提供了一些方便易用的、可扩展的缓冲机制。
这些缓冲机制可以使用在整体应用中的各个层面。
支持后期存储,支持的存储方式包括数据库方式和独立存储方式(Isolated storage), 便于应用方便的重新启动
便于使用
便于配置
支持使用配置工具
线程安全
可以确保在内存中的缓冲和后端存储保持同步
1.创建配置文件
在您的应用配置文件中增加一个缓冲应用
为要创建的数据项创建 Cache manager
每一个子项需要有独立的名字
确定哪一个是默认的Cache manager
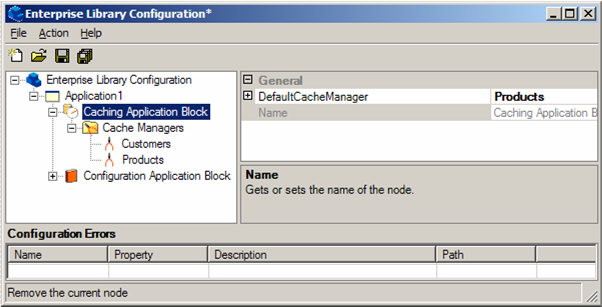
内存驻留型缓冲的典型应用:
应用程序经常使用同样的数据
一个应用程序经常需要重新获得数据
磁盘驻留型缓冲的典型应用:
数据量比较大
同时,从应用服务提供商(例如数据库)重新获取数据,开销比较大
在缓冲的生命周期中,必须经历系统的重新启动
创建默认 cache manager
CacheManager myCache = CacheManager.GetCacheManager();
创建命名的cache manager
CacheManager productsCache =
CacheManager.GetCacheManager(“Products”);
默认的增加一个条目
productsCache.Add(“ProductID123”, productObject);
基于时间的过期实例
DateTime refreshTime = new DateTime(2005, 3, 21, 2, 0, 0);
AbsoluteTime expireTime = new AbsoluteTime(refreshTime);
primitivesCache.Add("Key1", "Cache Item1", CacheItemPriority.Normal,
null, expireTime);
变化时间的过期
被访问5分钟后
TimeSpan refreshTime = new TimeSpan(0, 5, 0);
SlidingTime expireTime = new SlidingTime(refreshTime);
primitivesCache.Add("Key1", "Cache Item1", CacheItemPriority.Normal,
null, expireTime);
扩展时间应用范例
ExtendedFormatTime expireTime =
new ExtendedFormatTime("0 0 * * 6");
primitivesCache.Add("Key1", "Cache Item1", CacheItemPriority.Normal,
null, expireTime);
基于提醒机制的过期 文件依赖的例子
FileDependency expireNotice = new FileDependency(“Trigger.txt”);
productsCache.Add("Key1", "Cache Item1", CacheItemPriority.Normal,
null, expireNotice);
配置过期表决的频率
通过后台线程(BackgroundScheduler),移除过期事项
您可以对次线程进行配置
过高:CPU浪费,且CACHE没有起到作用
过低:内存消耗太大
建议使用性能计数器监视一下
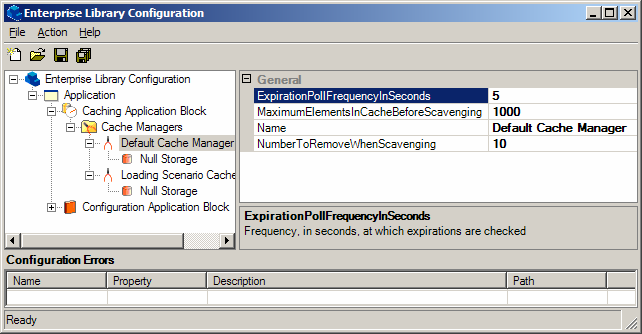
条目移除提示
Caching Application Block 提供了项目移除的提醒,并在一下情况下被激活
条目过期了
条目被显式的移除了
条目被策略的清楚了
需要实现 ICacheItemRefreshAction接口
一个类实现了 ICacheItemRefreshAction 接口,同时如果需要后端存储时,还必须被标识为 Serializable (Especially for persistent backing store)
[Serializable]
public class ProductCacheRefreshAction : ICacheItemRefreshAction
{
public void Refresh(string key, object expiredValue,
CacheItemRemovedReason removalReason)
{
// Item has been removed from cache.
// Perform desired actions here,
// based upon the removal reason (e.g. refresh the cache with the
// item).
}
}
从Cache manager中获取
类型要正确
一定要检查空值 (item not found in cache)
public Product ReadProductByID(string productID)
{
Product product = (Product)cache.GetData(productID);
if (product == null)
{
// Item not in cache
}
}
装载缓冲
缓冲的前期装载(Proactive loading)
应用启动时装载
优点
全部装载后,应用运行性能提升明显
缺点
启动时间长
可能带来不必要的资源浪费
为了提升启动性能而进行的——基于不同线程的装载,有造成了应用结构的复杂性
缓冲的被动装载(Reactive loading)
按需装载
优点
只有在需要的时候才装载,对资源的需求小
缺点
但是在首次装载的时候,速度慢
主动装载的实例
1:Create cache manager
CacheManager productsCache = CacheManager.GetCacheManager();
2:Add items during component initialization
// Retrieve the data from the source
ArrayList list = dataProvider.GetProductList();
// Add all the items to the cache
for (int i = 0; i < list.Count; i++)
{
Product product = (Product) list[i];
productsCache.Add( product.ProductID, product );
}
后期装载的实例
1:Create cache manager
CacheManager productsCache = CacheManager.GetCacheManager();
2:Add items when retrieved from data source
Product product = (Product) productsCache.GetData(productID);
if (product == null)
{
// Retrieve it from the data provider
// and cache it for more requests.
product = dataProvider.GetProductByID(productID);
if (product != null)
{
productsCache.Add(productID, product);
}
}
从缓冲中移除
1:Remove item with specified key
productsCache.Remove(“Product101Key”)
2:No error occurs if key is not found
因此通过这种方法,不能知道ITEM是否移除了
如果对象实现了ICacheRefreshItemAction,可以有信息
刷新缓冲
productsCache.Flush()
为缓冲建立优先级
productsCache.Add("Key1", "Cache Item1", CacheItemPriority.High,
new ProductCacheRefreshAction(), expireNotice)
实例
using
System;
using
System.Drawing;
using
System.Collections;
using
System.ComponentModel;
using
System.Windows.Forms;
using
System.Data;

using
Microsoft.Practices.EnterpriseLibrary.Caching;
using
Microsoft.Practices.EnterpriseLibrary.Caching.Expirations;
using
Microsoft.Practices.EnterpriseLibrary.Data;


namespace
CacheWindowsApplication

{

/**//// <summary>
/// Form1 的摘要说明。
/// </summary>
public class Form1 : System.Windows.Forms.Form

{
private System.Windows.Forms.Button btnQuery;

/**//// <summary>
/// 必需的设计器变量。
/// </summary>
private System.ComponentModel.Container components = null;
private System.Windows.Forms.Label label;
private System.Windows.Forms.ListBox listBox;
private System.Windows.Forms.Button btnGetFromCache;

// **********************************************
// Define parameters for demonstration
//***********************************************
private IDataReader myDr = null;
private System.Windows.Forms.Button btnTestExpire;
private CacheManager myCacheManager = null;
private System.Windows.Forms.Button btnReview;
private System.Windows.Forms.Button btnFileDependency;
private System.Windows.Forms.Button btnQueryByFile;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.Button button3;
private CacheManager IsolatedCacheManager =null;

public Form1()

{
//
// Windows 窗体设计器支持所必需的
//
InitializeComponent();

//
// TODO: 在 InitializeComponent 调用后添加任何构造函数代码
//
}


/**//// <summary>
/// 清理所有正在使用的资源。
/// </summary>
protected override void Dispose( bool disposing )

{
if( disposing )

{
if (components != null)

{
components.Dispose();
}
}
base.Dispose( disposing );
}


Windows 窗体设计器生成的代码#region Windows 窗体设计器生成的代码

/**//// <summary>
/// 设计器支持所需的方法 - 不要使用代码编辑器修改
/// 此方法的内容。
/// </summary>
private void InitializeComponent()

{
this.btnQuery = new System.Windows.Forms.Button();
this.label = new System.Windows.Forms.Label();
this.listBox = new System.Windows.Forms.ListBox();
this.btnGetFromCache = new System.Windows.Forms.Button();
this.btnTestExpire = new System.Windows.Forms.Button();
this.btnReview = new System.Windows.Forms.Button();
this.btnFileDependency = new System.Windows.Forms.Button();
this.btnQueryByFile = new System.Windows.Forms.Button();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.button3 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// btnQuery
//
this.btnQuery.Location = new System.Drawing.Point(24, 328);
this.btnQuery.Name = "btnQuery";
this.btnQuery.Size = new System.Drawing.Size(72, 24);
this.btnQuery.TabIndex = 1;
this.btnQuery.Text = "查询";
this.btnQuery.Click += new System.EventHandler(this.btnQuery_Click);
//
// label
//
this.label.Location = new System.Drawing.Point(32, 16);
this.label.Name = "label";
this.label.Size = new System.Drawing.Size(232, 23);
this.label.TabIndex = 2;
this.label.Text = "显示:";
//
// listBox
//
this.listBox.ItemHeight = 12;
this.listBox.Location = new System.Drawing.Point(32, 56);
this.listBox.Name = "listBox";
this.listBox.Size = new System.Drawing.Size(336, 244);
this.listBox.TabIndex = 3;
//
// btnGetFromCache
//
this.btnGetFromCache.Location = new System.Drawing.Point(104, 328);
this.btnGetFromCache.Name = "btnGetFromCache";
this.btnGetFromCache.TabIndex = 4;
this.btnGetFromCache.Text = "拿";
this.btnGetFromCache.Click += new System.EventHandler(this.btnGetFromCache_Click);
//
// btnTestExpire
//
this.btnTestExpire.Location = new System.Drawing.Point(192, 328);
this.btnTestExpire.Name = "btnTestExpire";
this.btnTestExpire.Size = new System.Drawing.Size(96, 23);
this.btnTestExpire.TabIndex = 5;
this.btnTestExpire.Text = "加个可过期的";
this.btnTestExpire.Click += new System.EventHandler(this.btnTestExpire_Click);
//
// btnReview
//
this.btnReview.Location = new System.Drawing.Point(304, 328);
this.btnReview.Name = "btnReview";
this.btnReview.Size = new System.Drawing.Size(72, 24);
this.btnReview.TabIndex = 6;
this.btnReview.Text = "在看一下";
this.btnReview.Click += new System.EventHandler(this.btnReview_Click);
//
// btnFileDependency
//
this.btnFileDependency.Location = new System.Drawing.Point(24, 360);
this.btnFileDependency.Name = "btnFileDependency";
this.btnFileDependency.Size = new System.Drawing.Size(104, 24);
this.btnFileDependency.TabIndex = 7;
this.btnFileDependency.Text = "文件类型的";
this.btnFileDependency.Click += new System.EventHandler(this.btnFileDependency_Click);
//
// btnQueryByFile
//
this.btnQueryByFile.Location = new System.Drawing.Point(144, 360);
this.btnQueryByFile.Name = "btnQueryByFile";
this.btnQueryByFile.Size = new System.Drawing.Size(104, 24);
this.btnQueryByFile.TabIndex = 8;
this.btnQueryByFile.Text = "文件类型查询";
this.btnQueryByFile.Click += new System.EventHandler(this.btnQueryByFile_Click);
//
// button1
//
this.button1.Location = new System.Drawing.Point(272, 360);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(104, 24);
this.button1.TabIndex = 9;
this.button1.Text = "文件类型不要了";
this.button1.Click += new System.EventHandler(this.button1_Click_1);
//
// button2
//
this.button2.Location = new System.Drawing.Point(24, 400);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(104, 24);
this.button2.TabIndex = 10;
this.button2.Text = "优先级问题";
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// button3
//
this.button3.Location = new System.Drawing.Point(144, 400);
this.button3.Name = "button3";
this.button3.Size = new System.Drawing.Size(232, 24);
this.button3.TabIndex = 11;
this.button3.Text = "在来几个高优先级";
this.button3.Click += new System.EventHandler(this.button3_Click);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(6, 14);
this.ClientSize = new System.Drawing.Size(408, 478);
this.Controls.Add(this.button3);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.Controls.Add(this.btnQueryByFile);
this.Controls.Add(this.btnFileDependency);
this.Controls.Add(this.btnReview);
this.Controls.Add(this.btnTestExpire);
this.Controls.Add(this.btnGetFromCache);
this.Controls.Add(this.listBox);
this.Controls.Add(this.label);
this.Controls.Add(this.btnQuery);
this.Name = "Form1";
this.Text = "Form1";
this.Load += new System.EventHandler(this.Form1_Load);
this.ResumeLayout(false);

}
#endregion


/**//// <summary>
/// 应用程序的主入口点。
/// </summary>
[STAThread]
static void Main()

{
Application.Run(new Form1());
}

private void btnQuery_Click(object sender, System.EventArgs e)

{
this.label.Text = System.Convert.ToString(myCacheManager.Count);
}

private void Form1_Load(object sender, System.EventArgs e)

{
Database db = DatabaseFactory.CreateDatabase("Database Instance");
IDataReader dr = db.ExecuteReader(CommandType.Text,"Select * from Products");

this.myDr=dr;
myCacheManager = CacheFactory.GetCacheManager();
myCacheManager.Add("MyDataReader",this.myDr);
}

private void btnGetFromCache_Click(object sender, System.EventArgs e)

{
IDataReader toBeDisplay = (IDataReader)myCacheManager.GetData("MyDataReader");
if(toBeDisplay != null)

{
while(toBeDisplay.Read())

{
this.listBox.Items.Add(toBeDisplay.GetValue(2));
}
}
}

private void btnTestExpire_Click(object sender, System.EventArgs e)

{
Database db = DatabaseFactory.CreateDatabase("Database Instance");
DataSet ds = db.ExecuteDataSet(CommandType.Text,"Select * from Products");
IsolatedCacheManager = CacheFactory.GetCacheManager("Isolated Cache Manager");
DateTime refreshTime = new DateTime(2005, 6, 24, 12, 51, 30);
AbsoluteTime expireTime = new AbsoluteTime(refreshTime);
IsolatedCacheManager.Add("MyDataSet",ds, CacheItemPriority.Normal, null,expireTime);
}

private void btnReview_Click(object sender, System.EventArgs e)

{
this.label.Text = System.Convert.ToString(IsolatedCacheManager.Count);
}

private void button1_Click(object sender, System.EventArgs e)

{
}

private void btnFileDependency_Click(object sender, System.EventArgs e)

{
FileDependency expireNotice = new FileDependency("DependencyFile.txt");
myCacheManager.Add("FileKey", "String: Test Cache Item Dependency", CacheItemPriority.Normal, null, expireNotice);
}

private void btnQueryByFile_Click(object sender, System.EventArgs e)

{
this.label.Text = System.Convert.ToString(myCacheManager.Count);
}

private void button1_Click_1(object sender, System.EventArgs e)

{
myCacheManager.Remove("FileKey");
}

private void button2_Click(object sender, System.EventArgs e)

{
for(int intCount=0; intCount<8; intCount++)

{
string strKeyName = "Key"+System.Convert.ToString(intCount);
if (intCount%2 == 0)

{
myCacheManager.Add(strKeyName, "High", CacheItemPriority.High, null, null);
}
else

{
myCacheManager.Add(strKeyName, "Low", CacheItemPriority.Low, null, null);
}
}
}

private void button3_Click(object sender, System.EventArgs e)

{
for(int intCount=0; intCount<5; intCount++)

{
string strKeyName = "HighKey"+System.Convert.ToString(intCount);
myCacheManager.Add(strKeyName, "High", CacheItemPriority.High, null, null);
}
}
}
}