XML解析技术概述
Demo2.java
import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import org.w3c.dom.Document; public class Demo2 { public static void main(String args[])throws Exception { //1.创建工程 DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); //2.得到dom解析器 DocumentBuilder builder = factory.newDocumentBuilder(); //3.解析xml文档,得到代表文档的document Document document = builder.parse("src/book.xml"); } }
xml解析技术概述和使用Jaxp对xml文档进行dom解析
package cn.lysine; import org.junit.Test; import org.w3c.dom.Document; import org.w3c.dom.Element; import org.w3c.dom.Node; import org.w3c.dom.NodeList; import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import javax.xml.parsers.ParserConfigurationException; //使用dom方式对xml文档进行 增删查改 CRUD public class Demo3 { // 读取xml文档中;<书名>java就业培训中心</书名> 节点的值 @Test public void read1() throws Exception { DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); NodeList list = document.getElementsByTagName("书名"); Node node = list.item(1); String content = node.getTextContent(); System.out.println(content); //输出 java就业培训中心 } //得到xml中标签属性的值 @Test public void read2() throws Exception { DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); // 得到根结点 Node root = document.getElementsByTagName("书架").item(0); list(root); } private void list(Node node) { System.out.println(node.getNodeName()); NodeList list = node.getChildNodes(); for(int i = 0; i <list.getLength(); i++ ){ Node childe = list.item(i); list(childe); } } //得到xml文档中标签属性的值:<书名 name="xxxx">java就业培训教材</书名> @Test public void read3() throws Exception{ DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); Element bookname = (Element)document.getElementsByTagName("书名").item(0); String value = bookname.getAttribute("name"); System.out.println(value); } //输出 xxxx }
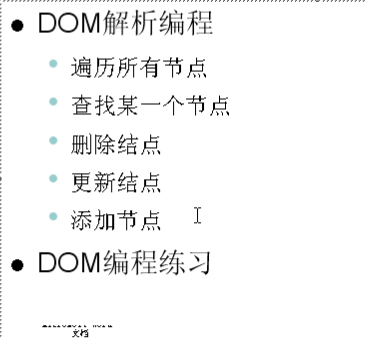
import java.io.FileOutputStream; import java.io.IOException; import org.junit.Test; import org.w3c.dom.Document; import org.w3c.dom.Element; import org.w3c.dom.Node; import org.w3c.dom.NodeList; import org.xml.sax.SAXException; import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import javax.xml.parsers.ParserConfigurationException; import javax.xml.transform.Transformer; import javax.xml.transform.TransformerFactory; import javax.xml.transform.dom.DOMSource; import javax.xml.transform.stream.StreamResult; //使用dom方式对xml文档进行 增删查改 CRUD public class Demo3 { // 读取xml文档中;<书名>java就业培训中心</书名> 节点的值 @Test public void read1() throws Exception { DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); NodeList list = document.getElementsByTagName("书名"); Node node = list.item(0); String content = node.getTextContent(); System.out.println(content); } //得到xml中标签属性的值 @Test public void read2() throws Exception { DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); // 得到根结点 Node root = document.getElementsByTagName("书架").item(0); list(root); } private void list(Node node) { System.out.println(node.getNodeName()); NodeList list = node.getChildNodes(); for(int i = 0; i <list.getLength(); i++ ){ Node childe = list.item(i); list(childe); } } //得到xml文档中标签属性的值:<书名 name="xxxx">java就业培训教材</书名> @Test public void read3() throws Exception{ DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); Element bookname = (Element)document.getElementsByTagName("书名").item(0); String value = bookname.getAttribute("name"); System.out.println(value); } //向xml文档中添加节点:<售价>59.00元</售价> @Test public void add() throws Exception{ DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); //创建节点 Element price = document.createElement("售价"); price.setTextContent("59.00元"); //得到参考节点 Element refNode = (Element) document.getElementsByTagName("售价").item(0); //得到要挂崽的节点 把创建的节点挂到第一本书上 Element book = (Element)document.getElementsByTagName("书").item(0); //往book节点的指定位置插崽 book.insertBefore(price,refNode); //把更新后内存写回到xml文档 TransformerFactory tffactory = TransformerFactory.newInstance(); Transformer tf = tffactory.newTransformer(); tf.transform(new DOMSource(document), new StreamResult(new FileOutputStream("src/book.xml"))); } //add 向xml文档中添加节点:<书名>java就业培训教程</书名> 上添加name=“xxxx”属性 @Test public void addAttr() throws Exception{ DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); Element bookname = (Element) document.getElementsByTagName("书名").item(0); bookname.setAttribute("name", "xxxxx"); //把更新后内存写回到xml文档 TransformerFactory tffactory = TransformerFactory.newInstance(); Transformer tf = tffactory.newTransformer(); tf.transform(new DOMSource(document),new StreamResult(new FileOutputStream("src/book.xml"))); } //删除整个xml @Test public void delete1() throws Exception{ DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); //得到要删除的结点 Element e = (Element) document.getElementsByTagName("售价").item(0); //得到要删除的结点的爸爸 Element book = (Element) document.getElementsByTagName("书").item(0); //爸爸再删崽 book.removeChild(e); //把更新后内存写回到xml文档 TransformerFactory tffactory = TransformerFactory.newInstance(); Transformer tf = tffactory.newTransformer(); tf.transform(new DOMSource(document),new StreamResult(new FileOutputStream("src/book.xml"))); } public void delete2() throws Exception{ DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); //得到要删除的结点 Element e = (Element) document.getElementsByTagName("售价").item(0); e.getParentNode().getParentNode().getParentNode().removeChild(e.getParentNode().getParentNode()); //把更新后内存写回到xml文档 TransformerFactory tffactory = TransformerFactory.newInstance(); Transformer tf = tffactory.newTransformer(); tf.transform(new DOMSource(document),new StreamResult(new FileOutputStream("src/book.xml"))); } //update 更新 public void update()throws ParserConfigurationException, SAXException, IOException,Exception{ DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("src/book.xml"); //得到要更新的结点 Element e = (Element) document.getElementsByTagName("售价").item(0); e.setTextContent("109元"); //把更新后内存写回到xml文档 TransformerFactory tffactory = TransformerFactory.newInstance(); Transformer tf = tffactory.newTransformer(); tf.transform(new DOMSource(document),new StreamResult(new FileOutputStream("src/book.xml"))); } }