time库的使用
# time库包括三类函数
- 时间获取:time() ctime() gmtime()
- 时间格式化:strftime() strptime()
- 程序计时:sleep() perf_counter()
time()获取当前时间戳,即计算机内部时间值,浮点数
表示从1970年1月1日0点0分0秒开始到现在
>>> import time
>>> time.time()
1540037306.0050652
2018.10.20 - 1970.01.01 = 48.09.19
60 * 60 * 24 * 30 * 12 = 31,104,000
48 * 31,104,000=1,244,160,000
>>> time.ctime()
'Sat Oct 20 20:18:45 2018'
周 月 日 时:分:秒 年
>>> time.gmtime()
time.struct_time(tm_year=2018, tm_mon=10, tm_mday=20, tm_hour=12, tm_min=20, tm_sec=37, tm_wday=5, tm_yday=293, tm_isdst=0)
计算机可识别模式
strftime(tpl,ts)
tpl是格式化模板字符串,用来定义输出效果
ts是计算机内部时间类型变量
>>> t = time.gmtime()
>>> time.strftime("%Y-%m-%d %H:%M:%S",t)
'2018-10-20 12:23:28'
%Y年份,0000~9999
%m月份,01~12
%B月份名称,January~December
%b月份名称缩写,Jan~Dec
%d日期,01~31
%A星期,Monday~Sunday
%a星期缩写,Mon~Sun
%H小时(24),00~23
%h小时(12),01~12
%p上/下午,AM,PM
%M分钟,00~59
%S秒,00~59
strptime(str,tpl)
>>> timeStr = '2018-10-20 12:23:28'
>>> time.strptime(timeStr,"%Y-%m-%d %H:%M:%S")
time.struct_time(tm_year=2018, tm_mon=10, tm_mday=20, tm_hour=12, tm_min=23, tm_sec=28, tm_wday=5, tm_yday=293, tm_isdst=-1)
程序计时指测量起止动作所经历时间的过程
测量时间:perf_counter()
产生时间:sleep()
perf_counter()
返回一个CPU级别的精确时间计数值,单位为s
由于计算值起点不确定,连续调用差值才有意义
>>> start = time.perf_counter()
>>> start
2041.101892374
>>> end = time.perf_counter()
>>> end
2070.363240708
>>> end -start
29.261348333999877
>>> time.perf_counter() - time.perf_counter()
0.0
测试程序运行的时间
sleep(s)
s拟休眠的时间,单位是秒,可以是浮点数
>>> def wait():
time.sleep(3.3)
>>> wait()
程序将等待3.3秒后再退出
文本进度条
#!/usr/bin/env python3
# -*- coding: UTF-8 -*-
#TestProBarV1.py
import time
scale = 10
print("------执行开始------")
for i in range(scale + 1):
a = '#' * i
b = '.' * (scale - i)
c = (i / scale) * 100
print("{:^3.0f}%[{}->{}]".format(c,a,b))
time.sleep(0.1)
print("------执行结束------")
====================== RESTART: C:\Python3.7.0\test.py ======================
------执行开始------
0 %[->..........]
10 %[#->.........]
20 %[##->........]
30 %[###->.......]
40 %[####->......]
50 %[#####->.....]
60 %[######->....]
70 %[#######->...]
80 %[########->..]
90 %[#########->.]
100%[##########->]
------执行结束------
刷新的本质:用后打印的字符覆盖之前的字符
不能换行:print()需要被控制
要能回退:打印后光标退回到之前的位置\r
#!/usr/bin/env python3
# -*- coding: UTF-8 -*-
#TestProBarV1.py
import time
for i in range(101):
print("\r{:3}%".format(i),end="")
time.sleep(0.1)
====================== RESTART: C:\Python3.7.0\test.py ======================
0%
1%
2%
3%
4%
5%
6%
7%
8%
9%
10%
11%
12%
13%
14%
15%
16%
17%
18%
19%
20%
21%
22%
23%
24%
25%
26%
27%
28%
29%
30%
31%
32%
33%
34%
35%
36%
37%
38%
39%
40%
41%
42%
43%
44%
45%
46%
47%
48%
49%
50%
51%
52%
53%
54%
55%
56%
57%
58%
59%
60%
61%
62%
63%
64%
65%
66%
67%
68%
69%
70%
71%
72%
73%
74%
75%
76%
77%
78%
79%
80%
81%
82%
83%
84%
85%
86%
87%
88%
89%
90%
91%
92%
93%
94%
95%
96%
97%
98%
99%
100%
使用IDLE输出会,因为这是开发环境下
Python 3.7.0 (v3.7.0:1bf9cc5093, Jun 27 2018, 04:59:51) [MSC v.1914 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import time
>>> for i in range(101):
... print("\r{:3}%".format(i),end="")
... time.sleep(0.1)
...
100%
使用窗口模式下输出则看到从0%到100%
#!/usr/bin/env python3
# -*- coding: UTF-8 -*-
#TestProBarV1.py
import time
scale = 50
print("执行开始".center(scale//2,"-"))
start = time.perf_counter()
for i in range(scale + 1):
a = '#' * i
b = '.' * (scale - i)
c = (i / scale) * 100
dur = time.perf_counter() - start
print("\r{:^3.0f}%[{}->{}]{:.2f}s".format(c,a,b,dur),end='')
time.sleep(0.1)
print("\n"+"执行结束".center(scale//2,'-'))
Microsoft Windows [版本 10.0.17134.345]
(c) 2018 Microsoft Corporation。保留所有权利。
C:\Windows\System32> path=%path%;D:\Python\Python37\
C:\Windows\System32>cd C:\Python3.7.0
C:\Python3.7.0>python test.py
-----------执行开始----------
100%[##################################################->]5.05s
-----------执行结束----------
Linear coonstant f(x) = x
Early Pause Speeds up f(x) = x+(1-sin(x*pai*2+pai/2)/-8
Late Pause Slows down f(x) = x+(1-sin(x*pai*2+pai)/8
Slow Wavy Constant f(x) = x+sin(x*pai*5)/20
Fast Wavy Constant f(x) = x+sin(x*pai*20)/80
Power Speed up f(x) = (x+(1-x)*0.03)^2
Inverse Power Slows down f(x) = 1+(1-x)^1.5*-1
Fast Power Speeds up f(x) = (x+(1-x)/2)^2
Inverse Fast Power Slows down f(x) = 1+(1-x)^3*-1
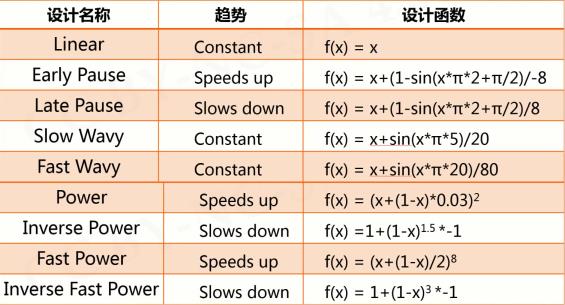
主要靠\r和end=''
#!/usr/bin/env python3
# -*- coding: UTF-8 -*-
#TestProBarV1.py
import time
for i in range(100):
print("\r",i,end='')
time.sleep(0.1)
C:\Python3.7.0>python test.py
99