
选择使用空白模板,点击Open this Template
2、输入报表名称,点击下一步
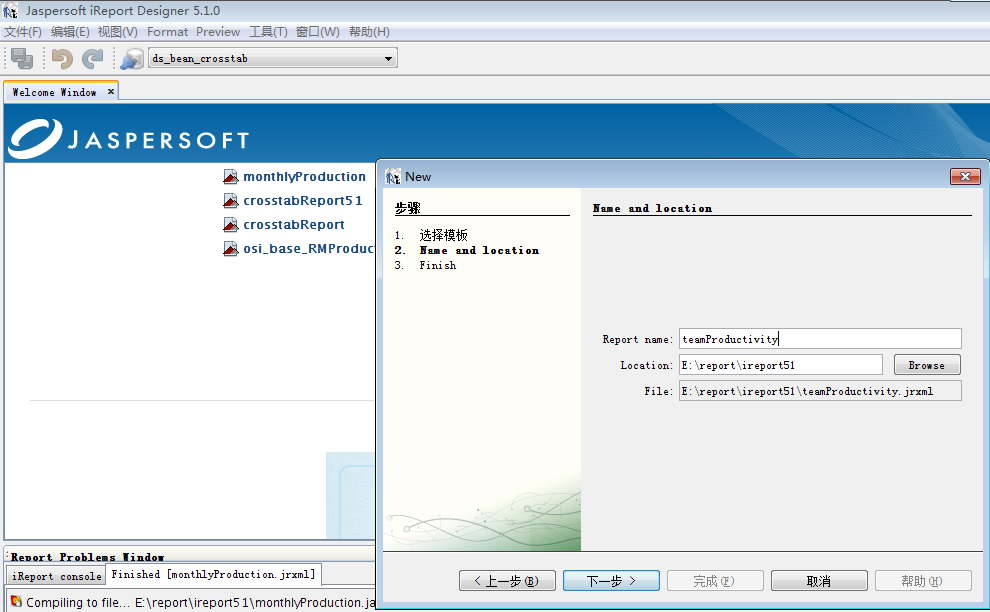
3、点击完成,就新建了一个新的空白报表
4、删除多余的band,只保留summary,报表的内容全放到这里
5、新建parameter,用来接收数据,一定要记得修改参数类型
6、新建dataset,填好名称,点击下一步
7、新建数据源
点击save,然后点击下一步
8、点击下一步
9、点击完成,dataset创建完毕
10、在java项目中,新建Javabean,用来存数据
public class CategoryBean {
private String id;
private String series;
private String category;
private String value;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getSeries() {
return series;
}
public void setSeries(String series) {
this.series = series;
}
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
11、将javabean打包成jar包,并且加入到iReport的classpath里
工具-选项-classpath-添加jar包
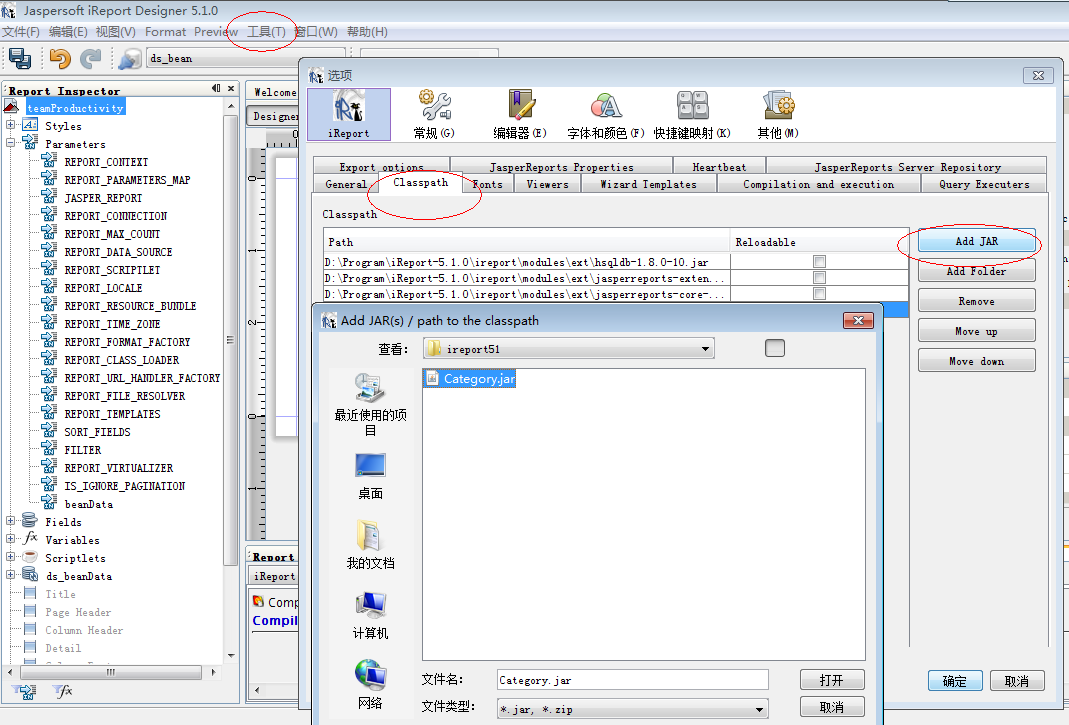
12、edit query

13、向报表中添加crosstab组件,选择刚才新建的数据源,点击下一步
14、设置行数据,选择series
15、设置列数据,选择category
16、设置data,选择value字段,function选择nothing
17、设置布局,去掉不需要的计算行列total的选项,点击完成
18,编辑交叉表数据,在添加好的crosstab上点右键-Crosstab data
注意选择合适的数据源类型和表达式
到这里组件的添加和设置就算完成了,表格的样式可以根据需要修改。
特别注意的是要注意勾选Data is pre-sorted,否则数据会重新排序。
19、点击编译生成jasper文件供调用
20、新建java类,用来填充数据,调用刚才生成的jasper文件并导出想要的文件格式,比如pdf,excel等
public class TeamProductivity {
public static void main(String[] args) {
try {
TeamProductivity report=new TeamProductivity();
report.genReport();
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* @throws Exception
* @Method: genReport
* @Author: Liaolz
* @Description: 生成报表文件
*
* @param
* @return void
* @throws
*/
public void genReport() throws Exception {
System.out.println("=========开始生成报表=========");
long startTime = System.currentTimeMillis();
//准备报表数据
List<CategoryBean> beanDataList = new ArrayList<CategoryBean>();
Map<String, Object> parameters = new HashMap<String, Object>();
setCrosstabData(beanDataList);
//主数据源
JRBeanCollectionDataSource ds = new JRBeanCollectionDataSource(beanDataList);
//交叉表数据源
JRBeanCollectionDataSource dsCrosstab = new JRBeanCollectionDataSource(beanDataList);
parameters.put("beanData", dsCrosstab);
//导出报表
String jasperFileName="E:\\report\\ireport51\\teamProductivity.jasper";
String exportFileName="e:\\export\\teamProductivity"+startTime;
exportReportToExcel(parameters,ds,jasperFileName,exportFileName);
System.out.println("=========生成报表完成,所需时间:" + ((System.currentTimeMillis() - startTime)/1000) + "秒=========");
}
private void setCrosstabData(List<CategoryBean> beanList){
String[] series={"产出箱数","生产小时","成型次数","人头数","","次品合计","A","B","C","D"};
String[] category={"早班","中班","备注"};
String[][] value={{"730箱","891箱",""},
{"0","0",""},
{"78","78",""},
{"0","0",""},
{"","",""},
{"0","0",""},
{"","","不规则"},
{"","","偏小"},
{"","",""},
{"","",""}};
setBeanList(beanList,series,category,value);
}
private void setBeanList(List<CategoryBean> beanList,String[] series,String[] category,String[][] value){
int seriesLen=series.length;
int categoryLen=category.length;
for(int i=0;i<seriesLen;i++){
for(int j=0;j<categoryLen;j++){
CategoryBean dataCrosstab = new CategoryBean();
dataCrosstab.setSeries(series[i]);
dataCrosstab.setCategory(category[j]);
if(value[i][j]!=null){
dataCrosstab.setValue(value[i][j]);
}
beanList.add(dataCrosstab);
}
}
}
private void exportReportToPDF(Map<String, Object> parameters,JRBeanCollectionDataSource ds,String jasperFileName,String exportFileName)throws Exception{
//生成JasperPrint
JasperReport report = (JasperReport)JRLoader.loadObject(new File(jasperFileName));
JasperPrint jasperPrint = JasperFillManager.fillReport(report, parameters, ds);
OutputStream ouputStream = new FileOutputStream(new File(exportFileName+".pdf"));
//使用JRPdfExproter导出器导出pdf
JRPdfExporter exporter = new JRPdfExporter();
//设置JasperPrintList
exporter.setParameter(JRExporterParameter.JASPER_PRINT, jasperPrint);
exporter.setParameter(JRExporterParameter.OUTPUT_STREAM, ouputStream);
exporter.exportReport();
ouputStream.close();
}
private void exportReportToExcel(Map<String, Object> parameters,JRBeanCollectionDataSource ds,String jasperFileName,String exportFileName)throws Exception{
JasperReport report=(JasperReport)JRLoader.loadObject(new File(jasperFileName));
JasperPrint jasperPrint =JasperFillManager.fillReport(report,parameters, ds);
FileOutputStream ouputStream = new FileOutputStream(exportFileName+".xls");
JRAbstractExporter exporter = new JExcelApiExporter();
exporter.setParameter(JRExporterParameter.JASPER_PRINT, jasperPrint);
exporter.setParameter(JRExporterParameter.OUTPUT_STREAM, ouputStream);
exporter.setParameter(JRXlsExporterParameter.IS_ONE_PAGE_PER_SHEET,Boolean.FALSE);
exporter.exportReport();
ouputStream.close();
}
}
导出的excel截图
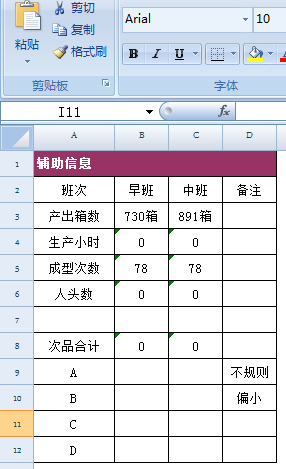
可以设置多个dataset,生成多个图表,如下所示