = (num = isRequired()) => { console.log(`printing ${num}`) };print(2);//printing 2print()// errorprint(null)//printing null
Neat, isn’t it?
整洁,不是吗?
格式化JSON代码 (Format JSON Code)
You would be quite familiar with JSON.stringify
by now. But are you aware that you can format your output by using stringify
? It is quite simple actually.
JSON.stringify
,您将非常熟悉JSON.stringify
。 但是您是否知道可以使用stringify
格式化输出? 实际上,这很简单。
The stringify
method takes three inputs. The value
, replacer
, and space
. The latter two are optional parameters. That is why we did not use them before. To indent our JSON, we must use the space
parameter.
stringify
方法需要三个输入。 value
, replacer
和space
。 后两个是可选参数。 这就是为什么我们以前不使用它们。 要缩进JSON,我们必须使用space
参数。
console.log(JSON.stringify({name:"John",Age:23},null,'\t'));>>>
{
"name": "John",
"Age": 23
}
Here’s a React component I’ve published to Bit. Feel free to play with the stringify example.
从数组获取唯一值 (Get Unique Values From An Array)
Getting unique values from an array required us to use the filter
method to filter out the repetitive values. But with the new Set
native object, things are really smooth and easy.
从数组中获取唯一值要求我们使用filter
方法来过滤出重复值。 但是,有了新的Set
本机对象,事情就变得非常顺利和容易。
let uniqueArray = [...new Set([1, 2, 3, 3,3,"school","school",'ball',false,false,true,true])];>>> [1, 2, 3, "school", "ball", false, true]
从数组中删除虚假值 (Removing Falsy Values From Arrays)
There can be instances where you might want to remove falsy values from an array. Falsy values are values in JavaScript which evaluate to FALSE. There are only six falsy values in JavaScript and they are,
在某些情况下,您可能想从数组中删除伪造的值。 虚假值是JavaScript中的值为FALSE的值。 JavaScript中只有六个伪造的值,它们是,
undefined
undefined
null
null
NaN
NaN
0
0
“”
(empty string)“”
(空字符串)false
false
The easiest way to filter out these falsy values is to use the below function.
滤除这些虚假值的最简单方法是使用以下函数。
myArray
.filter(Boolean);
If you want to make some modifications to your array and then filter the new array, you can try something like this. Keep in mind that the original myArray
remains unchanged.
如果要对数组进行一些修改然后过滤新数组,则可以尝试这样的操作。 请记住,原始myArray
保持不变。
myArray
.map(item => {
// Do your changes and return the new item
})
.filter(Boolean);
合并多个对象 (Merge Several Objects Together)
I have had several instances where I had the need to merge two or more classes, and this was my go-to approach.
我有几个实例需要合并两个或多个类,这是我的首选方法。
const user = {
name: 'John Ludwig',
gender: 'Male'
};const college = {
primary: 'Mani Primary School',
secondary: 'Lass Secondary School'
};const skills = {
programming: 'Extreme',
swimming: 'Average',
sleeping: 'Pro'
};const summary = {...user, ...college, ...skills};
The three dots are also called the spread operator in JavaScript. You can read more of their uses over here.
这三个点在JavaScript中也称为散布运算符。 您可以在这里阅读更多它们的用法。
排序数字数组 (Sort Number Arrays)
JavaScript arrays come with a built-in sort method. This sort method converts the array elements into strings and performs a lexicographical sort on it by default. This can cause issues when sorting number arrays. Hence here is a simple solution to overcome this problem.
JavaScript数组带有内置的sort方法。 默认情况下,此sort方法将数组元素转换为字符串,并对其进行字典排序。 在对数字数组进行排序时,这可能会导致问题。 因此,这里是解决此问题的简单解决方案。
[0,10,4,9,123,54,1].sort((a,b) => a-b);>>> [0, 1, 4, 9, 10, 54, 123]
You are providing a function to compare two elements in the number array to the sort method. This function helps us the receive the correct output.
您正在提供一个将number数组中的两个元素与sort方法进行比较的函数。 此功能可帮助我们接收正确的输出。
禁用右键 (Disable Right Click)
You might ever want to stop your users from right-clicking on your web page. Although this is very rare, there can be several instances where you would need this feature.
您可能曾经想阻止您的用户右键单击您的网页。 尽管这种情况很少见,但是在某些情况下您可能需要此功能。
<body oncontextmenu="return false">
<div></div></body>
This simple snippet would disable right click for your users.
这个简单的代码段将为您的用户禁用右键单击。
使用别名进行销毁 (Destructuring with Aliases)
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables. Rather than sticking with the existing object variable, we can rename them to our own preference.
解构赋值语法是一个JavaScript表达式,可以将数组中的值或对象中的属性解压缩为不同的变量。 可以不使用现有的对象变量,而可以将其重命名为自己的首选项。
const object = { number: 10 };// Grabbing number
const { number } = object;// Grabbing number and renaming it as otherNumber
const { number: otherNumber } = object;console.log(otherNumber); //10
获取数组中的最后一项 (Get the Last Items in an Array)
If you want to take the elements from the end of the array, you can use the slice
method with negative integers.
如果要从数组的末尾获取元素,则可以对负整数使用slice
方法。
let array = [0, 1, 2, 3, 4, 5, 6, 7]
console.log(array.slice(-1));
>>>[7]
console.log(array.slice(-2));
>>>[6, 7]
console.log(array.slice(-3));
>>>[5, 6, 7]
等到承诺完成 (Wait Until Promises Are Complete)
There might be instances where you will need to wait for several promises to be over. We can use Promise.all
to run our promises in parallel.
在某些情况下,您将需要等待多个承诺结束。 我们可以使用Promise.all
并行履行承诺。
Note: Promises will run concurrently in a single-core CPU and will run in parallel in multi-core CPU. Its main task is to wait until all the promises that are passed to it are resolved.
注意:Promise将在单核CPU中同时运行,而在多核CPU中并行运行。 它的主要任务是等待传递给它的所有承诺都得到解决。
const PromiseArray = [
Promise.resolve(100),
Promise.reject(null),
Promise.resolve("Data release"),
Promise.reject(new Error('Something went wrong'))];Promise.all(PromiseArray)
.then(data => console.log('all resolved! here are the resolve values:', data))
.catch(err => console.log('got rejected! reason:', err))
One main thing to note about Promise.all
is that the method throws an error when one of the promises reject. This would mean that your code will not wait until all your promises are complete.
关于Promise.all
主要注意Promise.all
是,当一个Promise.all
拒绝时,该方法将引发错误。 这意味着您的代码将不会等到您所有的诺言都完成。
If you want to wait until all your promises are complete, regardless whether they get rejected or resolved, you can use the Promise.allSettled
. This method is in the finalized version of ES2020.
如果您要等到所有承诺都完成后,无论它们被拒绝还是被解决,都可以使用Promise.allSettled
。 此方法在ES2020的最终版本中。
const PromiseArray = [
Promise.resolve(100),
Promise.reject(null),
Promise.resolve("Data release"),
Promise.reject(new Error('Something went wrong'))];Promise.allSettled(PromiseArray).then(res =>{
console.log(res);
}).catch(err => console.log(err));//[
//{status: "fulfilled", value: 100},
//{status: "rejected", reason: null},
//{status: "fulfilled", value: "Data release"},
//{status: "rejected", reason: Error: Something went wrong ...}
//]
Even though some of the promises are rejected, Promise.allSettled
returns the results from all your promises.
即使某些承诺被拒绝, Promise.allSettled
从所有承诺中返回结果。
使用Bit发布和重用React组件 (Publish and Reuse React Components with Bit)
Bit makes it easy to publish reusable React components from any codebase to Bit’s component hub.
使用Bit可以轻松地将可重用的React组件从任何代码库发布到Bit的组件中心。
Need to update a published component? bit import
it into your project, change it, and push it back with a bumped version.
是否需要更新已发布的组件? bit import
其bit import
到您的项目中,进行更改,然后将其推送到凹凸版本。
Share components with your team to maximize code reuse, speed up delivery, and build apps that scale.
与您的团队共享组件 ,以最大程度地重复使用代码,加快交付速度并构建可扩展的应用程序。
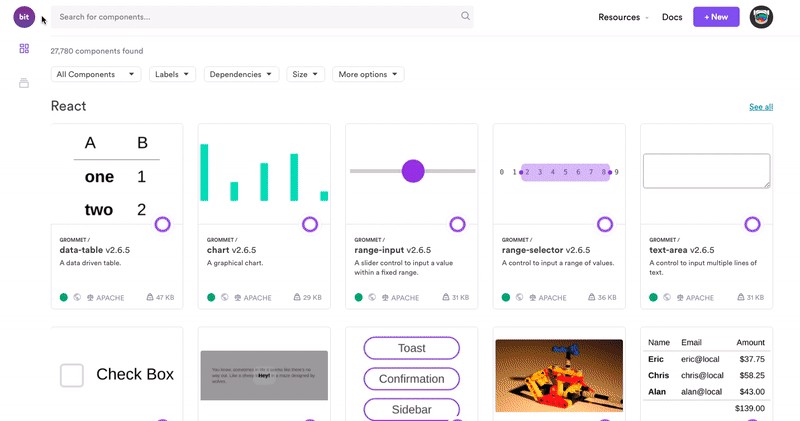
相关故事 (Related Stories)
That’s it for this article. If you have any other tricks that might be helpful, please do mention them in the comments.
本文就是这样。 如果您还有其他可能有用的技巧,请在评论中提及它们。
Happy Coding!!
快乐编码!
ResourcesBlog post by David WalshBlog post by Bret CameronBlog post by Ngninja
翻译自: https://blog.bitsrc.io/10-super-useful-tricks-for-javascript-developers-f1b76691199b