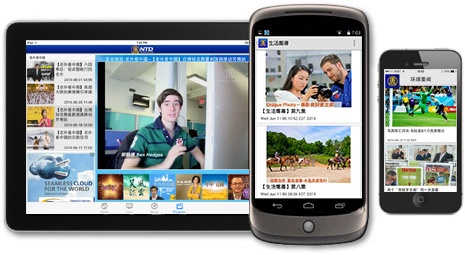
在android开发中,有一个很好用的控件:Fragment,利用Fragement可以实现在不同大小的屏幕上展示不同的布局风格,从而增加软件的适应性。
这次我们这个简易的新闻的应用,是想在平板和手机上呈现出不同的样式。对于屏幕小的手机,我们先仅展示标题,而后在点击后进入详情页,对于屏幕更充裕的平板,那仅仅展示表格题就不够了,我们可以在左侧显示标题,而在右侧显示新闻的具体内容(大概就是下面两幅图片中展示的样子)。
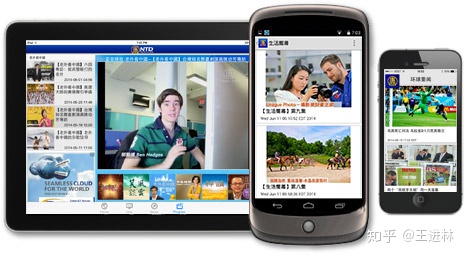
要进行这个应用窗口的制作,大概需要这样的几个步骤:
1.确定要用的碎片以及类
2.确定各个碎片中的布局以及子控件
3.碎片实例化后添加到窗体上
这次的新闻软件界面我们使用两个碎片,一个来存储标题的信息,一个用来存储新闻内容的信息,根据设备的屏幕大小对两个控件进行选择性的展示。将一个新闻的标题和内容划到News这一个类中进行操作。
新建一个News实体类,代码如下:
public class News {
private String title;//标题
private String content;//内容
public void setTitle(String title){
this.title = title;
}
public String getTitle(){
return tittle;
}
public void setContent(String content){
this.content = content;
}
public String getContent(){
return content;
}
}
下面先来设计下新闻内容的布局格式:
在layout文件夹下新建一个布局文件news_content_frag.xml文件,用作新闻内容的布局文件:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:orientation="horizontal"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:id="@+id/visibility_layout"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/news_title"/>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="#000"/>
<TextView
android:id="@+id/news_content"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:padding="15dp"
android:textSize="18sp"
/>
</LinearLayout>
<View
android:layout_width="1dp"
android:layout_height="match_parent"
android:layout_alignParentLeft="true"/>
</LinearLayout>
上面是一个放置标题的TextView控件,下方是一个放置具体新闻信息的TextView的控件,中间使用一条水平线进行分割。
然后新建一个NewsContentFragment的类来使用这个布局:
public class NewsContentFragment extends Fragment {
private View view;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container,@Nullable Bundle savedInstanceState) {
view = inflater.inflate(news_content_frag, container ,false );
//加载建立news_content_frag布局文件,作为第一个碎片
return view;
}
public void refesh(String newstitlt,String newsContent){
View visibilityLayout = view.findViewById(R.id.visibility_layout);
visibilityLayout.setVisibility(View.VISIBLE);
TextView newTitleText = (TextView)view.findViewById(R.id.news_title);
TextView newsContentText = (TextView)view.findViewById(R.id.news_content);
newTitleText.setText(newstitlt);
newsContentText.setText(newsContent);
}
}
首先在onCreate方法里加载了我们的这个布局,refresh方法对这个碎片的内容进行更新。这样,第一个碎片就算是做好了。接下来按照类似的方法,我们来进行标题列表碎片的建立:
布局文件:news_title_frag.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.v7.widget.RecyclerView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/news_title_recycler_view"
/>
</LinearLayout>
这里引入的控件是一个扩展出的RecyclerView控件,对于这个空间的具体使用,后面会继续推进更新。
对应这个控件我们也需要对里面的子控件的布局进行编辑:
新建news_item.xml文件
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/news_title"
android:maxLines="1"
android:ellipsize="end"
android:textSize="18sp"
android:paddingTop="15dp"
android:paddingBottom="15dp"
android:paddingLeft="10dp"
android:paddingRight="10dp"
>
</TextView>
类:NewsTitleFragment
public class NewsTitleFragment extends Fragment {
private boolean isTwoPane;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.news_title_frag, container, false);
return view;
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
if(getActivity().findViewById(R.id.news_content_layout)!= null){
isTwoPane = true;//找到news_content_layout布局时,就是双页布局
}else{
isTwoPane = false;//找不到news_content_layout布局时,就为单页布局
}
}
这里使用了一个id为news_content_layout,暂时还并没有建立起来,要实现有这个控件的时候使用双页布局,没有这个控件的时候使用单页布局,我们可以使用限定符来操作。
首先修改activity_main.xml文件:在单页模式下,仅展示一个新闻标题的碎片.
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".FraamentBestPractice">
<fragment
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/news_title_fragment"
android:name="com.example.myapplication.NewsTitleFragment"
/>
</FrameLayout>
然后在res文件夹下新建一个layout-sw600dp文件夹,文件夹下新建activity_main.xml,而后修改代码为:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<fragment
android:layout_width="0dp"
android:layout_height="match_parent"
android:id="@+id/news_title_fragment"
android:name="com.example.myapplication.NewsTitleFragment"
android:layout_weight="10"/>
<FrameLayout
android:layout_width="0dp"
android:layout_weight="3"
android:id="@+id/news_content_layout"
android:layout_height="match_parent">
<fragment
android:id="@+id/news_contents_fragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:name="com.example.myapplication.NewsContentFragment"
/>
></FrameLayout>
</LinearLayout>
这里在双页模式下引入了两个碎片,并将内容的碎片放入一个id为news_content_layout的布局下,能够找到这个id的时候就是双页布局。
这时候,我们需要知道,在单页显示的时候,我们最好还是新建一个活动来进行具体的内容更好,所以我们新建一个NewContentActivity来进行单页中新闻内容的展示:
public class NewsContetActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.news_contet);
// 获取新闻的标题和内容
String newsTitle = getIntent().getStringExtra("news_title");
String newsContent = getIntent().getStringExtra("newsContent");
NewsContentFragment newsContentFragment = (NewsContentFragment)getSupportFragmentManager().findFragmentById(R.id.news_contents_fragment);
newsContentFragment.refesh(newsTitle, newsContent);
}
public static void actioinStart(Context context, String newsTitle, String newsContent){
Intent intent = new Intent(context,NewsContetActivity.class);
intent.putExtra("news_title", newsTitle);
intent.putExtra("newsContent", newsContent);
context.startActivity(intent);
}
}
这里方法actioinStart是为了在建立活动时方便获取参数而设计的。
修改对应的布局文件:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".NewsContetActivity">
<fragment
android:id="@+id/news_contents_fragment"
android:name="com.example.myapplication.NewsContentFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
</LinearLayout>
这里直接在布局中引入NewsC。相当于直接把news_content_frag.xml引进来。
最后,在NewsTitleFragment中建立类种类,给列表设置适配器,并使用随机数的方法产生测试用的样例新闻标题和内容,修改后的代码如下:
public class NewsTitleFragment extends Fragment {
private boolean isTwoPane;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.news_title_frag, container, false);
RecyclerView newsTitleRecyclerView = (RecyclerView)view.findViewById(R.id.news_title_recycler_view);
LinearLayoutManager layoutManager = new LinearLayoutManager(getActivity());
newsTitleRecyclerView.setLayoutManager(layoutManager);
NewsAdapter adapter = new NewsAdapter(getNews());
newsTitleRecyclerView.setAdapter(adapter);
return view;
}
private List<News> getNews() {
List<News> newsList = new ArrayList<>();
for (int i = 1;i<=50;i++){
News news = new News();
news.setTittle("This is news title"+i);
news.setContent(getRandomLengthContent("This is new content"+i+"."));
newsList.add(news);
}
return newsList;
}
private String getRandomLengthContent(String content) {
Random random = new Random();
int length = random.nextInt(20)+1;
StringBuilder builder = new StringBuilder();
for(int i = 1;i<length;i++){
builder.append(content);
}
return builder.toString();
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
if(getActivity().findViewById(R.id.news_content_layout)!= null){
isTwoPane = true;//找到news_content_layout布局时,就是双页布局
}else{
isTwoPane = false;//找不到news_content_layout布局时,就为单页布局
}
}
class NewsAdapter extends RecyclerView.Adapter{
private List<News> mNewList;
public NewsAdapter(List<News> newsList){
mNewList = newsList;
}
class ViewHolder extends RecyclerView.ViewHolder{
TextView newsTitleText;
public ViewHolder(View itemView) {
super(itemView);
newsTitleText = (TextView)itemView.findViewById(R.id.news_title);
}
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.news_items, parent,false );
final ViewHolder holder = new ViewHolder(view);
view.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
News news = mNewList.get(holder.getAdapterPosition());
if(isTwoPane){
NewsContentFragment newsContentFragment = (NewsContentFragment)getFragmentManager().findFragmentById(R.id.news_contents_fragment);
newsContentFragment.refesh(news.getTittle(), news.getContent());
}else{
NewsContetActivity.actioinStart(getActivity(), news.getTittle(), news.getContent());
}
}
});
return holder;
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
News news = mNewList.get(position);
((ViewHolder)holder).newsTitleText.setText(news.getTittle());
}
@Override
public int getItemCount() {
return mNewList.size();
}
}
}
到这儿,适用于不同大小屏幕的简单新闻界面窗口就算完成了!