List<RuleHour> exSeasonOut = new ArrayList<RuleHour>();
<tr id="exSeasonOutTr">
<td>
<sky:datePicker name="ruleVO.exSeasonOut[${status.index}].fromDate,ruleVO.exSeasonOut[${status.index}].toDate" />
</td>
</tr>
但是页面中需要可以通过js动态添加行,实现代码如下
$("exSeason").on("click",addRow);
/* copy the tr(id) and append to the table, change the id、name of the new tr at the same time */
function addRow(id) {
var tr = $(id);
if(!tr.index) {
tr.index = 0;
}
tr.index++;
var newTr = tr.cloneNode(true);
newTr.innerHTML = newTr.innerHTML.replace(/[\d]/g, tr.index);
tr.parentElement.appendChild(newTr);
}
看一下这行代码的效果:
newTr.innerHTML = newTr.innerHTML.replace(/[\d]/g, tr.index);

结果td的起始标签不见了,替换后只剩下td的结束标签,原因未知

然后试着将td的innerHTML进行替换
newTr.childNodes[0].innerHTML = newTr.childNodes[0].innerHTML.replace(/[\d]/g, tr.index);
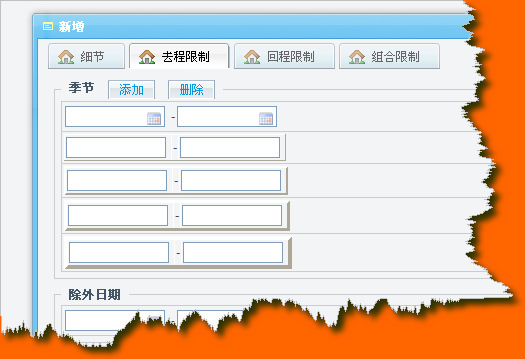
调试一看,样式及一些自定义属性不见了
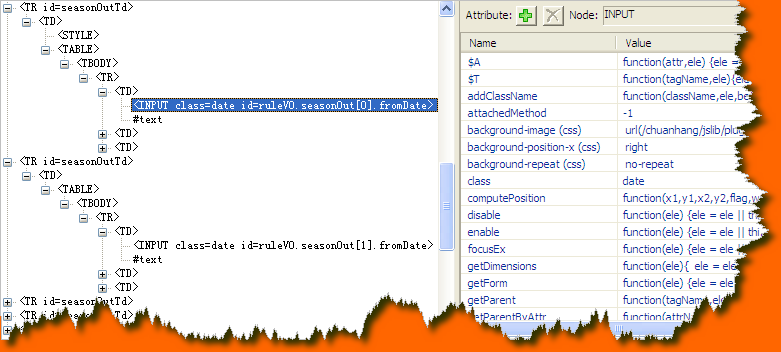
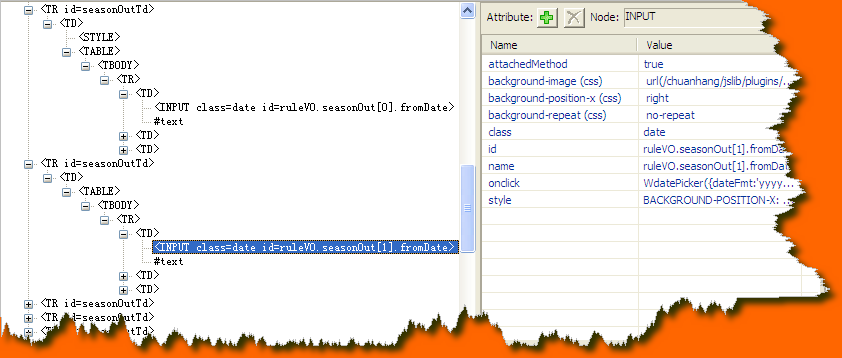
没办法,只能找出所有input,改变其id、name了
var inputs = newTr.$T("input");
for(var i=0;i<inputs.length;i++) {
inputs[i].id = inputs[i].id.replace(/[\d]/g, tr.index);
inputs[i].name = inputs[i].name.replace(/[\d]/g, tr.index);
}
完美了?测试看看效果
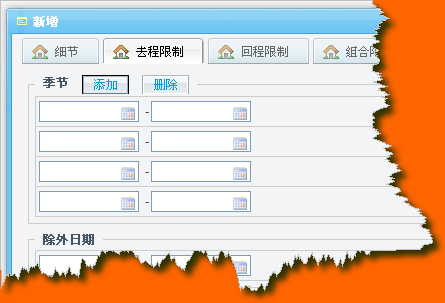
点击最下面的时间选择控件,结果发现,其定位到了第一行中的tr上去了,newTr中元素绑定的事件都绑定到原始tr了,看来cloneNode(true)会拷贝元素在内存中的所有状态
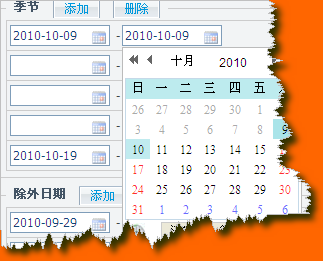
还是老老实实的通过dom创建元素吧:
<fieldset>
<legend>
<div style="float:left;">季节</div>
<div style="float:left;"> <input type="button" value="添加" onclick="addRow('seasonOutTr')"/></div>
<div style="float:left;"> <input type="button" value="删除" onclick="deleteRow('seasonOutTr')"/></div></legend>
<table width="100%" border="1" cellpadding="2" cellspacing="0" bordercolor="#cccccc"
style="border-collapse:collapse">
<s:iterator value="ruleVO.seasonOut" var="seasonOut" status="status">
<s:hidden name="ruleVO.seasonOut[%{#status.index}].id" />
<tr id="seasonOutTr">
<td>
<sky:datePicker name="ruleVO.seasonOut[${status.index}].fromDate,ruleVO.seasonOut[${status.index}].toDate" />
</td>
</tr>
</s:iterator>
</table>
</fieldset>
/* copy the tr(id) and append to the table, change the id、name of the new tr at the same time */
function addRow(id) {
var tr = $(id);
if(!tr.index) {
tr.index = 0;
}
tr.index++;
var newTr = tr.cloneNode(true);
//newTr.innerHTML = newTr.innerHTML.replace(/[\d]/g, tr.index);
//newTr.childNodes[0].innerHTML = newTr.childNodes[0].innerHTML.replace(/[\d]/g, tr.index);
var inputs = newTr.$T("input");
for(var i=0;i<inputs.length;i++) {
inputs[i].id = inputs[i].id.replace(/[\d]/g, tr.index);
inputs[i].name = inputs[i].name.replace(/[\d]/g, tr.index);
if(inputs[i].id.indexOf("from") != -1) {
inputs[i].onclick = function() {
WdatePicker({
dateFmt:'yyyy-MM-dd',
maxDate:'#F{$dp.$D(\'' + this.id.replace("from", "to") + '\')}'
});
};
} else {
inputs[i].onclick = function() {
WdatePicker({
dateFmt:'yyyy-MM-dd',
minDate:'#F{$dp.$D(\'' + this.id.replace("to", "from") + '\')}'
});
};
}
}
tr.parentElement.appendChild(newTr);
}
function addCombineFaresRow(id) {
var tr = $(id);
if(!tr.index) {
tr.index = 0;
}
tr.index++;
var newTr = tr.cloneNode(true);
var inputs = newTr.$T("input");
for(var i=0;i<inputs.length;i++) {
inputs[i].id = inputs[i].id.replace(/[\d]/g, tr.index);
inputs[i].name = inputs[i].name.replace(/[\d]/g, tr.index);
if(inputs[i].id.indexOf("seqId") != -1) {
inputs[i].value = tr.index+1;
}
}
tr.parentElement.appendChild(newTr);
}
/* delete the table's last tr */
function deleteRow(id) {
var tr = $(id);
if(!tr.index || tr.index==0) {
return;
}
var trs = tr.parentElement.childNodes;
tr.parentElement.removeChild(trs[trs.length-1]);
tr.index--;
}
function addTimeRow(id) {
var table = $(id);
var count = table.rows.length;
var tr = table.insertRow();
var td = tr.insertCell();
var names = [id + "["+count+"].fromDate", id + "["+count+"].toDate"];
td.appendChild(Sky.createEl({
tag: "input", name: names[0],
className: ["datePicker", "date"], onClick: function(){
WdatePicker({dateFmt:'yyyy-MM-dd',maxDate:'#F{$dp.$D(\''+names[1]+'\')}'});
}
}));
td.appendChild(Sky.createText(" - "));
td.appendChild(Sky.createEl({
tag: "input", name: names[1],
className: ["datePicker", "date"], onClick: function(){
WdatePicker({dateFmt:'yyyy-MM-dd',minDate:'#F{$dp.$D(\''+names[0]+'\')}'});
}
}));
}
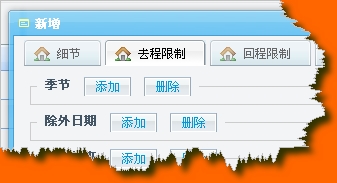
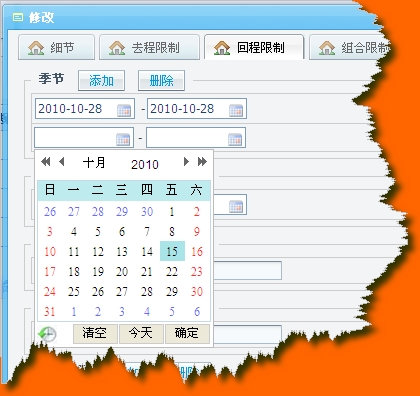
OK,搞定......