str.count 统计
字符串方法 str.count(),Python 官方文档描述如下:
help(str.count)
Help on method_descriptor:
count(...)
S.count(sub[, start[, end]]) -> int
Return the number of non-overlapping occurrences of substring sub in
string S[start:end]. Optional arguments start and end are
interpreted as in slice notation.
返回回子字符串 sub 在 [start, end] 范围内非重叠出现的次数。可选参数 start 与 end 会被解读为切片表示法。
只给定 sub 一个参数的话,于是从第一个字符开始搜索到字符串结束;如果,随后给定了一个可选参数的话,那么它是 start,于是从 start 开始,搜索到字符串结束;如果 start 之后还有参数的话,那么它是 end;于是从 start 开始,搜索到 end - 1 结束(即不包含索引值为 end 的那个字符)。
'python'.count('0')
0
'pyyython'.count('yy')
1
'pythonpythonn'.count('n',5)
3
'pythonpythonn'.count('n',5,7)
1
str.replace 替换
字符串方法 str.replace(),Python 官方文档描述如下:
help(str.replace)
Help on method_descriptor:
replace(self, old, new, count=-1, /)
Return a copy with all occurrences of substring old replaced by new.
count
Maximum number of occurrences to replace.
-1 (the default value) means replace all occurrences.
If the optional argument count is given, only the first count occurrences are
replaced.
返回字符串的副本,其中出现的所有子字符串 old 都将被替换为 new。如果给出了可选参数 count,则只替换前 count 次出现。
'python python'.replace('p','C')
'Cython Cython'
'python python'.replace('py','Cpy',1)
'Cpython python'
如果 old 为空字符串,则在每个字符之间(包括前后)插入 new:
'python python'.replace('','C')
'CpCyCtChCoCnC CpCyCtChCoCnC'
如果 new 为空字符串,则相当于去除了 old:
'python python'.replace('p','')
'ython ython'
str.expandtabs 替换制表符
字符串方法 str.expandtabs(),Python 官方文档描述如下:
help(str.expandtabs)
Help on method_descriptor:
expandtabs(self, /, tabsize=8)
Return a copy where all tab characters are expanded using spaces.
If tabsize is not given, a tab size of 8 characters is assumed.
返回字符串的副本,其中所有的制表符会由一个或多个空格替换,具体取决于当前列位置和给定的制表符宽度。每 tabsize 个字符设为一个制表位(默认值 8 时设定的制表位在列 0, 8, 16 依次类推)。
要展开字符串,当前列将被设为零并逐一检查字符串中的每个字符。如果字符为制表符 (\t),则会在结果中插入一个或多个空格符,直到当前列等于下一个制表位。(制表符本身不会被复制。)
如果字符为换行符 (\n) 或回车符 (\r),它会被复制并将当前列重设为零。任何其他字符会被不加修改地复制并将当前列加一,不论该字符在被打印时会如何显示。
'01\t012\t0123\t01234'.expandtabs()
'01 012 0123 01234'
'01\t012\t0123\t01234'.expandtabs(4)
'01 012 0123 01234'
'\n\t01\r2\t0123\t01234'.expandtabs(4)
'\n 01\r2 0123 01234'
str.split 拆分
字符串方法 str.split(),Python 官方文档描述如下:
help(str.split)
Help on method_descriptor:
split(self, /, sep=None, maxsplit=-1)
Return a list of the words in the string, using sep as the delimiter string.
sep
The delimiter according which to split the string.
None (the default value) means split according to any whitespace,
and discard empty strings from the result.
maxsplit
Maximum number of splits to do.
-1 (the default value) means no limit.
返回一个由字符串内单词组成的列表,使用 sep 作为分隔字符串。如果给出了 sep,则连续的分隔符不会被组合在一起而是被视为分隔空字符串;如果给出了 maxsplit,则最多进行 maxsplit 次拆分(因此,列表最多会有 maxsplit+1 个元素)。如果 maxsplit 未指定或为 -1,则不限制拆分次数(进行所有可能的拆分)。
'a.bc'.split('.')
['a', 'bc']
'a.b.c'.split('.',maxsplit=1)
['a', 'b.c']
'a.b..c'.split('.')
['a', 'b', '', 'c']
'a.b..c'.split('..')
['a.b', 'c']
''.split('.')
['']
如果 sep 未指定或为 None,则会应用另一种拆分算法:连续的空格会被视为单个分隔符,如果字符串包含前缀或后缀空格,其结果将不包含开头或末尾的空字符串。因此,使用 None 拆分空字符串或仅包含空格的字符串将返回 []。
'a b c '.split()
['a', 'b', 'c']
' \n '.split()
[]
''.split()
[]
str.rsplit 拆分
字符串方法 str.rsplit(),Python 官方文档描述如下:
help(str.rsplit)
Help on method_descriptor:
rsplit(self, /, sep=None, maxsplit=-1)
Return a list of the words in the string, using sep as the delimiter string.
sep
The delimiter according which to split the string.
None (the default value) means split according to any whitespace,
and discard empty strings from the result.
maxsplit
Maximum number of splits to do.
-1 (the default value) means no limit.
Splits are done starting at the end of the string and working to the front.
返回一个由字符串内单词组成的列表,使用 sep 作为分隔字符串。如果给出了 maxsplit,则最多进行 maxsplit 次拆分,从 最右边开始。如果 sep 未指定或为 None,任何空白字符串都会被作为分隔符。除了从右边开始拆分,rsplit() 的其他行为都类似于 split()。
'p\nyth on '.rsplit()
['p', 'yth', 'on']
'p\nyth on'.rsplit('y')
['p\n', 'th on']
'p\nyth on'.rsplit(maxsplit=1)
['p\nyth', 'on']
'p\nyth on '.rsplit(maxsplit=2)
['p', 'yth', 'on']
多个分隔符在一起,则会解读为拆分空字符串:
'\n\np\nyth on'.rsplit('\n')
['', '', 'p', 'yth on']
str.partition 拆分
字符串方法 str.partition(),Python 官方文档描述如下:
help(str.partition)
Help on method_descriptor:
partition(self, sep, /)
Partition the string into three parts using the given separator.
This will search for the separator in the string. If the separator is found,
returns a 3-tuple containing the part before the separator, the separator
itself, and the part after it.
If the separator is not found, returns a 3-tuple containing the original string
and two empty strings.
在 sep 首次出现的位置拆分字符串,返回一个 3 元组,其中包含分隔符之前的部分、分隔符本身,以及分隔符之后的部分。如果分隔符未找到,则返回的 3 元组中包含字符本身以及两个空字符串。
'python'.partition('m')
('python', '', '')
'python'.partition('th')
('py', 'th', 'on')
'python'.partition('ht')
('python', '', '')
str.rpartition 拆分
字符串方法 str.rpartition(),Python 官方文档描述如下:
help(str.rpartition)
Help on method_descriptor:
rpartition(self, sep, /)
Partition the string into three parts using the given separator.
This will search for the separator in the string, starting at the end. If
the separator is found, returns a 3-tuple containing the part before the
separator, the separator itself, and the part after it.
If the separator is not found, returns a 3-tuple containing two empty strings
and the original string.
在 sep 最后一次出现的位置拆分字符串,返回一个 3 元组,其中包含分隔符之前的部分、分隔符本身,以及分隔符之后的部分。如果分隔符未找到,则返回的 3 元组中包含两个空字符串以及字符串本身。
'python'.rpartition('n')
('pytho', 'n', '')
'pythhhon'.rpartition('hh')
('pyth', 'hh', 'on')
分隔符未找到,则返回的 3 元组中 字符串本身 排在最后:
'python'.rpartition('ht')
('', '', 'python')
str.splitlines 按行拆分
字符串方法 str.splitlines(),Python 官方文档描述如下:
help(str.splitlines)
Help on method_descriptor:
splitlines(self, /, keepends=False)
Return a list of the lines in the string, breaking at line boundaries.
Line breaks are not included in the resulting list unless keepends is given and
true.
返回由原字符串中各行组成的列表,在行边界的位置拆分。结果列表中不包含行边界,除非给出了 keepends 且为真值。
此方法会以下列行边界进行拆分。特别地,行边界是 universal newlines 的一个超集。
\n
换行\r
回车\r\n
回车 + 换行\v
或\x0b
行制表符\f
或\x0c
换表单\x1c
文件分隔符\x1d
组分隔符\x1e
记录分隔符\x85
下一行\u2028
行分隔符\u2029
段分隔符
'ab c\n\nde fg\rkl\r\n'.splitlines()
['ab c', '', 'de fg', 'kl']
'ab c\n\nde fg\rkl\r\n'.splitlines(keepends=True)
['ab c\n', '\n', 'de fg\r', 'kl\r\n']
分隔空字符串此方法将返回一个空列表;末尾的换行不会令结果中增加额外的空字符串:
''.splitlines()
[]
"One line\nTwo lines\n".splitlines()
['One line', 'Two lines']
'One line\nTwo lines\n'.split('\n')
['One line', 'Two lines', '']
转自 《Python基础知识点自测手册》 (https://xue.cn/hub/app/books/64?from=wh01)。
强烈推荐在 XUE.cn 上自学编程:不需要做任何配置,即可使用手机、平板、电脑,随时随地写代码、修改书中代码、运行验证代码。
注册请使用我的邀请码:https://xue.cn?inviter=xxmbz 你和我都能获得 2 天学习时长,谢谢!
end
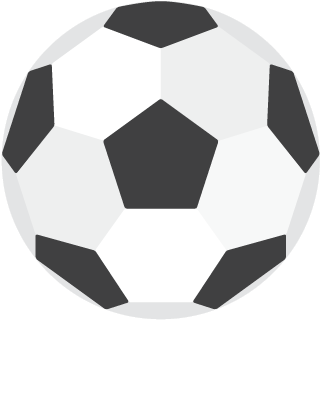