制作钉钉应用
In this article you will know how to create a simple movie info search app using React. And then I will guide you how to overcome the interactive challenges you will facing while developing a project and to become a successful React developer.
在本文中,您将了解如何使用React创建一个简单的电影信息搜索应用程序。 然后,我将指导您如何克服在开发项目时将面临的互动挑战,并成为一名成功的React开发人员。
Before get into the topic, let’s see about react. It is a powerful frontend framework used to build user interface for mobile as well as web development. You can create complex UI in react using reusable components.
在进入主题之前,让我们来了解一下React。 它是一个强大的前端框架,用于为移动和Web开发构建用户界面。 您可以使用可重用的组件来在响应中创建复杂的UI。
Let’s get deep into our project, the list of steps we are going to follow to develop this application:
让我们深入研究我们的项目,开发该应用程序将遵循的步骤列表:
- Getting an API key 获取API密钥
- Adding base styles 添加基本样式
- Create and style components. 创建组件并设置样式。
- Create functions 创建功能
- Manage state using hooks 使用挂钩管理状态
- Create and style cards 创建和设置卡片样式
先决条件 (Prerequisites)
Before get started, you should have basic knowledge in HTML, CSS and JavaScript to develop this React code.
在开始之前,您应该具有HTML,CSS和JavaScript的基本知识来开发此React代码。
Let’s get started!
让我们开始吧!
获取API密钥 (Getting an API Key)
The API we are going to use for displaying the movie information is themoviedb.org. Go to this website and sign up for free account and then head to settings tab in your account and click to generate an API .
我们将用于显示电影信息的API是themoviedb.org。 转到该网站并注册一个免费帐户,然后转到帐户中的“设置”标签,然后单击以生成API。
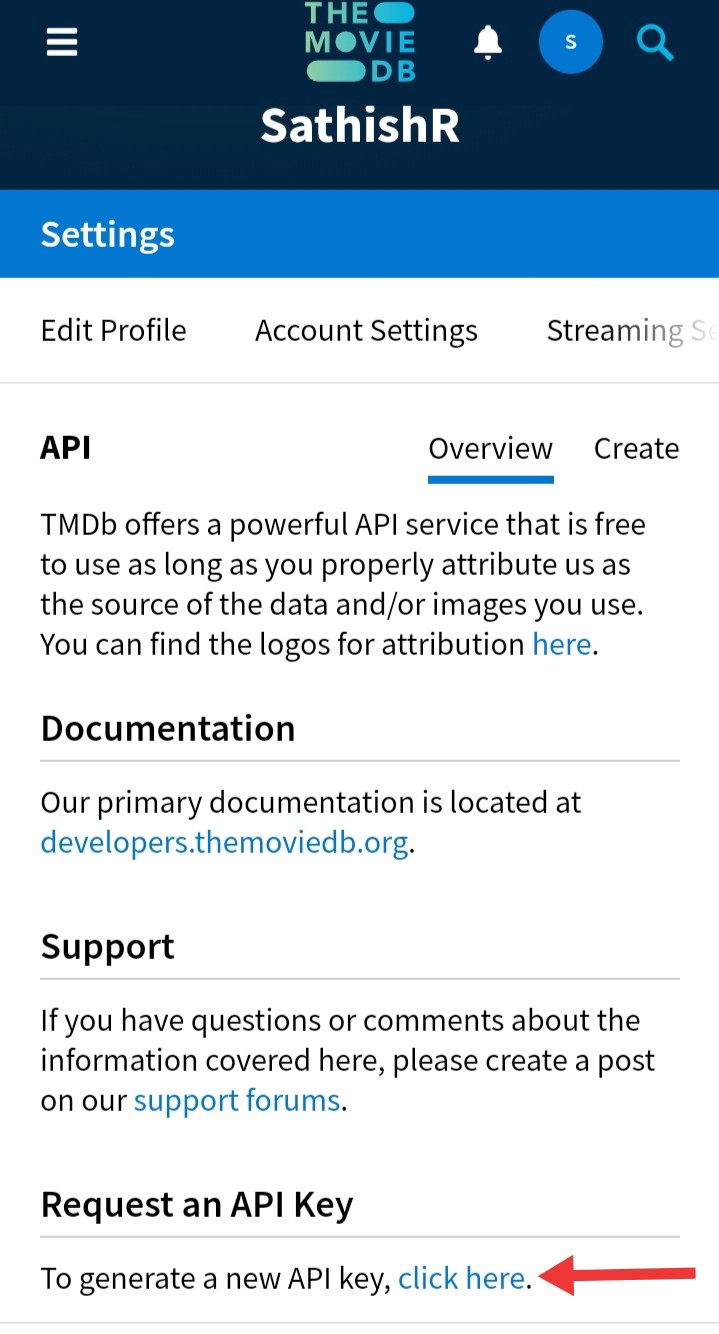
添加基本样式 (Adding base styles)
The basic React application we instantiated is looks likes this:
我们实例化的基本React应用程序如下所示:
import React from "react";
import ReactDOM from "react-dom";
class Main extends React.Component {
render() {
return <h1>Hello world!</h1>;
}
}
ReactDOM.render(<Main />, document.getElementById("root"));
And then add some basic styles to style.css includes margins, padding, and style sheet set to default to make centering the app. The style.css looks like this:
然后将一些基本样式添加到style.css中,其中包括设置为默认值以使应用居中的边距,填充和样式表。 style.css看起来像这样:
html {
font-size: 10px;
}
* {
border-sizing: border-box;
}body {
margin:0;
padding:0;
background-color: rgba(244,244,244);
color: #333;
}
p {
font-size: 1.6 rem;
}
small {
font-size: 1.2 rem;
}
. container {
margin: 0;
max-width: 1000px;
padding: 40px;
}
.title {
font-size: 4.4 rem;
text-align: centre;
}
设置搜索电影组件的样式 (Style the Search Movie Component)
Next step is to style our new component. Styles for our <form>, <label>, <input> and <button> and then add a media query to style.css to adjust the styles on larger devices.
下一步是设置新组件的样式。 我们的<form> , <label> , <input>和<button>的样式,然后将媒体查询添加到style.css以调整较大设备上的样式。
@media (min-width: 786px) {
.form {
grid-template-columns: auto 1fr auto;
grid-gap: 1rem;
align-items: center;
}
.input {
margin-bottom: 0;
}
}
创建搜索电影功能 (Create Search Movie Functions)
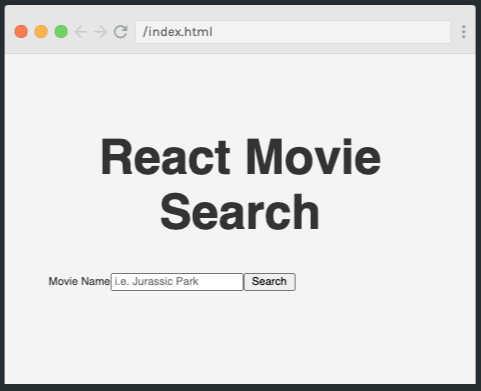
We have to create an asynchronous function that will fetch API to retrieve the movie information the MovieDB API and display the information into our component. Add the following code in searchMovie.js.
我们必须创建一个异步函数,该函数将获取API来检索MovieDB API的电影信息并将该信息显示到我们的组件中。 在searchMovie.js中添加以下代码。
export default function SearchMovies(){
const searchMovies = async (e) => {
e.preventDefault();
const query = "Jurassic Park";
const url = `https://api.themoviedb.org/3/search/movie?api_key=5dcf7f28a88be0edc01bbbde06f024ab&language=en-US&query=${query}&page=1&include_adult=false`;
try {
const res = await fetch(url);
const data = await res.json();
console.log(data);
}catch(err){
console.error(err);
}
}
使用挂钩管理状态 (Manage State Using Hooks)
Let us show how to use the state to track the user’s query with the useState hook.
让我们展示如何使用状态通过useState挂钩来跟踪用户的查询。
const [query, setQuery] = useState("");
And then set the onChange on our <input> to bind it to the state.
然后在<input>上设置onChange以将其绑定到状态。
<input
className="input"
type="text"
name="query"
placeholder="i.e. Jurassic Park"
value={query}
onChange={(e) => setQuery(e.target.value)}
/>
创建和设置电影卡的样式 (Create and Style the Movie Card)
Now let us show to style our movie card to make more attractive and user friendly. We start with an card container <div>.
现在,让我们展示一下电影卡的样式,使其更具吸引力和用户友好性。 我们从卡片容器<div>开始。
.card {
padding: 2rem 4rem;
border-radius: 10px;
box-shadow: 1px 1px 5px rgba(0,0,0,0.25);
margin-bottom: 2rem;
background-color: white;
}
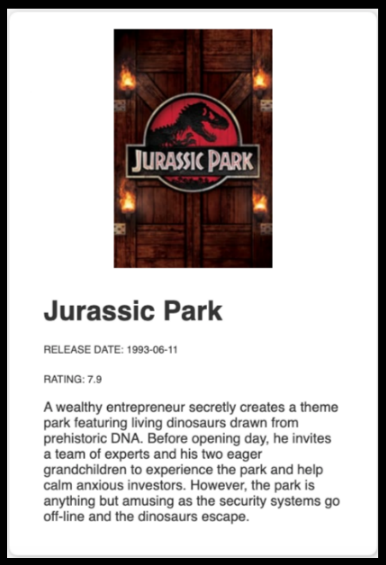
Now we have done all this steps as a separate modules. Let’s see the how the final searchMovie.js is looks like.
现在,我们将所有这些步骤作为一个单独的模块完成。 让我们看看最终的searchMovie.js的样子。
import React, {useState} from "react";
export default function SearchMovies(){
//states- input query, movies
const [query, setQuery] = useState('');
//create the state for movies, and update that state appropriate
const [movies, setMovies] = useState([]);
const searchMovies = async (e) => {
e.preventDefault();
const url = `https://api.themoviedb.org/3/search/movie?api_key=5dcf7f28a88be0edc01bbbde06f024ab&language=en-US&query=${query}&page=1&include_adult=false`;
try {
const res = await fetch(url);
const data = await res.json();
setMovies(data.results);
}catch(err){
console.error(err);
}
}
return (
<form className="form" onSubmit={searchMovies}>
<label className="label" htmlFor="query">Movie Name</label>
<input className="input" type="text" name="query"
placeholder="i.e. Jurassic Park"
value={query} onChange={(e) => setQuery(e.target.value)}
/>
<button className="button" type="submit">Search</button>
</form>
)
}
结论 (Conclusion)
I hope you enjoyed a lot and know how to create a simple components in React and how to fetch API from third party websites and passing it into our component and displays the information. You can also add lot of functionalities to this application.
我希望您很喜欢并且知道如何在React中创建一个简单的组件以及如何从第三方网站获取API并将其传递到我们的组件中并显示信息。 您还可以向此应用程序添加许多功能。
Have fun and Thanks for reading!
玩得开心,感谢您的阅读!
普通英语JavaScript (JavaScript In Plain English)
Enjoyed this article? If so, get more similar content by subscribing to Decoded, our YouTube channel!
喜欢这篇文章吗? 如果是这样,请订阅我们的YouTube频道解码,以获得更多类似的内容!
翻译自: https://medium.com/javascript-in-plain-english/build-a-movie-info-search-app-ea39e1df6fdd
制作钉钉应用