json dom
Many former colleagues and friends have reached out to me and asked: “How did you get this efficient and fast when working inside the UI area?”
许多以前的同事和朋友与我联系并问:“在UI区域内工作时,您是如何高效而快速地获得这种信息的?”
While I have been using Javascript for 20 years, experience is really just a small point in a very fast evolving ecosystem.
在我使用Javascript 20年的同时,经验确实只是在快速发展的生态系统中的一小部分。
The biggest impact was switching to JSON based virtual DOM, which boosted my own productivity by at least 200%. Yes, this literally means that I can develop complex UI code 3 times faster as I could before.
最大的影响是切换到基于JSON的虚拟DOM,这使我自己的生产率提高了至少200%。 是的,从字面上看,这意味着我可以比以前快3倍地开发复杂的UI代码。
Since these concepts are still mostly unknown, I am writing this article to share my knowledge with you. The goal is that you can benefit as well and get to a new level of Frontend Development.
由于这些概念仍然几乎是未知的,因此我写这篇文章是为了与您分享我的知识。 目标是您也可以从中受益并达到前端开发的新水平。
内容 (Content)
- Introduction 介绍
- What is JSON based virtual DOM? 什么是基于JSON的虚拟DOM?
- Which properties can I use on a vdom node? 我可以在vdom节点上使用哪些属性?
- Which implementation are we using for this article? 本文将使用哪种实现?
- A quick overview of the neo.mjs JS class system enhancements neo.mjs JS类系统增强功能的快速概述
- Is our virtual DOM structure a Template? 我们的虚拟DOM结构是模板吗?
- Constructing our first JSON based virtual DOM 构造我们的第一个基于JSON的虚拟DOM
- Basic VDOM operations 基本VDOM操作
- Changing multiple Configs 更改多个配置
The removeDom vdom property
removeDom vdom属性
- The flag vdom property 标志vdom属性
Adding Components into a Component (Highlight of this article)
将组件添加到组件中 (本文重点)
- getVdomRoot() getVdomRoot()
Using the VDom Engine itself is optional (Highlight of this article)
使用VDom引擎本身是可选的 (本文重点)
- Using Component Trees 使用组件树
Extending Classes (Highlight of this article)
扩展类 (本文重点)
- Final Thoughts 最后的想法
1.简介 (1. Introduction)
This is going to be a pretty long article. Please be open minded and curious, since these concepts are disruptive.
这将是一篇很长的文章。 由于这些概念具有破坏性,请保持开放的态度和好奇心。
Before we can dive deep into the benefits, it is important to cover the basics.
在深入了解这些好处之前,必须涵盖基础知识。
I marked the highlights of this article in bold inside the Content overview. I strongly recommend to read the article from top to bottom though.
我在内容概述中用粗体标记了本文的重点。 我强烈建议您从上至下阅读该文章。
Especially the section when it comes to extending classes is mind blowing.
尤其是关于扩展课程的部分令人震惊。
Using JSON based virtual DOM is especially useful, in case you want to create complex Components or Apps:
如果要创建复杂的组件或应用程序,则使用基于JSON的虚拟DOM尤其有用:
2.什么是基于JSON的虚拟DOM? (2. What is JSON based virtual DOM?)
With the term I am not referring to a JSON based String, but a nested Javascript structure of Objects and Arrays, following the JSON Syntax.
我所说的术语不是指基于JSON的字符串,而是指遵循JSON语法的嵌套对象和数组的Javascript结构。
Simply put:
简单的说:
const jsonVdom = JSON.parse(jsonVdomString);
To give you a quick example of how this can look like:
给你一个简单的例子,看起来像这样:
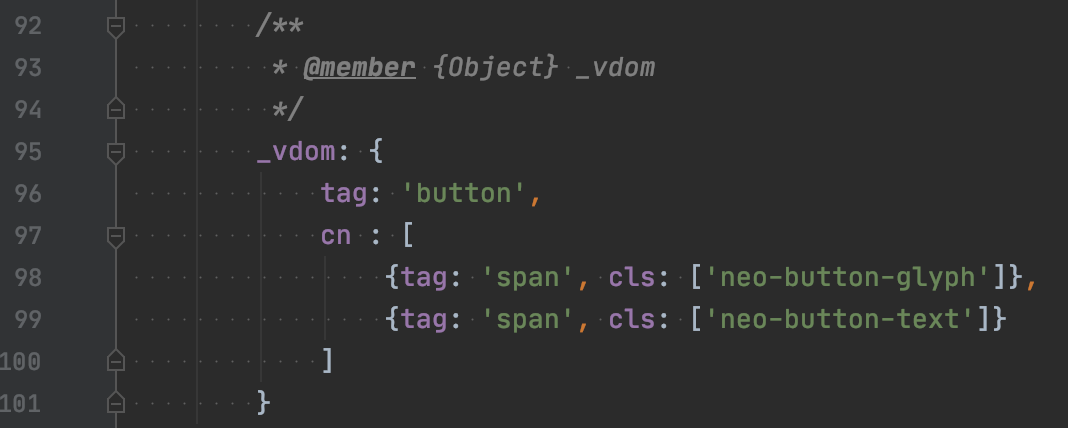
Many junior developers stopped looking deeper into this already at this point.
此时,许多初级开发人员已停止对此进行更深入的研究。
- Wait, I can achieve the same in Angular, React or Vue with a 3lines pseudo XML template. 等等,我可以使用3lines伪XML模板在Angular,React或Vue中实现相同的功能。
- This does not look nice, since it is less compact. 这看起来不太好,因为它不那么紧凑。
- Pseudo XML templates better match the DSL (domain specific language) pattern. 伪XML模板与DSL(特定于域的语言)模式更好地匹配。
Since you are an open minded, curious and passionate developer, you will keep reading to figure out, what the real benefits are.
由于您是一个思维开放,好奇和热情的开发人员,因此您将继续阅读以找出真正的好处。
- Agreed, it is a bit longer. It is not a template though. Meaning: it is a persistent structure, which is meant to get changed dynamically at runtime. You can change it the same way before & after a Component gets mounted. 同意,这会更长一些。 它不是模板。 含义:这是一个持久性结构,旨在在运行时动态更改。 您可以在安装组件之前和之后以相同的方式进行更改。
- This is not a comparison about Apples & Oranges. It does not matter if it looks nice at the first glance. What counts is what you can do with it. 这不是关于Apples&Oranges的比较。 乍一看是否好都没关系。 重要的是您可以用它做什么。
- If you compare a pseudo XML template to the way HTML looks (e.g. inside the Chrome Dev Tools: Inspect Element), you are correct. However, JS provides an API to work with DOM nodes, which is way more object oriented. Unless you are deeply in love with regular expressions, you will agree that Javascript is meant for working with Objects & Arrays, rather than working with Strings. 如果您将伪XML模板与HTML的外观进行比较(例如,在Chrome Dev Tools:Inspect Element中),那么您是正确的。 但是,JS提供了一个与DOM节点一起使用的API,这更加面向对象。 除非您对正则表达式深感兴趣,否则您将同意Javascript是用于对象和数组的,而不是用于字符串的。
3.我可以在vdom节点上使用哪些属性? (3. Which properties can I use on a vdom node?)
Inside the neo.mjs implementation, we can use:
在neo.mjs实现内部,我们可以使用:
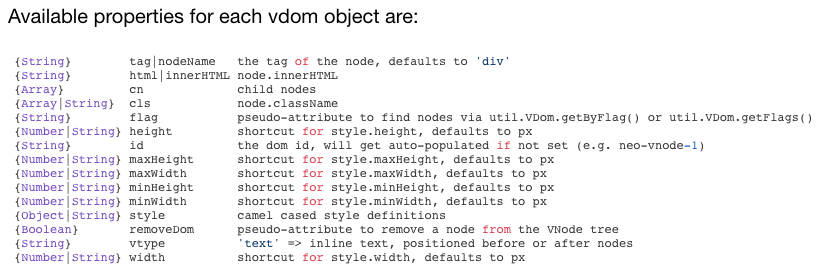
You can find the details on how these properties get applied here:
您可以在此处找到有关如何应用这些属性的详细信息:
https://github.com/neomjs/neo/blob/dev/src/vdom/Helper.mjs#L262
https://github.com/neomjs/neo/blob/dev/src/vdom/Helper.mjs#L262
In short:
简而言之:
- tag is the most important one. You can pick any html tags or tags of custom WebComponents. 标签是最重要的。 您可以选择任何html标记或自定义WebComponents的标记。
- A vdom engine relies on each node having an id, to figure out which nodes got moved to different spots. You can manually assign ids, otherwise each node will get an automatically generated one (neo-vnode-x). vdom引擎依赖于每个具有ID的节点来确定哪些节点已移动到不同位置。 您可以手动分配ID,否则每个节点将获得一个自动生成的ID(neo-vnode-x)。
- html: the innerHTML of your node, in case it does not have child nodes. html:您的节点的innerHTML(如果没有子节点的话)。
- cn: the child nodes, which have the same properties available. You can nest your vdom structure as deeply as you like to. cn:子节点,具有相同的可用属性。 您可以随意嵌套vdom结构。
style: You can define your style attribute as a String. It is strongly recommended though to stick to an Object based syntax. E.g.:
样式:您可以将样式属性定义为字符串。 强烈建议您坚持使用基于对象的语法。 例如:
style: {borderColor: ‘red’, marginRight: ‘10px’, marginTop: ‘-5px’}
样式:{borderColor:“红色”,marginRight:“ 10px”,marginTop:“-5px”}
You can use camelCase for style attribute names.
您可以将camelCase用作样式属性名称。
- height, maxHeight, maxWidth, minHeight, minWidth, width are just convenience shortcuts, which will get put into the style object. height,maxHeight,maxWidth,minHeight,minWidth,width只是方便快捷方式,它们将被放入样式对象中。
You can put in any HTML tag attributes directly into a vdom object.
您可以将任何HTML标记属性直接放入vdom对象。
E.g.: tabIndex: -1
例如:tabIndex:-1
- vtype: ‘text’, flag & removeDom will get covered more in detail later on. vtype:'text',flag&removeDom稍后将详细介绍。
4.本文将使用哪种实现? (4. Which implementation are we using for this article?)
Since the neo.mjs implementation is the most advanced one, I will stick to it for this article.
由于neo.mjs实现是最高级的实现,因此在本文中我将坚持使用它。
neo.mjs is a disruptive next generation Javascript Frontend Framework, which enables you to build blazing fast multithreading UIs.
neo.mjs是一个具有破坏性的下一代Javascript前端框架,使您能够构建出色的快速多线程UI。
The project is using the MIT license, so you can use it for free:
该项目正在使用MIT许可证,因此您可以免费使用它:
You don’t have to use neo.mjs, in case you want to use JSON based virtual DOM. The MIT license enables you to extract & use the vdom implementation as you like to. You could also create an own implementation following the same design patterns.
如果您想使用基于JSON的虚拟DOM,则不必使用neo.mjs。 MIT许可证使您可以根据需要提取和使用vdom实现。 您还可以按照相同的设计模式创建自己的实现。
In case you are thinking about using neo.mjs, you should take a quick look at the Workers Setup:
如果您正在考虑使用neo.mjs,则应快速查看“工人设置”:
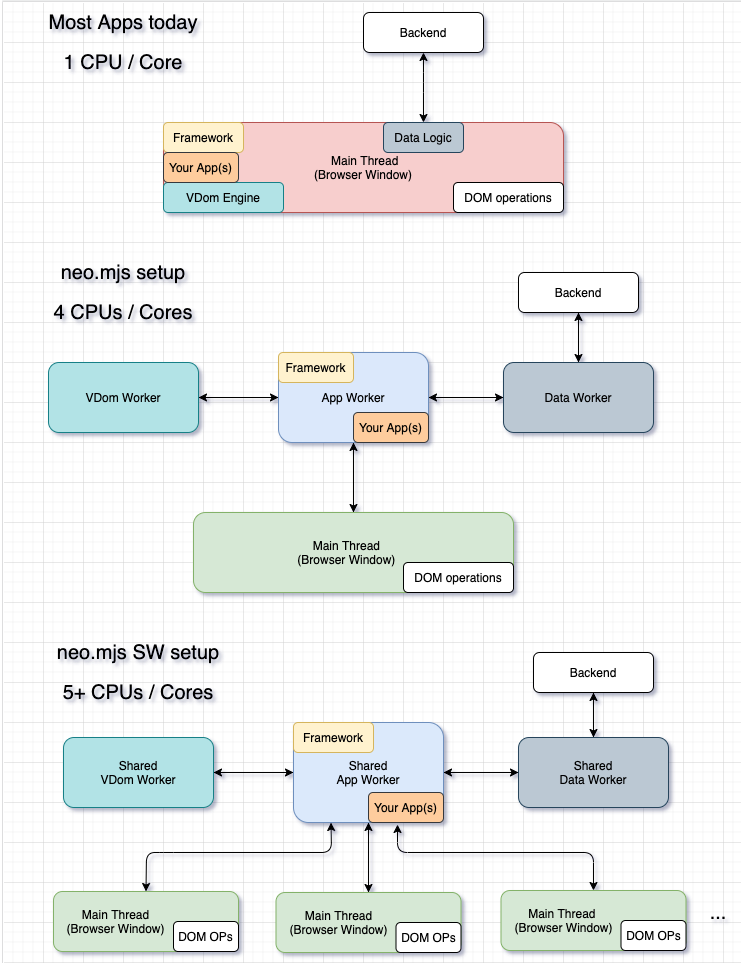
The important part for this article is that the VDom Engine lives inside a separate thread. This means that all calls to the Engine are async.
本文的重要部分是VDom Engine位于单独的线程中。 这意味着对引擎的所有调用都是异步的。
5. neo.mjs JS类系统增强功能的快速概述 (5. A quick overview of the neo.mjs JS class system enhancements)
ES6 is out there for a long time, so I am assuming you are familiar with the Javascript class system at this point.
ES6已经存在很长时间了,因此我假设您现在已经熟悉Javascript类系统。
The missing part are still class properties.
缺少的部分仍然是类属性。
To fill this gap, neo.mjs has enhanced JS classes with a Custom Config System.
为了填补这一空白,neo.mjs使用自定义配置系统增强了JS类。
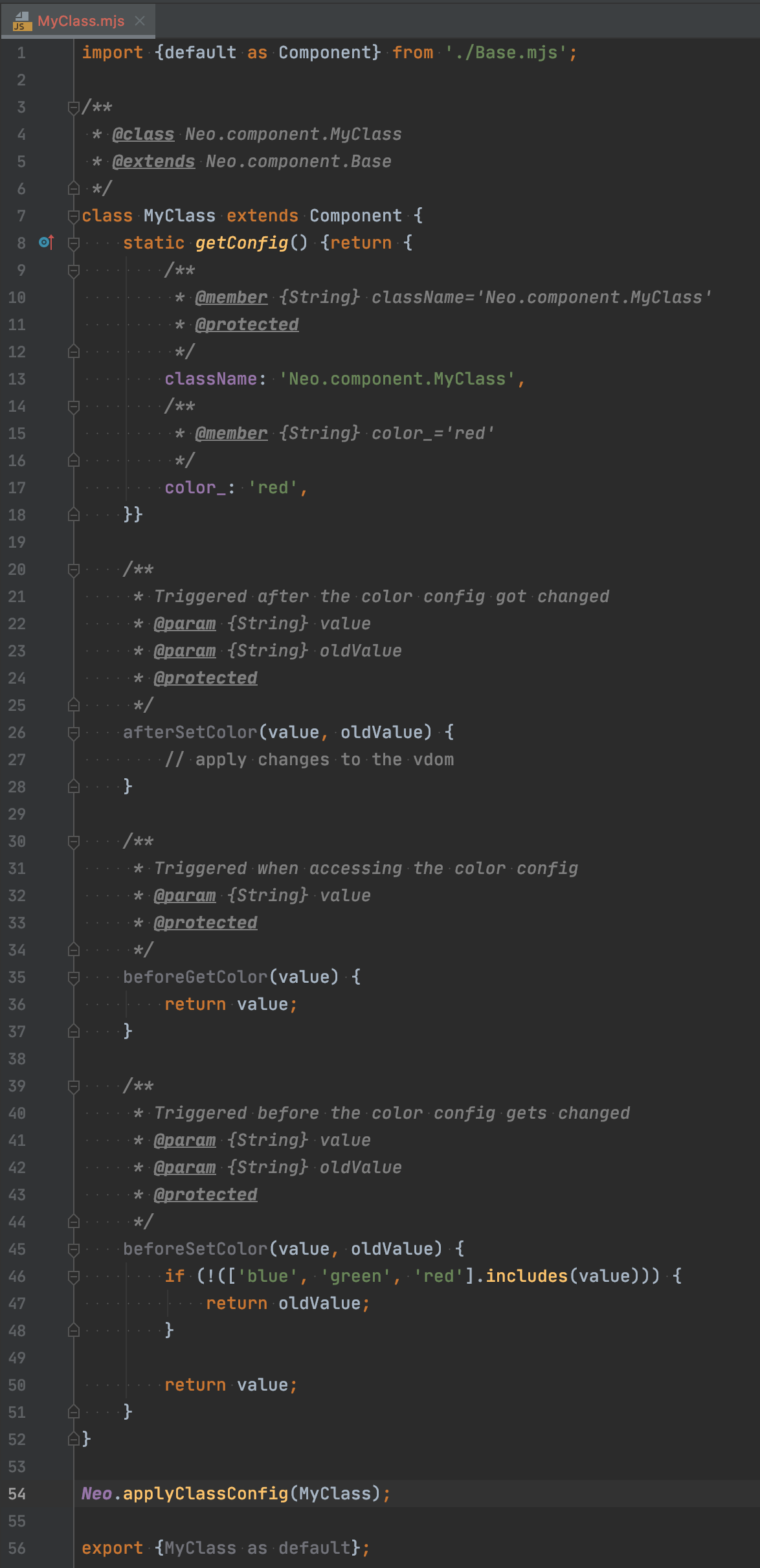
In short: Each class has the static getConfig() method available.
简而言之:每个类都有可用的静态getConfig()方法。
Inside of it, you can specify your class properties.
在其中,您可以指定您的类属性。
In case a config ends wi