看代码:
ThinkphpLibraryThinkSessionDriver中新建redis缓存文件:Redis.class.php
ThinkphpCommonfunction.php 中 function session($name='',$value='') //session说明文件
一:配置文件中新加:
//redis操作session
'SESSION_AUTO_START' => true, // 是否自动开启Session
'SESSION_TYPE' => 'Redis', //session类型
'SESSION_PERSISTENT' => 1, //是否长连接(对于php来说0和1都一样)
'SESSION_CACHE_TIME' => 3000, //连接超时时间(秒)
'SESSION_EXPIRE' => 0, //session有效期(单位:秒) 0表示永久缓存
'SESSION_PREFIX' => 'sses_', //session前缀
二:Redis.class.php中内容:
<?php
namespace ThinkSessionDriver;
/**
* Redis Session驱动
* 要求安装phpredis扩展:https://github.com/nicolasff/phpredis
* @category Think
* @package Session
* @subpackage Driver
* @version TP3.2~TP3.2.1
*/
class Redis implements SessionHandlerInterface{
/**
* Redis句柄
*/
private $handler;
private $get_result;
public function __construct(){
if ( !extension_loaded('redis') ) {
E(L('_NOT_SUPPERT_').':redis');
}
if(empty($options)) {
$options = array (
'host' => C('REDIS_HOST') ? C('REDIS_HOST') : '127.0.0.1',
'port' => C('REDIS_PORT') ? C('REDIS_PORT') : 6379,
'timeout' => C('SESSION_CACHE_TIME') ? C('SESSION_CACHE_TIME') : false,
'persistent' => C('SESSION_PERSISTENT') ? C('SESSION_PERSISTENT') : false,
'auth' => C('REDIS_AUTH') ? C('REDIS_AUTH') : false,
'dbindex' => C('REDIS_DBINDEX') ? C('REDIS_DBINDEX') : 0, //选择存库
);
}
$options['host'] = explode(',', $options['host']);
$options['port'] = explode(',', $options['port']);
$options['auth'] = explode(',', $options['auth']);
foreach ($options['host'] as $key=>$value) {
if (!isset($options['port'][$key])) {
$options['port'][$key] = $options['port'][0];
}
if (!isset($options['auth'][$key])) {
$options['auth'][$key] = $options['auth'][0];
}
}
$this->options = $options;
$expire = C('SESSION_EXPIRE');
$this->options['expire'] = isset($expire) ? (int)$expire : (int)ini_get('session.gc_maxlifetime');;
$this->options['prefix'] = isset($options['prefix']) ? $options['prefix'] : C('SESSION_PREFIX');
$this->options['dbindex'] = isset($options['dbindex'])? $options['dbindex'] : 0; //选择存库
$this->handler = new Redis;
}
/**
* 连接Redis服务端
* @access public
* @param bool $is_master : 是否连接主服务器
*/
public function connect($is_master = true) {
if ($is_master) {
$i = 0;
} else {
$count = count($this->options['host']);
if ($count == 1) {
$i = 0;
} else {
$i = rand(1, $count - 1); //多个从服务器随机选择
}
}
$func = $this->options['persistent'] ? 'pconnect' : 'connect';
try {
if ($this->options['timeout'] === false) {
$result = $this->handler->$func($this->options['host'][$i], $this->options['port'][$i]);
if (!$result)
throw new ThinkException('Redis Error', 100);
} else {
$result = $this->handler->$func($this->options['host'][$i], $this->options['port'][$i], $this->options['timeout']);
if (!$result)
throw new ThinkException('Redis Error', 101);
}
if ($this->options['auth'][$i]) {
$result = $this->handler->auth($this->options['auth'][$i]);
if (!$result) {
throw new ThinkException('Redis Error', 102);
}
}
//选择db存库
if (0 != $this->options['dbindex']) {
$this->handler->select($this->options['dbindex']);
}
} catch ( Exception $e ) {
exit('Error Message:'.$e->getMessage().'<br>Error Code:'.$e->getCode().'');
}
}
/**
* 打开Session
* @access public
* @param string $savePath
* @param mixed $sessName
*/
public function open($savePath, $sessName) {
return true;
}
/**
* 关闭Session
* @access public
*/
public function close() {
if ($this->options['persistent'] == 'pconnect') {
$this->handler->close();
}
return true;
}
/**
* 读取Session
* @access public
* @param string $sessID
*/
public function read($sessID) {
$this->connect(0);
$this->get_result = $this->handler->get($this->options['prefix'].$sessID);
return $this->get_result;
}
/**
* 写入Session
* @access public
* @param string $sessID
* @param String $sessData
*/
public function write($sessID, $sessData) {
if (!$sessData || $sessData == $this->get_result) {
return true;
}
$this->connect(1);
$expire = $this->options['expire'];
$sessID = $this->options['prefix'].$sessID;
if(is_int($expire) && $expire > 0) {
$result = $this->handler->setex($sessID, $expire, $sessData);
$re = $result ? 'true' : 'false';
}else{
$result = $this->handler->set($sessID, $sessData);
$re = $result ? 'true' : 'false';
}
return $result;
}
/**
* 删除Session
* @access public
* @param string $sessID
*/
public function destroy($sessID) {
$this->connect(1);
return $this->handler->delete($this->options['prefix'].$sessID);
}
/**
* Session 垃圾回收
* @access public
* @param string $sessMaxLifeTime
*/
public function gc($sessMaxLifeTime) {
return true;
}
/**
* 打开Session
* @access public
* @param string $savePath
* @param mixed $sessName
*/
public function execute() {
session_set_save_handler(
array(&$this, "open"),
array(&$this, "close"),
array(&$this, "read"),
array(&$this, "write"),
array(&$this, "destroy"),
array(&$this, "gc")
);
}
public function __destruct() {
if ($this->options['persistent'] == 'pconnect') {
$this->handler->close();
}
session_write_close();
}
}
以上内容希望帮助到大家,很多PHPer在进阶的时候总会遇到一些问题和瓶颈,业务代码写多了没有方向感,不知道该从那里入手去提升,对此我整理了一些资料,包括但不限于:分布式架构、高可扩展、高性能、高并发、服务器性能调优、TP6,laravel,YII2,Redis,Swoole、Swoft、Kafka、Mysql优化、shell脚本、Docker、微服务、Nginx等多个知识点高级进阶干货需要的可以免费分享给大家,需要
PHP进阶架构师>>>视频、面试文档免费获取shimo.im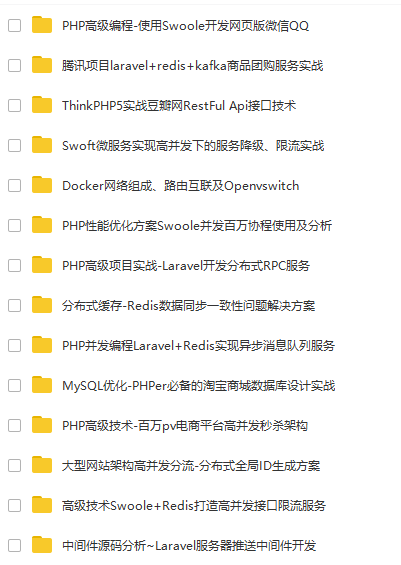
或 者关注咱们下面的知乎专栏
PHP大神进阶zhuanlan.zhihu.com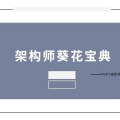