這篇文章針對 TBCommandField 類別做進一步的擴展,我們希望在刪除訊息中可以加入指定的欄位值,讓使用者明確知道刪除的資料,例如刪除某個產品資料時,可以顯示這個產品的名稱在提示訊息中。
針對這個需求,在 TBCommandField 類別新增一個 DataField 屬性,設定刪除提示訊息中顯示欄位值的資料欄位名稱。因為要取得繫結的欄位資料,所以在 SetDeleteButton 私有方法中,設定刪除鈕 DataBinding 事件處理函式為 OnDataBindField,當 GridView 做 DataBind 時,就會引發子控制項的 DataBinding 事件,此時就會執行 OnDataBindField 方法,這時可以取得繫結的資料的 DataRowView,然後就可以從 DataRowView 中取得 DateField 的欄位值。
實作程式碼如下
1
Imports
System
2 Imports System.Collections.Generic
3 Imports System.ComponentModel
4 Imports System.Text
5 Imports System.Web
6 Imports System.Web.UI
7 Imports System.Web.UI.WebControls
8
9
10 Public Class TBCommandField
11 Inherits CommandField
12
13 Private FDeleteConfirmMessage As String = String .Empty
14 Private FDataField As String = String .Empty
15
16 ' '' <summary>
17 ' '' 刪除提示訊息。
18 ' '' </summary>
19 Public Property DeleteConfirmMessage() As String
20 Get
21 Return FDeleteConfirmMessage
22 End Get
23 Set ( ByVal value As String )
24 FDeleteConfirmMessage = value
25 End Set
26 End Property
27
28 ' '' <summary>
29 ' '' 刪除提示訊息顯示欄位值的資料欄位名稱。
30 ' '' </summary>
31 Public Property DataField() As String
32 Get
33 Return FDataField
34 End Get
35 Set ( ByVal value As String )
36 FDataField = value
37 End Set
38 End Property
39
40 ' '' <summary>
41 ' '' 初始化儲存格。
42 ' '' </summary>
43 ' '' <param name="cell">要初始化的儲存格。</param>
44 ' '' <param name="cellType">儲存格類型。</param>
45 ' '' <param name="rowState">儲存格狀態。</param>
46 ' '' <param name="rowIndex">資料列之以零起始的索引。</param>
47 ' '' <remarks></remarks>
48 Public Overrides Sub InitializeCell( ByVal cell As DataControlFieldCell, ByVal cellType As DataControlCellType,
ByVal rowState As DataControlRowState, ByVal rowIndex As Integer )
49 MyBase .InitializeCell(cell, cellType, rowState, rowIndex)
50 If Me .ShowDeleteButton AndAlso Me .Visible AndAlso Me .DeleteConfirmMessage <> String .Empty Then
51 SetDeleteButton(cell)
52 End If
53 End Sub
54
55 ' '' <summary>
56 ' '' 建立新的 TBCommandField 物件。
57 ' '' </summary>
58 Protected Overrides Function CreateField() As DataControlField
59 Return New TBCommandField()
60 End Function
61
62 ' '' <summary>
63 ' '' 將目前 TBCommandField 物件的屬性複製到指定之 DataControlField 物件。
64 ' '' </summary>
65 ' '' <param name="newField">目的 DataControlField 物件。</param>
66 Protected Overrides Sub CopyProperties( ByVal NewField As DataControlField)
67 Dim oNewField As TBCommandField
68
69 oNewField = DirectCast (NewField, TBCommandField)
70 oNewField.DeleteConfirmMessage = Me .DeleteConfirmMessage
71 oNewField.DataField = Me .DataField
72 MyBase .CopyProperties(NewField)
73 End Sub
74
75 ' '' <summary>
76 ' '' 設定刪除鈕。
77 ' '' </summary>
78 ' '' <param name="Cell">儲存格。</param>
79 Private Sub SetDeleteButton( ByVal Cell As DataControlFieldCell)
80 Dim oControl As Control
81
82 For Each oControl In Cell.Controls
83 If TypeOf (oControl) Is IButtonControl Then
84 If DirectCast (oControl, IButtonControl).CommandName = " Delete " Then
85 ' 設定刪除鈕的 DataBinding 事件處理函式
86 AddHandler oControl.DataBinding, New EventHandler( AddressOf Me .OnDataBindField)
87 Exit Sub
88 End If
89 End If
90 Next
91 End Sub
92
93 ' '' <summary>
94 ' '' 將欄位值繫結至 TBCommandField 物件。
95 ' '' </summary>
96 Protected Overridable Sub OnDataBindField( ByVal sender As Object , ByVal e As EventArgs)
97 Dim oControl As Control
98 Dim oDataItem As Object
99 Dim oDataRowView As Data.DataRowView
100 Dim sScript As String
101 Dim sMessage As String
102
103 oControl = DirectCast (sender, Control)
104
105 If Me .DataField <> String .Empty Then
106 ' 處理訊息中的欄位值
107 oDataItem = DataBinder.GetDataItem(oControl.NamingContainer)
108 oDataRowView = CType (oDataItem, Data.DataRowView)
109 sMessage = String .Format( Me .DeleteConfirmMessage, oDataRowView.Item( Me .DataField).ToString())
110 Else
111 sMessage = Me .DeleteConfirmMessage
112 End If
113
114 sMessage = Strings.Replace(sMessage, " ' " , " \' " ) ' 處理單引號
115 sScript = " if (confirm(' " & sMessage & " ')==false) {return false;} "
116 DirectCast (oControl, WebControl).Attributes( " onclick " ) = sScript
117 End Sub
118
119 End Class
120
2 Imports System.Collections.Generic
3 Imports System.ComponentModel
4 Imports System.Text
5 Imports System.Web
6 Imports System.Web.UI
7 Imports System.Web.UI.WebControls
8
9
10 Public Class TBCommandField
11 Inherits CommandField
12
13 Private FDeleteConfirmMessage As String = String .Empty
14 Private FDataField As String = String .Empty
15
16 ' '' <summary>
17 ' '' 刪除提示訊息。
18 ' '' </summary>
19 Public Property DeleteConfirmMessage() As String
20 Get
21 Return FDeleteConfirmMessage
22 End Get
23 Set ( ByVal value As String )
24 FDeleteConfirmMessage = value
25 End Set
26 End Property
27
28 ' '' <summary>
29 ' '' 刪除提示訊息顯示欄位值的資料欄位名稱。
30 ' '' </summary>
31 Public Property DataField() As String
32 Get
33 Return FDataField
34 End Get
35 Set ( ByVal value As String )
36 FDataField = value
37 End Set
38 End Property
39
40 ' '' <summary>
41 ' '' 初始化儲存格。
42 ' '' </summary>
43 ' '' <param name="cell">要初始化的儲存格。</param>
44 ' '' <param name="cellType">儲存格類型。</param>
45 ' '' <param name="rowState">儲存格狀態。</param>
46 ' '' <param name="rowIndex">資料列之以零起始的索引。</param>
47 ' '' <remarks></remarks>
48 Public Overrides Sub InitializeCell( ByVal cell As DataControlFieldCell, ByVal cellType As DataControlCellType,
ByVal rowState As DataControlRowState, ByVal rowIndex As Integer )
49 MyBase .InitializeCell(cell, cellType, rowState, rowIndex)
50 If Me .ShowDeleteButton AndAlso Me .Visible AndAlso Me .DeleteConfirmMessage <> String .Empty Then
51 SetDeleteButton(cell)
52 End If
53 End Sub
54
55 ' '' <summary>
56 ' '' 建立新的 TBCommandField 物件。
57 ' '' </summary>
58 Protected Overrides Function CreateField() As DataControlField
59 Return New TBCommandField()
60 End Function
61
62 ' '' <summary>
63 ' '' 將目前 TBCommandField 物件的屬性複製到指定之 DataControlField 物件。
64 ' '' </summary>
65 ' '' <param name="newField">目的 DataControlField 物件。</param>
66 Protected Overrides Sub CopyProperties( ByVal NewField As DataControlField)
67 Dim oNewField As TBCommandField
68
69 oNewField = DirectCast (NewField, TBCommandField)
70 oNewField.DeleteConfirmMessage = Me .DeleteConfirmMessage
71 oNewField.DataField = Me .DataField
72 MyBase .CopyProperties(NewField)
73 End Sub
74
75 ' '' <summary>
76 ' '' 設定刪除鈕。
77 ' '' </summary>
78 ' '' <param name="Cell">儲存格。</param>
79 Private Sub SetDeleteButton( ByVal Cell As DataControlFieldCell)
80 Dim oControl As Control
81
82 For Each oControl In Cell.Controls
83 If TypeOf (oControl) Is IButtonControl Then
84 If DirectCast (oControl, IButtonControl).CommandName = " Delete " Then
85 ' 設定刪除鈕的 DataBinding 事件處理函式
86 AddHandler oControl.DataBinding, New EventHandler( AddressOf Me .OnDataBindField)
87 Exit Sub
88 End If
89 End If
90 Next
91 End Sub
92
93 ' '' <summary>
94 ' '' 將欄位值繫結至 TBCommandField 物件。
95 ' '' </summary>
96 Protected Overridable Sub OnDataBindField( ByVal sender As Object , ByVal e As EventArgs)
97 Dim oControl As Control
98 Dim oDataItem As Object
99 Dim oDataRowView As Data.DataRowView
100 Dim sScript As String
101 Dim sMessage As String
102
103 oControl = DirectCast (sender, Control)
104
105 If Me .DataField <> String .Empty Then
106 ' 處理訊息中的欄位值
107 oDataItem = DataBinder.GetDataItem(oControl.NamingContainer)
108 oDataRowView = CType (oDataItem, Data.DataRowView)
109 sMessage = String .Format( Me .DeleteConfirmMessage, oDataRowView.Item( Me .DataField).ToString())
110 Else
111 sMessage = Me .DeleteConfirmMessage
112 End If
113
114 sMessage = Strings.Replace(sMessage, " ' " , " \' " ) ' 處理單引號
115 sScript = " if (confirm(' " & sMessage & " ')==false) {return false;} "
116 DirectCast (oControl, WebControl).Attributes( " onclick " ) = sScript
117 End Sub
118
119 End Class
120
使用時在 aspx 程式碼中設定其 DeleteConfirmMessage 及 DateField 屬性就在刪除提示訊息加入欄位值。其中 DeleteConfirmMessage="確定刪除 {0} 這筆資料嗎?" 中的 {0} 就是 ProductName 欄位值顯示的位置。
<
bee:TBCommandField
ShowDeleteButton
="True"
ShowEditButton
="True"
DataField
="ProductName"
DeleteConfirmMessage
="確定刪除 {0} 這筆資料嗎?"
ButtonType
="Button"
/>
執行結果如下
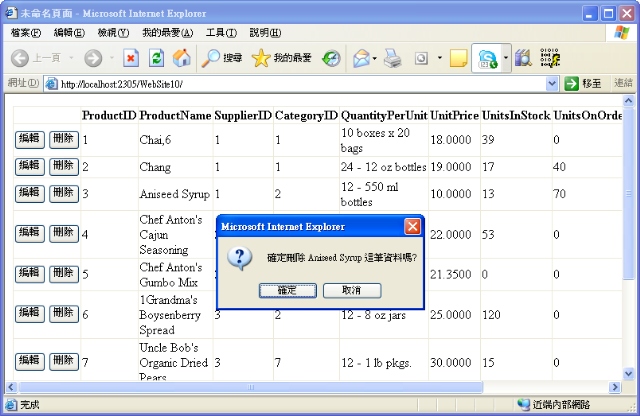