在开发的时候,经常会在页面上迭代输出一个集合中的内容,可以用在jsp页面上写脚本输出,这样比较麻烦,而且代码量很大,也不易维护。更好的方法是使用JSTL或Struts中的标签,代码简洁而且符合设计模式,这里我们使用JSP中的自定义标签,来实现和JSTL中的标签类似的功能。这里主要用到了TagSupport中的doStartTag和doAfterBody方法。
代码如下:
01 package org.spring;
02
03 /**
04 * @author Spring QQ196697651
05 */
06 import java.util.Collection;
07
08 import javax.servlet.jsp.JspException;
09 import javax.servlet.jsp.tagext.TagSupport;
10
11 public class IterationTag extends TagSupport {
12
13 private Collection<Object> items;
14
15 private String name;
16
17 private int i = 1;
18
19
20 @SuppressWarnings("unchecked")
21 public void setItems(String name) {
22 if(pageContext.getAttribute(name) != null){
23 items = (Collection<Object>)pageContext.getAttribute(name);
24 }else if(pageContext.getRequest().getAttribute(name) != null){
25 items = (Collection<Object>)pageContext.getRequest().getAttribute(name);
26 }else if(pageContext.getSession().getAttribute(name) != null){
27 items = (Collection<Object>)pageContext.getSession().getAttribute(name);
28 }else if(pageContext.getServletContext().getAttribute(name) != null){
29 items = (Collection<Object>)pageContext.getServletContext().getAttribute(name);
30 }else{
31 items = null;
32 }
33
34 }
35
36 public void setName(String name) {
37 this.name = name;
38 }
39
40
41 @Override
42 public int doStartTag() throws JspException {
43 if(items != null && items.size() > 0){
44 pageContext.setAttribute(name, items.toArray()[0]);
45 return EVAL_BODY_INCLUDE;
46 }else{
47 return SKIP_BODY;
48 }
49
50 }
51
52 @Override
53 public int doAfterBody() throws JspException {
54
55 if(i < items.size()){
56 pageContext.setAttribute(name, items.toArray()[i]);
57 i++;
58 return EVAL_BODY_AGAIN;
59
60 }else{
61 i = 1;
62 return SKIP_BODY;
63 }
64 }
65
66
67
68
69 }
02 <taglib xmlns= "http://java.sun.com/xml/ns/j2ee"
03 xmlns:xsi= "http://www.w3.rog/2001/XMLSchema-instance"
04 xsi:schemaLocation= "http://java.sun.com/xml/ns/j2ee
05 http://java.sun.com/xml/ns/j2ee/web-jsptaglibrary_2_0.xsd"
06 version= "2.0" >
07
08 <tlib-version>1.0 </tlib-version>
09 <short-name>spring </short-name>
10 <uri>/Spring </uri>
11
12 <tag>
13 <name>showIP </name>
14 <tag-class>org.spring.ShowIP </tag-class>
15 <body-content>empty </body-content>
16 </tag>
17
18 <tag>
19 <name>iterate </name>
20 <tag-class>org.spring.IterationTag </tag-class>
21 <body-content>JSP </body-content>
22 <attribute>
23 <name>name </name>
24 <required>true </required>
25 </attribute>
26 <attribute>
27 <name>items </name>
28 <required>true </required>
29 <rtexprvalue>true </rtexprvalue>
30 </attribute>
31 </tag>
32 </taglib>
01 <%@page contentType="text/html; charset=utf-8" %>
02 <%@taglib prefix="sp" uri="/Spring" %>
03
04
05 <%@page import="java.util.*"%>
06
07 <%
08 List<String> books = new ArrayList<String>();
09 books.add("Ant in Action");
10 books.add("Think in Java");
11 books.add("Effective Java");
12 books.add("Head First EJB");
13 books.add("J2EE Development With Out EJB");
14
15 pageContext.setAttribute("books",books);
16
17 %>
18 <html>
19 <head>
20 <title>迭代标签示例</title>
21 </head>
22 <body>
23 <ul>
24 <sp:iterate items="books" name="item">
25 <li>${item }</li>
26 </sp:iterate>
27 </ul>
28 </body>
29 </html>
运行效果:
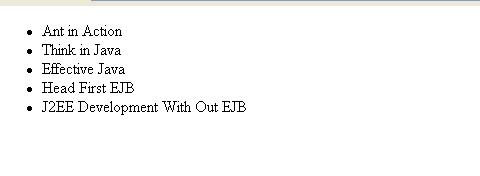
转载于:https://blog.51cto.com/honv77/522488