1、 介绍
本教程文章是基于以下编写的:
- Spring Framework 4.3.9 RELEASE
- MyEclipse 2017 CI
在本文中使用Maven来声明Spring库,而不是下载Spring库,并以正常的方式来声明。
Maven是一个工具,可以帮你自动,高效地管理您的库,它已成为惯例,所有 Java 程序员必须知道。如果你不知道如何使用Maven,可以花10分钟就学会如何使用它:
如果你想下载Spring和声明库,您可以用传统的方式见附录在文件的结尾。
2、 Spring框架
下图显示了Spring框架的结构。
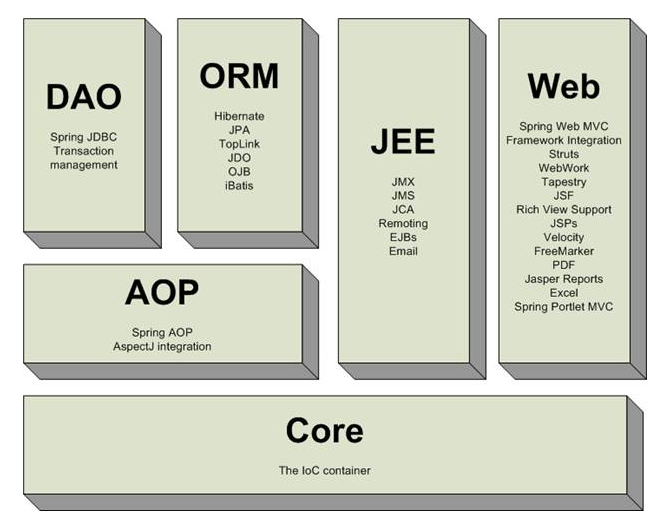
- IoC Container: 这是最重要的,也是最基础的, Spring的基础。它的作用是配置和Java对象的生命周期管理。这篇教程中我们将学习这一部分。
- DAO, ORM, AOP, WEB: 该模块可用于将工具或框架集成到了Spring。
2.1 反转控制和依赖注入
要了解这个问题,我们使用以下几类:
// MyInterface接口 public interface MyInterface { public void sayHello(); } // SpringInterfaceImpl实现了MyInterface接口 public class SpringInterfaceImpl implements MyInterface { public void sayHello() { System.out.println("Spring say Hello!"); } } // StrutsInterfaceImpl实现了MyInterface接口 public class StrutsInterfaceImpl implements MyInterface { public void sayHello() { System.out.println("Struts say Hello!"); } } // 添加一个MyInterface的服务类 public class MyInterfaceService { // 定义一个接口类型的引用变量 private MyInterface myInterface; // MyInterfaceService类的构造方法 // 初始化myInterface的引用对象实例 public MyInterfaceService() { this.myInterface = new StrutsInterfaceImpl(); } }
显而易见的是 MyInterfaceService 类管理创建 MyInterface 对象。
- 另外,在上述情况下,当 MyInterfaceService 对象从它的构造创建时,MyInterface对象也被创建了。 它是从StrutsInterfaceImpl 创建。
现在的问题是,您要创建一个MyInterfaceService对象,MyInterface对象也同时被创建,但它必须是SpringInterfaceImpl。
所以 MyInterfaceService 是控制“对象创建” MyInterface 的。我们为什么不创建 MyInterface 转让由第三方,
而是使用 MyInterfaceService ?因为我们有“反转控制”(IOC)的定义。
并且IoC容器将充当管理者角色,创建了MyInterfaceService 和 MyInterface 。
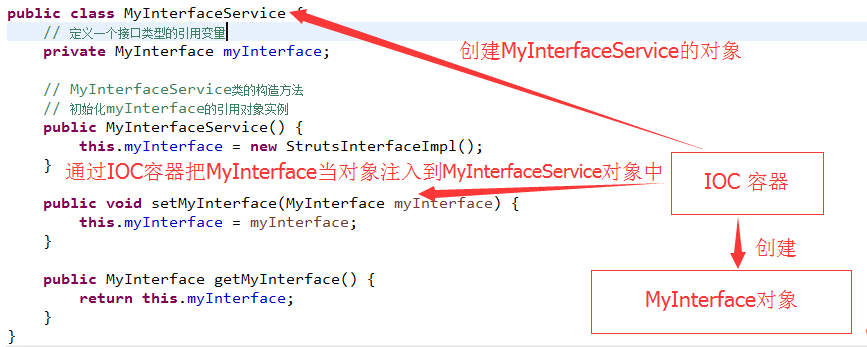
IoC容器创建 MyInterfaceService 对象,是通过 setter 方法传递 MyInterface 对象到MyInterfaceService。IoC容器做的是“依赖注入”到MyInterfaceService。这里的相关性是指对象之间的依赖关系: MyInterfaceService 和 MyInterface.
在这一点上,我们已经明确了什么是 IoC和DI。让我们举个例子来更好的理解。
3、 创建项目
- File/New/Other...
输入:
- Group Id: com.qf
- Artifact Id: HelloSpring
4、 声明Spring的基础库
这是 Spring的 HelloWorld 例子,所以我们只使用基本的Spring库(核心)。打开pom.xml文件来将使用的库声明:
- pom.xml 使用以下内容重新覆盖原上面的内容。
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.qf</groupId> <artifactId>HelloSpring</artifactId> <version>0.0.1-SNAPSHOT</version> <dependencies> <!-- https://mvnrepository.com/artifact/org.springframework/spring-core --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>4.3.9.RELEASE</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework/spring-context --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>4.3.9.RELEASE</version> </dependency> </dependencies> <build> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.8</source> <target>1.8</target> </configuration> </plugin> </plugins> </build> </project>
5、 工程代码
HelloWorld.java
package com.qf.spring; public interface HelloWorld { void sayHello(); }
SpringHelloWorld.java
package com.qf.spring.impl; import com.qf.spring.HelloWorld; public class SpringHelloWorld implements HelloWorld { @Override public void sayHello() { System.out.println("Spring Say Hello!!"); } }
StrutsHelloWorld.java
package com.qf.spring.impl; import com.qf.spring.HelloWorld; public class StrutsHelloWorld implements HelloWorld { @Override public void sayHello() { System.out.println("Struts Say Hello!!"); } }
HelloWorldService.java
package com.qf.spring; public class HelloWorldService { private HelloWorld helloWorld; public void setHelloWorld(HelloWorld helloWorld) { this.helloWorld = helloWorld; } public HelloWorld getHelloWorld() { return this.helloWorld; } }
HelloProgram.java
package com.qf.spring; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class HelloProgram { public static void main(String[] args) { ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml"); HelloWorldService helloWorldService = applicationContext.getBean(HelloWorldService.class); HelloWorld helloWorld = helloWorldService.getHelloWorld(); helloWorld.sayHello(); } }
applicationContext.xml
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="springHelloWorld" class="com.qf.spring.impl.SpringHelloWorld"></bean> <bean id="strutsHelloWorld" class="com.qf.spring.impl.StrutsHelloWorld"></bean> <bean id="helloWorldService" class="com.qf.spring.HelloWorldService"> <property name="helloWorld" ref="springHelloWorld"/> </bean> </beans>
6、 运行示例
运行 HelloProgram.java
运行 HelloProgram 类的结果如下:
打开 applicationContext.xml 文件并更改配置:
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="springHelloWorld" class="com.qf.spring.impl.SpringHelloWorld"></bean> <bean id="strutsHelloWorld" class="com.qf.spring.impl.StrutsHelloWorld"></bean> <bean id="helloWorldService" class="com.qf.spring.HelloWorldService"> <property name="helloWorld" ref="strutsHelloWorld"/> </bean> </beans>
重新运行 HelloProgram 类并得到以下结果。