数据容器 tuple
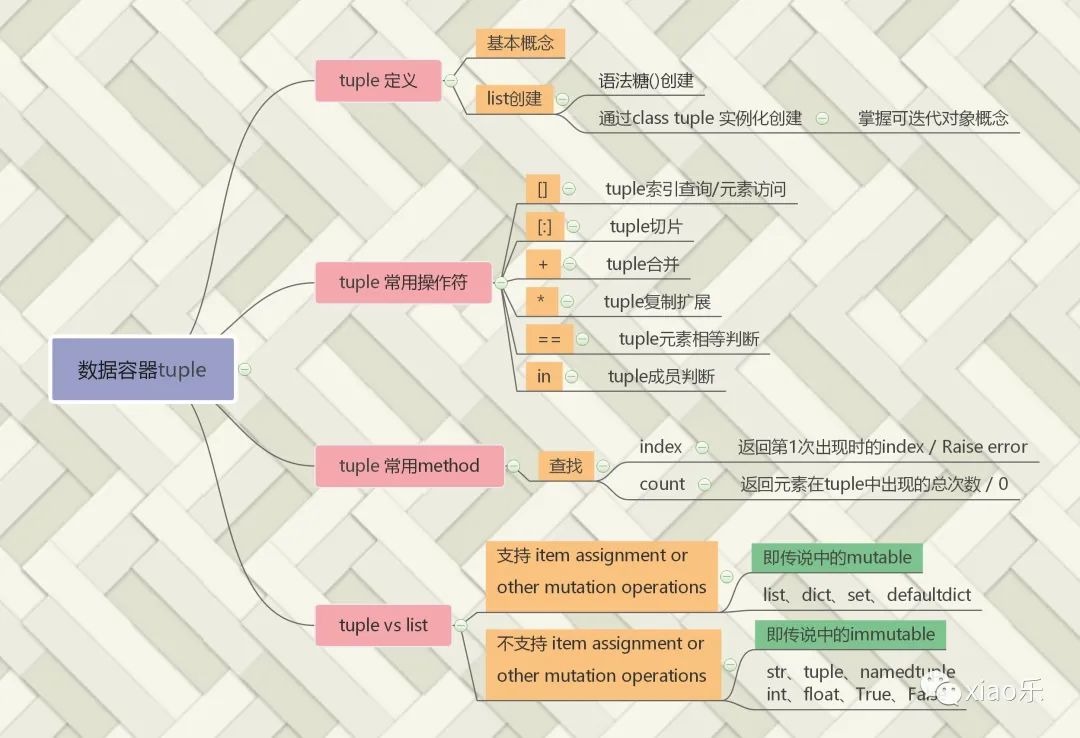
list 定义
list基本概念
tuple是Python中另外最基本的数据结构。序列中的每个元素都分配一个索引index - 它的位置,或索引,第一个索引是0,第二个索引是1,依此类推。
tuple与list有着相同的数据结构, 区别在于tuple是静态的数据类型,list是动态的数据类型。
tuple对象创建
(1)语法糖()创建
代码示例
print("------------ 利用 () 创建 tuple ")
print("字符串tuple:", ('a', 'b', 'c', 'd', 'e', 'f', 'g'))
print("数字tuple:",( (1, 2, 3), (11, 22, 33, 444), (-1, -2)))
print("空tuple:", ())
------------ 利用 () 创建 tuple
字符串tuple: ('a', 'b', 'c', 'd', 'e', 'f', 'g')
数字tuple: ((1, 2, 3), (11, 22, 33, 444), (-1, -2))
空tuple: ()
(2)通过class tuple实例化创建
class tuple(object):
"""
Built-in immutable sequence.
If no argument is given, the constructor returns an empty tuple.
If iterable is specified the tuple is initialized from iterable's items.
If the argument is a tuple, the return value is the same object.
"""
def __init__(self, seq=()): # known special case of tuple.__init__
"""
Built-in immutable sequence.
If no argument is given, the constructor returns an empty tuple.
If iterable is specified the tuple is initialized from iterable's items.
If the argument is a tuple, the return value is the same object.
# (copied from class doc)
"""
pass
- 不传入数据,则
seq为()
,默认构建空tuple - The argument must be an iterable if specified,其构造过程就是将可迭代对象遍历,然后将遍历所得元素依次塞入 list中,因此:
- 传入 字符串 -------> 每个字符组成的tuple
- 传入 list -------> list变tuple
- 传入 tuple -------> 原先的tuple
- 传入 dict ------->dcit 的 key 组成的tuple
print("------------ 利用 class tuple 实例化 创建 tuple ")
print("传入 空 生成tuple:", tuple())
print("传入 'abcdefg' 生成tuple:", tuple('abcdefg'))
print("传入 (11,22,33,123) 生成tuple:", tuple((11, 22, 33, 123)))
print("传入 {'name': 'xiaole', 'height' :170 } 生成列表:",
tuple({'name': 'xiaole', 'height': 170}))
------------ 利用 class tuple 实例化 创建 tuple
传入 空 生成tuple: ()
传入 'abcdefg' 生成tuple: ('a', 'b', 'c', 'd', 'e', 'f', 'g')
传入 (11,22,33,123) 生成tuple: (11, 22, 33, 123)
传入 {'name': 'xiaole', 'height' :170 } 生成列表: ('name', 'height')
tuple 常用操作符
元组的常用操作符 | 描述 |
---|---|
[] | 对元组进行索引访问 |
[:] | 对元组进行切片操作 |
+ | 对元组进行相加 |
* | 对元组进行重复输出 |
>,== | 等关系运算符,对元组进行关系运算 |
in | 判断元素是否在元组中 |
[] tuple索引查询/元素访问
基本语法
element = tuple_demo[index]
功能
tuple也是一种线性的序列结构,可以通过index来访问tuple中的元素,[]中给定索引值index即可访问tuple中的元素。
Python 中的索引分为正索引和负索引。 正索引从 0 开始进行编号, 表示数据集合中的第一个元素。 负索引从-1 开始编号, 表示tuple中倒数第一个元素。
正负索引值必须在有效的范围之内, 否则会抛出越界访问的错误信息。
越界访问的错误信息 ---> IndexError: list index out of range
示例、利用[]访问list数据容器的元素、修改元素值
print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
numbers = (1, 2, 3, 4)
# 1.读取列表中的第一个元素
first_number = numbers[0]
print('当前tuple为:', numbers, ", 其中第一个元素为:", first_number)
# 2.读取列表中的最后一个元素
last_number = numbers[-1]
print('当前tuple为:', numbers, ", 其中最后一个元素为:", last_number)
print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
当前tuple为: (1, 2, 3, 4) , 其中第一个元素为: 1
当前tuple为: (1, 2, 3, 4) , 其中最后一个元素为: 4
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
[:] tuple切片
new_list = tuple_demo[:]
功能:对数据容器tuple进行切片
注意事项:切片为浅拷贝(shallow copy),修改切片数据对原数据有影响
示例
print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
# 定义tuple类型变量
numbers = (1, 2, 3, 4)
# 切片操作
print("切片 numbers[0:2]为:", numbers[0:2]) # 第 0~1 号元素
print("切片 numbers[2:]为:", ) # 第 2~end 号元素
print("切片 numbers[-3:-1]为:", ) # 2~end-1 第 号元素
print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
切片 numbers[0:2]为: (1, 2)
切片 numbers[2:]为:
切片 numbers[-3:-1]为:
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ tuple合并
基本语法
new_tuple = tuple0 + tuple1
功能:tuple的加法是把两个tuple中的元素合并,并返回一个新tuple。
代码示例、
print("-----------------------")
# 定义变量 negative_numbers 表示负数
negative_numbers = (-1, -2, -3, -4)
# 定义变量 positive_numbers 表示正数
positive_numbers = (1, 2, 3, 4)
# tuple合并
print("合并后的tuple", negative_numbers + positive_numbers)
print("原负数tuple", negative_numbers)
print("原整数tuple", positive_numbers)
print("-----------------------")
-----------------------
合并后的tuple (-1, -2, -3, -4, 1, 2, 3, 4)
原负数tuple (-1, -2, -3, -4)
原整数tuple (1, 2, 3, 4)
-----------------------
* tuple赋值扩展
基本语法:
new_tuple = tuple1 * number
功能:对tuple进行复制扩展, 返回一个新的tuple。
代码示例:tuple三倍扩展
print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
# tuple 定义与三倍扩展
numbers = (-1,-2,-3)
print("扩展后的tuple",numbers * 3)
print("原tuple",numbers )
print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
扩展后的tuple (-1, -2, -3, -1, -2, -3, -1, -2, -3)
原tuple (-1, -2, -3)
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
== tuple元素相等判断
基本语法
boolean_var = tuple0 == tuple1
功能、按序比较,从tuple的第1个元素开始, 逐元素进行比较tuple元素值是否相等
代码示例
print("-----------------------")
# 定义元素
tuple1 = (1, 2, 3, 4)
tuple2 = (4, 3, 2, 1)
tuple3 = (1, 2, 3, 4)
print("tuple{} 与 tuple{} 相等? {}".format(tuple1, tuple2, tuple1 == tuple2))
print("tuple{} 与 tuple{} 相等? {}".format(tuple1, tuple3, tuple1 == tuple3))
print("tuple{} 与 tuple{} 相等? {}".format(tuple2, tuple3, tuple2 == tuple3))
print("-----------------------")
-----------------------
tuple(1, 2, 3, 4) 与 tuple(4, 3, 2, 1) 相等? False
tuple(1, 2, 3, 4) 与 tuple(1, 2, 3, 4) 相等? True
tuple(4, 3, 2, 1) 与 tuple(1, 2, 3, 4) 相等? False
-----------------------
in tuple成员判断
基本语法
boolean_var = element in tuple
功能:使用 "in" 操作符来判断元素是否存在于tuple中, 若存在返回值为布尔类型 True, 否则返回 False。
代码示例
natural_numbers = (1, 2, 3, 4, 5, 6, 7, 8, 9)
test_numbers = (1, 3, 9,12)
for ix, number in enumerate(test_numbers):
if number in natural_numbers: # 判断是否在集合中
print("the {}-th element {} is in numbers {}".format(ix , number, numbers))
else:
print("the {2}-th element {0} is not in numbers {1}".format(number, numbers ,ix))
the 0-th element 1 is in numbers (-1, -2, -3)
the 1-th element 3 is in numbers (-1, -2, -3)
the 2-th element 9 is in numbers (-1, -2, -3)
the 3-th element 12 is not in numbers (-1, -2, -3)
tuple 常用method
tuple是一种静态的数据类型, 不可以对tuple执行修改等操作, tuple中提供的方法主要是对元素进行查找。
notes:与之对应的,list是一种动态的数据类型,可以通过[]对list中的元素进行修改
tuple元素 修改
- 通过 []操作符 对列表的元素值进行访问
tuple元素 查找统计
(1)index方法
class tuple(object):
def index(self, *args, **kwargs): # real signature unknown
"""
Return first index of value.
Raises ValueError if the value is not present.
"""
pass
基本语法
tuple_test = (1,'a', true ) # 定义一个tuple
tuple_test.index( 'a') # 获取 tuple_test 中'a'的index
功能:在tuple中查找元素是否存在, 如果存在会返回该元素的索引, 如果不存在会抛出ValueError 。
代码示例
students = ('Lily', 'Lucy', 'xiao乐', 'Lily')
print('当前tuple{}中元素{}第1次出现的位置为{}'.format(students, 'Lily', students.index('Lily')))
print('当前tuple{}中元素{}在index{}以后 第1次出现的位置为{}'.format(students,
'Lily', 1, students.index('Lily', 1)))
当前tuple('Lily', 'Lucy', 'xiao乐', 'Lily')中元素Lily第1次出现的位置为0
当前tuple('Lily', 'Lucy', 'xiao乐', 'Lily')中元素Lily在index1以后 第1次出现的位置为3
(2)count方法
class tuple(object):
def count(self, *args, **kwargs): # real signature unknown
""" Return number of occurrences of value. """
pass
基本语法
tuple_test = [1,'a', true , 'a'] # 定义一个tuple
tuple_test.count( 'a') # 获取tuple_test 中'a'出现的次数
功能:查找元素值 value 在tuple中出现的次数, 元素值 value 不存在时, 返回 0
代码示例
students = ('Lily', 'Lucy', 'xiao乐', 'Lily')
element = 'Lily'
print("元素{} 在 tuple {} 中出现的次数为 {}".format(element, students, students.count(element)))
element1 = 'xiaoLe'
print("元素{} 在 tuple {} 中出现的次数为 {}".format(element1, students, students.count(element1)))
元素Lily 在 tuple ('Lily', 'Lucy', 'xiao乐', 'Lily') 中出现的次数为 2
元素xiaoLe 在 tuple ('Lily', 'Lucy', 'xiao乐', 'Lily') 中出现的次数为 0
tuple vs list
不支持 item assignment or other mutation operations
即传说中的 immutable
不支持 item assignment or other mutation operation是指不可以对该数据类型进行修改,即只读的数据类型,例如str、tuple
使用[]操作符对str、tuple进行赋值修改,Python会Raise Error
string = "xiaole很帅"
string[-1]="穷"
TypeError Traceback (most recent call last)
in
1 string = "xiaole很帅"
----> 2 string[-1]="穷"
TypeError: 'str' object does not support item assignment
tuple_test = tuple( "你和我")
print(tuple_test )
tuple_test[-1]="她"
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
in
1 tuple_test = tuple( "你和我")
2 print(tuple_test )
----> 3 tuple_test[-1]="她"
TypeError: 'tuple' object does not support item assignment
notes:xiaole很穷?你和她? 统统都不存在的
支持 item assignment or other mutation operations
即传说中的 mutable
支持item assignment or other mutation operation 的数据类型中的元素是可以修改的,常用的动态数据类型包括list、dict、set、defaultdict等
通过**[]与=、或者set的add方法**等对数据容器中的元素进行修改,修改前后的列表与修改前的动态数据类型具有相同的id值
dict_test=dict( name = "xiaole", height=180 )
print("修改前:", dict_test, "===> id值" ,id(dict_test) )
dict_test["height"]=177
print("修改前:", dict_test, "===> id值" ,id(dict_test) )
修改前: {'name': 'xiaole', 'height': 180} ===> id值 2330246949608
修改前: {'name': 'xiaole', 'height': 177} ===> id值 2330246949608
set_test = set("你我")
print( set_test )
print(set_test.add("她"))
set_test.add("她")
print(set_test )
{'我', '你'}
None
{'我', '你', '她'}
References
[1] 菜鸟教程 Python 元组(Tuple) https://www.runoob.com/python/python-tuples.html
[2] 薯条老师Python系列 http://www.chipscoco.com/
Motto
日拱一卒,功不唐捐
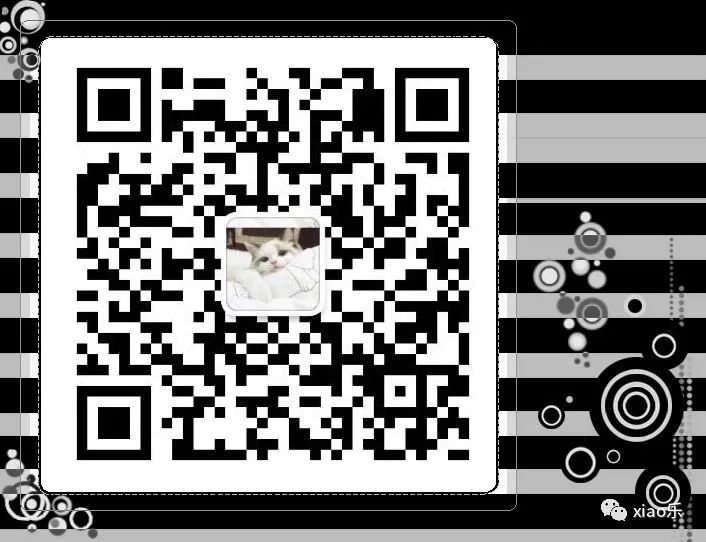