leetcode首个正数
A Step-by-Step Guide with Code Walk Through
代码遍历的分步指南
In this Leetcode problem, we’re told that we’re given an ordered array of positive integers that has some missing numbers as input, along with a number (k). Our task: find the kth missing number in that input array. Sounds more complicated than it is. Let’s get at it.
在这个Leetcode问题中,我们被告知我们得到了一个正整数的有序数组,该数组具有一些缺少的数字作为输入以及数字(k)。 我们的任务:在该输入数组中找到第k个缺失数字。 听起来比它复杂。 让我们开始吧。
Let’s say we have an array with the number [1, 2, 3, 4], and we’re told to find the 2nd missing number. Confusing. Doesn’t seem like there is a missing number in that array.
假设我们有一个数字为[1、2、3、4]的数组,并被告知要找到第二个缺失的数字。 令人困惑。 似乎该数组中没有缺失的数字。
We’d have to assume that the two missing number are 5 and 6, and thus, the 2nd missing number is 6, and the full array would actually be [1, 2, 3, 4, 5, 6].
我们必须假设两个缺失数是5和6,因此,第二个缺失数是6,而整个数组实际上是[1、2、3、4、5、6]。
什么是算法? (What’s the Algorithm?)
As a human, if you’re tasked to find the kth missing numbers in a sequence, I’d argue you’d do something like,
作为人类,如果您要按顺序查找第k个缺失数字,我会说您会做类似的事情,
- Start from 1, counting up 从1开始,向上计数
- When a number seems to be missing, make note of it by incrementing an internal counter. 如果似乎缺少数字,请通过增加内部计数器记下它。
- When you’ve reached the number of missing numbers you were asked to find, stop, and say which number should’ve been there. 当您达到丢失的数字数量时,系统会要求您查找,停止并说出应该去的数字。
编码解决方案 (Coding a Solution)
Let’s initialize a function called findKthPositive that takes in an array (arr) and a number (k) as input. And, as described above, we’re going to need to count how many numbers are missing in our sequence, so let’s initialize a missingCounter variable:
让我们初始化一个名为findKthPositive的函数,该函数接受一个数组( arr )和一个数字( k )作为输入。 而且,如上所述,我们需要计算序列中有多少个数字丢失,所以让我们初始化一个missingCounter变量:
function findKthPositive(arr, k) {
let missingCounter = 0;
}
Next, we have to walk through our sequence. Let’s do that with a for loop. Typically we setup a for loop to run the entire length of an array. But what do we do when our array has missing values?
接下来,我们必须按照顺序进行操作。 让我们用for循环来实现。 通常,我们设置一个for循环来运行数组的整个长度。 但是,当数组缺少值时我们该怎么办?
One way is to tell our for loop to keep running while a condition hasn’t been met. That condition should be to keep going, while I haven’t reached the amount of missing numbers that k tells me:
一种方法是告诉我们的for循环在未满足条件时继续运行。 这个条件应该是继续前进,而我还没有达到k告诉我的缺失数字的数量:
function findKthPositive(arr, k) {
let missingCounter = 0;for (let i = 1; missingCounter != k; i++) {
}
}
Also, because the problem states we’re getting positive integers as an input, we can go ahead and start at 1, instead of 0 like we normally do.
另外,由于问题表明我们得到正整数作为输入,因此我们可以继续从1开始而不是像通常那样从0开始。
Next, we’re going to test if the number we’re currently iterating with is in the array. If it’s not, we should increment our missingCounter. And we’ll keep doing that until our missingCounter = k:
接下来,我们将测试当前要迭代的数字是否在数组中。 如果不是,我们应该增加我们的missingCounter。 我们将继续这样做,直到我们的missingCounter = k:
function findKthPositive(arr, k) {
let missingCounter = 0;for (let i = 1; missingCounter != k; i++) { if (!arr.includes(i)) missingCounter++;
if (missingCounter === k) return i;
}
}
At the moment the missingCounter = k, we’ve found that missing number, and we should return it.
目前missingCounter = k,我们已经找到了那个遗漏的数字,应该将其返回。
代码演练 (Code Walkthrough)
If this is still a bit abstract, let’s walk through the example.
如果仍然有点抽象,让我们来看一下示例。
- We’ve initialized our missingCounter variable with 0, and entered a for loop with i = 1, since 0 ≠ 2. 我们用0初始化了missingCounter变量,并输入了一个i = 1的for循环,因为0≠2。
- We meet the conditional: is 1 in our array? It is, so we don’t increment the missingCounter. 我们满足条件:数组中是否为1? 是的,因此我们不会增加missingCounter。
- We’ve already agreed 0 ≠ 2 so we don’t return anything, and go back up the loop. 我们已经同意0≠2,所以我们不返回任何内容,而是返回循环。
- Now i = 2. Is 2 in the array? It is, so we so we don’t increment the missingCounter. 现在我=2。数组中是否有2? 是的,因此我们不增加missingCounter。
- missingCounter is still 0. 0 ≠ 2 so we don’t return anything, and go back up the loop. missingCounter仍为0。0≠2,因此我们不返回任何内容,而是返回循环。
- Now i = 3. Is 3 in the array? It is, so we so we don’t increment the missingCounter. 现在我=3。数组中是否有3个? 是的,因此我们不增加missingCounter。
- missingCounter is still 0. 0 ≠ 2 so we don’t return anything, and go back up the loop. missingCounter仍为0。0≠2,因此我们不返回任何内容,而是返回循环。
- Now i = 4. Is 4 in the array? It is, so we so we don’t increment the missingCounter. 现在i =4。数组中是否有4个? 是的,因此我们不增加missingCounter。
- missingCounter is still 0. 0 ≠ 2 so we don’t return anything, and go back up the loop. missingCounter仍为0。0≠2,因此我们不返回任何内容,而是返回循环。
Now i = 5. Is 5 in the array? It is not. So this time we increment missingCounter to 1.
现在i =5。数组中是否有5个? 不是。 所以这一次我们将missingCounter增加到1。
- missingCounter is now 1. 1≠ 2 so we don’t return anything, and go back up the loop. missingCounter现在为1。1≠2,因此我们不返回任何内容,而是返回循环。
Now i = 6. Is 6 in the array? It is not. So this time we increment missingCounter to 2.
现在i =6。数组中是否有6个? 不是。 所以这一次我们将missingCounter增加到2。
missingCounter is now 2. 2 = 2 so now return i, which is 6.
missingCounter现在为2。2 = 2,所以现在返回i,即6 。
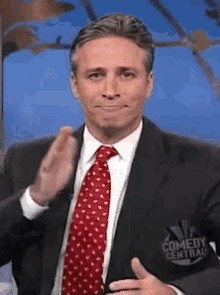
Questions? Leave them in the comment. Thanks for reading!
有什么问题吗 将它们留在评论中。 谢谢阅读!
And remember…
记住...
Grit > talent.
坚毅>才能。
leetcode首个正数