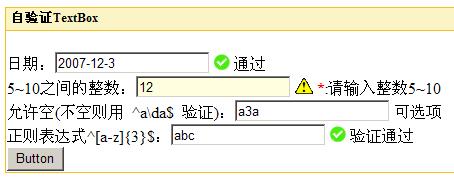
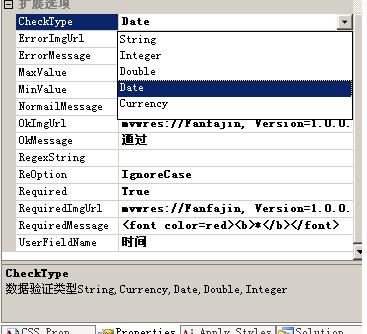
以下为代码
using
System;
using System.Data;
using System.Drawing;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.ComponentModel;
using System.Reflection;
using System.Text.RegularExpressions;
[assembly: System.Web.UI.WebResource( " Fanfajin.MyWebControls.MyTextBox.ok.gif " , " image/gif " )]
[assembly: System.Web.UI.WebResource( " Fanfajin.MyWebControls.MyTextBox.error.gif " , " image/gif " )]
[assembly: System.Web.UI.WebResource( " Fanfajin.MyWebControls.MyTextBox.required.gif " , " image/gif " )]
namespace Fanfajin.MyWebControls
{
/// <summary>
/// 自定义控件MyTextBox
/// </summary>
[ToolboxBitmap( typeof (TextBox)),
DefaultProperty( " Text " ),
ToolboxData( " <{0}:MyTextBox runat='server'></{0}:MyTextBox> " )]
public class MyTextBox : TextBox, IValidator
{
#region 私有变量
private bool _valid = true ; // 验证结果
private string _errorMessage = " 验证失败 " ; // 错误提示信息
private string _ErrorImgUrl = "" ; // 错误图片
private string _okMessage = " 验证通过 " ; // 验证通过提示信息
private string _OkImgUrl = "" ; // 验证通过图片
private string _requiedMessage = " <font color=red><b>*</b></font> " ; // 必填提示信息
private string _RequiredImgUrl = "" ; // 必填项图片地址
private string _normalMessage = " 可选项 " ;
private string _minValue = string .Empty;
private string _maxValue = string .Empty;
private string _userFieldName = string .Empty; // 验证域名称
private ValidationDataType _CheckType = ValidationDataType.String; // 验证数据类型
private string _Regex = string .Empty; // 正则表达式
private RegexOptions _RegexOptions = RegexOptions.IgnoreCase; // 匹配模式
#endregion
#region ViewState 保存视图的属性
/// <summary>
/// 是否必填
/// </summary>
[Category( " 扩展选项 " ),
Description( " 是否必填项 " )]
public bool Required
{
get
{
if (ViewState[ " Required " ] == null )
{
return true ;
}
return ( bool )ViewState[ " Required " ];
}
set
{
ViewState[ " Required " ] = value;
}
}
/// <summary>
/// 是否验证过了(不管有没有通过,就是看看控件是不是还没开始验证)
/// </summary>
[Description( " 是否验证过了(不管有没有通过,就是看看控件是不是还没开始验证) " )]
[Browsable( false )]
public bool IsChecked
{
get
{
if (ViewState[ " IsChecked " ] == null )
{
return false ;
}
return ( bool )ViewState[ " IsChecked " ];
}
set
{
ViewState[ " IsChecked " ] = value;
}
}
#endregion
#region 公开的属性成员
/// <summary>
/// 验证类型
/// </summary>
[Category( " 扩展选项 " )]
[Description( " 数据验证类型String,Currency,Date,Double,Integer " )]
public ValidationDataType CheckType
{
get { return _CheckType; }
set { _CheckType = value; }
}
/// <summary>
/// 最小值(字符串时 比较长度最小值)
/// </summary>
[Category( " 扩展选项 " ),
Description( " 最小值(字符串时比较长度最大值) " )]
public string MinValue
{
get { return _minValue; }
set { _minValue = value; }
}
/// <summary>
/// 最大值(字符串时 比较长度最大值)
/// </summary>
[Category( " 扩展选项 " ),
Description( " 最大值(字符串时 比较长度最大值) " )]
public string MaxValue
{
get { return _maxValue; }
set { _maxValue = value; }
}
/// <summary>
/// 验证域名称
/// </summary>
[Category( " 扩展选项 " ),
Description( " 验证域名称 " )]
public string UserFieldName
{
get
{
if ( this ._userFieldName == String.Empty)
this ._userFieldName = this .ID.Replace( " txt " , "" );
return this ._userFieldName;
}
set { this ._userFieldName = value; }
}
/// <summary>
/// 验证域正则表达式
/// </summary>
[Category( " 扩展选项 " ),
Description( " 验证域正则表达式 " ),
DefaultValue( "" )]
public string RegexString
{
get { return _Regex; }
set { _Regex = value; }
}
/// <summary>
/// 正则表达式匹配模式
/// </summary>
[Category( " 扩展选项 " ),
Description( " 正则表达式验证模式 " )]
public RegexOptions ReOption
{
get { return _RegexOptions; }
set { _RegexOptions = value; }
}
/// <summary>
/// 验证成功提示信息
/// </summary>
[Category( " 扩展选项 " ),
Description( " 验证成功提示信息 " )]
public string OkMessage
{
get { return _okMessage; }
set { _okMessage = value; }
}
/// <summary>
/// 必填项提示信息
/// </summary>
[Category( " 扩展选项 " ),
Description( " 必填项提示信息 " )]
public string RequiredMessage
{
get { return _requiedMessage; }
set { _requiedMessage = value; }
}
/// <summary>
/// 正常提示信息
/// </summary>
[Category( " 扩展选项 " ),
Description( " 正常提示信息 " )]
public string NormailMessage
{
get { return _normalMessage; }
set { _normalMessage = value; }
}
#endregion
#region IValidator接口成员
/// <summary>
/// IValidator接口成员错误信息
/// </summary>
[Category( " 扩展选项 " ),
Description( " 错误信息 " )]
public string ErrorMessage
{
get { return _errorMessage; }
set { _errorMessage = value; }
}
/// <summary>
/// IValidator接口成员
/// </summary>
[Description( " IValidator接口成员 是否通过了验证 " )]
[Browsable( false )]
public bool IsValid
{
get { return _valid; }
set
{
_valid = value;
if ( ! _valid)
{
this .BackColor = Color.LightYellow;
}
else
{
this .BackColor = Color.White;
}
}
}
/// <summary>
/// IValidate接口成员
/// </summary>
public void Validate()
{
this .IsValid = true ;
this .IsChecked = true ;
// 如果是空的话,看看是否允许空值
bool isBlank = ( this .Text.Trim() == "" );
if (isBlank)
{
if (Required)
{
this .ErrorMessage = String.Format( " '{0}' 不能为空! " , this .UserFieldName);
this .IsValid = false ;
}
else
{
this .IsValid = true ;
return ;
}
}
// 验证类型是否正确
bool isOk = BaseCompareValidator.CanConvert( this .Text, this ._CheckType);
if ( ! isOk)
{
// this.ErrorMessage = String.Format("'{0}' 请输入正确的类型" + this._CheckType.ToString(), this.UserFieldName);
this .ErrorMessage += " [类型错误] " ;
this .IsValid = false ;
return ;
}
// 验证最小值 MinValue与 Text对比
isOk = TextBoxCompare.DoCompare( this .Text, MinValue, ValidationCompareOperator.GreaterThanEqual, _CheckType);
if ( ! isOk)
{
this .ErrorMessage += String.Format( " 值或长度小于{0} " , this .MinValue);
this .IsValid = false ;
return ;
}
// 验证最大值 MaxValue与 Text对比
isOk = TextBoxCompare.DoCompare( this .Text, MaxValue, ValidationCompareOperator.LessThanEqual, _CheckType);
if ( ! isOk)
{
// this.ErrorMessage = String.Format("'{0}' 的值不能超过 {1}", this.UserFieldName, this.MaxValue);
this .IsValid = false ;
return ;
}
// 验证正则表达式
if ( this .RegexString != "" )
{
isOk = Regex.IsMatch( this .Text, this .RegexString, ReOption);
if ( ! isOk)
{
// this.ErrorMessage = String.Format("'{0}' 的格式不对!", this.UserFieldName);
this .IsValid = false ;
return ;
}
}
}
/// <summary>
/// 文本框后的图片地址
/// </summary>
[
Category( " 扩展选项 " ),
Description( " 验证失败时,文本框后面的图片URL地址 " )
]
public string ErrorImgUrl
{
get
{
if (_ErrorImgUrl == null || _ErrorImgUrl == "" )
{
return this .Page.ClientScript.GetWebResourceUrl( this .GetType(), " Fanfajin.MyWebControls.MyTextBox.error.gif " );
}
else
{
return _ErrorImgUrl;
}
}
set { _ErrorImgUrl = value; }
}
/// <summary>
/// 文本框后的图片地址
/// </summary>
[
Category( " 扩展选项 " ),
Description( " 验证成功时,文本框后面的图片URL地址 " )
]
public string OkImgUrl
{
get
{
if (_OkImgUrl == null || _OkImgUrl == "" )
{
// 返回默认的验证成功时的图片地址
return this .Page.ClientScript.GetWebResourceUrl( this .GetType(), " Fanfajin.MyWebControls.MyTextBox.ok.gif " );
}
else
{
return _OkImgUrl;
}
}
set { _OkImgUrl = value; }
}
/// <summary>
/// 文本框后的图片地址
/// </summary>
[
Category( " 扩展选项 " ),
Description( " 验证成功时,文本框后面的图片URL地址 " )
]
public string RequiredImgUrl
{
get
{
if (_RequiredImgUrl == null || _RequiredImgUrl == "" )
{
// 返回默认的验证成功时的图片地址
return this .Page.ClientScript.GetWebResourceUrl( this .GetType(), " Fanfajin.MyWebControls.MyTextBox.required.gif " );
}
else
{
return _RequiredImgUrl;
}
}
set { _RequiredImgUrl = value; }
}
#endregion
/// <summary>
/// OnInit
/// </summary>
/// <param name="e"></param>
protected override void OnInit(EventArgs e)
{
base .OnInit(e);
Page.Validators.Add( this );
}
/// <summary>
/// OnUnload
/// </summary>
/// <param name="e"></param>
protected override void OnUnload(EventArgs e)
{
if (Page != null )
{
Page.Validators.Remove( this );
}
base .OnUnload(e);
}
/// <summary>
/// 加入错误提示信息
/// </summary>
/// <param name="writer"></param>
protected override void Render(HtmlTextWriter writer)
{
base .Render(writer);
if (IsChecked)
{
if ( this .IsValid)
{
if (Required)
{
writer.Write( " <img src=\ "" + this.OkImgUrl + " \ " > " + this .OkMessage);
}
else
{
writer.Write( " " + this .NormailMessage);
}
}
else
{
if (Required)
{
writer.Write( " <img src=\ "" + this.ErrorImgUrl + " \ " > " + this .RequiredMessage + " : " + this .ErrorMessage);
}
else
{
writer.Write( " <img src=\ "" + this.ErrorImgUrl + " \ " > " + " ( " + this .NormailMessage + " ) " + this .RequiredMessage + " : " + this .ErrorMessage);
}
}
}
else // 还没开始验证
{
if (Required)
{
writer.Write( " <img src=\ "" + this.RequiredImgUrl + " \ " > " + this .RequiredMessage);
}
else
{
writer.Write( " " + this .NormailMessage + " " + this .RequiredMessage);
}
}
}
/// <summary>
/// 验证类(从 BaseCompareValidator 派生 )
/// </summary>
class TextBoxCompare : BaseCompareValidator
{
/// <summary>
/// 执行验证
/// </summary>
/// <param name="from"></param>
/// <param name="to"></param>
/// <param name="cmp"></param>
/// <param name="objType"></param>
/// <returns></returns>
public static bool DoCompare( string from, string to, ValidationCompareOperator cmp, ValidationDataType objType)
{
if (to == null || to.Length == 0 ) return true ;
if (objType == ValidationDataType.String)
{
return BaseCompareValidator.Compare(from.Length.ToString(), to.ToString(), cmp, ValidationDataType.Integer);
}
return BaseCompareValidator.Compare(from, to, cmp, objType);
}
// 在派生类中重写时,此方法包含确定输入控件中的值是否有效的代码。
protected override bool EvaluateIsValid()
{
return true ;
}
}
}
}
using System.Data;
using System.Drawing;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.ComponentModel;
using System.Reflection;
using System.Text.RegularExpressions;
[assembly: System.Web.UI.WebResource( " Fanfajin.MyWebControls.MyTextBox.ok.gif " , " image/gif " )]
[assembly: System.Web.UI.WebResource( " Fanfajin.MyWebControls.MyTextBox.error.gif " , " image/gif " )]
[assembly: System.Web.UI.WebResource( " Fanfajin.MyWebControls.MyTextBox.required.gif " , " image/gif " )]
namespace Fanfajin.MyWebControls
{
/// <summary>
/// 自定义控件MyTextBox
/// </summary>
[ToolboxBitmap( typeof (TextBox)),
DefaultProperty( " Text " ),
ToolboxData( " <{0}:MyTextBox runat='server'></{0}:MyTextBox> " )]
public class MyTextBox : TextBox, IValidator
{
#region 私有变量
private bool _valid = true ; // 验证结果
private string _errorMessage = " 验证失败 " ; // 错误提示信息
private string _ErrorImgUrl = "" ; // 错误图片
private string _okMessage = " 验证通过 " ; // 验证通过提示信息
private string _OkImgUrl = "" ; // 验证通过图片
private string _requiedMessage = " <font color=red><b>*</b></font> " ; // 必填提示信息
private string _RequiredImgUrl = "" ; // 必填项图片地址
private string _normalMessage = " 可选项 " ;
private string _minValue = string .Empty;
private string _maxValue = string .Empty;
private string _userFieldName = string .Empty; // 验证域名称
private ValidationDataType _CheckType = ValidationDataType.String; // 验证数据类型
private string _Regex = string .Empty; // 正则表达式
private RegexOptions _RegexOptions = RegexOptions.IgnoreCase; // 匹配模式
#endregion
#region ViewState 保存视图的属性
/// <summary>
/// 是否必填
/// </summary>
[Category( " 扩展选项 " ),
Description( " 是否必填项 " )]
public bool Required
{
get
{
if (ViewState[ " Required " ] == null )
{
return true ;
}
return ( bool )ViewState[ " Required " ];
}
set
{
ViewState[ " Required " ] = value;
}
}
/// <summary>
/// 是否验证过了(不管有没有通过,就是看看控件是不是还没开始验证)
/// </summary>
[Description( " 是否验证过了(不管有没有通过,就是看看控件是不是还没开始验证) " )]
[Browsable( false )]
public bool IsChecked
{
get
{
if (ViewState[ " IsChecked " ] == null )
{
return false ;
}
return ( bool )ViewState[ " IsChecked " ];
}
set
{
ViewState[ " IsChecked " ] = value;
}
}
#endregion
#region 公开的属性成员
/// <summary>
/// 验证类型
/// </summary>
[Category( " 扩展选项 " )]
[Description( " 数据验证类型String,Currency,Date,Double,Integer " )]
public ValidationDataType CheckType
{
get { return _CheckType; }
set { _CheckType = value; }
}
/// <summary>
/// 最小值(字符串时 比较长度最小值)
/// </summary>
[Category( " 扩展选项 " ),
Description( " 最小值(字符串时比较长度最大值) " )]
public string MinValue
{
get { return _minValue; }
set { _minValue = value; }
}
/// <summary>
/// 最大值(字符串时 比较长度最大值)
/// </summary>
[Category( " 扩展选项 " ),
Description( " 最大值(字符串时 比较长度最大值) " )]
public string MaxValue
{
get { return _maxValue; }
set { _maxValue = value; }
}
/// <summary>
/// 验证域名称
/// </summary>
[Category( " 扩展选项 " ),
Description( " 验证域名称 " )]
public string UserFieldName
{
get
{
if ( this ._userFieldName == String.Empty)
this ._userFieldName = this .ID.Replace( " txt " , "" );
return this ._userFieldName;
}
set { this ._userFieldName = value; }
}
/// <summary>
/// 验证域正则表达式
/// </summary>
[Category( " 扩展选项 " ),
Description( " 验证域正则表达式 " ),
DefaultValue( "" )]
public string RegexString
{
get { return _Regex; }
set { _Regex = value; }
}
/// <summary>
/// 正则表达式匹配模式
/// </summary>
[Category( " 扩展选项 " ),
Description( " 正则表达式验证模式 " )]
public RegexOptions ReOption
{
get { return _RegexOptions; }
set { _RegexOptions = value; }
}
/// <summary>
/// 验证成功提示信息
/// </summary>
[Category( " 扩展选项 " ),
Description( " 验证成功提示信息 " )]
public string OkMessage
{
get { return _okMessage; }
set { _okMessage = value; }
}
/// <summary>
/// 必填项提示信息
/// </summary>
[Category( " 扩展选项 " ),
Description( " 必填项提示信息 " )]
public string RequiredMessage
{
get { return _requiedMessage; }
set { _requiedMessage = value; }
}
/// <summary>
/// 正常提示信息
/// </summary>
[Category( " 扩展选项 " ),
Description( " 正常提示信息 " )]
public string NormailMessage
{
get { return _normalMessage; }
set { _normalMessage = value; }
}
#endregion
#region IValidator接口成员
/// <summary>
/// IValidator接口成员错误信息
/// </summary>
[Category( " 扩展选项 " ),
Description( " 错误信息 " )]
public string ErrorMessage
{
get { return _errorMessage; }
set { _errorMessage = value; }
}
/// <summary>
/// IValidator接口成员
/// </summary>
[Description( " IValidator接口成员 是否通过了验证 " )]
[Browsable( false )]
public bool IsValid
{
get { return _valid; }
set
{
_valid = value;
if ( ! _valid)
{
this .BackColor = Color.LightYellow;
}
else
{
this .BackColor = Color.White;
}
}
}
/// <summary>
/// IValidate接口成员
/// </summary>
public void Validate()
{
this .IsValid = true ;
this .IsChecked = true ;
// 如果是空的话,看看是否允许空值
bool isBlank = ( this .Text.Trim() == "" );
if (isBlank)
{
if (Required)
{
this .ErrorMessage = String.Format( " '{0}' 不能为空! " , this .UserFieldName);
this .IsValid = false ;
}
else
{
this .IsValid = true ;
return ;
}
}
// 验证类型是否正确
bool isOk = BaseCompareValidator.CanConvert( this .Text, this ._CheckType);
if ( ! isOk)
{
// this.ErrorMessage = String.Format("'{0}' 请输入正确的类型" + this._CheckType.ToString(), this.UserFieldName);
this .ErrorMessage += " [类型错误] " ;
this .IsValid = false ;
return ;
}
// 验证最小值 MinValue与 Text对比
isOk = TextBoxCompare.DoCompare( this .Text, MinValue, ValidationCompareOperator.GreaterThanEqual, _CheckType);
if ( ! isOk)
{
this .ErrorMessage += String.Format( " 值或长度小于{0} " , this .MinValue);
this .IsValid = false ;
return ;
}
// 验证最大值 MaxValue与 Text对比
isOk = TextBoxCompare.DoCompare( this .Text, MaxValue, ValidationCompareOperator.LessThanEqual, _CheckType);
if ( ! isOk)
{
// this.ErrorMessage = String.Format("'{0}' 的值不能超过 {1}", this.UserFieldName, this.MaxValue);
this .IsValid = false ;
return ;
}
// 验证正则表达式
if ( this .RegexString != "" )
{
isOk = Regex.IsMatch( this .Text, this .RegexString, ReOption);
if ( ! isOk)
{
// this.ErrorMessage = String.Format("'{0}' 的格式不对!", this.UserFieldName);
this .IsValid = false ;
return ;
}
}
}
/// <summary>
/// 文本框后的图片地址
/// </summary>
[
Category( " 扩展选项 " ),
Description( " 验证失败时,文本框后面的图片URL地址 " )
]
public string ErrorImgUrl
{
get
{
if (_ErrorImgUrl == null || _ErrorImgUrl == "" )
{
return this .Page.ClientScript.GetWebResourceUrl( this .GetType(), " Fanfajin.MyWebControls.MyTextBox.error.gif " );
}
else
{
return _ErrorImgUrl;
}
}
set { _ErrorImgUrl = value; }
}
/// <summary>
/// 文本框后的图片地址
/// </summary>
[
Category( " 扩展选项 " ),
Description( " 验证成功时,文本框后面的图片URL地址 " )
]
public string OkImgUrl
{
get
{
if (_OkImgUrl == null || _OkImgUrl == "" )
{
// 返回默认的验证成功时的图片地址
return this .Page.ClientScript.GetWebResourceUrl( this .GetType(), " Fanfajin.MyWebControls.MyTextBox.ok.gif " );
}
else
{
return _OkImgUrl;
}
}
set { _OkImgUrl = value; }
}
/// <summary>
/// 文本框后的图片地址
/// </summary>
[
Category( " 扩展选项 " ),
Description( " 验证成功时,文本框后面的图片URL地址 " )
]
public string RequiredImgUrl
{
get
{
if (_RequiredImgUrl == null || _RequiredImgUrl == "" )
{
// 返回默认的验证成功时的图片地址
return this .Page.ClientScript.GetWebResourceUrl( this .GetType(), " Fanfajin.MyWebControls.MyTextBox.required.gif " );
}
else
{
return _RequiredImgUrl;
}
}
set { _RequiredImgUrl = value; }
}
#endregion
/// <summary>
/// OnInit
/// </summary>
/// <param name="e"></param>
protected override void OnInit(EventArgs e)
{
base .OnInit(e);
Page.Validators.Add( this );
}
/// <summary>
/// OnUnload
/// </summary>
/// <param name="e"></param>
protected override void OnUnload(EventArgs e)
{
if (Page != null )
{
Page.Validators.Remove( this );
}
base .OnUnload(e);
}
/// <summary>
/// 加入错误提示信息
/// </summary>
/// <param name="writer"></param>
protected override void Render(HtmlTextWriter writer)
{
base .Render(writer);
if (IsChecked)
{
if ( this .IsValid)
{
if (Required)
{
writer.Write( " <img src=\ "" + this.OkImgUrl + " \ " > " + this .OkMessage);
}
else
{
writer.Write( " " + this .NormailMessage);
}
}
else
{
if (Required)
{
writer.Write( " <img src=\ "" + this.ErrorImgUrl + " \ " > " + this .RequiredMessage + " : " + this .ErrorMessage);
}
else
{
writer.Write( " <img src=\ "" + this.ErrorImgUrl + " \ " > " + " ( " + this .NormailMessage + " ) " + this .RequiredMessage + " : " + this .ErrorMessage);
}
}
}
else // 还没开始验证
{
if (Required)
{
writer.Write( " <img src=\ "" + this.RequiredImgUrl + " \ " > " + this .RequiredMessage);
}
else
{
writer.Write( " " + this .NormailMessage + " " + this .RequiredMessage);
}
}
}
/// <summary>
/// 验证类(从 BaseCompareValidator 派生 )
/// </summary>
class TextBoxCompare : BaseCompareValidator
{
/// <summary>
/// 执行验证
/// </summary>
/// <param name="from"></param>
/// <param name="to"></param>
/// <param name="cmp"></param>
/// <param name="objType"></param>
/// <returns></returns>
public static bool DoCompare( string from, string to, ValidationCompareOperator cmp, ValidationDataType objType)
{
if (to == null || to.Length == 0 ) return true ;
if (objType == ValidationDataType.String)
{
return BaseCompareValidator.Compare(from.Length.ToString(), to.ToString(), cmp, ValidationDataType.Integer);
}
return BaseCompareValidator.Compare(from, to, cmp, objType);
}
// 在派生类中重写时,此方法包含确定输入控件中的值是否有效的代码。
protected override bool EvaluateIsValid()
{
return true ;
}
}
}
}
源码下载 http://files.cnblogs.com/fanfajin/MyTextBox2.rar
下载的源码中去掉了一些没什么用的东西了,可以在页面中加入一个ValidationSummary 验证控件来显示ErrorMessage,