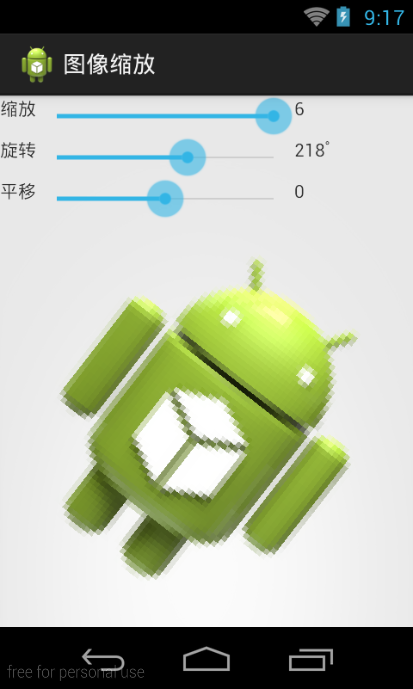
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="缩放" />
<SeekBar
android:id="@+id/seekBar_scale"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:max="5"/>
<TextView
android:id="@+id/scale_data"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="1" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="旋转" />
<SeekBar
android:id="@+id/seekBar_rotate"
android:max="360"
android:layout_width="200dp"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/rotate_data"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0°" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="平移" />
<SeekBar
android:id="@+id/seekBar_tran"
android:max="100"
android:progress="50"
android:layout_width="200dp"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/tran_data"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center" >
<ImageView
android:id="@+id/iv_2"
android:src="@drawable/ic_launcher"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
</LinearLayout>
package com.pas.image.tosmall;
import android.os.Bundle;
import android.app.Activity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Matrix;
import android.graphics.Paint;
import android.view.Menu;
import android.widget.ImageView;
import android.widget.SeekBar;
import android.widget.TextView;
import android.widget.SeekBar.OnSeekBarChangeListener;
public class MainActivity extends Activity implements OnSeekBarChangeListener
{
private ImageView iv2;
private Bitmap basebitmap;
private Bitmap scalebitmap;
//缩放
private SeekBar bar_scale;
private TextView tv_scale;
//旋转
private SeekBar bar_rotate;
private TextView tv_rotate;
//平移
private SeekBar bar_tran;
private TextView tv_tran;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
iv2 = (ImageView) findViewById(R.id.iv_2);
bar_scale=(SeekBar) findViewById(R.id.seekBar_scale);
bar_rotate=(SeekBar) findViewById(R.id.seekBar_rotate);
bar_tran=(SeekBar) findViewById(R.id.seekBar_tran);
bar_scale.setOnSeekBarChangeListener(this);
bar_rotate.setOnSeekBarChangeListener(this);
bar_tran.setOnSeekBarChangeListener(this);
tv_scale=(TextView) findViewById(R.id.scale_data);
tv_rotate=(TextView) findViewById(R.id.rotate_data);
tv_tran=(TextView) findViewById(R.id.tran_data);
basebitmap = BitmapFactory.decodeResource(getResources(), R.drawable.ic_launcher);
}
/**
* 缩放
*/
private void scale()
{
int scale=bar_scale.getProgress()+1;
int degrees=bar_rotate.getProgress();
int trans=bar_tran.getProgress();
int width=basebitmap.getWidth()*scale+Math.abs(trans-50);
int height=basebitmap.getHeight()*scale+Math.abs(trans-50);
scalebitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(scalebitmap);
Paint paint = new Paint();
paint.setStrokeWidth(5);
paint.setColor(Color.BLACK);
//消除锯齿
paint.setAntiAlias(true);
Matrix matrix = new Matrix();
//缩放
matrix.postScale(scale, scale);
//旋转 度数(0-360) 基准点
matrix.postRotate(degrees, width/2f, height/2f);
//平移
matrix.postTranslate(trans-50,trans-50);
//镜像
matrix.postScale(-1, 1);
matrix.postTranslate(scalebitmap.getWidth(), 0);
//倒影
matrix.postScale(1, -1);
matrix.postTranslate(0, scalebitmap.getHeight());
canvas.drawBitmap(basebitmap, matrix, paint);
iv2.setImageBitmap(scalebitmap);
}
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser)
{
if(seekBar==bar_scale)
{
tv_scale.setText(progress+1+"");
}
if(seekBar==bar_rotate)
{
tv_rotate.setText(progress+"゜");
}
if(seekBar==bar_tran)
{
tv_tran.setText(progress-50+"");
}
scale();
}
@Override
public void onStartTrackingTouch(SeekBar seekBar)
{
}
@Override
public void onStopTrackingTouch(SeekBar seekBar)
{
}
}