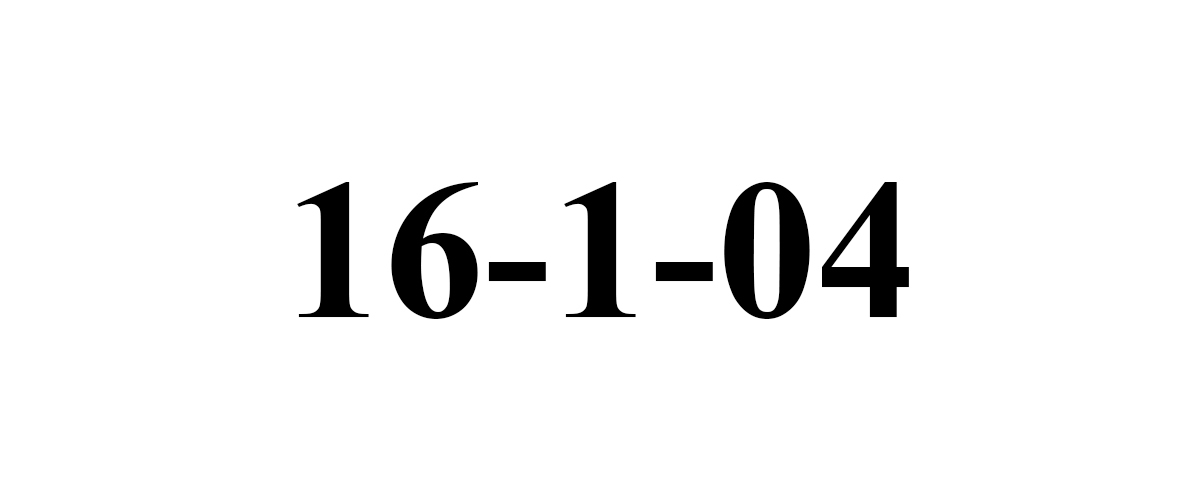
一、基础复习
1.前后端交互
通过浏览器调用接口,拿到后台的数据,再做前端的数据渲染。
2. 接口调用方式
因为Vue不操作DOM,所以用后两种
- ajax
- jQuery的ajax
- fetch
- axios
3. URL
3.1 传统的URL
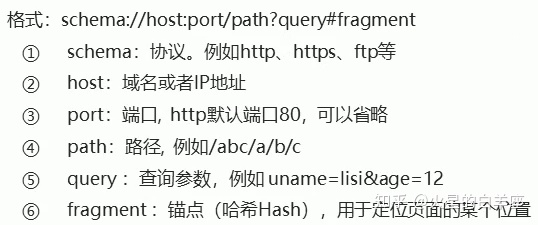
3.2 Restful格式的URL
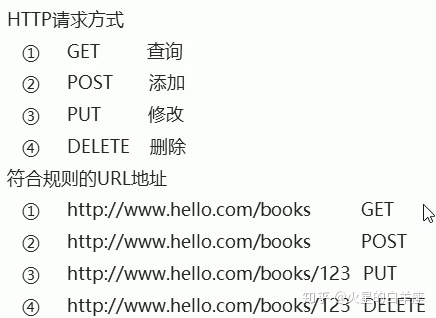
4. promise
ES6引入的一种语法,专门用来处理异步编程。
JS层面的异步编程有:
- 定时任务
- ajax
- 事件函数
4.1 演示jQuery的ajax的异步
先执行外面的,后执行里面的
路由
app.get('/data', (req, res) => {
res.send('Hello World!')
})
前端
var ret = '---';
$.ajax({
url: 'http://localhost:3000/data',
success: function(data) {
ret = data;
console.log(ret) // 2 Hello World!
}
});
console.log(ret) // 1 ---
控制台先打印 --- 后打印 Hello World!
多次写ajax请求,顺序无法控制
路由
app.get('/data', (req, res) => {
res.send('Hello World!')
})
app.get('/data1', (req, res) => {
setTimeout(function(){
res.send('Hello TOM!')
},1000);
})
app.get('/data2', (req, res) => {
res.send('Hello JERRY!')
})
前端
$.ajax({
url: 'http://localhost:3000/data',
success: function(data) {
console.log(data)
}
});
$.ajax({
url: 'http://localhost:3000/data1',
success: function(data) {
console.log(data)
}
});
$.ajax({
url: 'http://localhost:3000/data2',
success: function(data) {
console.log(data)
}
});
级别相同,没有顺序
若想要按顺序打印,需要嵌套(回调地狱)
$.ajax({
url: 'http://localhost:3000/data',
success: function(data) {
console.log(data)
$.ajax({
url: 'http://localhost:3000/data1',
success: function(data) {
console.log(data)
$.ajax({
url: 'http://localhost:3000/data2',
success: function(data) {
console.log(data)
}
});
}
});
}
});
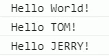
4.2 promise演示
- 解决回调地狱问题
- 简洁的API,更容易控制异步操作
简单演示
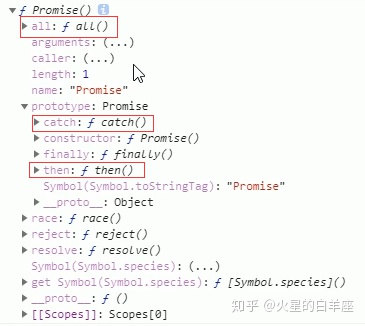
var p = new Promise(function(resolve, reject){
// 这里用于实现异步任务
setTimeout(function(){
var flag = false;
if(flag) {
resolve('hello');
}else{
reject('出错了');
}
}, 100);
});
p.then(function(data){
console.log(data) // flag为true打印hello
},function(info){
console.log(info) // flag改为false打印 出错了
});
成功时触发resolve,失败触发re