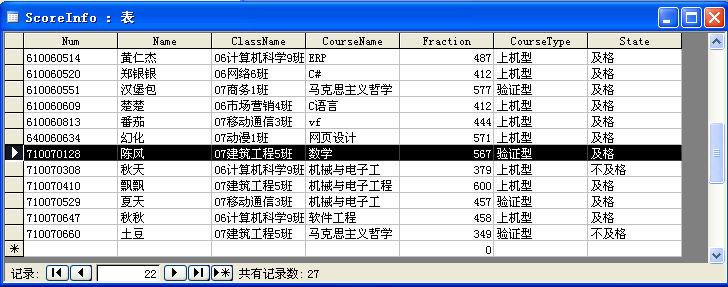
1 using System; 2 using System.Collections.Generic; 3 using System.ComponentModel; 4 using System.Data; 5 using System.Drawing; 6 using System.Linq; 7 using System.Text; 8 using System.Windows.Forms; 9 using System.Data.OleDb; 10 using System.Data.Common; 11 namespace WindowsFormsApplication1 12 { 13 public partial class Form1 : Form 14 { 15 public Form1() 16 { 17 InitializeComponent(); 18 } 19 private CurrencyManager currencyManager1; 20 private DataSet dataSet1 = new DataSet(); 21 22 private void Form1_Load(object sender, EventArgs e) 23 { 24 string connstring = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=MangerDataBase.mdb"; 25 string sqlstring = "SELECT * FROM ScoreInfo "; 26 OleDbConnection oleDbConnection1 = new OleDbConnection(connstring); 27 oleDbConnection1.Open(); 28 OleDbDataAdapter oleDbDataAdapter = new OleDbDataAdapter(sqlstring, connstring); 29 30 oleDbDataAdapter.Fill(dataSet1, "ScoreInfo"); 31 textBox1.DataBindings.Add("Text", dataSet1, "ScoreInfo.Num"); 32 textBox2.DataBindings.Add("Text", dataSet1, "ScoreInfo.Name"); 33 textBox3.DataBindings.Add("Text", dataSet1, "ScoreInfo.ClassName"); 34 textBox4.DataBindings.Add("Text", dataSet1, "ScoreInfo.CourseName"); 35 textBox5.DataBindings.Add("Text", dataSet1, "ScoreInfo.Fraction"); 36 textBox6.DataBindings.Add("Text", dataSet1, "ScoreInfo.CourseType"); 37 textBox7.DataBindings.Add("Text", dataSet1, "ScoreInfo.State"); 38 currencyManager1 = (CurrencyManager)this.BindingContext[dataSet1, "ScoreInfo"]; 39 currencyManager1.Position = 0; 40 } 41 //新建 42 private void button0_Click(object sender, EventArgs e) 43 { 44 textBox1.Text= ""; 45 //textBox2.Text= ""; 46 //textBox3.Text= ""; 47 //textBox4.Text= ""; 48 //textBox5.Text= ""; 49 //textBox6.Text= ""; 50 //textBox7.Text = ""; 51 } 52 //插入数据 53 private void button01_Click(object sender, EventArgs e) 54 { 55 if ( (textBox1.Text.Trim() == "") || 56 (textBox2.Text.Trim() == "") || 57 (textBox3.Text.Trim() == "") || 58 (textBox4.Text.Trim() == "") || 59 (textBox5.Text.Trim() == "") || 60 (textBox6.Text.Trim() == "") || 61 (textBox7.Text.Trim() == "") 62 ) 63 { 64 MessageBox.Show("请填完整"); 65 } 66 else 67 { 68 string sqlconnect = "Provider=Microsoft.Jet.OLEDB.4.0;data source=MangerDataBase.mdb"; 69 OleDbConnection oleDbConnection1 = new OleDbConnection(sqlconnect); 70 oleDbConnection1.Close(); 71 oleDbConnection1.Open(); 72 OleDbCommand oleDbCommand1 = new OleDbCommand("", oleDbConnection1); 73 string sql = "SELECT Num FROM ScoreInfo WHERE Num='" + textBox1.Text.Trim() + "'"; 74 oleDbCommand1.CommandText = sql; 75 if (oleDbCommand1.ExecuteScalar() == null) 76 { 77 //学号 78 //姓名 79 //班级名 80 //课程名称 81 //分数 82 //课程类型 83 //状态 84 sql = "INSERT INTO ScoreInfo VALUES('" + textBox1.Text.Trim() + "','" + 85 textBox2.Text.Trim() + "','" + 86 textBox3.Text.Trim() + "','" + 87 textBox4.Text.Trim() + "','" + 88 textBox5.Text.Trim() + "','" + 89 textBox6.Text.Trim() + "','" + "')"; 90 oleDbCommand1.CommandText = sql; 91 oleDbCommand1.ExecuteNonQuery(); 92 MessageBox.Show("用户添加成功!"); 93 } 94 else 95 { 96 MessageBox.Show("该用户已经存在,请重新输入!"); 97 oleDbConnection1.Close(); 98 } 99 } 100 } 101 //第一条 102 private void button1_Click(object sender, EventArgs e) 103 { 104 currencyManager1.Position = 0; 105 button1.Enabled = false; 106 button4.Enabled = true; 107 } 108 //上一条 109 private void button2_Click(object sender, EventArgs e) 110 { 111 if (this.currencyManager1.Position == 0) 112 { 113 MessageBox.Show("已经是第一条记录", "信息提示", MessageBoxButtons.OK, MessageBoxIcon.Information); 114 return; 115 } 116 else 117 this.currencyManager1.Position--; 118 } 119 //下一条 120 private void button3_Click(object sender, EventArgs e) 121 { 122 if (currencyManager1.Position == currencyManager1.Count - 1) 123 { 124 MessageBox.Show("已经是末一条记录", "信息提示", MessageBoxButtons.OK, MessageBoxIcon.Information); 125 return; 126 } 127 else 128 currencyManager1.Position++; 129 } 130 //最后一条 131 private void button4_Click(object sender, EventArgs e) 132 { 133 currencyManager1.Position = currencyManager1.Count - 1; 134 135 button4.Enabled = false; 136 button1.Enabled = true; 137 } 138 //更新 139 private void button5_Click(object sender, EventArgs e) 140 { 141 if ((textBox1.Text.Trim() == "")|| 142 (textBox2.Text.Trim() == "")|| 143 (textBox3.Text.Trim() == "")|| 144 (textBox4.Text.Trim() == "")|| 145 (textBox5.Text.Trim() == "")|| 146 (textBox6.Text.Trim() == "")|| 147 (textBox7.Text.Trim() == "") 148 ) 149 { 150 MessageBox.Show("请填完整"); 151 return; 152 } 153 string connstring = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=MangerDataBase.mdb"; 154 OleDbConnection oleDbConnection1 = new OleDbConnection(connstring); 155 oleDbConnection1.Open(); 156 string upstring = "UPDATE ScoreInfo SET Name='" + textBox2.Text.Trim() + 157 "',ClassName='" + textBox3.Text.Trim() + 158 "',CourseName='" + textBox4.Text.Trim() + 159 "',Fraction='" + textBox5.Text.Trim() + 160 "',CourseType='" + textBox7.Text.Trim() + 161 "',State='" + textBox6.Text.Trim() + 162 "' WHERE Num='" + textBox1.Text.Trim() + "'"; 163 OleDbCommand oleDbCommand1 = new OleDbCommand(upstring, oleDbConnection1); 164 oleDbCommand1.ExecuteNonQuery(); 165 MessageBox.Show("更新成功!"); 166 } 167 //删除数据 168 private void button6_Click(object sender, EventArgs e) 169 { 170 try 171 { 172 string connstring = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=MangerDataBase.mdb"; 173 OleDbConnection oleDbConnection1 = new OleDbConnection(connstring); 174 oleDbConnection1.Open(); 175 string sqldelete = "DELETE FROM ScoreInfo WHERE Num = '" + textBox1.Text.Trim() + "'"; 176 177 OleDbCommand oleDbCommand1 = new OleDbCommand(sqldelete, oleDbConnection1); 178 oleDbCommand1.ExecuteNonQuery(); 179 dataSet1.Tables["ScoreInfo"].Rows[currencyManager1.Position].Delete(); 180 dataSet1.Tables["ScoreInfo"].AcceptChanges(); 181 currencyManager1.Position = 0; 182 oleDbConnection1.Close(); 183 MessageBox.Show("删除成功!"); 184 } 185 catch (Exception Err) 186 { 187 MessageBox.Show("删除数据记录失败:" + Err.Message); 188 } 189 } 190 } 191 }