要求:给一个表做查询.很简单.简单到我不知他的什么要求.我那就只好对DataTable做查询了.数据也不知道有什么东东.本来还想对IList<T>做到查询的,有点麻烦,暂时不做了.
先放一个接口.定义了相关查询要的规范,也可以说是契约吗.
Code
1
public interface QueryClass
2

{
3
/**//// <summary>
4
/// 数据集的栏位
5
/// </summary>
6
List<string> Columns
7
{
8
get;
9
}
10
/**//// <summary>
11
/// 查询条件
12
/// </summary>
13
string Where
14
{
15
get;
16
set;
17
}
18
/**//// <summary>
19
/// 数据集
20
/// </summary>
21
object DataSource
22
{
23
get;
24
set;
25
}
26
/**//// <summary>
27
/// 查询
28
/// </summary>
29
/// <returns></returns>
30
object Select();
31
/**//// <summary>
32
/// And查询
33
/// </summary>
34
/// <param name="column"></param>
35
/// <param name="sing"></param>
36
/// <param name="value"></param>
37
/// <returns></returns>
38
string And(string column, string sing, string value);
39
string Or(string column, string sing, string value);
40
/**//// <summary>
41
/// 自动完成
42
/// </summary>
43
/// <param name="column"></param>
44
/// <returns></returns>
45
List<string> AutoComplete(string column);
46
/**//// <summary>
47
/// 查询条件变化后引起的事件
48
/// </summary>
49
event Event_Select event_Select;
50
/**//// <summary>
51
/// 自动完成选择多少需要的数据
52
/// </summary>
53
int AutoCompleteCount
{ get;set;}
54
List<string> AutoComplete(string column, string value);
55
}
56
/**//// <summary>
57
/// 事件所需的委托
58
/// </summary>
59
/// <param name="data"></param>
60
/// <returns></returns>
61
public delegate object Event_Select(object data);
下面是相关DataTable的实现.如下
Code
1
public class QueryTable : QueryClass
2

{
3
public event Event_Select event_Select;
4
private DataTable table;
5
public object DataSource
6
{
7
get
8
{
9
return table;
10
}
11
set
12
{
13
table = value as DataTable;
14
}
15
}
16
public QueryTable()
17
{
18
}
19
private List<string> columns;
20
public List<string> Columns
21
{
22
get
23
{
24
if (columns == null)
25
{
26
columns = new List<string>();
27
foreach (DataColumn c in table.Columns)
28
{
29
columns.Add(c.ColumnName);
30
}
31
}
32
return columns;
33
}
34
}
35
private string where = string.Empty;
36
public string Where
37
{
38
get
39
{
40
return where;
41
}
42
set
43
{
44
//当改变Where后就会引发函数Select(在里面会引发事件,使包含这个对象的对象也可以做相应的变化)
45
where = value;
46
Select();
47
}
48
}
49
public object Select()
50
{
51
DataTable newtable = new DataTable();
52
newtable = table.Clone();
53
DataRow[] rows = table.Select(where);
54
foreach(DataRow row in rows)
55
{
56
newtable.ImportRow(row);
57
}
58
// DataSource = newtable;
59
event_Select(newtable);
60
return newtable;
61
}
62
public string Select(string column, string sing, string value)
63
{
64
return And(column, sing, value);
65
}
66
public string And(string column, string sing, string value)
67
{
68
if (!string.IsNullOrEmpty(where))
69
{
70
where += " and ";
71
}
72
if (sing == "like")
73
{
74
value = "%" + value + "%";
75
}
76
Where += column + " " + sing + " " + "'" + value + "'" + " ";
77
return where;
78
}
79
public string Or(string column, string sing, string value)
80
{
81
if (!string.IsNullOrEmpty(where))
82
{
83
where += " or ";
84
}
85
if (sing == "like")
86
{
87
value = "%" + value + "%";
88
}
89
Where += column + " " + sing + " " + "'" + value + "'" + " ";
90
return where;
91
}
92
/**//// <summary>
93
/// 这个是把当前数据表的当前栏位的数据全部加进来,可能给上层去处理分析那些是用的,数据量少时这方法好(这个方法上层只要调用一次就行)
94
/// </summary>
95
/// <param name="column"></param>
96
/// <returns></returns>
97
public List<string> AutoComplete(string column)
98
{
99
List<string> items = new List<string>();
100
if (Columns.Contains(column))
101
{
102
foreach (DataRow row in table.Rows)
103
{
104
string c = row[column].ToString();
105
if (!items.Contains(c))
106
items.Add(c);
107
}
108
}
109
return items;
110
}
111
private int autoCompleteCount = 0;
112
public int AutoCompleteCount
113
{
114
get
115
{
116
if (autoCompleteCount == 0)
117
return int.MaxValue;
118
return autoCompleteCount;
119
}
120
set
121
{
122
autoCompleteCount = value;
123
}
124
}
125
/**//// <summary>
126
/// 根据当前栏位与输入进来要匹配的字符串来加入,适合数据量大的.实时的直接分析处理.(这个方法需要上层多次来调用,实时更新)
127
/// </summary>
128
/// <param name="column"></param>
129
/// <param name="value"></param>
130
/// <returns></returns>
131
public List<string> AutoComplete(string column,string value)
132
{
133
List<string> items = new List<string>();
134
if (Columns.Contains(column))
135
{
136
foreach (DataRow row in table.Rows)
137
{
138
string c = row[column].ToString();
139
if (!items.Contains(c))
140
{
141
if (c.Contains(value))
142
{
143
items.Add(c);
144
if (items.Count >= autoCompleteCount)
145
return items;
146
}
147
}
148
}
149
}
150
return items;
151
}
152
}
上面的实现后,定义一个类,用于与外部的类和上面的接口来交互.如下
Code
1
[Serializable]
2
public class QueryData
3

{
4
private QueryClass query;
5
private readonly string str_default = "--请选择--";
6
private readonly string str_error = "请输入正确的格式";
7
/**//// <summary>
8
/// 封装查询接口
9
/// </summary>
10
public QueryClass Query
11
{
12
get
13
{
14
return query;
15
}
16
private set
17
{
18
query = value;
19
}
20
}
21
static QueryData()
22
{
23
24
}
25
public QueryData(object data)
26
{
27
//暂时只支持DataTable,而IList接口因为相关有些功能暂时没完成
28
//用Reflector相看GridView的相关实现(如对DataSocuce对IList的实现)
29
//没有成功,不知那位有GridView的源码,能编译运行的.希望可以跟踪查看相关实现
30
if (data is DataTable)
31
{
32
query = new QueryTable();
33
}
34
dataSource = data;
35
query.DataSource = data;
36
query.event_Select += new Event_Select(query_event_Select);
37
}
38
/**//// <summary>
39
/// 当query对象的查询条件改变时,会引发这个函数使自己的数据集改变
40
/// </summary>
41
/// <param name="data">数据集</param>
42
/// <returns></returns>
43
public object query_event_Select(object data)
44
{
45
dataSource = data;
46
return dataSource;
47
}
48
49
private object dataSource;
50
/**//// <summary>
51
/// 数据集
52
/// </summary>
53
public object DataSource
54
{
55
get
56
{
57
return dataSource;
58
}
59
set
60
{
61
dataSource = value;
62
}
63
}
64
/**//// <summary>
65
/// 数据集的栏位
66
/// </summary>
67
/// <param name="list"></param>
68
public void Columns(DropDownList list)
69
{
70
list.Items.Clear();
71
foreach (string c in query.Columns)
72
{
73
list.Items.Add(c);
74
}
75
list.Items.Insert(0, str_default);
76
}
77
/**//// <summary>
78
/// 支持的查询比较
79
/// </summary>
80
/// <param name="list"></param>
81
public void Sign(DropDownList list)
82
{
83
list.Items.Clear();
84
list.DataSource = CompareSign.TableSing;
85
list.DataValueField = "Value";
86
list.DataTextField = "Key";
87
list.DataBind();
88
list.Items.Insert(0, str_default);
89
}
90
private string _value = string.Empty;
91
/**//// <summary>
92
/// 现暂留
93
/// </summary>
94
public string Value
95
{
96
get
97
{
98
return _value;
99
}
100
set
101
{
102
_value = value;
103
}
104
}
105
/**//// <summary>
106
/// 实现数据And的查询
107
/// </summary>
108
/// <param name="column"></param>
109
/// <param name="sing"></param>
110
/// <param name="_value"></param>
111
/// <returns></returns>
112
public string And(string column,string sing,string _value)
113
{
114
if (column == str_default || sing == str_default || string.IsNullOrEmpty(_value))
115
return str_error;
116
Value = _value;
117
query.And(column, sing, _value);
118
return query.Where;
119
}
120
/**//// <summary>
121
/// 实现数据Or的查询
122
/// </summary>
123
/// <param name="column"></param>
124
/// <param name="sing"></param>
125
/// <param name="_value"></param>
126
/// <returns></returns>
127
public string Or(string column, string sing, string _value)
128
{
129
if (column == str_default || sing == str_default || string.IsNullOrEmpty(_value))
130
return str_error;
131
Value = _value;
132
query.Or(column, sing, _value);
133
return query.Where;
134
}
135
136
/**//// <summary>
137
/// 给自动完成的相关接口
138
/// </summary>
139
/// <param name="column"></param>
140
/// <param name="value"></param>
141
/// <returns></returns>
142
public List<string> AutoComplete(string column, string value)
143
{
144
return query.AutoComplete(column, value);
145
}
146
}
后台就这么多了,本来我想直接做成一个用户控件,可是那样限制太多了.所以就没用了.用了一个表的数据实现了下,效
果可以接受.
页面没什么了,用QueryData类就好了,因为页面刷新后类就有为空了,用ViewState保存就好.我是如下做的.
Code
1
public QueryData queryData
2
{
3
get
4
{
5
if (ViewState["queryData"] != null)
6
return (QueryData)ViewState["queryData"];
7
else
8
{
9
queryData = new QueryData(你的数据集);
10
return queryData;
11
}
12
}
13
set
14
{
15
ViewState["queryData"] = value;
16
}
17
}
应下面一位朋友的要求,因为我没做什么美化,开始不好意思拿出来.差不多如下.

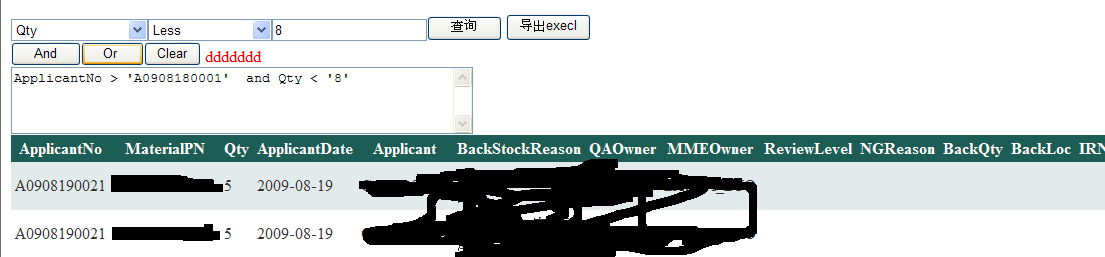
感想:
如果你永远把你的不足给藏着.那么你永远也进步不了.