用Python打造微信天气预报机器人
16051502 姬利君
最近杭州的天气越来越变化多端,动不动就下雨。所以,拥有一款好的天气预报工具,对于我们大学生来说,还真是挺重要的了。自己动手,丰衣足食,我们来用Python打造一个天气预报的微信机器人吧。
- [ ] 环境配置:
Python3.7
Windows10
- [ ] 相关模块:
json模块;
requests模块;
itchat模块;
以及一些Python自带的模块。
1.获取天气
主要原理很简单,首先,需要找一个天气的API接口(这里我们使用的是http://api.map.baidu.com/telematics/v3/weather?location=%s&output=json&ak=TueGDhCvwI6fOrQnLM0qmXxY9N0OkOiQ&callback=?),使用requests发起请求,接受返回的结果,用python中内置的包json 将json字符串转换为python的字典或列表,然后从字典中取出数据。
具体可以看代码:
city = input('请输入要查询的城市名称:')
url = 'http://api.map.baidu.com/telematics/v3/weather?location=%s&output=json&ak=TueGDhCvwI6fOrQnLM0qmXxY9N0OkOiQ&callback=?'%city
# 使用requests发起请求,接受返回的结果
rs = requests.get(url)
# 使用loads函数,将json字符串转换为python的字典或列表
rs_dict = json.loads(rs.text)
# 取出error
error_code = rs_dict['error']
# 如果取出的error为0,表示数据正常,否则没有查询到结果
if error_code == 0:
# 从字典中取出数据
results = rs_dict['results']
# 根据索引取出天气信息字典
info_dict = results[0]
# 根据字典的key,取出城市名称
city_name = info_dict['currentCity']
# 取出pm值
pm25 = info_dict['pm25']
print('当前城市:%s pm值:%s'%(city_name,pm25))
# 取出天气信息列表
weather_data = info_dict['weather_data']
# for循环取出每一天天气的小字典
for weather_dict in weather_data:
# 取出日期,天气,风级,温度
date = weather_dict['date']
weather = weather_dict['weather']
wind = weather_dict['wind']
temperature = weather_dict['temperature']
print('%s %s %s %s'%(date,weather,wind,temperature))
2.发送天气预报
在获取到天气预报的数据以后,接下来就是通过itchat模块把信息发送到我们的微信上面了。原理也很简单,先扫码登录我们的微信机器人,然后通过备注名获取要发送的好友,send过去就OK啦。
具体看下面代码:
nickname = input('please input your friends' nickname : ' )
# 想给谁发信息,先查找到这个朋友,name后填微信备注即可
users = itchat.search_friends(name=nickname)
# users = itchat.search_friends(name='起风了') # 使用备注名来查找实际用户名
# 获取好友全部信息,返回一个列表,列表内是一个字典
print(users)
# 获取`UserName`,用于发送消息
userName = users[0]['UserName']
itchat.send(date+weather+wind+temperature, toUserName=userName)
- 定时预报:
如果想实现定时预报,可以在程序中加个while True:,然后每天定时获取天气,send过去。但这依赖于你的程序一直处于运行中,当然,如果你有一个云主机,把程序挂在主机上面,就可以实现定时预报啦。
- 向特定好友发送天气预报的完整代码:
```python # 天气预告 # url 统一资源定位符 # 引入 requests import requests # 引入python中内置的包json. 用来解析和生成json数据的 import json import itchat
def weather_main(): city = input('请输入要查询的城市名称:') url = 'http://api.map.baidu.com/telematics/v3/weather?location=%s&output=json&ak=TueGDhCvwI6fOrQnLM0qmXxY9N0OkOiQ&callback=?'%city # 使用requests发起请求,接受返回的结果 rs = requests.get(url) # 使用loads函数,将json字符串转换为python的字典或列表 rs_dict = json.loads(rs.text) # 取出error error_code = rs_dict['error'] # 如果取出的error为0,表示数据正常,否则没有查询到结果 if error_code == 0: # 从字典中取出数据 results = rs_dict['results'] # 根据索引取出天气信息字典 info_dict = results[0] # 根据字典的key,取出城市名称 city_name = info_dict['currentCity'] # 取出pm值 pm25 = info_dict['pm25'] print('当前城市:%s pm值:%s'%(city_name,pm25)) # 取出天气信息列表 weather_data = info_dict['weather_data'] # for循环取出每一天天气的小字典 for weather_dict in weather_data: # 取出日期,天气,风级,温度 date = weather_dict['date'] weather = weather_dict['weather'] wind = weather_dict['wind'] temperature = weather_dict['temperature'] print('%s %s %s %s'%(date,weather,wind,temperature))
nickname = input('please input your friends' nickname : ' )
# 想给谁发信息,先查找到这个朋友,name后填微信备注即可
users = itchat.search_friends(name=nickname)
# users = itchat.search_friends(name='起风了') # 使用备注名来查找实际用户名
# 获取好友全部信息,返回一个列表,列表内是一个字典
print(users)
# 获取`UserName`,用于发送消息
userName = users[0]['UserName']
itchat.send(date+weather+wind+temperature, toUserName=userName)
print('succeed')
else:
print('没有查询到天气信息')
itchat.auto_login() weather_main() ```
3.通过关键字发送天气预报
关键字回复
前面我们已经实现了天气的获取和发送,接下来利用itchat的几个功能,实现关键词的提取和发送相关信息。
在这里呢,我们需要写一个函数装饰一下itchat.msg_register([TEXT])这个函数,表示收到消息以后,额外执行一些我们期望执行的操作。
# 如果对方发的是文字,则我们给对方回复以下的东西
@itchat.msg_register([TEXT])
def text_reply(msg):
match = re.search('天气',msg['Text'])
if match:
city = msg['Text'][msg['Text'].find("+")+1:]
weather_main(msg['FromUserName'], city)
这部分操作也很简单,首先获取我们收到的文本消息,然后在里面找找看有没有我们想要的关键词,比如“天气”等,最后,将天气后面的城市给提取出来,获取必要的信息后,将发信人,城市传给上面写好的weather_main()函数里面,实现消息发送。当然,上面的weather_main()也要做相应修改,不过改动不大,大家看最后代码即可。
定时发送
这个功能就比较简单了,一个sleep函数睡到底就行。主要是设置隔多长时间给特定的人发送天气预报。
def timer(n):
"""
每n秒执行一次
"""
while True:
weather_main("阳", "杭州") # 此处为要执行的任务
time.sleep(n)
完整代码:
# 天气预告
# url 统一资源定位符
# windows + r cmd 打开命令行工具 输入pip install requests
# 引入 requests
import requests
# 引入python中内置的包json. 用来解析和生成json数据的
import json
import itchat
import re
from itchat.content import *
def weather_main(userName, theCity):
city = theCity
url = 'http://api.map.baidu.com/telematics/v3/weather?location=%s&output=json&ak=TueGDhCvwI6fOrQnLM0qmXxY9N0OkOiQ&callback=?' % city
# 使用requests发起请求,接受返回的结果
rs = requests.get(url)
# 使用loads函数,将json字符串转换为python的字典或列表
rs_dict = json.loads(rs.text)
# 取出error
error_code = rs_dict['error']
# 如果取出的error为0,表示数据正常,否则没有查询到结果
if error_code == 0:
# 从字典中取出数据
results = rs_dict['results']
# 根据索引取出天气信息字典
info_dict = results[0]
# 根据字典的key,取出城市名称
city_name = info_dict['currentCity']
# 取出pm值
pm25 = info_dict['pm25']
print('当前城市:%s pm值:%s'%(city_name,pm25))
# 取出天气信息列表
weather_data = info_dict['weather_data']
# for循环取出每一天天气的小字典
for weather_dict in weather_data:
# 取出日期,天气,风级,温度
date = weather_dict['date']
weather = weather_dict['weather']
wind = weather_dict['wind']
temperature = weather_dict['temperature']
print('%s %s %s %s'%(date,weather,wind,temperature))
itchat.send(date+weather+wind+temperature, toUserName=userName)
print('succeed')
else:
itchat.send("没有找到该城市的天气哦,是不是输入错误了。", toUserName=userName)
# 如果对方发的是文字,则我们给对方回复以下的东西
@itchat.msg_register([TEXT])
def text_reply(msg):
match = re.search('天气',msg['Text'])
if match:
city = msg['Text'][msg['Text'].find("+")+1:]
weather_main(msg['FromUserName'], city)
itchat.auto_login()
itchat.run()
def timer(n):
"""
每n秒执行一次
"""
while True:
weather_main("阳", "杭州") # 此处为要执行的任务
time.sleep(n)
效果展示
- 向指定好友发送天气预报:
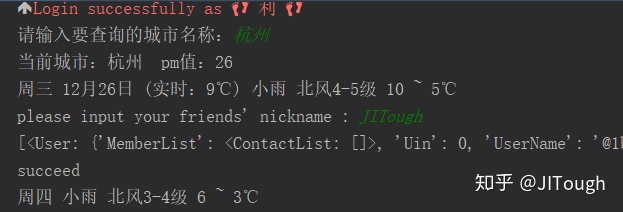
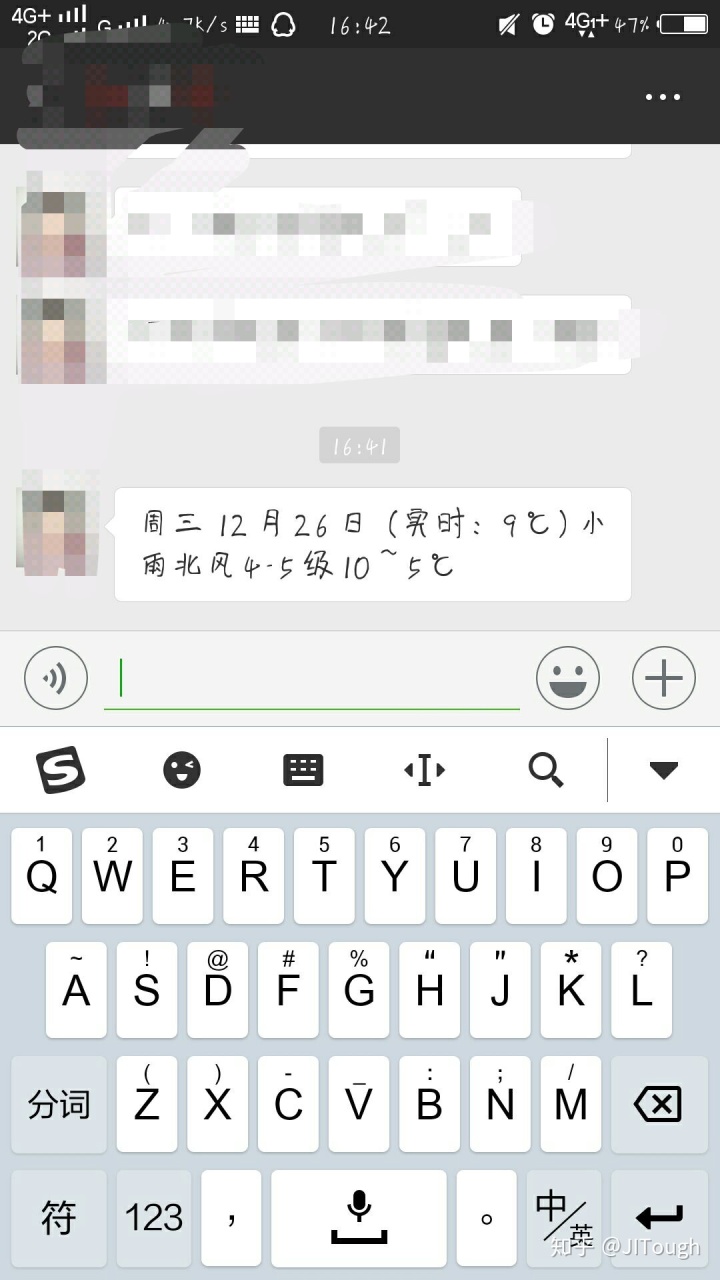
- 通过关键字发送天气预报
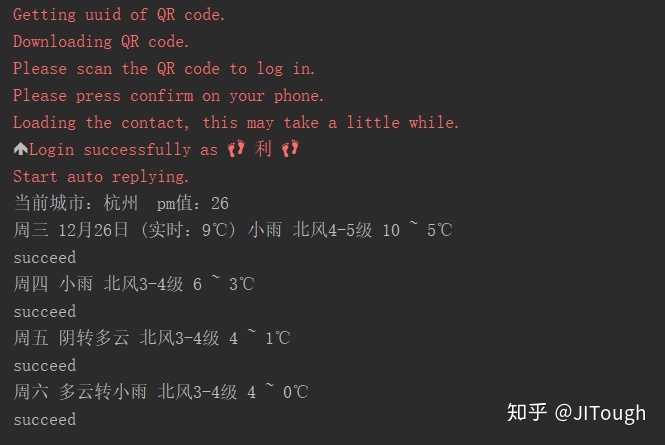
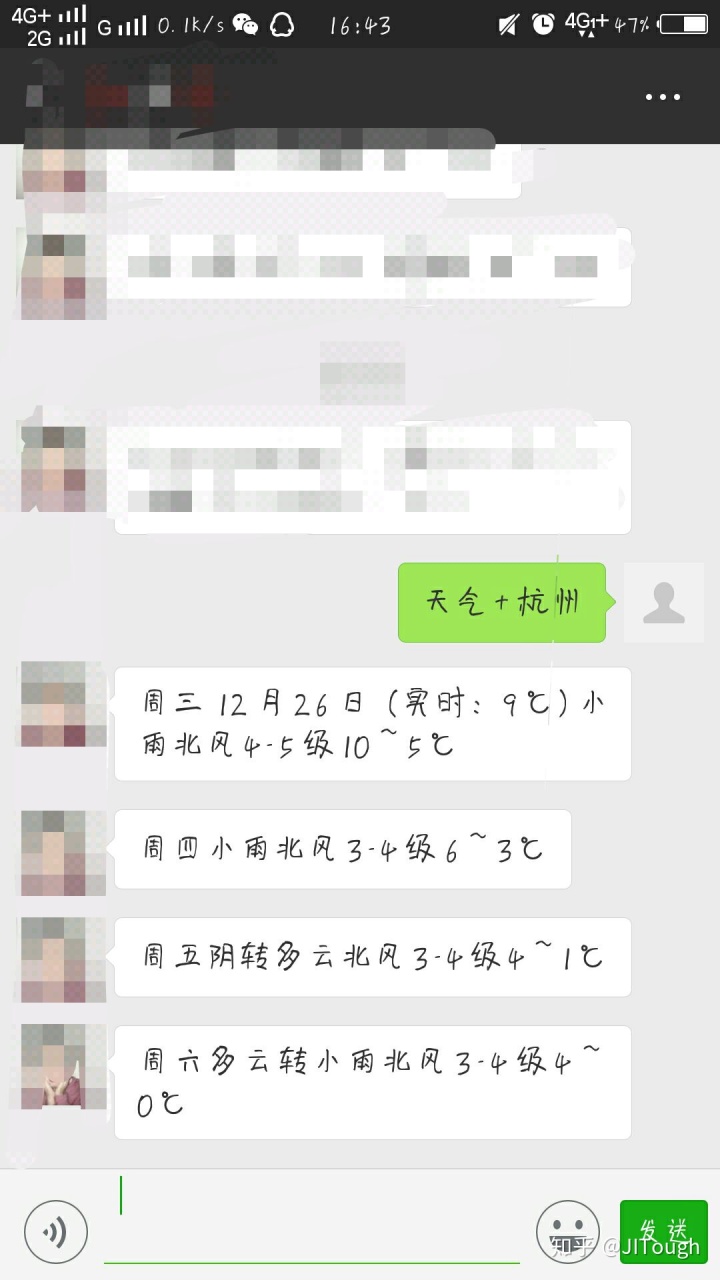