一、概念
模板引擎是为了使用户界面与业务数据(内容)分离而产生的,它可以生成特定格式的文档,用于网站的模板引擎就会生成一个标准的HTML文档。
Thymeleaf是一款用于渲染XML/XHTML/HTML 5内容的模板引擎。
类似JSP、Velocity、FreeMaker等,可以简单的与Spring MVC等web框架进行集成作为web应用的模板引擎。与其他模板引擎相比,Thymeleaf最大的特点是能够直接在浏览器中打开并正确显示模板页面,而不需要启动整个web 应用,Spring Boot推荐使用Thymeleaf替代JSP,Spring Boot 2.0中默认使用Thymeleaf 3.0版本作为模板引擎。
A、Thymeleaf特点
- Thymeleaf在有网络和无网络的环境下皆可运行,即它可以让美工在浏览器查看页面的静态效果,也可以让程序员在服务器查看带数据的动态页面效果。因为由于它支持html原型,然后在html标签里增加额外的属性来达到模板+数据的展示方式。浏览器解释html时会忽略未定义的标签属性,所以Thymeleaf的模板可以静态地运行;当有数据返回到页面时,Thymeleaf标签会动态地替换掉静态内容,使页面动态显示。
- Thymeleaf开箱即用,它提供标准和Spring标准两种方言,可以直接套用模板实现JSTL、OGNL表达式效果,避免套模板、改jstl、改标签的困扰。
- Thymeleaf提供Spring标准方言和一个与SpringMVC完美集成的可选模块,可以快速的实现表单绑定、属性编辑器、国际化等功能。
B、对比
Thymeleaf 的模板语法并不会破坏文档的结构,模板依旧是有效的XML文档。模板还可以用作工作原型,Thymeleaf会在运行期替换掉静态值。Velocity与FreeMarker则是连续的文本处理器。
下面的代码示例分别使用Velocity、FreeMarker与Thymeleaf打印出一条消息:
Velocity: <p>$message</p>
FreeMarker: <p>${message}</p>
Thymeleaf: <p th:text="${message}">Hello World!</p>
但是因为Thymeleaf使用了XML DOM解析器,因此它并不适合于处理大规模的XML文件。
二、配置
A、引入Maven配置
<properties>
<!-- thymeleaf覆盖parent版本 -->
<thymeleaf.version>3.0.3.RELEASE</thymeleaf.version>
<thymeleaf-layout-dialect.version>2.1.1</thymeleaf-layout-dialect.version>
<thymeleaf-extras-springsecurity4.version>3.0.2.RELEASE</thymeleaf-extras-springsecurity4.version>
</properties>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf</artifactId>
<version>${thymeleaf.version}</version>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring4</artifactId>
<version>3.0.2.RELEASE</version>
</dependency>
B、添加SpringBoot配置
# 默认为HTML5模式
spring.thymeleaf.mode=HTML
# 不使用会报网页模板寻找不到异常
spring.thymeleaf.prefix=classpath:/templates/
# 默认使用html后缀
spring.thymeleaf.suffix=.html
# 测试环境,关闭缓存
spring.thymeleaf.cache=false
C、进行相关Bean配置
@Configuration
public class WebMvcConfig implements WebMvcConfigurer, ApplicationContextAware
{
@Value("${spring.thymeleaf.cache}")
private boolean thymeleafCacheEnable = true;
private ApplicationContext applicationContext;
/**
* 获取Spring上下文applicationContext
*/
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException
{
this.applicationContext = applicationContext;
}
/**
* 模板资源解析器
*/
@Bean
@ConfigurationProperties(prefix = "spring.thymeleaf")
public SpringResourceTemplateResolver templateResolver()
{
SpringResourceTemplateResolver templateResolver = new SpringResourceTemplateResolver();
templateResolver.setApplicationContext(this.applicationContext);
templateResolver.setCharacterEncoding("UTF-8");
templateResolver.setCacheable(thymeleafCacheEnable);
return templateResolver;
}
/**
* Thymeleaf标准方言解释器
*/
@Bean
public SpringTemplateEngine templateEngine()
{
SpringTemplateEngine templateEngine = new SpringTemplateEngine();
templateEngine.setTemplateResolver(templateResolver());
// 支持Spring EL表达式
templateEngine.setEnableSpringELCompiler(true);
// 支持SpringSecurity方言
//SpringSecurityDialect securityDialect = new SpringSecurityDialect();
//templateEngine.addDialect(securityDialect);
return templateEngine;
}
/**
* 视图解析器
*/
@Bean
public ThymeleafViewResolver viewResolver()
{
ThymeleafViewResolver viewResolver = new ThymeleafViewResolver();
viewResolver.setTemplateEngine(templateEngine());
return viewResolver;
}
/**
* 静态资源加载配置
*/
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry)
{
registry.addResourceHandler("/static/**").addResourceLocations("classpath:/static/");
}
}
三、编写Controller和前端页面
A、Controller
@Slf4j
@Controller
public class HomeController
{
@LogBack
@GetMapping("/")
public String index(Model model)
{
model.addAttribute("name", "isisiwish");
log.info("index");
return "index";
}
}
B、HTML模板
页面模板默认放在srcmainresourcestemplatesindex.html目录下,使用@Controller注解,返回模板名称即可。所有使用Thymeleaf的页面必须在HTML标签声明Thymeleaf。
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Search House</title>
<link href="/static/css/bootstrap.css" rel="stylesheet" type="text/css"/>
<link href="/static/css/common.css" rel='stylesheet' type='text/css'/>
</head>
<body>
<h2>Search House Index</h2>
<h4 th:text="${name}"></h4>
</body>
</html>
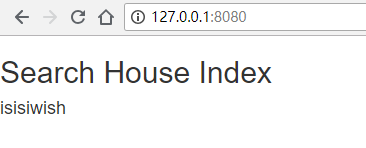
[20:24:48.365] [t.c.searchhouse.util.aop.LogAspect-http-nio-8080-exec-1:31] [INFO] - before
[20:24:48.375] [t.c.searchhouse.web.HomeController-http-nio-8080-exec-1:25] [INFO] - index
[20:24:48.376] [t.c.searchhouse.util.aop.LogAspect-http-nio-8080-exec-1:37] [INFO] - after
成功接入Thymeleaf模板引擎。
四、中文乱码问题
A、设置HTML编码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>403 Forbidden</title>
</head>
<body>
<h1>Access Denied</h1>
<h2>无权访问该页面</h2>
<a href="/">返回首页</a>
</body>
</html>
B、修改Config,设置字符编码为UTF-8
/**
* 模板资源解析器
*/
@Bean
@ConfigurationProperties(prefix = "spring.thymeleaf")
public SpringResourceTemplateResolver templateResolver()
{
SpringResourceTemplateResolver templateResolver = new SpringResourceTemplateResolver();
templateResolver.setApplicationContext(this.applicationContext);
templateResolver.setCharacterEncoding("UTF-8");
templateResolver.setCacheable(thymeleafCacheEnable);
return templateResolver;
}
/**
* 视图解析器
*/
@Bean
public ThymeleafViewResolver viewResolver()
{
ThymeleafViewResolver viewResolver = new ThymeleafViewResolver();
viewResolver.setTemplateEngine(templateEngine());
viewResolver.setCharacterEncoding("UTF-8");
return viewResolver;
}
Thymeleaf是一个非常灵活和优秀的前端页面模板引擎,使用Thymeleaf可以非常灵活的对页面进行布局,复用通用页面。Thymeleaf提供了各种常用的语法,非常方便在前端页面中使用。