|
编写程序,该程序通过一个发射按钮可以发射一个弹球,该球遇到边界时会自动弹回,在移动固定次数后,该球停止运动。要求每次点击发射按钮都会发射一个弹球,如果点击多次,则多个弹球同时运动。该程序通过另一个结束可以结束程序的运行。参考界面如图所示。 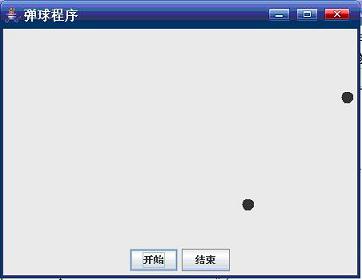 代码: package com.MoveBall;
import java.awt.*;
import java.awt.event.*;
import java.awt.geom.Ellipse2D;
import java.awt.geom.Rectangle2D;
import java.util.ArrayList;
import javax.swing.*;
public class MoveBall extends JFrame
{
//主程序
public static void main(String[] args){
EventQueue.invokeLater(new Runnable()
{
public void run(){
JFrame frame = new BounceFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
//小球弹跳
class BallRunable implements Runnable
{
public BallRunable(Ball aBall,Component aComponent){
ball = aBall;
component = aComponent;
}
public void run(){
try{
for(int i=1;i<=STEPTS;i++)
{
ball.move(component.getBounds());
component.repaint();
Thread.sleep(DELAY);
}
}
catch(InterruptedException e){
}
}
private Ball ball;
private Component component;
public static final int STEPTS = 1000;
public static final int DELAY = 5;
}
//定义窗体
class BounceFrame extends JFrame{
public BounceFrame(){
setSize(DEFAULT_WIDTH,DEFAULT_HEIGHT);
setTitle("MoveBall");
comp = new BallComponent();
add(comp,BorderLayout.CENTER);
JPanel buttonPanel = new JPanel();
addButton(buttonPanel,"开始",new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
addBall();
}
});
addButton(buttonPanel,"结束",new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
System.exit(0);
}
});
add(buttonPanel,BorderLayout.SOUTH);
}
public void addButton(Container c,String title,ActionListener listener)
{
JButton button = new JButton(title);
c.add(button);
button.addActionListener(listener);
}
public void addBall(){
Ball b = new Ball();
comp.add(b);
Runnable r = new BallRunable(b,comp);
Thread t = new Thread(r);
t.start();
}
private BallComponent comp;
public static final int DEFAULT_WIDTH = 450;
public static final int DEFAULT_HEIGHT = 350;
public static final int STEPS = 1000;
public static final int DELAY = 3;
}
//定义小球
class Ball
{
public void move(Rectangle2D bounds){
x += dx;
y += dy;
if(x < bounds.getMinX()){
x = bounds.getMinX();
dx = -dx;
}
if(x + XSIZE >= bounds.getMaxX())
{
x = bounds.getMaxX() - XSIZE;
dx = - dx;
}
if(y < bounds.getMinY())
{
y = bounds.getMinY();
dy = - dy;
}
if(y + YSIZE >= bounds.getMaxY())
{
y = bounds.getMaxY() - YSIZE;
dy = -dy;
}
}
public void move(Component component, Object bounds) {
// TODO Auto-generated method stub
}
public Ellipse2D getShape()
{
return new Ellipse2D.Double(x,y,XSIZE,YSIZE);
}
private static final int XSIZE = 15;
private static final int YSIZE = 15;
private double x = 0;
private double y = 0;
private double dx =1;
private double dy = 1;
}
//定义小球显示窗体
class BallComponent extends JPanel{
public void add(Ball b)
{
balls.add(b);
}
public void paintComponent(Graphics g)
{
super.paintComponent(g);
Graphics2D g2 = (Graphics2D) g;
for(Ball b : balls)
{
g2.fill(b.getShape());
}
}
private ArrayList<Ball> balls = new ArrayList<Ball>();
}
|
转载于:https://my.oschina.net/clear/blog/34988