如何建立web服务并引用的细节,不是本文的介绍的目标,不再赘述。在silverlight调用服务器端服务的时候,默认情况下是进行异步调用的,代码如下:
private
void
button2_Click(
object
sender, RoutedEventArgs e)
{
Service1Client sc = new Service1Client();
sc.DoWorkCompleted += new EventHandler < DoWorkCompletedEventArgs > (sc_DoWorkCompleted);
sc.DoWorkAsync(textBox1.Text);
}
void sc_DoWorkCompleted( object sender, DoWorkCompletedEventArgs e)
{
textBox2.Text = e.Result;
}
{
Service1Client sc = new Service1Client();
sc.DoWorkCompleted += new EventHandler < DoWorkCompletedEventArgs > (sc_DoWorkCompleted);
sc.DoWorkAsync(textBox1.Text);
}
void sc_DoWorkCompleted( object sender, DoWorkCompletedEventArgs e)
{
textBox2.Text = e.Result;
}
若是你的调用非常复杂的话,比如当这个调用完成的时候开始下一个调用,然后又进行下一个调用,各个调用之间存在关联关系的话,一直XX_DoWorkCompleted会让你头大,并且不利于代码的管理。若碰到过这样的问题的朋友一定很希望如果能够同步调用就好了,这篇文章将帮到你。或者现在不需要,等你需要的时候记得用就行了,别像我当初那样难为的不行。
主要是需要引用一个类库的问题,这个类库是外国人写的,名称为DanielVaughan.dll,下载完之后,首先需要在项目中添加对它的引用,如下图,
然后在程序中添加对两个空间的引用,如下图:
将原来的添加botton1事件:
private
void
button1_Click(
object
sender, RoutedEventArgs e)
{
string dd = textBox1.Text;
string res = " NULL " ;
ThreadPool.QueueUserWorkItem( delegate
{
Service1 sv = ChannelManager.Instance.GetChannel < Service1 > ();
/* Perform synchronous WCF call. */
res = SynchronousChannelBroker.PerformAction < string , string > (sv.BeginDoWork, sv.EndDoWork, dd);
Dispatcher.BeginInvoke( delegate
{
textBox2.Text += " \r\n同步调用-- " + res + " \r\n " ;
});
});
}
{
string dd = textBox1.Text;
string res = " NULL " ;
ThreadPool.QueueUserWorkItem( delegate
{
Service1 sv = ChannelManager.Instance.GetChannel < Service1 > ();
/* Perform synchronous WCF call. */
res = SynchronousChannelBroker.PerformAction < string , string > (sv.BeginDoWork, sv.EndDoWork, dd);
Dispatcher.BeginInvoke( delegate
{
textBox2.Text += " \r\n同步调用-- " + res + " \r\n " ;
});
});
}
这样就可以实现对WebClient的同步调用了,当你需要关联调用WebClient3次以上的时候 可以考虑使用这个类库,如果只是简单的调用下的话,没有必要使用。
页面全部代码:
<
UserControl x:Class
=
"
SilverlightApplication2.MainPage
"
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
xmlns:d = " http://schemas.microsoft.com/expression/blend/2008 "
xmlns:mc = " http://schemas.openxmlformats.org/markup-compatibility/2006 "
mc:Ignorable = " d "
d:DesignHeight = " 300 " d:DesignWidth = " 400 " xmlns:dataInput = " clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Data.Input " Width = " 640 " Height = " 480 " >
< Grid x:Name = " LayoutRoot " >
< Grid.Background >
< LinearGradientBrush EndPoint = " 0.443,0.621 " StartPoint = " 0.443,-2.509 " >
< GradientStop Color = " #FF5C6768 " />
< GradientStop Color = " White " Offset = " 1 " />
</ LinearGradientBrush >
</ Grid.Background >
< Button Content = " 同步调用服务 " Height = " 40 " HorizontalAlignment = " Left " Margin = " 67,98,0,0 " Name = " button1 " VerticalAlignment = " Top " Width = " 120 " Click = " button1_Click " />
< dataInput:Label Height = " 50 " HorizontalAlignment = " Left " Margin = " 67,188,0,0 " Name = " label2 " VerticalAlignment = " Top " Width = " 46 " Content = " 状态: " FontSize = " 16 " />
< TextBox Height = " 40 " HorizontalAlignment = " Left " Margin = " 165,27,0,0 " Name = " textBox1 " VerticalAlignment = " Top " Width = " 300 " FontSize = " 16 " />
< TextBox Height = " 100 " HorizontalAlignment = " Left " Margin = " 146,188,0,0 " Name = " textBox2 " VerticalAlignment = " Top " Width = " 400 " FontSize = " 16 " TextWrapping = " Wrap " Text = " 尚未调用服务 " />
< Button Content = " 异步调用服务 " Height = " 40 " HorizontalAlignment = " Left " Margin = " 346,98,0,0 " Name = " button2 " VerticalAlignment = " Top " Width = " 120 " Click = " button2_Click " />
< dataInput:Label Height = " 40 " HorizontalAlignment = " Left " Margin = " 67,27,0,0 " Name = " label1 " VerticalAlignment = " Top " Width = " 92 " FontSize = " 16 " Content = " 输入文本: " />
</ Grid >
</ UserControl >
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
xmlns:d = " http://schemas.microsoft.com/expression/blend/2008 "
xmlns:mc = " http://schemas.openxmlformats.org/markup-compatibility/2006 "
mc:Ignorable = " d "
d:DesignHeight = " 300 " d:DesignWidth = " 400 " xmlns:dataInput = " clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Data.Input " Width = " 640 " Height = " 480 " >
< Grid x:Name = " LayoutRoot " >
< Grid.Background >
< LinearGradientBrush EndPoint = " 0.443,0.621 " StartPoint = " 0.443,-2.509 " >
< GradientStop Color = " #FF5C6768 " />
< GradientStop Color = " White " Offset = " 1 " />
</ LinearGradientBrush >
</ Grid.Background >
< Button Content = " 同步调用服务 " Height = " 40 " HorizontalAlignment = " Left " Margin = " 67,98,0,0 " Name = " button1 " VerticalAlignment = " Top " Width = " 120 " Click = " button1_Click " />
< dataInput:Label Height = " 50 " HorizontalAlignment = " Left " Margin = " 67,188,0,0 " Name = " label2 " VerticalAlignment = " Top " Width = " 46 " Content = " 状态: " FontSize = " 16 " />
< TextBox Height = " 40 " HorizontalAlignment = " Left " Margin = " 165,27,0,0 " Name = " textBox1 " VerticalAlignment = " Top " Width = " 300 " FontSize = " 16 " />
< TextBox Height = " 100 " HorizontalAlignment = " Left " Margin = " 146,188,0,0 " Name = " textBox2 " VerticalAlignment = " Top " Width = " 400 " FontSize = " 16 " TextWrapping = " Wrap " Text = " 尚未调用服务 " />
< Button Content = " 异步调用服务 " Height = " 40 " HorizontalAlignment = " Left " Margin = " 346,98,0,0 " Name = " button2 " VerticalAlignment = " Top " Width = " 120 " Click = " button2_Click " />
< dataInput:Label Height = " 40 " HorizontalAlignment = " Left " Margin = " 67,27,0,0 " Name = " label1 " VerticalAlignment = " Top " Width = " 92 " FontSize = " 16 " Content = " 输入文本: " />
</ Grid >
</ UserControl >
处理程序全部代码:
using
System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using SilverlightApplication2.ServiceReference1;
using System.Threading;
using DanielVaughan;
namespace SilverlightApplication2
{
public partial class MainPage : UserControl
{
public MainPage()
{
InitializeComponent();
UISynchronizationContext.Instance.Initialize(Dispatcher);
}
private void button1_Click( object sender, RoutedEventArgs e)
{
string dd = textBox1.Text;
string res = " NULL " ;
ThreadPool.QueueUserWorkItem( delegate
{
Service1 sv = ChannelManager.Instance.GetChannel < Service1 > ();
/* Perform synchronous WCF call. */
res = SynchronousChannelBroker.PerformAction < string , string > (sv.BeginDoWork, sv.EndDoWork, dd);
Dispatcher.BeginInvoke( delegate
{
textBox2.Text += " \r\n同步调用-- " + res + " \r\n " ;
});
});
}
private void button2_Click( object sender, RoutedEventArgs e)
{
Service1Client sc = new Service1Client();
sc.DoWorkCompleted += new EventHandler < DoWorkCompletedEventArgs > (sc_DoWorkCompleted);
sc.DoWorkAsync(textBox1.Text);
}
void sc_DoWorkCompleted( object sender, DoWorkCompletedEventArgs e)
{
textBox2.Text += " 异步调用-- " + e.Result + " \r\n " ;
}
}
}
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using SilverlightApplication2.ServiceReference1;
using System.Threading;
using DanielVaughan;
namespace SilverlightApplication2
{
public partial class MainPage : UserControl
{
public MainPage()
{
InitializeComponent();
UISynchronizationContext.Instance.Initialize(Dispatcher);
}
private void button1_Click( object sender, RoutedEventArgs e)
{
string dd = textBox1.Text;
string res = " NULL " ;
ThreadPool.QueueUserWorkItem( delegate
{
Service1 sv = ChannelManager.Instance.GetChannel < Service1 > ();
/* Perform synchronous WCF call. */
res = SynchronousChannelBroker.PerformAction < string , string > (sv.BeginDoWork, sv.EndDoWork, dd);
Dispatcher.BeginInvoke( delegate
{
textBox2.Text += " \r\n同步调用-- " + res + " \r\n " ;
});
});
}
private void button2_Click( object sender, RoutedEventArgs e)
{
Service1Client sc = new Service1Client();
sc.DoWorkCompleted += new EventHandler < DoWorkCompletedEventArgs > (sc_DoWorkCompleted);
sc.DoWorkAsync(textBox1.Text);
}
void sc_DoWorkCompleted( object sender, DoWorkCompletedEventArgs e)
{
textBox2.Text += " 异步调用-- " + e.Result + " \r\n " ;
}
}
}
Service代码:
using
System;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Activation;
namespace SilverlightApplication2.Web
{
[ServiceContract(Namespace = "" )]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class Service1
{
[OperationContract]
public string DoWork( string aa)
{
// 在此处添加操作实现
return " 调用服务完成,返回你输入的值: " + aa;
}
// 在此处添加更多操作并使用 [OperationContract] 标记它们
}
}
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Activation;
namespace SilverlightApplication2.Web
{
[ServiceContract(Namespace = "" )]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class Service1
{
[OperationContract]
public string DoWork( string aa)
{
// 在此处添加操作实现
return " 调用服务完成,返回你输入的值: " + aa;
}
// 在此处添加更多操作并使用 [OperationContract] 标记它们
}
}
程序运行截图:
1.
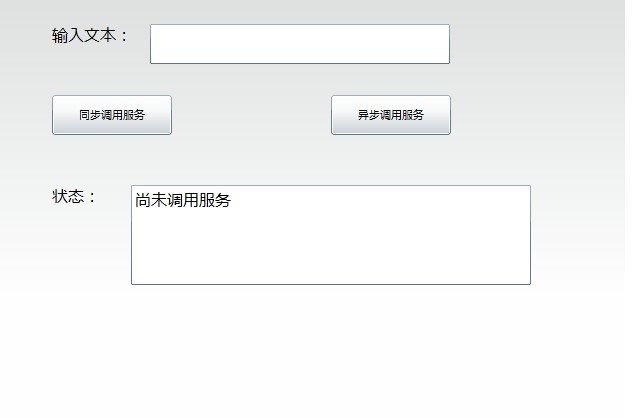
2.
3.
欢迎广大园友共同探讨,觉得好的话请推荐下。本人技术水平有限,如有不足之处,还请园友多多批评指正,谢谢。