using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace PictureTurnPdf
{
public partial class FrmMain : Form
{
public FrmMain()
{
InitializeComponent();
}
private void FrmMain_Load(object sender, EventArgs e)
{
cbPicBrowse.SelectedIndex = 0;
cbSaveBrowse.SelectedIndex = 0;
}
#region txtPicBrowse改变事件
private void txtPicBrowse_TextChanged(object sender, EventArgs e)
{
listBox1.Items.Clear();
btnCombine.Enabled = btnTurnPdf.Enabled = false;
if (txtPicBrowse.Text != "")
{
DirectoryInfo TheFolder1 = new DirectoryInfo(txtPicBrowse.Text);
foreach (FileInfo NextFile in TheFolder1.GetFiles(cbPicBrowse.Text))
this.listBox1.Items.Add(NextFile.Name);
label6.Text = string.Format("共:{0} 个文件", listBox1.Items.Count);
if (listBox1.Items.Count >= 2 && txtSavePath.Text != "")
btnCombine.Enabled = btnTurnPdf.Enabled = true;
else
btnCombine.Enabled = btnTurnPdf.Enabled = false;
}
}
#endregion
#region cbPicBrowse改变事件
private void cbPicBrowse_SelectedIndexChanged(object sender, EventArgs e)
{
cbSaveBrowse.SelectedIndex = cbPicBrowse.SelectedIndex;
}
#endregion
#region 合并图片选择按钮
private void btnPicBrowse_Click(object sender, EventArgs e)
{
txtPicBrowse.Text = GetButton_Click(sender);
}
/// <summary>
/// 根据按钮获取对应选择路径
/// </summary>
/// <param name="sender"></param>
/// <returns></returns>
public string GetButton_Click(object sender)
{
string sPath = "";
switch (((Button)sender).Name)
{
case "btnPicBrowse":
if (folderBrowserDialog1.ShowDialog() == DialogResult.OK)
{
sPath = folderBrowserDialog1.SelectedPath.ToString();
}
break;
case "btnSaveBrowse":
if (folderBrowserDialog1.ShowDialog() == DialogResult.OK)
{
sPath = folderBrowserDialog1.SelectedPath.ToString();
}
break;
}
return sPath;
}
#endregion
#region txtSavePath改变事件
private void txtSavePath_TextChanged(object sender, EventArgs e)
{
if (listBox1.Items.Count >= 2 && txtSavePath.Text != "")
btnCombine.Enabled = btnTurnPdf.Enabled = true;
else
btnCombine.Enabled = btnTurnPdf.Enabled = false;
}
#endregion
#region 合并的路径选择按钮
private void btnSaveBrowse_Click(object sender, EventArgs e)
{
txtSavePath.Text = GetButton_Click(sender);
}
#endregion
#region 开始合并按钮
private void btnCombine_Click(object sender, EventArgs e)
{
try
{
cbPicBrowse.Enabled = false;
cbSaveBrowse.Enabled = false;
btnPicBrowse.Enabled = false;
btnSaveBrowse.Enabled = false;
btnCombine.Enabled = btnTurnPdf.Enabled = false;
numUdown.Enabled = false;
rbtn1.Enabled = false;
rbtn2.Enabled = false;
Bitmap[] bit = new Bitmap[(int)numUdown.Value];
int j = 0;
pBar.Maximum = listBox1.Items.Count;
pBar.Value = 0;
for (int i = 1; i < listBox1.Items.Count + 1; i++)
{
string imgstr = listBox1.Items[i - 1].ToString();
bit[j] = new Bitmap(txtPicBrowse.Text + "\\" + imgstr);
j++;
if (i % (int)numUdown.Value == 0)
{
j = 0;
string str;
str = txtSavePath.Text + "\\temp" + ((int)(i / (int)numUdown.Value)).ToString() + cbSaveBrowse.Text;
if (this.cbSaveBrowse.SelectedIndex == 0)
{
MergerImg(bit).Save(str, System.Drawing.Imaging.ImageFormat.Bmp);
}
else
{
MergerImg(bit).Save(str, System.Drawing.Imaging.ImageFormat.Jpeg);
}
}
if (pBar.Value < pBar.Maximum)
pBar.Value += 1;
labSchedule.Text = ((pBar.Value * 100) / (pBar.Maximum)).ToString() + " %";
Application.DoEvents();
}
MessageBox.Show("合并完成!", "提示", MessageBoxButtons.OK);
}
catch (Exception ee)
{
MessageBox.Show(ee.Message);
}
finally
{
cbPicBrowse.Enabled = true;
cbSaveBrowse.Enabled = true;
btnPicBrowse.Enabled = true;
btnSaveBrowse.Enabled = true;
btnCombine.Enabled = btnTurnPdf.Enabled = true;
numUdown.Enabled = true;
rbtn1.Enabled = true;
rbtn2.Enabled = true;
}
}
/// <summary>
/// 合并方法
/// </summary>
/// <param name="maps"></param>
/// <returns></returns>
private Bitmap MergerImg(params Bitmap[] maps)
{
int X = 0;
int Y = 0;
Bitmap newImg;
for (int i = 0; i < maps.Length; i++)
{
//X = X > maps[i].Width ? X : maps[i].Width;
//Y += maps[i].Height;
//增加合并图片之间的距离
X = X > maps[i].Width ? X + 300 : maps[i].Width + 300;
Y += maps[i].Height + 200;
}
newImg = new Bitmap(X, Y);
X = 0;
Y = 0;
Graphics g = Graphics.FromImage(newImg);
g.Clear(System.Drawing.Color.White);
for (int i = 0; i < maps.Length; i++)
{
g.DrawImage(maps[i], X, Y, maps[i].Width, maps[i].Height);
Y += maps[i].Height;
}
g.Dispose();
return newImg;
}
#endregion
#region 图片转PDF按钮
private void btnTurnPdf_Click(object sender, EventArgs e)
{
string[] files =new string[listBox1.Items.Count];
for (int i = 0; i < listBox1.Items.Count; i++)
{
string imgstr = listBox1.Items[i].ToString();
files[i] = txtPicBrowse.Text + "\\" + imgstr;
}
iTextSharp.text.Document document = new iTextSharp.text.Document(iTextSharp.text.PageSize.A4, 25, 25, 25, 25);
try
{
iTextSharp.text.pdf.PdfWriter.GetInstance(document, new FileStream(txtSavePath.Text + "\\save.pdf", FileMode.Create, FileAccess.ReadWrite));
document.Open();
iTextSharp.text.Image image;
for (int i = 0; i < files.Length; i++)
{
if (String.IsNullOrEmpty(files[i])) break;
image = iTextSharp.text.Image.GetInstance(files[i]);
if (image.Height > iTextSharp.text.PageSize.A4.Height - 25)
{
image.ScaleToFit(iTextSharp.text.PageSize.A4.Width - 25, iTextSharp.text.PageSize.A4.Height - 25);
}
else if (image.Width > iTextSharp.text.PageSize.A4.Width - 25)
{
image.ScaleToFit(iTextSharp.text.PageSize.A4.Width - 25, iTextSharp.text.PageSize.A4.Height - 25);
}
image.Alignment = iTextSharp.text.Image.ALIGN_MIDDLE;
document.NewPage();
document.Add(image);
//iTextSharp.text.Chunk c1 = new iTextSharp.text.Chunk("Hello World");
//iTextSharp.text.Phrase p1 = new iTextSharp.text.Phrase();
//p1.Leading = 150; //行间距
//document.Add(p1);
}
}
catch (Exception ex)
{
Console.WriteLine("转换失败,原因:" + ex.Message);
}
document.Close();
}
#endregion
}
}
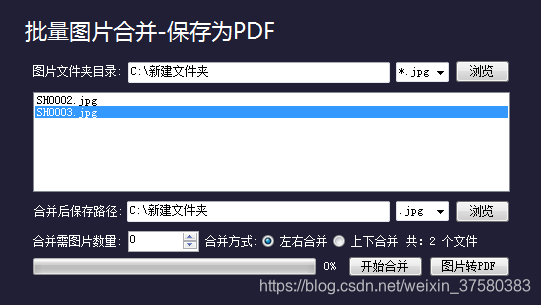