随着分布式技术的普及和海量数据的增长,io的能力越来越重要,java提供的io模块提供了足够的扩展性来适应。
我是李福春,我在准备面试,今天的问题是:
java中的io有哪几种?
java中的io分3类:
1,BIO ,即同步阻塞IO,对应java.io包提供的工具;基于流模型,虽然直观,代码实现也简单,但是扩展性差,消耗资源大,容易成为系统的瓶颈;
2,NIO,同步非阻塞io,对应java.nio包提供的工具,基于io多路复用;
核心类: Channel ,Selector , Buffer , Charset
selector是io多路复用的基础,实现了一个线程高效管理多个客户端连接,通过事件监听处理感兴趣的事件。
3,AIO,即异步非阻塞io, 基于事件和回调
io的类层级
java各种IO的例子
java.io客户端连接服务端例子
package org.example.mianshi.io;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
/**
* 说明:传统流式io 客户端连接服务端例子
* @author carter
* 创建时间: 2020年03月25日 9:58 下午
**/
public class JavaIOApp {
public static void main(String[] args) {
final Server server = new Server();
new Thread(server).start();
try (
Socket socket = new Socket(InetAddress.getLocalHost(), server.getPort());
) {
final BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
bufferedReader.lines().forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
public static class Server implements Runnable {
private ServerSocket serverSocket;
public int getPort() {
return serverSocket.getLocalPort();
}
@Override
public void run() {
try (ServerSocket serverSocket = new ServerSocket(0);) {
this.serverSocket = serverSocket;
while (true) {
final Socket socket = serverSocket.accept();
new RequestHandler(socket).start();
}
} catch (IOException e) {
e.printStackTrace();
}
}
private class RequestHandler extends Thread {
private Socket socket;
public RequestHandler(Socket socket) {
this.socket = socket;
}
@Override
public void run() {
try (
final PrintWriter printWriter = new PrintWriter(socket.getOutputStream());
) {
printWriter.write("hello world");
printWriter.flush();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
使用连接池优化
package org.example.mianshi.io;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
/**
* 说明:传统流式io 客户端连接服务端例子
* @author carter
* 创建时间: 2020年03月25日 9:58 下午
**/
public class ThreadPoolJavaIOApp {
public static void main(String[] args) {
final Server server = new Server();
new Thread(server).start();
try (
Socket socket = new Socket(InetAddress.getLocalHost(), server.getPort());
) {
final BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
bufferedReader.lines().forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
public static class Server implements Runnable {
private ExecutorService threadPool = Executors.newFixedThreadPool(4);
private ServerSocket serverSocket;
public int getPort() {
return serverSocket.getLocalPort();
}
@Override
public void run() {
try (ServerSocket serverSocket = new ServerSocket(0);) {
this.serverSocket = serverSocket;
while (true) {
final Socket socket = serverSocket.accept();
threadPool.submit(new RequestHandler(socket));
}
} catch (IOException e) {
e.printStackTrace();
}finally {
threadPool.shutdown();
}
}
private class RequestHandler implements Runnable {
private Socket socket;
public RequestHandler(Socket socket) {
this.socket = socket;
}
@Override
public void run() {
try (
final PrintWriter printWriter = new PrintWriter(socket.getOutputStream());
) {
printWriter.write("hello world");
printWriter.flush();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
java.nio例子
package org.example.mianshi.io;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.Socket;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.nio.charset.Charset;
/**
* 说明:nio的客户端连接服务端例子
* @author carter
* 创建时间: 2020年03月25日 10:32 下午
**/
public class JavaNioApp {
public static void main(String[] args) {
new Server().start();
try (
Socket socket = new Socket(InetAddress.getLocalHost(), 8888);
) {
final BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
bufferedReader.lines().forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
public static class Server extends Thread {
@Override
public void run() {
try {
ServerSocketChannel serverSocketChannel = ServerSocketChannel.open();
serverSocketChannel.bind(new InetSocketAddress(InetAddress.getLocalHost(), 8888));
serverSocketChannel.configureBlocking(false);
final Selector selector = Selector.open();
serverSocketChannel.register(selector, SelectionKey.OP_ACCEPT);
while (true) {
selector.select();
selector.selectedKeys().forEach(selectionKey -> {
sayHelloWorld((ServerSocketChannel) selectionKey.channel());
});
}
} catch (IOException e) {
e.printStackTrace();
}
}
private void sayHelloWorld(ServerSocketChannel channel) {
try (SocketChannel socketChannel = channel.accept()) {
socketChannel.write(Charset.defaultCharset().encode("hello world nio"));
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
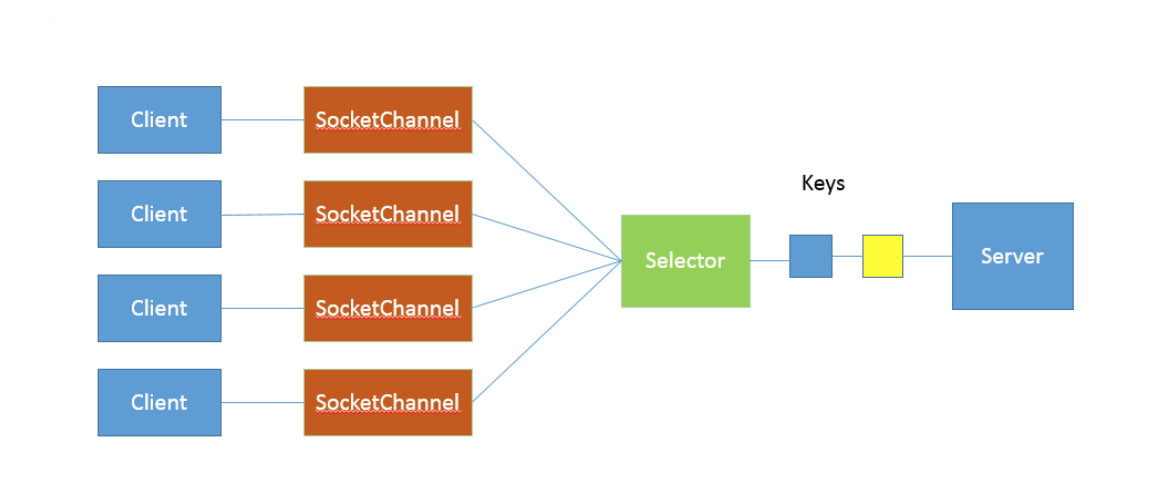
java.nio2例子
package org.example.mianshi.io;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.Socket;
import java.nio.channels.AsynchronousServerSocketChannel;
import java.nio.channels.AsynchronousSocketChannel;
import java.nio.channels.CompletionHandler;
import java.nio.charset.Charset;
/**
* 说明:TODO
* @author carter
* 创建时间: 2020年03月25日 10:54 下午
**/
public class JavaNio2App {
public static void main(String[] args) {
new Server().start();
try (
Socket socket = new Socket(InetAddress.getLocalHost(), 9999);
) {
final BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
bufferedReader.lines().forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
public static class Server extends Thread {
@Override
public void run() {
try {
AsynchronousServerSocketChannel serverSocketChannel = AsynchronousServerSocketChannel.open()
.bind(new InetSocketAddress(InetAddress.getLocalHost(), 9999));
serverSocketChannel.accept(serverSocketChannel, new CompletionHandler() {
@Override
public void completed(AsynchronousSocketChannel socketChannel,
AsynchronousServerSocketChannel serverSocketChannel1) {
// serverSocketChannel1.accept(socketChannel, this);
socketChannel.write(Charset.defaultCharset().encode("hello world nio2 "));
try {
socketChannel.close();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void failed(Throwable exc, AsynchronousServerSocketChannel attachment) {
exc.printStackTrace();
}
});
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
本例子暂时无法运行。只为展示过程;
小结
本篇主要介绍了java提供的3中io,即 BIO,NIO,AIO ; 并提供了一些示例代码辅助理解。
原创不易,转载请注明出处。