设计:用Swing中Graphics绘制图片,图片使用随机背景色,随机字符串;绘制字符串随机位置,随机大小,随机颜色;背景中加入若干随机位置和颜色的混乱线、混乱点。
效果:
源代码:
package com;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.p_w_picpath.BufferedImage;
import java.util.Random;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class AuthCode extends JDialog implements ActionListener
{
private JPanel p_w_picpathPanel, buttonPanel;r /> private JTextField field;
private JButton okButton, changeButton;
private Random random;
private BufferedImage p_w_picpath;
private String[] s;
public AuthCode()
{
init();
setTitle('验证码');
Toolkit kit = Toolkit.getDefaultToolkit();
Dimension s = kit.getScreenSize();
int screenWidth = s.width;
int screenHeight = s.height;
setBounds(screenWidth / 3, screenHeight / 3, 300, 200);
setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
}
public void init()
{
p_w_picpathPanel = new JPanel();
field = new JTextField();
buttonPanel = new JPanel();
okButton = new JButton('确定');
changeButton = new JButton('更换');
setImage();
p_w_picpathPanel.add(new JLabel(new ImageIcon(p_w_picpath)));
buttonPanel.add(changeButton);
buttonPanel.add(okButton);
add(p_w_picpathPanel, BorderLayout.NORTH);
add(field, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
okButton.addActionListener(this);
changeButton.addActionListener(this);
}
public static void main(String[] args)
{
new AuthCode().setVisible(true);
}
public void setImage()
{
// 创建内存图像
int width = 200, height = 90;
p_w_picpath = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = p_w_picpath.getGraphics();
// 取得随机数对象
random = new Random();
// 填充矩形区域
g.setColor(new Color(random.nextInt(255), random.nextInt(255), random
.nextInt(255)));
g.fillRect(0, 0, width, height);
// 画矩形边框
g.setColor(new Color(255, 255, 255));
g.drawRect(0, 0, width - 1, height - 1);
// 画1000个混乱点
for (int i = 0; i < 1000; i++)
{
int x = random.nextInt(width);
int y = random.nextInt(height);
int xl = x;
int yl = y;
g.drawLine(x, y, xl, yl);
g.setColor(new Color(20 + random.nextInt(200), 20 + random
.nextInt(200), 20 + random.nextInt(200)));
}
// 画100条混乱线
for (int i = 0; i < 100; i++)
{
int x = random.nextInt(width);
int y = random.nextInt(height);
int xl = random.nextInt(100);
int yl = random.nextInt(100);
g.drawLine(x, y, x + xl, y + yl);
g.setColor(new Color(20 + random.nextInt(200), 20 + random
.nextInt(200), 20 + random.nextInt(200)));
}
// 随机获取四个字母或数字型字符
s = new String[4];
s[0] = setRndomString()[random.nextInt(62)];
s[1] = setRndomString()[random.nextInt(62)];
s[2] = setRndomString()[random.nextInt(62)];
s[3] = setRndomString()[random.nextInt(62)];
// 随机生成四个字符大小和位置,并将字符画到图片上
g.setFont(new Font('Times New Roman', Font.PLAIN, 30 + random
.nextInt(20)));
g.setColor(new Color(20 + random.nextInt(110),
20 + random.nextInt(110), 20 + random.nextInt(110)));
g.drawString(s[0], 20 + random.nextInt(15), 40 + random.nextInt(30));
g.setFont(new Font('Times New Roman', Font.PLAIN, 30 + random
.nextInt(20)));
g.setColor(new Color(20 + random.nextInt(110),
20 + random.nextInt(110), 20 + random.nextInt(110)));
g.drawString(s[1], 50 + random.nextInt(15), 40 + random.nextInt(30));
g.setFont(new Font('Times New Roman', Font.PLAIN, 30 + random
.nextInt(20)));
g.setColor(new Color(20 + random.nextInt(110),
20 + random.nextInt(110), 20 + random.nextInt(110)));
g.drawString(s[2], 90 + random.nextInt(15), 40 + random.nextInt(30));
g.setFont(new Font('Times New Roman', Font.PLAIN, 30 + random
.nextInt(20)));
g.setColor(new Color(20 + random.nextInt(110),
20 + random.nextInt(110), 20 + random.nextInt(110)));
g.drawString(s[3], 130 + random.nextInt(15), 40 + random.nextInt(30));
g.dispose();
}
public String[] setRndomString()
{
String[] s = new String[62];
for (int i = 0; i < 10; i++)
{
s[i] = Integer.toString(i);
}
for (int i = 10; i < 36; i++)
{
s[i] = Character.toString((char) ('a' + i - 10));
}
for (int i = 36; i < 62; i++)
{
s[i] = Character.toString((char) ('A' + i - 36));
}
return s;
}
public void actionPerformed(ActionEvent e)
{
if (e.getSource() == okButton)
{
if (field.getText().toLowerCase().equals(
s[0].toLowerCase() + s[1].toLowerCase()
+ s[2].toLowerCase() + s[3].toLowerCase()))
{
JOptionPane.showMessageDialog(this, '输入正确,登陆成功!');
} else
{
JOptionPane.showMessageDialog(this, '输入错误,登陆失败!');
}
} else
{
setImage();
p_w_picpathPanel.removeAll();
p_w_picpathPanel.add(new JLabel(new ImageIcon(p_w_picpath)));
p_w_picpathPanel.updateUI();
}
}
}
效果:
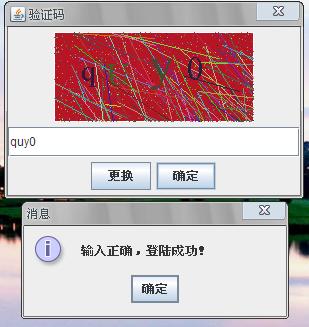
源代码:
package com;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.p_w_picpath.BufferedImage;
import java.util.Random;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class AuthCode extends JDialog implements ActionListener
{
private JPanel p_w_picpathPanel, buttonPanel;r /> private JTextField field;
private JButton okButton, changeButton;
private Random random;
private BufferedImage p_w_picpath;
private String[] s;
public AuthCode()
{
init();
setTitle('验证码');
Toolkit kit = Toolkit.getDefaultToolkit();
Dimension s = kit.getScreenSize();
int screenWidth = s.width;
int screenHeight = s.height;
setBounds(screenWidth / 3, screenHeight / 3, 300, 200);
setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
}
public void init()
{
p_w_picpathPanel = new JPanel();
field = new JTextField();
buttonPanel = new JPanel();
okButton = new JButton('确定');
changeButton = new JButton('更换');
setImage();
p_w_picpathPanel.add(new JLabel(new ImageIcon(p_w_picpath)));
buttonPanel.add(changeButton);
buttonPanel.add(okButton);
add(p_w_picpathPanel, BorderLayout.NORTH);
add(field, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
okButton.addActionListener(this);
changeButton.addActionListener(this);
}
public static void main(String[] args)
{
new AuthCode().setVisible(true);
}
public void setImage()
{
// 创建内存图像
int width = 200, height = 90;
p_w_picpath = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = p_w_picpath.getGraphics();
// 取得随机数对象
random = new Random();
// 填充矩形区域
g.setColor(new Color(random.nextInt(255), random.nextInt(255), random
.nextInt(255)));
g.fillRect(0, 0, width, height);
// 画矩形边框
g.setColor(new Color(255, 255, 255));
g.drawRect(0, 0, width - 1, height - 1);
// 画1000个混乱点
for (int i = 0; i < 1000; i++)
{
int x = random.nextInt(width);
int y = random.nextInt(height);
int xl = x;
int yl = y;
g.drawLine(x, y, xl, yl);
g.setColor(new Color(20 + random.nextInt(200), 20 + random
.nextInt(200), 20 + random.nextInt(200)));
}
// 画100条混乱线
for (int i = 0; i < 100; i++)
{
int x = random.nextInt(width);
int y = random.nextInt(height);
int xl = random.nextInt(100);
int yl = random.nextInt(100);
g.drawLine(x, y, x + xl, y + yl);
g.setColor(new Color(20 + random.nextInt(200), 20 + random
.nextInt(200), 20 + random.nextInt(200)));
}
// 随机获取四个字母或数字型字符
s = new String[4];
s[0] = setRndomString()[random.nextInt(62)];
s[1] = setRndomString()[random.nextInt(62)];
s[2] = setRndomString()[random.nextInt(62)];
s[3] = setRndomString()[random.nextInt(62)];
// 随机生成四个字符大小和位置,并将字符画到图片上
g.setFont(new Font('Times New Roman', Font.PLAIN, 30 + random
.nextInt(20)));
g.setColor(new Color(20 + random.nextInt(110),
20 + random.nextInt(110), 20 + random.nextInt(110)));
g.drawString(s[0], 20 + random.nextInt(15), 40 + random.nextInt(30));
g.setFont(new Font('Times New Roman', Font.PLAIN, 30 + random
.nextInt(20)));
g.setColor(new Color(20 + random.nextInt(110),
20 + random.nextInt(110), 20 + random.nextInt(110)));
g.drawString(s[1], 50 + random.nextInt(15), 40 + random.nextInt(30));
g.setFont(new Font('Times New Roman', Font.PLAIN, 30 + random
.nextInt(20)));
g.setColor(new Color(20 + random.nextInt(110),
20 + random.nextInt(110), 20 + random.nextInt(110)));
g.drawString(s[2], 90 + random.nextInt(15), 40 + random.nextInt(30));
g.setFont(new Font('Times New Roman', Font.PLAIN, 30 + random
.nextInt(20)));
g.setColor(new Color(20 + random.nextInt(110),
20 + random.nextInt(110), 20 + random.nextInt(110)));
g.drawString(s[3], 130 + random.nextInt(15), 40 + random.nextInt(30));
g.dispose();
}
public String[] setRndomString()
{
String[] s = new String[62];
for (int i = 0; i < 10; i++)
{
s[i] = Integer.toString(i);
}
for (int i = 10; i < 36; i++)
{
s[i] = Character.toString((char) ('a' + i - 10));
}
for (int i = 36; i < 62; i++)
{
s[i] = Character.toString((char) ('A' + i - 36));
}
return s;
}
public void actionPerformed(ActionEvent e)
{
if (e.getSource() == okButton)
{
if (field.getText().toLowerCase().equals(
s[0].toLowerCase() + s[1].toLowerCase()
+ s[2].toLowerCase() + s[3].toLowerCase()))
{
JOptionPane.showMessageDialog(this, '输入正确,登陆成功!');
} else
{
JOptionPane.showMessageDialog(this, '输入错误,登陆失败!');
}
} else
{
setImage();
p_w_picpathPanel.removeAll();
p_w_picpathPanel.add(new JLabel(new ImageIcon(p_w_picpath)));
p_w_picpathPanel.updateUI();
}
}
}
转载于:https://blog.51cto.com/7090376/1358259