一、网格UICollectionView最典型的例子是iBooks。其主要属性如下:
--- ViewController.swift ---
--- Main.storyboard ---
1,layout
该属性表示布局方式,有Flow、Custom两种布局方式。默认是Flow流式布局。
2,Accessories
是否显示页眉和页脚
3,各种尺寸属性
Cell Size:单元格尺寸
Header Size:页眉尺寸
Footer Size:页脚尺寸
Min Spacing:单元格之间间距
Section Insets:格分区上下左右空白区域大小。
二、流布局的简单样例
1,先创建一个ASimple View Application,删除默认的View Controller,拖入一个Collection View Controller到界面上,这时我们可以看到已经同时添加了Collection View和Collection View Cell控件。
2,勾选Collection View Controller属性面板里的Is Initial View Controller复选框,设置为启动视图控制器。
3,在Collection View Cell里拖入一个Image View和Label并摆放好位置和大小,用于显示图标和名称。
4,设置Image View的tag为1,Label的tag为2,Colletion View Cell的Identifier为DesignViewCell。
效果图如下:
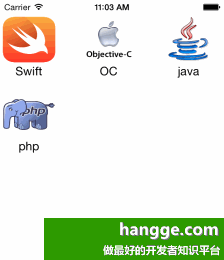
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
|
import
UIKit
class
ViewController: UICollectionViewController {
//课程名称和图片,每一门课程用字典来表示
let courses = [
[
"name"
:
"Swift"
,
"pic"
:
"swift.png"
],
[
"name"
:
"OC"
,
"pic"
:
"oc.jpg"
],
[
"name"
:
"java"
,
"pic"
:
"java.png"
],
[
"name"
:
"php"
,
"pic"
:
"php.jpeg"
]
]
override
func viewDidLoad() {
super
.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
// 已经在界面上设计了Cell并定义了identity,不需要注册CollectionViewCell
//self.collectionView.registerClass(UICollectionViewCell.self,
// forCellWithReuseIdentifier: "ViewCell")
//默认背景是黑色和label一致
self.collectionView?.backgroundColor = UIColor.whiteColor()
}
override
func didReceiveMemoryWarning() {
super
.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// CollectionView行数
override
func collectionView(collectionView: UICollectionView, numberOfItemsInSection section: Int)
-> Int {
return
courses.count;
}
// 获取单元格
override
func collectionView(collectionView: UICollectionView,
cellForItemAtIndexPath indexPath: NSIndexPath) -> UICollectionViewCell {
// storyboard里设计的单元格
let identify:
String
=
"DesignViewCell"
// 获取设计的单元格,不需要再动态添加界面元素
let cell = self.collectionView?.dequeueReusableCellWithReuseIdentifier(
identify, forIndexPath: indexPath)
as
UICollectionViewCell
// 从界面查找到控件元素并设置属性
(cell.contentView.viewWithTag(
1
)
as
! UIImageView).image =
UIImage(named: courses[indexPath.item][
"pic"
]!)
(cell.contentView.viewWithTag(
2
)
as
! UILabel).text = courses[indexPath.item][
"name"
]
return
cell
}
}
|
--- Main.storyboard ---
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
|
<?
xml
version
=
"1.0"
encoding
=
"UTF-8"
standalone
=
"no"
?>
<
document
type
=
"com.apple.InterfaceBuilder3.CocoaTouch.Storyboard.XIB"
version
=
"3.0"
toolsVersion
=
"6254"
systemVersion
=
"14B25"
targetRuntime
=
"iOS.CocoaTouch"
propertyAccessControl
=
"none"
useAutolayout
=
"YES"
useTraitCollections
=
"YES"
initialViewController
=
"fNw-sO-QDe"
>
<
dependencies
>
<
plugIn
identifier
=
"com.apple.InterfaceBuilder.IBCocoaTouchPlugin"
version
=
"6247"
/>
</
dependencies
>
<
scenes
>
<!--View Controller-->
<
scene
sceneID
=
"wxT-ij-Le6"
>
<
objects
>
<
collectionViewController
id
=
"fNw-sO-QDe"
customClass
=
"ViewController"
customModule
=
"SwiftInAction_008_015"
customModuleProvider
=
"target"
sceneMemberID
=
"viewController"
>
<
collectionView
key
=
"view"
clipsSubviews
=
"YES"
multipleTouchEnabled
=
"YES"
contentMode
=
"scaleToFill"
dataMode
=
"prototypes"
id
=
"pK1-nH-r5x"
>
<
rect
key
=
"frame"
x
=
"0.0"
y
=
"0.0"
width
=
"600"
height
=
"600"
/>
<
autoresizingMask
key
=
"autoresizingMask"
widthSizable
=
"YES"
heightSizable
=
"YES"
/>
<
color
key
=
"backgroundColor"
white
=
"0.66666666666666663"
alpha
=
"1"
colorSpace
=
"calibratedWhite"
/>
<
collectionViewFlowLayout
key
=
"collectionViewLayout"
minimumLineSpacing
=
"10"
minimumInteritemSpacing
=
"10"
id
=
"UDB-fy-TG6"
>
<
size
key
=
"itemSize"
width
=
"92"
height
=
"97"
/>
<
size
key
=
"headerReferenceSize"
width
=
"0.0"
height
=
"20"
/>
<
size
key
=
"footerReferenceSize"
width
=
"0.0"
height
=
"0.0"
/>
<
inset
key
=
"sectionInset"
minX
=
"0.0"
minY
=
"0.0"
maxX
=
"0.0"
maxY
=
"0.0"
/>
</
collectionViewFlowLayout
>
<
cells
>
<
collectionViewCell
opaque
=
"NO"
clipsSubviews
=
"YES"
multipleTouchEnabled
=
"YES"
contentMode
=
"center"
restorationIdentifier
=
"ViewCell"
reuseIdentifier
=
"DesignViewCell"
id
=
"FKz-79-V4r"
>
<
rect
key
=
"frame"
x
=
"0.0"
y
=
"0.0"
width
=
"50"
height
=
"50"
/>
<
autoresizingMask
key
=
"autoresizingMask"
/>
<
view
key
=
"contentView"
opaque
=
"NO"
clipsSubviews
=
"YES"
multipleTouchEnabled
=
"YES"
contentMode
=
"center"
>
<
rect
key
=
"frame"
x
=
"0.0"
y
=
"0.0"
width
=
"92"
height
=
"97"
/>
<
autoresizingMask
key
=
"autoresizingMask"
/>
<
subviews
>
<
imageView
userInteractionEnabled
=
"NO"
tag
=
"1"
contentMode
=
"scaleToFill"
horizontalHuggingPriority
=
"251"
verticalHuggingPriority
=
"251"
fixedFrame
=
"YES"
translatesAutoresizingMaskIntoConstraints
=
"NO"
id
=
"ZmJ-ad-pZs"
>
<
rect
key
=
"frame"
x
=
"4"
y
=
"4"
width
=
"75"
height
=
"65"
/>
</
imageView
>
<
label
opaque
=
"NO"
clipsSubviews
=
"YES"
userInteractionEnabled
=
"NO"
tag
=
"2"
contentMode
=
"left"
horizontalHuggingPriority
=
"251"
verticalHuggingPriority
=
"251"
fixedFrame
=
"YES"
text
=
"Label"
textAlignment
=
"center"
lineBreakMode
=
"tailTruncation"
baselineAdjustment
=
"alignBaselines"
adjustsFontSizeToFit
=
"NO"
translatesAutoresizingMaskIntoConstraints
=
"NO"
id
=
"U4J-Ys-QBF"
>
<
rect
key
=
"frame"
x
=
"4"
y
=
"71"
width
=
"75"
height
=
"21"
/>
<
fontDescription
key
=
"fontDescription"
type
=
"system"
pointSize
=
"17"
/>
<
color
key
=
"textColor"
cocoaTouchSystemColor
=
"darkTextColor"
/>
<
nil
key
=
"highlightedColor"
/>
</
label
>
</
subviews
>
<
color
key
=
"backgroundColor"
white
=
"0.0"
alpha
=
"0.0"
colorSpace
=
"calibratedWhite"
/>
</
view
>
</
collectionViewCell
>
</
cells
>
<
collectionReusableView
key
=
"sectionHeaderView"
opaque
=
"NO"
clipsSubviews
=
"YES"
multipleTouchEnabled
=
"YES"
contentMode
=
"center"
id
=
"rmh-sg-HAy"
>
<
rect
key
=
"frame"
x
=
"0.0"
y
=
"0.0"
width
=
"600"
height
=
"20"
/>
<
autoresizingMask
key
=
"autoresizingMask"
/>
</
collectionReusableView
>
<
connections
>
<
outlet
property
=
"dataSource"
destination
=
"fNw-sO-QDe"
id
=
"LWA-AL-0di"
/>
<
outlet
property
=
"delegate"
destination
=
"fNw-sO-QDe"
id
=
"RRE-KH-WU2"
/>
</
connections
>
</
collectionView
>
</
collectionViewController
>
<
placeholder
placeholderIdentifier
=
"IBFirstResponder"
id
=
"tTf-V2-wvH"
userLabel
=
"First Responder"
sceneMemberID
=
"firstResponder"
/>
</
objects
>
<
point
key
=
"canvasLocation"
x
=
"174"
y
=
"137"
/>
</
scene
>
</
scenes
>
</
document
>
|