1 属性操作
1.1 HTML attribute -> DOM property
1/* 把HTML的属性转化为DOM的属性 */
2---
3<div>
4 <label for="userName">用户名:albel>
5 <input id="userName" type="text" class="u-txt">
6div>
7---
8//input--html
9id -->"userName"
10type -->"text"
11class-->"u-text"
12//input--DOM
13id -->"userName"
14type -->"text"
15className -->"u-txt"
16
17//label--DOM
18htmlFor -->"userName"
1.2 通过属性名访问修改属性
1---
2<div>
3 <label for="userName">用户名:label>
4 <input id="userName" type="text" class="u-txt">
5div>
6---
7var input = document.querySelector('#userName');
8//读取
9input.className;//"u-txt"
10input["id"];//"userName"
11//写入属性
12input.value = "LWL@126.com";
13input.disabled = true;
14//<input id="userName" type="text" class="u-txt" value="LWL@126.com disabled>15/* 通过属性名可以设置的属性类型为 ——>转换过的实用对象 */16/* 17 className :"u-txt" -->String
18 maxLength :10 -->Number
19 disabled :true -->Boolean
20 onclick :function onclick(event){} -->Function
21*/
22
23//通过属性名访问的特点:通用性不好,会有名字异常;扩展性没有;但设置的直接为实用对象;
1.3 Get/SetAttribute
1---
2<div>
3 <label for="userName">用户名:label>
4 <input id="userName" type="text" class="u-text">
5div>
6---
7//读取
8/* var attribute = element.getAttribute(attributeName); */
9input.getAttribute("class");//"u-txt"
10//写入
11/* element.setAttribute(name,value) */
12input.setAttribute("value","LWL@126.com");
13//<input id="userName" type="text" class="u-txt" value="LWL@126.com">
14/* 通过get/setAttribute设置的属性类型为 ——>属性字符串 */
15/*
16 class :"u-txt" -->String
17 maxlength :"10" -->String
18 disabled :"" -->String
19 onclick :"showSuggest();"
20*/
21
22//通过get/setAttribute设置属性的特点:只能设置字符串;但是通用性好;
以上两种属性访问修改方法的使用范例:
1---
2<div>
3 <h2>手机号码登录h2>
4 <label for="userName">用户名:label>
5 <input id="userName" type="text">
6 <label for="secuNum">密码:label>
7 <input id="secuNum" type="text">
8 <button id="logbtn">登录button>
9div>
10---
11//让登录按钮disabled
12button.disabled = true;
13button.setAttribute("class","disabled");//Are you sure ?????
1.4 dataset
1/* HTMLElement.dataset */
2/* data-* 属性集 */
3/* 在元素上保存数据 */
4---
5
"user" 6 data-id="19910620" 7 data-account-name="LWL" 8 data-name="卢万林" 9 data-email="lwl2374@126.com" 10 data-mobile="15528332669"11>12 LWL13
14---
15/* div.dataset.16 id --> "19910620"17 accountName --> "LWL"18 name --> "卢万林"19 email --> "lwl2374@126.com"20 mobiel --> "15528332669"21*/
22//功能需求,在-卢万林-被hover的时候,在名字的右边出现一张表格,里面是个人信息;
23//html
24
class="mb-s" id="p3">25
"b434">26 "b434li1" data-id="19910610" data-account-name="LWL" data-name="Adom|Wanlin-Lu"27 data-email="lwl2374@126.com" data-mobile="15528332668">Wanlin-Lu28 "b434li2" data-id="18820625" data-account-name="HYJ" data-name="huoyuanjia"29 data-email="huoyuanjia@126.com" data-mobile="18780121320">big brother3031
"display:none" id="card">32
33 "accountName">34 name:"name">35 email:"email">36 tele:"mobile">37 38
39//css40#card table th,td,caption{41 border:1px solid #888888;42 padding:2px;43 font-size:14px;44}45#card table th{46 color:#444;47}48#p3{49 position:relative;50}51#card{52 position:absolute;53 left:150px;54 top:50px;55}56#card table{57 border-collapse: collapse;58}59//js60function $(id){61 return document.getElementById(id);62}63var b434ul = $("b434");64var lis = b434ul.getElementsByTagName("li");65for(var i=0,li;li=lis[i];i++){66 li.onmouseenter = function(event){67 event = event||window.event;68 var pers = event.target||event.srcElement;69 var data = pers.dataset;7071 $("accountName").innerText = data.accountName;72 $("name").innerText = data.name;73 $("email").innerText = data.email;74 $("mobile").innerText = data.mobile;7576 $("card").style.display="block";77 };78 li.onmouseleave = function(event){79 $("card").style.display="none";80 };81}
dataset 兼容实现
1/* dataset 兼容实现 */
2function dataset(element){
3 if(element.dataset){
4 return element.dataset;
5 }else{
6 var attributes = element.attributes;
7 var name = [],value = [];
8 var obj = {};
9 for(var i=0;i10 if(attributes[i].nodeName.slice(0,5)=="data-"){
11 name.push(attributes[i].nodeName.slice(5));
12 value.push(attributes[i].nodeValue);
13 }
14 }
15 for(var j=0;j16 obj[name[j]] = value[j];
17 }
18 return obj;
19 }
20}
21//测试dataset(element)
22var b434li2 = $("b434li2");
23var ss = dataset(b434li2);
24console.log(ss);
25//DOMStringMap {id: "18820625", accountName: "HYJ", name: "huoyuanjia", email: "huoyuanjia@126.com", mobile: "18780121320"}
2 样式操作
2.1 CSS-->DOM
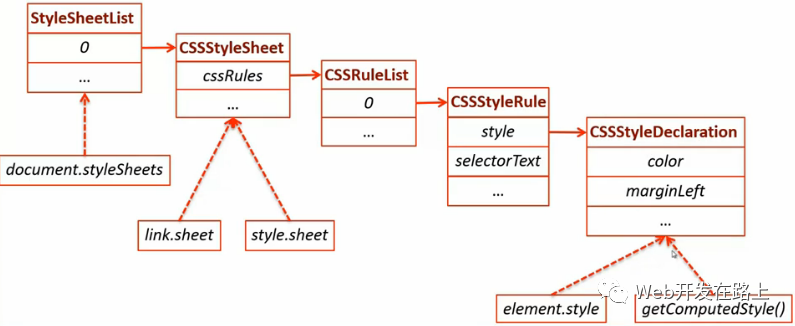
CSSDOM
1//综合样式
2/* document.styelSheets-->elementsheet(|)-->cssRules[i]--->style-->styleName */
3---
4<style> 5 body{margin:20px;} 6 p{color:#aaa;line-height:20px;} 7style>
8---
9//获取line-height
10element.sheet.cssRules[1].style.lineHeight;//"20px"--->这句是有问题的,得不到这样的结果!!
11
12//行内样式
13/* element.style.styleName */
14---
15<p style="color:red;font-size:10px;">you are right,I an a paragraphp>
16---
17
18//获取color
19p.style.color;//"red"
20//获取font-size
21p.style.fontSize;//"10px"
2.2 更新样式
方法有两类、三种:
element.style
,element.style.cssText
和更新element的className
1---
2
3---
4//将上面input元素的边框颜色和文字颜色都设置为red
5
6/* 方法一:element.style */
7element.style.borderColor = 'red';
8element.styel.color = 'red';
9//该方法的问题:更新一个属性需要一条语句;不是我们熟悉的CSS
10
11/* 方法二:element.style.cssText */
12element.style.cssText = "border-color:red;color:red;";
13//该方法的问题:和方法一类似,样式混合在逻辑中;
14
15/* 方法三:更新class */
16---css
17.invalid{
18 border-color:red;
19 color:red;
20}
21---
22//给input添加class
23element.className += " invalid";//
应用拓展:换肤
1在练习的时候补充具体代码,现在只需要了解原理即可!
2html>
3
4
5
6 换肤
7
8
9
10
11
12
13
14
15
张惠妹
张惠妹
16
中国国宝级传奇天后,被歌迷们亲切的称为‘阿妹’。但是这几年却很少看到她的演出了,或许她已经过上了另一种幸福的生活吧?
17
18
19
20 创建外部样式表21 创建内部样式表22
23
59
60
2.3 获取样式
1/* element.style */
2---
3
4---
5element.style.color;//'red'
6//`element.style`只能获取元素的行内样式,而且获得的还不一定是元素的实际样式。
7
8/* window.getComputedStyle() */
9/* var style = window.getComputedStyle(element[,psedoElt]); */
10---html
11
12---
13//获取input的color
14window.getComputedStyle(element).color;//"rgb(0,0,0)"
15---html
16
17---
18//获取input的color
19window.getComputedStyle(element).color;//"rgb(255,0,0)"
20//window.getComputedStyle是一个CSSStyleDeclaration对象(CSS属性都列出来了,数量巨大),可以在console中查看
21
22/* ie9-中使用element.currentStyle */