在开始前我们在这先附一段最简单的代码


- (void)viewDidLoad { [super viewDidLoad]; UICollectionViewFlowLayout *layout = [[UICollectionViewFlowLayout alloc] init]; UICollectionView *colView = [[UICollectionView alloc] initWithFrame:self.view.bounds collectionViewLayout:layout]; colView.backgroundColor = [UIColor grayColor]; [colView registerClass:[UICollectionViewCell class] forCellWithReuseIdentifier:@"myCell"]; colView.delegate = self; colView.dataSource = self; [self.view addSubview:colView]; } - (NSArray *)loadData { NSArray *arr = [NSArray arrayWithObjects:@"cell", @"cell2", @"cell3", @"cell4", @"cell5", @"cell6", @"cell7",nil]; return arr; } #pragma mark - UICollectionViewDataSource - (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section { return [[self loadData] count]; } - (NSInteger)numberOfSectionsInCollectionView:(UICollectionView *)collectionView { return 1; } - (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath { static NSString *cellID = @"myCell"; UICollectionViewCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:cellID forIndexPath:indexPath]; cell.backgroundColor = [UIColor redColor]; return cell; }
当你运行时将看到,它与UITableView的布局区别。
一、介绍
UICollectionView类负责管理数据的有序集合以及以自定义布局的模式来呈现这些数据,它提供了一些常用的表格(table)功能,此外还增加了许多单栏布局。UICollectionView支持可以用于实现多列网格、 平铺的布局、 圆形的布局和更多的自定义布局,甚至你可以动态地改变它的布局。
除了将它嵌入在您的用户界面,您可以使用 UICollectionView 对象的方法以确保item的可视化表示匹配您的数据源对象中的顺序。因此,每当您添加、 删除或重新排列您的集合中的数据,您可以使用此类的方法来插入、 删除和重新排列相应cell的状态。您还使用集合视图对象来管理所选的item。
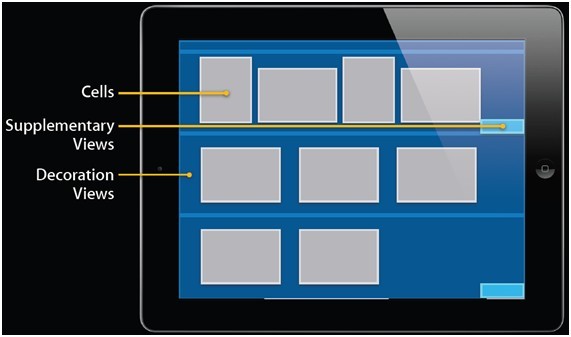
2.初始化,在初始化之前我们需要常见布局实例
UICollectionViewFlowLayout *layout = [[UICollectionViewFlowLayout alloc] init];
UICollectionView *colView = [[UICollectionView alloc] initWithFrame:self.view.bounds collectionViewLayout:layout];
3.注册UICollectionView使用的cell类型。
【必须先注册,否则会报异常
*** Terminating app due to uncaught exception 'NSInternalInconsistencyException', reason: 'could not dequeue a view of kind: UICollectionElementKindCell with identifier myCell - must register a nib or a class for the identifier or connect a prototype cell in a storyboard'
】
[colView registerClass:[UICollectionViewCell class] forCellWithReuseIdentifier:@"myCell"];
4.设置代理
colView.delegate = self; colView.dataSource = self;
5.实现协议
UICollectionViewDataSource
//配置UICollectionView的每个section的item数
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section
{
return [[self loadData] count];
}
//配置section数
- (NSInteger)numberOfSectionsInCollectionView:(UICollectionView *)collectionView
{
return 1;
}
//呈现数据
- (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *cellID = @"myCell";
UICollectionViewCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:cellID forIndexPath:indexPath];
cell.backgroundColor = [UIColor redColor];
return cell;
}
UICollectionViewDelegateFlowLayout
//配置每个item的size
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout *)collectionViewLayout sizeForItemAtIndexPath:(NSIndexPath *)indexPath
{
return CGSizeMake(100, 60);
}
//配置item的边距
- (UIEdgeInsets)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout *)collectionViewLayout insetForSectionAtIndex:(NSInteger)section
{
return UIEdgeInsetsMake(20, 20, 0, 20);
}
效果
我们明晰地可以看到,它已经进行自动布局,为了更能说明问题,我们现在将
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout *)collectionViewLayout sizeForItemAtIndexPath:(NSIndexPath *)indexPath
{
return CGSizeMake(200, 60);
}
return CGSizeMake(60, 60);
UICollectionViewDelegate
//点击item时触发
- (void)collectionView:(UICollectionView *)collectionView didSelectItemAtIndexPath:(NSIndexPath *)indexPath
{
NSLog(@"您点击了item:%@", [[self loadData] objectAtIndex:indexPath.row]);
[collectionView cellForItemAtIndexPath:indexPath].backgroundColor = [UIColor redColor];
}
//当前ite是否可以点击
- (BOOL) collectionView:(UICollectionView *)collectionView shouldSelectItemAtIndexPath:(nonnull NSIndexPath *)indexPath
{
if (indexPath.row % 2)
{
return YES;
}
return NO;
}
我们也可以通过设置UICollectionViewCell的selectedBackgroundView属性来改变cell选中时的背景视图。
我们还可以设置哪些cell点击时不高亮,点击时cell的样式和点击后cell的样式
- (BOOL)collectionView:(UICollectionView *)collectionView shouldHighlightItemAtIndexPath:(NSIndexPath *)indexPath
{
return YES;
}
- (void)collectionView:(UICollectionView *)collectionView didHighlightItemAtIndexPath:(NSIndexPath *)indexPath
{
UICollectionViewCell *cell = [collectionView cellForItemAtIndexPath:indexPath];
cell.backgroundColor = [UIColor redColor];
}
- (void)collectionView:(UICollectionView *)collectionView didUnhighlightItemAtIndexPath:(NSIndexPath *)indexPath
{
UICollectionViewCell *cell = [collectionView cellForItemAtIndexPath:indexPath];
cell.backgroundColor = [UIColor grayColor];
}