作者:叫我詹躲躲
转发链接: https:// juejin.im/post/5edb6c6b e51d4578a2555a9b
176.如何确定设备是移动设备还是台式机/笔记本电脑?
const detectDeviceType = () =>
/Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(navigator.userAgent)
? 'Mobile'
: 'Desktop';
// 事例
detectDeviceType(); // "Mobile" or "Desktop"
复制代码
177.How to get the current URL?
const currentURL = () => window.location.href
// 事例
currentURL() // 'https://google.com'
复制代码
178.如何创建一个包含当前URL参数的对象?
const getURLParameters = url =>
(url.match(/([^?=&]+)(=([^&]*))/g) || []).reduce(
(a, v) => ((a[v.slice(0, v.indexOf('='))] = v.slice(v.indexOf('=') + 1)), a),
{}
);
// 事例
getURLParameters('http://url.com/page?n=Adam&s=Smith'); // {n: 'Adam', s: 'Smith'}
getURLParameters('google.com'); // {}
复制代码
179.如何将一组表单元素转化为对象?
const formToObject = form =>
Array.from(new FormData(form)).reduce(
(acc, [key, value]) => ({
...acc,
[key]: value
}),
{}
);
// 事例
formToObject(document.querySelector('#form'));
// { email: 'test@email.com', name: 'Test Name' }
复制代码
180.如何从对象检索给定选择器指示的一组属性?
const get = (from, ...selectors) =>
[...selectors].map(s =>
s
.replace(/[([^[]]*)]/g, '.$1.')
.split('.')
.filter(t => t !== '')
.reduce((prev, cur) => prev && prev[cur], from)
);
const obj = { selector: { to: { val: 'val to select' } }, target: [1, 2, { a: 'test' }] };
// Example
get(obj, 'selector.to.val', 'target[0]', 'target[2].a');
// ['val to select', 1, 'test']
复制代码
181.如何在等待指定时间后调用提供的函数?
const delay = (fn, wait, ...args) => setTimeout(fn, wait, ...args);
delay(
function(text) {
console.log(text);
},
1000,
'later'
);
// 1秒后打印 'later'
复制代码
182.如何在给定元素上触发特定事件且能选择地传递自定义数据?
const triggerEvent = (el, eventType, detail) =>
el.dispatchEvent(new CustomEvent(eventType, { detail }));
// 事例
triggerEvent(document.getElementById('myId'), 'click');
triggerEvent(document.getElementById('myId'), 'click', { username: 'bob' });
自定义事件的函数有 Event、CustomEvent 和 dispatchEvent
// 向 window派发一个resize内置事件
window.dispatchEvent(new Event('resize'))
// 直接自定义事件,使用 Event 构造函数:
var event = new Event('build');
var elem = document.querySelector('#id')
// 监听事件
elem.addEventListener('build', function (e) { ... }, false);
// 触发事件.
elem.dispatchEvent(event);
CustomEvent 可以创建一个更高度自定义事件,还可以附带一些数据,具体用法如下:
var myEvent = new CustomEvent(eventname, options);
其中 options 可以是:
{
detail: {
...
},
bubbles: true, //是否冒泡
cancelable: false //是否取消默认事件
}
其中 detail 可以存放一些初始化的信息,可以在触发的时候调用。其他属性就是定义该事件是否具有冒泡等等功能。
内置的事件会由浏览器根据某些操作进行触发,自定义的事件就需要人工触发。
dispatchEvent 函数就是用来触发某个事件:
element.dispatchEvent(customEvent);
上面代码表示,在 element 上面触发 customEvent 这个事件。
// add an appropriate event listener
obj.addEventListener("cat", function(e) { process(e.detail) });
// create and dispatch the event
var event = new CustomEvent("cat", {"detail":{"hazcheeseburger":true}});
obj.dispatchEvent(event);
使用自定义事件需要注意兼容性问题,而使用 jQuery 就简单多了:
// 绑定自定义事件
$(element).on('myCustomEvent', function(){});
// 触发事件
$(element).trigger('myCustomEvent');
// 此外,你还可以在触发自定义事件时传递更多参数信息:
$( "p" ).on( "myCustomEvent", function( event, myName ) {
$( this ).text( myName + ", hi there!" );
});
$( "button" ).click(function () {
$( "p" ).trigger( "myCustomEvent", [ "John" ] );
});
复制代码
183.如何从元素中移除事件监听器?
const off = (el, evt, fn, opts = false) => el.removeEventListener(evt, fn, opts);
const fn = () => console.log('!');
document.body.addEventListener('click', fn);
off(document.body, 'click', fn);
复制代码
184.如何获得给定毫秒数的可读格式?
const formatDuration = ms => {
if (ms < 0) ms = -ms;
const time = {
day: Math.floor(ms / 86400000),
hour: Math.floor(ms / 3600000) % 24,
minute: Math.floor(ms / 60000) % 60,
second: Math.floor(ms / 1000) % 60,
millisecond: Math.floor(ms) % 1000
};
return Object.entries(time)
.filter(val => val[1] !== 0)
.map(([key, val]) => `${val} ${key}${val !== 1 ? 's' : ''}`)
.join(', ');
};
// 事例
formatDuration(1001); // '1 second, 1 millisecond'
formatDuration(34325055574);
// '397 days, 6 hours, 44 minutes, 15 seconds, 574 milliseconds'
复制代码
185.如何获得两个日期之间的差异(以天为单位)?
const getDaysDiffBetweenDates = (dateInitial, dateFinal) =>
(dateFinal - dateInitial) / (1000 * 3600 * 24);
// 事例
getDaysDiffBetweenDates(new Date('2017-12-13'), new Date('2017-12-22')); // 9
复制代码
186.如何向传递的URL发出GET请求?
const httpGet = (url, callback, err = console.error) => {
const request = new XMLHttpRequest();
request.open('GET', url, true);
request.onload = () => callback(request.responseText);
request.onerror = () => err(request);
request.send();
};
httpGet(
'https://jsonplaceholder.typicode.com/posts/1',
console.log
);
// {"userId": 1, "id": 1, "title": "sample title", "body": "my text"}
复制代码
187.如何对传递的URL发出POST请求?
const httpPost = (url, data, callback, err = console.error) => {
const request = new XMLHttpRequest();
request.open('POST', url, true);
request.setRequestHeader('Content-type', 'application/json; charset=utf-8');
request.onload = () => callback(request.responseText);
request.onerror = () => err(request);
request.send(data);
};
const newPost = {
userId: 1,
id: 1337,
title: 'Foo',
body: 'bar bar bar'
};
const data = JSON.stringify(newPost);
httpPost(
'https://jsonplaceholder.typicode.com/posts',
data,
console.log
);
// {"userId": 1, "id": 1337, "title": "Foo", "body": "bar bar bar"}
复制代码
188.如何为指定选择器创建具有指定范围,步长和持续时间的计数器?
const counter = (selector, start, end, step = 1, duration = 2000) => {
let current = start,
_step = (end - start) * step < 0 ? -step : step,
timer = setInterval(() => {
current += _step;
document.querySelector(selector).innerHTML = current;
if (current >= end) document.querySelector(selector).innerHTML = end;
if (current >= end) clearInterval(timer);
}, Math.abs(Math.floor(duration / (end - start))));
return timer;
};
// 事例
counter('#my-id', 1, 1000, 5, 2000);
// 让 `id=“my-id”`的元素创建一个2秒计时器
复制代码
189.如何将字符串复制到剪贴板?
const el = document.createElement('textarea');
el.value = str;
el.setAttribute('readonly', '');
el.style.position = 'absolute';
el.style.left = '-9999px';
document.body.appendChild(el);
const selected =
document.getSelection().rangeCount > 0 ? document.getSelection().getRangeAt(0) : false;
el.select();
document.execCommand('copy');
document.body.removeChild(el);
if (selected) {
document.getSelection().removeAllRanges();
document.getSelection().addRange(selected);
}
};
// 事例
copyToClipboard('Lorem ipsum');
// 'Lorem ipsum' copied to clipboard
复制代码
190.如何确定页面的浏览器选项卡是否聚焦?
const isBrowserTabFocused = () => !document.hidden;
// 事例
isBrowserTabFocused(); // true
复制代码
191.如何创建目录(如果不存在)?
const fs = require('fs');
const createDirIfNotExists = dir => (!fs.existsSync(dir) ? fs.mkdirSync(dir) : undefined);
// 事例
createDirIfNotExists('test');
这里面的方法大都挺实用,可以解决很多开发过程问题,大家就好好利用起来吧。
复制代码
192.日期型函数封装
function formatTime(date) {
if(!!date){
if(!(date instanceof Date))
date = new Date(date);
var month = date.getMonth() + 1
var day = date.getDate()
return `${month}月${day}日`;
}
}
function formatDay(date) {
if(!!date){
var year = date.getFullYear()
var month = date.getMonth() + 1
var day = date.getDate()
return [year, month, day].map(formatNumber).join('-');
}
}
function formatDay2(date) {
if(!!date){
var year = date.getFullYear()
var month = date.getMonth() + 1
var day = date.getDate()
return [year, month, day].map(formatNumber).join('/');
}
}
function formatWeek(date){
if(!!date){
var day = date.getDay();
switch (day) {
case 0:
return '周日'
break;
case 1:
return '周一'
break;
case 2:
return '周二'
break;
case 3:
return '周三'
break;
case 4:
return '周四'
break;
case 5:
return '周五'
break;
case 6:
return '周六'
break;
}
}
}
function formatHour(date){
if(!!date){
var hour = new Date(date).getHours();
var minute = new Date(date).getMinutes();
return [hour, minute].map(formatNumber).join(':');
}
}
function timestamp(date, divisor=1000){
if(date == undefined){
return;
}else if(typeof date == 'number'){
return Math.floor(date/divisor);
}else if(typeof date == 'string'){
var strs = date.split(/[^0-9]/);
return Math.floor(+new Date(strs[0] || 0,(strs[1] || 0)-1,strs[2] || 0,strs[3] || 0,strs[4] || 0,strs[5] || 0)/divisor);
}else if(Date.prototype.isPrototypeOf(date)){
return Math.floor(+date/divisor);
}
}
function detimestamp(date){
if(!!date){
return new Date(date*1000);
}
}
function formatNumber(n) {//给在0-9的日期加上0
n = n.toString()
return n[1] ? n : '0' + n
}
module.exports = {
formatTime: formatTime,
formatDay: formatDay,
formatDay2: formatDay2,
formatHour: formatHour,
formatWeek: formatWeek,
timestamp: timestamp,
detimestamp: detimestamp
}
复制代码
193.时间戳转时间
/**
* 时间戳转化为年 月 日 时 分 秒
* number: 传入时间戳
* format:返回格式,支持自定义,但参数必须与formateArr里保持一致
*/
function formatTime(number,format) {
var formateArr = ['Y','M','D','h','m','s'];
var returnArr = [];
var date = new Date(number * 1000);
returnArr.push(date.getFullYear());
returnArr.push(formatNumber(date.getMonth() + 1));
returnArr.push(formatNumber(date.getDate()));
returnArr.push(formatNumber(date.getHours()));
returnArr.push(formatNumber(date.getMinutes()));
returnArr.push(formatNumber(date.getSeconds()));
for (var i in returnArr)
{
format = format.replace(formateArr[i], returnArr[i]);
}
return format;
}
//数据转化
function formatNumber(n) {
n = n.toString()
return n[1] ? n : '0' + n
}
调用示例:
var sjc = 1488481383;//时间戳
console.log(time.formatTime(sjc,'Y/M/D h:m:s'));//转换为日期:2017/03/03 03:03:03
console.log(time.formatTime(sjc, 'h:m'));//转换为日期:03:03
复制代码
194.js中获取上下文路径
js中获取上下文路径
//js获取项目根路径,如: http://localhost:8083/uimcardprj
function getRootPath(){
//获取当前网址,如: http://localhost:8083/uimcardprj/share/meun.jsp
var curWwwPath=window.document.location.href;
//获取主机地址之后的目录,如: uimcardprj/share/meun.jsp
var pathName=window.document.location.pathname;
var pos=curWwwPath.indexOf(pathName);
//获取主机地址,如: http://localhost:8083
var localhostPaht=curWwwPath.substring(0,pos);
//获取带"/"的项目名,如:/uimcardprj
var projectName=pathName.substring(0,pathName.substr(1).indexOf('/')+1);
return(localhostPaht+projectName);
}
复制代码
195.JS大小转化B KB MB GB的转化方法
function conver(limit){
var size = "";
if( limit < 0.1 * 1024 ){ //如果小于0.1KB转化成B
size = limit.toFixed(2) + "B";
}else if(limit < 0.1 * 1024 * 1024 ){//如果小于0.1MB转化成KB
size = (limit / 1024).toFixed(2) + "KB";
}else if(limit < 0.1 * 1024 * 1024 * 1024){ //如果小于0.1GB转化成MB
size = (limit / (1024 * 1024)).toFixed(2) + "MB";
}else{ //其他转化成GB
size = (limit / (1024 * 1024 * 1024)).toFixed(2) + "GB";
}
var sizestr = size + "";
var len = sizestr.indexOf(".");
var dec = sizestr.substr(len + 1, 2);
if(dec == "00"){//当小数点后为00时 去掉小数部分
return sizestr.substring(0,len) + sizestr.substr(len + 3,2);
}
return sizestr;
}
复制代码
196.js全屏和退出全屏
function fullScreen() {
var el = document.documentElement;
var rfs = el.requestFullScreen || el.webkitRequestFullScreen || el.mozRequestFullScreen || el.msRequestFullScreen;
//typeof rfs != "undefined" && rfs
if (rfs) {
rfs.call(el);
} else if (typeof window.ActiveXObject !== "undefined") {
//for IE,这里其实就是模拟了按下键盘的F11,使浏览器全屏
var wscript = new ActiveXObject("WScript.Shell");
if (wscript != null) {
wscript.SendKeys("{F11}");
}
}
}
//退出全屏
function exitScreen() {
var el = document;
var cfs = el.cancelFullScreen || el.webkitCancelFullScreen || el.mozCancelFullScreen || el.exitFullScreen;
//typeof cfs != "undefined" && cfs
if (cfs) {
cfs.call(el);
} else if (typeof window.ActiveXObject !== "undefined") {
//for IE,这里和fullScreen相同,模拟按下F11键退出全屏
var wscript = new ActiveXObject("WScript.Shell");
if (wscript != null) {
wscript.SendKeys("{F11}");
}
}
}
复制代码
197.格式化时间,转化为几分钟前,几秒钟前
/**
* 格式化时间,转化为几分钟前,几秒钟前
* @param timestamp 时间戳,单位是毫秒
*/
function timeFormat(timestamp) {
var mistiming = Math.round((Date.now() - timestamp) / 1000);
var arrr = ['年', '个月', '星期', '天', '小时', '分钟', '秒'];
var arrn = [31536000, 2592000, 604800, 86400, 3600, 60, 1];
for (var i = 0; i < arrn.length; i++) {
var inm = Math.floor(mistiming / arrn[i]);
if (inm != 0) {
return inm + arrr[i] + '前';
}
}
}
复制代码
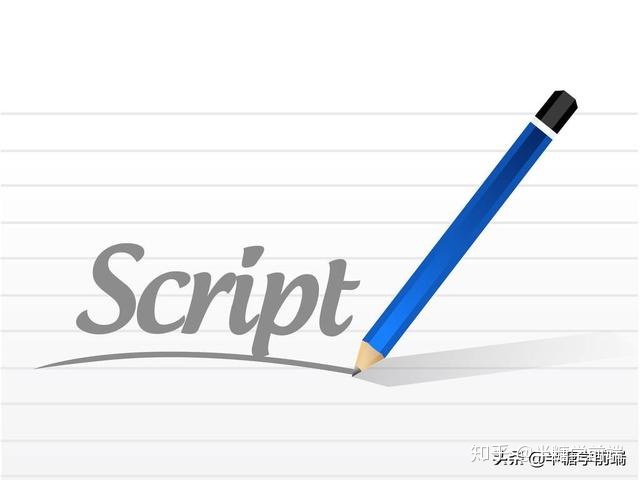
198 获取n天之前的日期 getDaysBeforeDate(10) 10天前
/**
* 获取n天之前的日期 getDaysBeforeDate(10) 10天前
* @param day 天数
*/
function getDaysBeforeDate(day) {
var date = new Date(),
timestamp, newDate;
timestamp = date.getTime();
// 获取三天前的日期
newDate = new Date(timestamp - day * 24 * 3600 * 1000);
var year = newDate.getFullYear();
// 月+1是因为js中月份是按0开始的
var month = newDate.getMonth() + 1;
var day = newDate.getDate();
if (day < 10) { // 如果日小于10,前面拼接0
day = '0' + day;
}
if (month < 10) { // 如果月小于10,前面拼接0
month = '0' + month;
}
return [year, month, day].join('/');
}
复制代码
199.获取跳转的classId,通过hash方式获取
/**
* 获取跳转的classId,通过hash方式获取
* @return 返回值
*/
$scope.getQueryString = function() {
var url= {},
a = '';
(a = window.location.search.substr(1)) || (a = window.location.hash.split('?')[1])
a.split(/&/g).forEach(function(item) {
url[(item = item.split('='))[0]] = item[1];
})
return url
}
复制代码
200.过滤器指定字段
function filterArrBySex(data, name) {
if (!name) {
console.log(name)
return data;
} else {
return data.filter(function(ele, index, self) {
if (ele.name.includes(name)) {
return ele
}
})
}
}
复制代码
201.根据身份证获取出生年月
/**
* 根据身份证获取出生年月
* @param idCard
*/
function getBirthdayFromIdCard(idCard) {
var birthday = "";
if (idCard != null && idCard != "") {
if (idCard.length == 15) {
birthday = "19" + idCard.substr(6, 6);
} else if (idCard.length == 18) {
birthday = idCard.substr(6, 8);
}
birthday = birthday.replace(/(.{4})(.{2})/, "$1-$2-");
}
return birthday;
}
复制代码
202.根据身份证获取年龄
/**
* 根据身份证获取年龄
* @param UUserCard
*/
function IdCard(UUserCard) {
//获取年龄
var myDate = new Date();
var month = myDate.getMonth() + 1;
var day = myDate.getDate();
var age = myDate.getFullYear() - UUserCard.substring(6, 10) - 1;
if (UUserCard.substring(10, 12) < month || UUserCard.substring(10, 12) == month && UUserCard.substring(12, 14) <= day) {
age++;
}
return age
}
复制代码
//vue购物车金额结算
window.onload = function() {
var vm = new Vue({
el: '#app',
data: {
items: [{
id: 1,
name: "苹果",
price: 10,
count: 1,
url: "http://www.jq22.com/img/cs/500x500-1.png"
},
{
id: 2,
name: "蝴蝶",
price: 8,
count: 5,
url: "http://www.jq22.com/img/cs/500x500-2.png"
},
{
id: 3,
name: "小狗",
price: 100,
count: 1,
url: "http://www.jq22.com/img/cs/500x500-3.png"
},
{
id: 4,
name: "鲜花",
price: 10,
count: 1,
url: "http://www.jq22.com/img/cs/500x500-4.png"
}
],
search: ""
},
methods: {
},
filters: { //过滤器
numFilter: function(data, n) { //data==item.price 当前过滤的数据 n==2
return "¥" + data.toFixed(n)
}
},
computed: { //计算属性
totalCount: function() {
var n = 0;
this.items.forEach((v, i) => {
n += v.count
});
return n;
},
totalPrice: function() {
var money = 0;
this.items.forEach((v, i) => {
money += v.count * v.price
});
return money;
},
searchFor: function() {
if (!this.search) {
return this.items
}
return this.items.filter((v, i) => {
if (v.name.indexOf(this.search) !== -1) { //匹配成功
return v
}
})
}
}
})
}
//设置iframe高度
function setIframeHeight(sonH) { //debugger;
var height = 0;
//比较父子页面高度,以高度更大的为准
var parentH = $(window).height(); //iframe最小高度应为浏览器视口高度,否则门户管理页面弹窗大小会受限制
height = parentH > sonH ? parentH : sonH;
$('#mainIframe').height(height);
//子页面有传值过来,覆盖iframe的最小高度-2000px
$("#mainIframe").css("min-height", 'auto');
$(".body-bg").css("height", height + 200);
try {
var childBody = $("#mainIframe")[0].contentWindow.document.getElementsByTagName("body")[0];
//childBody.style.minHeight = height + "px";
} catch (error) {
if (error.message.indexOf("Blocked from frame") > -1) console.warn("当前页面存在跨域!");
}
}
(function($) {
$("#username").keyup(function(event) {
if (event.keyCode == 13) $("#password").focus().select();
});
$("#password").keyup(function(event) {
if (event.keyCode == 13) $("#login-button").click();
});
})(jQuery);
//回到顶部
function goTop() {
window.scrollTo({
top: 0,
behavior: 'smooth',
});
}
window.onscroll = function() {
var t = document.documentElement.scrollTop;
if (t > 50) {
$(".toTop").fadeIn();
} else {
$(".toTop").fadeOut();
}
}
function urlAnalysis() {
var url = window.location.href;
var paraString = url.substring(url.indexOf("?") + 1, url.length);
var indexFirst = paraString.indexOf("&");
var paraStringTitle = paraString.slice(0, indexFirst);
paraStringElse = paraString.slice(indexFirst + 1, paraString.length);
var paraStringUrl = paraStringElse;
//区分是否传参:functionId
if (paraString.indexOf("functionId") > -1) {
var indexSecond = paraStringElse.indexOf("&");
var paraStringFId = paraStringElse.slice(0, indexSecond);
var functionId = paraStringFId.split("=")[1];
var $body = angular.element(document.body);
var $rootScope = $body.scope().$root;
$rootScope.navFunctionId = functionId;
paraStringUrl = paraStringElse.slice(indexSecond + 1, paraStringElse.length);
}
var title = paraStringTitle.split("=")[1] === "undefined" ? "" : decodeURI(paraStringTitle.split("=")[1]);
var indexUrl = paraStringUrl.indexOf("=");
var iframeUrl = paraStringUrl.slice(indexUrl + 1, paraStringUrl.length);
document.title = title;
$("#mainIframe").attr("src", iframeUrl);
}
// 监听enter按下事件,页面跳转
$scope.enterEvent = function(e) {
var keycode = window.event ? e.keyCode : e.which;
if (keycode == 13) {
console.log($scope.searchVal)
console.log('监听成功')
var baseUrl = '#/pages/knowlege/knowlegeSeach.html'
window.open(baseUrl)
}
};
function showDate() {
var myDate = new Date();
var year = myDate.getFullYear();
var month = myDate.getMonth() + 1;
month = month < 10 ? '0' + month : month;
var date = myDate.getDate();
date = date < 10 ? '0' + date : date;
var weekDay = myDate.getDay();
switch (weekDay) {
case 0:
weekDay = "天";
break;
case 1:
weekDay = "一";
break;
case 2:
weekDay = "二";
break;
case 3:
weekDay = "三";
break;
case 4:
weekDay = "四";
break;
case 5:
weekDay = "五";
break;
case 6:
weekDay = "六";
break;
"name"
}
$scope.year = year;
$scope.month = month;
$scope.date = date;
$scope.weekDay = weekDay;
}
//获取跳转的classId,通过hash方式获取
$scope.getQueryString = function() {
var obg = {},
a = '';
(a = window.location.search.substr(1)) || (a = window.location.hash.split('?')[1])
a.split(/&/g).forEach(function(item) {
obg[(item = item.split('='))[0]] = item[1];
})
return obg
}
var classId = $scope.getQueryString();
//vue购物车金额结算
window.onload = function() {
var vm = new Vue({
el: '#app',
data: {
items: [{
id: 1,
name: "苹果",
price: 10,
count: 1,
url: "http://www.jq22.com/img/cs/500x500-1.png"
},
{
id: 2,
name: "蝴蝶",
price: 8,
count: 5,
url: "http://www.jq22.com/img/cs/500x500-2.png"
},
{
id: 3,
name: "小狗",
price: 100,
count: 1,
url: "http://www.jq22.com/img/cs/500x500-3.png"
},
{
id: 4,
name: "鲜花",
price: 10,
count: 1,
url: "http://www.jq22.com/img/cs/500x500-4.png"
}
],
search: ""
},
methods: {
},
filters: { //过滤器
numFilter: function(data, n) { //data==item.price 当前过滤的数据 n==2
return "¥" + data.toFixed(n)
}
},
computed: { //计算属性
totalCount: function() {
var n = 0;
this.items.forEach((v, i) => {
n += v.count
});
return n;
},
totalPrice: function() {
var money = 0;
this.items.forEach((v, i) => {
money += v.count * v.price
});
return money;
},
searchFor: function() {
if (!this.search) {
return this.items
}
return this.items.filter((v, i) => {
if (v.name.indexOf(this.search) !== -1) { //匹配成功
return v
}
})
}
}
})
}
//设置iframe高度
function setIframeHeight(sonH) { //debugger;
var height = 0;
//比较父子页面高度,以高度更大的为准
var parentH = $(window).height(); //iframe最小高度应为浏览器视口高度,否则门户管理页面弹窗大小会受限制
height = parentH > sonH ? parentH : sonH;
$('#mainIframe').height(height);
//子页面有传值过来,覆盖iframe的最小高度-2000px
$("#mainIframe").css("min-height", 'auto');
$(".body-bg").css("height", height + 200);
try {
var childBody = $("#mainIframe")[0].contentWindow.document.getElementsByTagName("body")[0];
//childBody.style.minHeight = height + "px";
} catch (error) {
if (error.message.indexOf("Blocked from frame") > -1) console.warn("当前页面存在跨域!");
}
}
(function($) {
$("#username").keyup(function(event) {
if (event.keyCode == 13) $("#password").focus().select();
});
$("#password").keyup(function(event) {
if (event.keyCode == 13) $("#login-button").click();
});
})(jQuery);
//回到顶部
function goTop() {
window.scrollTo({
top: 0,
behavior: 'smooth',
});
}
window.onscroll = function() {
var t = document.documentElement.scrollTop;
if (t > 50) {
$(".toTop").fadeIn();
} else {
$(".toTop").fadeOut();
}
}
function urlAnalysis() {
var url = window.location.href;
var paraString = url.substring(url.indexOf("?") + 1, url.length);
var indexFirst = paraString.indexOf("&");
var paraStringTitle = paraString.slice(0, indexFirst);
paraStringElse = paraString.slice(indexFirst + 1, paraString.length);
var paraStringUrl = paraStringElse;
//区分是否传参:functionId
if (paraString.indexOf("functionId") > -1) {
var indexSecond = paraStringElse.indexOf("&");
var paraStringFId = paraStringElse.slice(0, indexSecond);
var functionId = paraStringFId.split("=")[1];
var $body = angular.element(document.body);
var $rootScope = $body.scope().$root;
$rootScope.navFunctionId = functionId;
paraStringUrl = paraStringElse.slice(indexSecond + 1, paraStringElse.length);
}
var title = paraStringTitle.split("=")[1] === "undefined" ? "" : decodeURI(paraStringTitle.split("=")[1]);
var indexUrl = paraStringUrl.indexOf("=");
var iframeUrl = paraStringUrl.slice(indexUrl + 1, paraStringUrl.length);
document.title = title;
$("#mainIframe").attr("src", iframeUrl);
}
// 监听enter按下事件,页面跳转
$scope.enterEvent = function(e) {
var keycode = window.event ? e.keyCode : e.which;
if (keycode == 13) {
console.log($scope.searchVal)
console.log('监听成功')
var baseUrl = '#/pages/knowlege/knowlegeSeach.html'
window.open(baseUrl)
}
};
function showDate() {
var myDate = new Date();
var year = myDate.getFullYear();
var month = myDate.getMonth() + 1;
month = month < 10 ? '0' + month : month;
var date = myDate.getDate();
date = date < 10 ? '0' + date : date;
var weekDay = myDate.getDay();
switch (weekDay) {
case 0:
weekDay = "天";
break;
case 1:
weekDay = "一";
break;
case 2:
weekDay = "二";
break;
case 3:
weekDay = "三";
break;
case 4:
weekDay = "四";
break;
case 5:
weekDay = "五";
break;
case 6:
weekDay = "六";
break;
"name"
}
$scope.year = year;
$scope.month = month;
$scope.date = date;
$scope.weekDay = weekDay;
}
//获取跳转的classId,通过hash方式获取
$scope.getQueryString = function() {
var obg = {},
a = '';
(a = window.location.search.substr(1)) || (a = window.location.hash.split('?')[1])
a.split(/&/g).forEach(function(item) {
obg[(item = item.split('='))[0]] = item[1];
})
return obg
}
var classId = $scope.getQueryString();
本篇未完结,请留意下一篇