在任何Application的开发中, 对不可预知的异常进行troubleshooting时,异常处理显得尤为重要。对于一般的.NET系统来说,我们简单地借助try/catch可以很容 易地实现这一功能。但是对于 一个分布式的环境来说,异常处理就没有那么简单了。按照面向服务的原则,我们把一些可复用的业务逻辑以Service的形式实现,各个Service处于 一个自治的环境中,一个Service需要和另一个Service进行交互,只需要获得该Service的描述(Description)就可以了(比如 WSDL,Schema和Strategy)。借助标准的、平台无关的通信构架,各个Service之间通过标准的Soap Message进行交互。Service Description、Standard Communication Infrastructure、Soap Message based Communication促使各Service以松耦合的方式结合在一起。但是由于各个Service是自治的,如果一个Service调用另一个 Service,在服务提供方抛出的Exception必须被封装在Soap Message中,方能被处于另一方的服务的使用者获得、从而进行合理的处理。下面我们结合一个简单的Sample来简单地介绍我们可以通过哪些方式在 WCF中进行Exception Handling。
一、传统的Exception Handling
我们沿用我们一直使用的Calculator的例子和简单的4层构架:
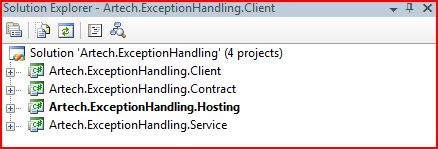
1. Service Contract- Artech.ExceptionHandling.Contract
using
System;
using
System.Collections.Generic;
using
System.Text;
using
System.ServiceModel;

namespace
Artech.ExceptionHandling.Contract
{
[ServiceContract]
public interface ICalculator
{
[OperationContract]
double Divide(double x, double y);
}
}
定义了一个单一的进行除法运算的Operation。
2. Service:Artech.ExceptionHandling.Service. CalculatorService
using
System;
using
System.Collections.Generic;
using
System.Text;
using
Artech.ExceptionHandling.Contract;

namespace
Artech.ExceptionHandling.Service
{
public class CalculatorService:ICalculator
{
ICalculator Members
}
}
如果被除数是零,抛出一个DivideByZeroException Exception。
3. Service Hosting
Configuration:
<?
xml version="1.0" encoding="utf-8"
?>
<
configuration
>
<
system
.serviceModel
>
<
behaviors
>
<
serviceBehaviors
>
<
behavior
name
="calculatorServiceBehavior"
>
<
serviceMetadata
httpGetEnabled
="true"
/>
</
behavior
>
</
serviceBehaviors
>
</
behaviors
>
<
services
>
<
service
behaviorConfiguration
="calculatorServiceBehavior"
name
="Artech.ExceptionHandling.Service.CalculatorService"
>
<
endpoint
binding
="basicHttpBinding"
bindingConfiguration
=""
contract
="Artech.ExceptionHandling.Contract.ICalculator"
/>
<
host
>
<
baseAddresses
>
<
add
baseAddress
="http://localhost:8888/Calculator"
/>
</
baseAddresses
>
</
host
>
</
service
>
</
services
>
</
system.serviceModel
>
</
configuration
>
Program
using
System;
using
System.Collections.Generic;
using
System.Text;
using
System.ServiceModel;
using
Artech.ExceptionHandling.Service;

namespace
Artech.ExceptionHandling.Hosting
{
class Program
{
static void Main(string[] args)
{
using (ServiceHost calculatorHost = new ServiceHost(typeof(CalculatorService)))
{
calculatorHost.Opened += delegate
{
Console.WriteLine("The Calculator service has begun to listen via the address:{0}", calculatorHost.BaseAddresses[0]);
};
calculatorHost.Open();
Console.Read();
}
}
}
}
4. Client
Configuration:
<?
xml version="1.0" encoding="utf-8"
?>
<
configuration
>
<
system
.serviceModel
>
<
client
>
<
endpoint
address
=http://localhost:8888/Calculator
binding
="basicHttpBinding"
contract
="Artech.ExceptionHandling.Contract.ICalculator"
name
="defualtEndpoint"
/>
</
client
>
</
system.serviceModel
>
</
configuration
>
Program
using
System;
using
System.Collections.Generic;
using
System.Text;
using
Artech.ExceptionHandling.Contract;
using
System.ServiceModel;

namespace
Artech.ExceptionHandling.Client
{
class Program
{
static void Main(string[] args)
{
ChannelFactory<ICalculator> calculatorFactory = new ChannelFactory<ICalculator>("defualtEndpoint");
ICalculator calculator = calculatorFactory.CreateChannel();
try
{
Console.WriteLine("Try to invoke Divide method");
Console.WriteLine("x / y = {2} when x = {0} and y = {1}", 2, 0, calculator.Divide(2,0));
}
catch (Exception ex)
{
Console.WriteLine("An Exception is thrown.\n\tException Type:{0}\n\tError Message:{1}", ex.GetType(), ex.Message);
}
Console.Read();
}
}
}
把Service调用放在一个try/catch block中,看看Service端抛出的DivideByZeroException Exception能否被Catch。
我们运行这个程序,看看Client有怎样的输出:
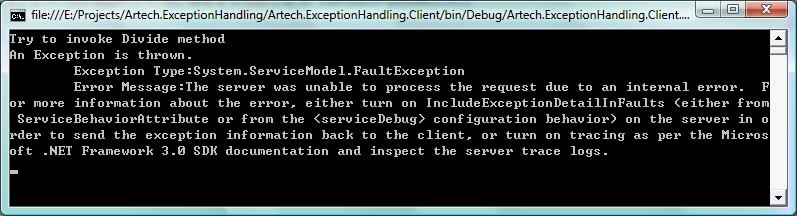
我们发现Client catch住的不是我们Service端真正抛出的DivideByZeroException Exception,而是一个比较General的FaultException。Error message也是很general:
"
The server was unable to process the request due to an internal error. For more information about the error, either turn on IncludeExceptionDetailInFaults (either from ServiceBehaviorAttribute or from the <serviceDebug> configuration behavior) on the server in order to send the exception information back to the client, or turn on tracing as per the Microsoft .NET Framework 3.0 SDK documentation and inspect the server trace logs.
"
二、基于ServiceDebug的Exception Handling
很显然Client端Catch住的 Exception对我们进行troubleshooting。为了利于我们进行有效的Debug,WCF提供了ServiceDebug Service Behavior。我们通过includeExceptionDetailInFaults属性设为true,那么如果Service抛出 Exception,WCF会简单得包装这个Exception并把它置于Soap中Response到Service的访问者。介于此,我修改了 Hosting的Configuration:
<?
xml version="1.0" encoding="utf-8"
?>
<
configuration
>
<
system
.serviceModel
>
<
behaviors
>
<
serviceBehaviors
>
<
behavior
name
="calculatorServiceBehavior"
>
<
serviceMetadata
httpGetEnabled
="true"
/>
<
serviceDebug
includeExceptionDetailInFaults
="true"
/>
</
behavior
>
</
serviceBehaviors
>
</
behaviors
>
<
services
>
<
service
behaviorConfiguration
="calculatorServiceBehavior"
name
="Artech.ExceptionHandling.Service.CalculatorService"
>
<
endpoint
binding
="basicHttpBinding"
bindingConfiguration
=""
contract
="Artech.ExceptionHandling.Contract.ICalculator"
/>
<
host
>
<
baseAddresses
>
<
add
baseAddress
="http://localhost:8888/Calculator"
/>
</
baseAddresses
>
</
host
>
</
service
>
</
services
>
</
system.serviceModel
>
</
configuration
>
现在再次运行程序,看看现在的运行结果:

可 以看到我们我们Catch的是一个FaultException< ExceptionDetail>Type的Exception,不是原来的FaultException。该Exception的Detail属 性就是Service抛出的DivideByZeroException Exception。有兴趣的人可以自己测试一下。而且我们在Service端指定的Error Message也被Client获得。这种方式的Exception Handling方式确实比上面一种具有很强的指示性,对我们进行Debug确实很有帮助。但是这种方式确实不能正式用于我们最终发布的版本中,因为它会 把Exception所有的信息返回到Client端,很容易泄露一些很敏感的信息。这也正是WCF把这个列入ServiceDebug Service Behavior的原因。
三、基于Fault Contract 的Exception Handling
既然上面通过定制ServiceDebug只能 用于Debug阶段。我们必须寻求另外一种Exception Handling的方式。那就是我们现在将要介绍的基于FaultContract的解决方案。我们知道WCF采用一种基于 Contract,Contract定义了进行交互的双方进行消息交换所遵循的准则和规范。Service Contract定义了包含了所有Operation的Service的接口,Data Contract定义了交互的数据的结构,而FaultContract实际上定义需要再双方之间进行交互的了异常、错误的表示。我们现在来看看如何来使 用基于FaultContract的Exception Handling。
我们首先来定义一个表示Fault的类:MathError。考虑到这个类需要在Service 和Client使用,我把它定义在Artech.ExceptionHandling.Contract中:
using
System;
using
System.Collections.Generic;
using
System.Text;
using
System.Runtime.Serialization;

namespace
Artech.ExceptionHandling.Contract
{
[DataContract]
public class MathError
{
private string _operation;
private string _errorMessage;

public MathError(string operation, string errorMessage)
{
this._operation = operation;
this._errorMessage = errorMessage;
}

[DataMember]
public string Operation
{
get { return _operation; }
set { _operation = value; }
}

[DataMember]
public string ErrorMessage
{
get { return _errorMessage; }
set { _errorMessage = value; }
}
}
}
在MathError中定义了两个成员:表示出 错操作的Operation和出错信息的ErrorMessage。由于该类的对象需要在Endpoint之间传递,所以必须是可序列化的,在WCF中, 我们一般用两个不同的Serializer实现Object和XML的Serialization和 Deserialization:Datacontract Serializer和XML Serializer。而对于Fault,只能使用前者。
定义了MathError,我们需要通过FaultContract将其运用到Service Contract中制定的Operation上面,我们通过下面的方式来实现:
using
System;
using
System.Collections.Generic;
using
System.Text;
using
System.ServiceModel;

namespace
Artech.ExceptionHandling.Contract
{
[ServiceContract]
public interface ICalculator
{
[OperationContract]
[FaultContract(typeof(MathError))]
double Divide(double x, double y);
}
}
我们在Divide上运用了 FaultContract,并指定了封装了Fault对应的类型,那么最终这个基于MathError类型的FaultContract会被写入 Service Description中,Client通过获取该Service Description(一般是获取WSDL),它就被识别它,就会将从接收到的Soap中对该Fault的XML Mapping到具体的MathError类型。
接着我们在Service Implementation中以抛出Exception的方式植入这个MathError对象:
using
System;
using
System.Collections.Generic;
using
System.Text;
using
Artech.ExceptionHandling.Contract;
using
System.ServiceModel;

namespace
Artech.ExceptionHandling.Service
{
public class CalculatorService:ICalculator
{
ICalculator Members
}
}
在被除数为0的时候,抛出FaultException<MathError> Exception,并指定具体的MathError对象,以及一个FaultCode(一般指明出错的来源)和FaultReason(出错的原因)。
我们现在先不修改Client的Exception Handling的相关代码,先运行Hosting,看看WSDL中什么特别之处:
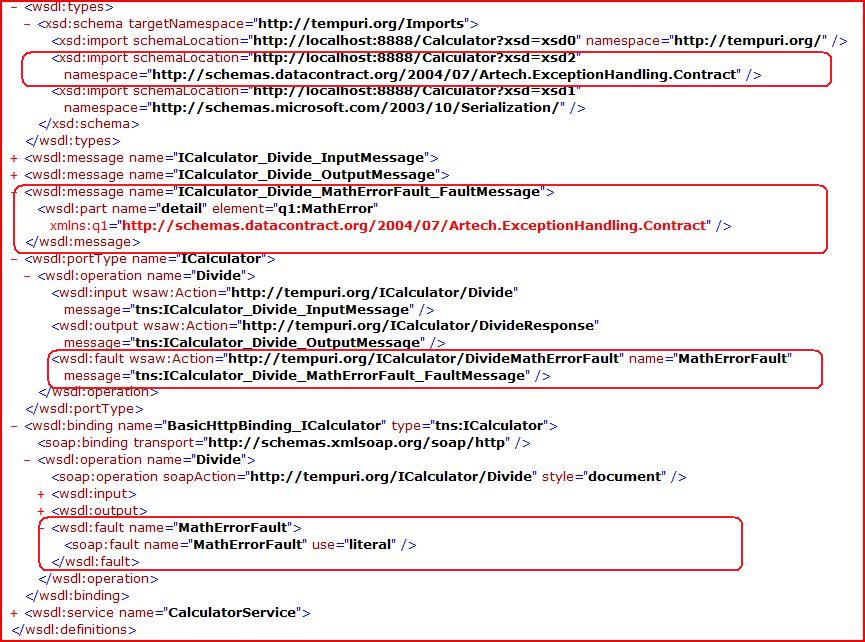
通 过上面的Screenshot,我们可以看到,在PortType section中的Divide Operation定义了Message为tns:ICalculator_Divide_MathErrorFault_FaultMessage 的<wsdl:fault>节点。通过查看Message Section,我们发现tns:ICalculator_Divide_MathErrorFault_FaultMessage的Element为 q1:MathError,该q1:MathError type实际上是被定义在一个XSD中,其Uri为http://localhost:8888/Calculator?xsd=xsd2,我们定义的所 有DataContract都在其中,下面的整个内容:
<?
xml version="1.0" encoding="utf-8"
?>
<
xs:schema
elementFormDefault
="qualified"
targetNamespace
="http://schemas.datacontract.org/2004/07/Artech.ExceptionHandling.Contract"
xmlns:xs
="http://www.w3.org/2001/XMLSchema"
xmlns:tns
="http://schemas.datacontract.org/2004/07/Artech.ExceptionHandling.Contract"
>
<
xs:complexType
name
="MathError"
>
<
xs:sequence
>
<
xs:element
minOccurs
="0"
name
="ErrorMessage"
nillable
="true"
type
="xs:string"
/>
<
xs:element
minOccurs
="0"
name
="Operation"
nillable
="true"
type
="xs:string"
/>
</
xs:sequence
>
</
xs:complexType
>
<
xs:element
name
="MathError"
nillable
="true"
type
="tns:MathError"
/>
</
xs:schema
>
弄清楚了Fault在WSDL中表示后,我们来修改我们Client端的代码,来有效地进行Exception Handling:
static
void
Main(
string
[] args)
{
ChannelFactory<ICalculator> calculatorFactory = new ChannelFactory<ICalculator>("defualtEndpoint");
ICalculator calculator = calculatorFactory.CreateChannel();
try
{
Console.WriteLine("Try to invoke Divide method");
Console.WriteLine("x / y = {2} when x = {0} and y = {1}", 2, 0, calculator.Divide(2, 0));
}
catch (FaultException<MathError> ex)
{
MathError error = ex.Detail;
Console.WriteLine("An Fault is thrown.\n\tFault code:{0}\n\tFault Reason:{1}\n\tOperation:{2}\n\tMessage:{3}", ex.Code, ex.Reason, error.Operation, error.ErrorMessage);
}

catch (Exception ex)
{
Console.WriteLine("An Exception is thrown.\n\tException Type:{0}\n\tError Message:{1}", ex.GetType(), ex.Message);
}
Console.Read();
}
下面是运行后的输出结果:
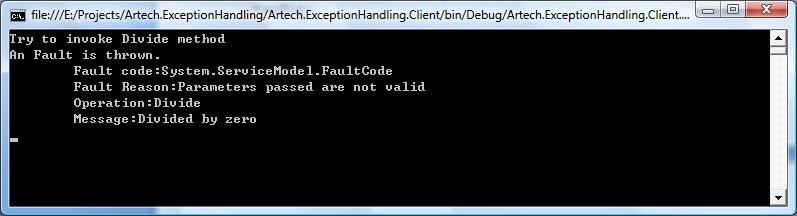