1 效果图
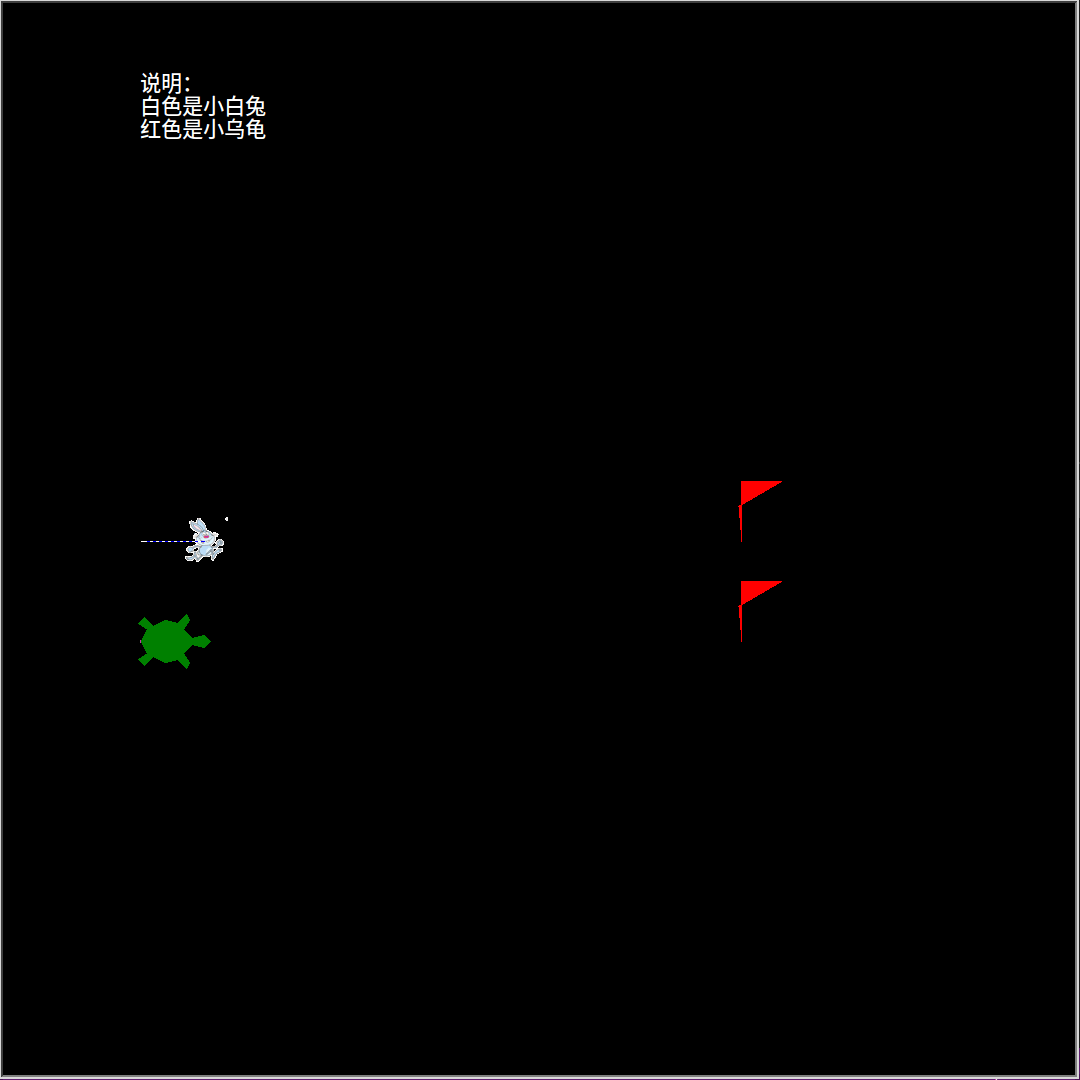
2 知识点
======
2.1 turtle的动画设计,通过游戏设计,增强学习兴趣。
2.2 turtle.shape已有图形调用和自定义shape图形(gif格式)。
2.3 python编程设计思维和相关知识复习。
2.4 代码讲解清楚,小白秒懂,一秒入门,值得收藏。
2.5 python3.8+deepin-linux操作系统。
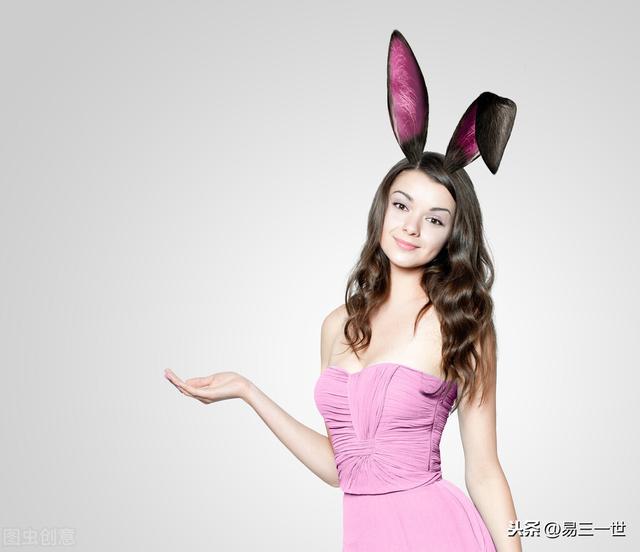
3 图片初步处理
============
3.1 图片来源今日头条正版免费图库,截图第2个兔子。
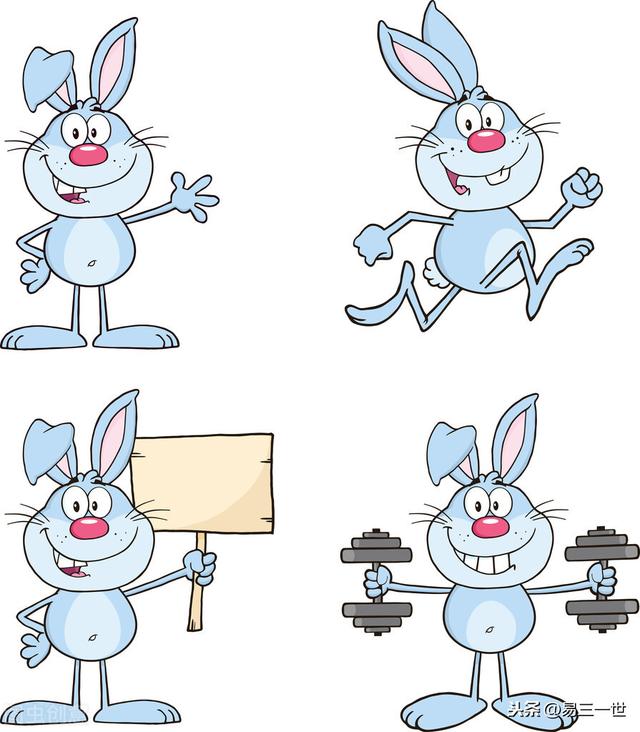
3.2 截图成1.png,然后将图片背景透明化处理
import PIL.Image as Image# 以第一个像素为准,相同色改为透明def transparent_back(img): img = img.convert('RGBA') L, H = img.size color_0 = img.getpixel((0,0)) for h in range(H): for l in range(L): dot = (l,h) color_1 = img.getpixel(dot) if color_1 == color_0: color_1 = color_1[:-1] + (0,) img.putpixel(dot,color_1) return imgif __name__ == '__main__': img=Image.open('/home/xgj/Desktop/guiturace/1.png') img=transparent_back(img) img.save('/home/xgj/Desktop/guiturace/11.png')
3.3 然后将图片11.png大小缩小到48*48
from PIL import Image def Image_PreProcessing():# 待处理图片存储路径im = Image.open('/home/xgj/Desktop/guiturace/11.png')# Resize图片大小,入口参数为一个tuple,新的图片大小imBackground = im.resize((48,48))#处理后的图片的存储路径,以及存储格式imBackground.save('/home/xgj/Desktop/guiturace/111.png','PNG')if __name__ == "__main__":Image_PreProcessing()
3.4 新建文件夹png,将111.png复制6个,取名为1~6.png,生成/png/rabbit.gif
import imageio,osfrom PIL import ImageGIF=[]filepath="/home/xgj/Desktop/guiturace/png"#文件路径filenames=os.listdir(filepath)for filename in os.listdir(filepath): GIF.append(imageio.imread(filepath+"/"+filename))#这个duration是播放速度,数值越小,速度越快imageio.mimsave(filepath+"/"+'rabbit.gif',GIF,duration=0.1)
3.5 效果图

4 turtle版龟兔赛跑的主代码
====================
4.1 第1步:导入模块
from turtle import *import turtle as t
4.2 第2步:大窗口设置,自定义图形先注册和文字说明
#设置标题t.title("龟兔赛跑turtle版")#设置背景色t.bgcolor("black")#需要在这里注册自定义的图形#仅仅支持gif格式t.register_shape('/home/xgj/Desktop/guiturace/png/rabbit.gif')#书写文字说明t.goto(-400,400)t.pencolor('white')t.write('说明:白色是小白兔红色是小乌龟')t.ht() #隐藏画笔
4.3 画终点小红旗
#小白兔终点的小红旗t.penup()t.speed(1)t.goto(200,0)t.pendown()t.color("red")t.begin_fill()t.left(90)t.forward(60)t.right(90)t.forward(40)t.right(150)t.forward(50)t.end_fill()t.penup()t.ht()#小乌龟的终点的小红旗t.penup()t.speed(1)t.goto(200,-100)t.pendown()t.color("red")t.begin_fill()t.right(120)t.forward(60)t.right(90)t.forward(40)t.right(150)t.forward(50)t.end_fill()t.penup()t.ht()
4.4 第4步:定义小白兔
rabbit=Turtle()#定义兔rabbit.hideturtle()#让兔子隐形#获取已有图形#rabbit.shape('arrow')#兔子的形状#形状:“arrow”, “turtle”, “circle”, “square”, “triangle”, “classic”。#前面需要先注册自定义图形,后面才能调用自定义图形rabbit.shape('/home/xgj/Desktop/guiturace/png/rabbit.gif')rabbit.shapesize(3) #兔子的大小rabbit.color("white") #兔子的颜色rabbit.up()#将兔子移动到起点,准备比赛rabbit.back(400)#把兔子往后移400rabbit.showturtle()#让兔子显露出来rabbit.down()#兔子移动时划线def rabbitmove():#定义一个新的函数 兔子移动 if usedtime<50:#如果用时小于50 rabbit.forward(3)#兔子向前奔跑 rabbit.color("white")#兔子的颜色是白色 elif usedtime<415:#如果用时 50<=usedtime<415 兔子就去睡觉,位移不变 rabbit.forward(0)#兔子向前0 else:#否则就追赶 rabbit.forward(6)#兔子向前6 #颜色可变性,对自定义图形不变色 if usedtime%2==0: rabbit.color("white") else: rabbit.color("blue")
4.5 定义小乌龟
tortoise=Turtle()#引入乌龟图案tortoise.hideturtle()#让乌龟隐形tortoise.shape('turtle')#确定乌龟的形状tortoise.shapesize(3) #乌龟大小tortoise.color("purple")#确定乌龟的颜色tortoise.pensize(3)#确定笔画的粗细tortoise.up()#将乌龟移动到起点,准备比赛#小乌龟下移tortoise.goto(0,-100)tortoise.back(400)#将乌龟后移400tortoise.showturtle()#把乌龟显露出来tortoise.down()#乌龟移动时划线def tortoisemove():#定义一个新的函数 乌龟移动 tortoise.forward(1)#乌龟一直在缓慢的爬行 #颜色可变性 if usedtime%2==0: tortoise.color("green") else: tortoise.color("red")
4.6 第6步:移动设置
usedtime=0#定义用时 赋初值为0def move():#定义函数 移动 global usedtime#全局形式 移动均参照usedtime rabbitposition = rabbit.xcor()#兔子的位置是兔子的x坐标 tortoiseposition = tortoise.xcor()#乌龟的位置是乌龟的x坐标 if max(rabbitposition,tortoiseposition)>200:#如果兔子和乌龟的位置中最大的超过200 writer= Turtle()#writer定义为Turtle writer.hideturtle()#隐藏乌龟(兔子) if tortoiseposition >200:#如果乌龟的位置大于200 msg='小乌龟赢了!' else: msg ='小白兔赢了!' writer.color("white") writer.write(msg, align='center',font=('simfang', 20,'bold')) else:#否则就移动 rabbitmove()#兔子移动 tortoisemove()#乌龟移动 ontimer(move,100)#定时让它们有动画效果 usedtime= usedtime+1#循环,用时+1
4.7 第7步:结尾
#第7步:定时器开启,移动参数ontimer(move,100)#比赛开始,定时#画面显示,并等待关闭done()
5 参考这里的代码,对下面的代码进行删减,修改,注释,增加功能等。感谢。
https://blog.csdn.net/qq_45381011/article/details/95789558?utm_medium=distribute.pc_relevant.none-task-blog-baidujs_baidulandingword-6&spm=1001.2101.3001.4242