具体实现功能
- 切换选项卡
- 添加选项卡
- 删除选项卡
- 编辑选项卡
html结构
<div class="tabsbox" id="tab">
<nav class="firstnav">
<ul>
<li class="liactive">
<span>测试1</span>
<span class="close">×</span>
</li>
<li><span>测试2</span><span class="close">×</span></li>
<li><span>测试3</span><span class="close">×</span></li>
</ul>
<div class="tabadd">
<span>+</span>
</div>
</nav>
<div class="tabscon">
<section class="conactive">内容1</section>
<section>内容2</section>
<section>内容3</section>
</div>
</div>
css部分
* {
margin: 0;
padding: 0;
}
main {
width: 900px;
margin: 0px auto;
}
h4 {
text-align: center;
line-height: 50px;
}
.tabsbox {
width: 800px;
margin: 0 auto;
border: 1px solid orange;
}
.firstnav {
position: relative;
line-height: 40px;
height: 40px;
text-align: center;
border-bottom: 1px solid #999;
}
.firstnav li {
list-style: none;
width: 100px;
float: left;
border-right: 1px solid #999;
position: relative;
cursor: pointer;
}
.firstnav li span {
user-select: none;
}
.firstnav li.liactive::after {
content: "";
width: 100%;
height: 2px;
position: absolute;
z-index: 11;
bottom: -2px;
left: 0;
background: #fff;
}
.firstnav .close {
cursor: pointer;
position: absolute;
right: 2px;
top: 2px;
font-size: 14px;
color: #666;
border: 1px solid #ccc;
width: 14px;
height: 14px;
line-height: 12px;
text-align: center;
border-radius: 100%;
}
.tabscon {
height: 300px;
white-space: normal;
}
.tabscon input {
width: 100%;
height: 80px;
}
.tabscon section {
padding: 30px;
display: none;
}
.tabscon section.conactive {
display: block;
}
.tabadd {
position: absolute;
padding: 0 10px;
right: 10px;
top: 0px;
font-size: 20px;
cursor: pointer;
user-select: none;
}
input {
width: 50px;
line-height: 20px;
}
jQuery部分
$(function() {
$('ul').on('click', 'li', function() {
$(this).addClass('liactive').siblings().removeClass('liactive')
var index = $(this).index()
$('.tabscon section').eq(index).stop().show().siblings().hide()
})
$('.tabadd').click(function() {
if ($('li').length >= 7) {
return
}
var li = `
<li><span>New Tab</span><span class="close">×</span></li>
`
var section = `
<section>新增内容</section>
`
$('ul').append(li)
$('.tabscon').append(section)
$('ul').children().eq($('ul li').length - 1).click()
})
$('ul').on('click', '.close', function() {
var index = $(this).parent('li').index()
if ($(this).parent().hasClass('liactive')) {
if (index != 0) {
$(this).parent('li').prev().click()
} else if ($('ul li').length != 1) {
$(this).parent('li').next().click()
}
}
$(this).parent().remove()
$('.tabscon section').eq(index).remove()
})
$('ul').on('dblclick', 'li', function() {
$(this).children().eq(0).html('<input type="text" value="' + $(this).children().eq(0).text() + '"/>')
$(this).find('input').select()
$('input').blur(function() {
$(this).parent().text($(this).val())
})
$('input').keyup(function(e) {
if (e.keyCode == 13) {
$(this).blur()
}
})
})
})
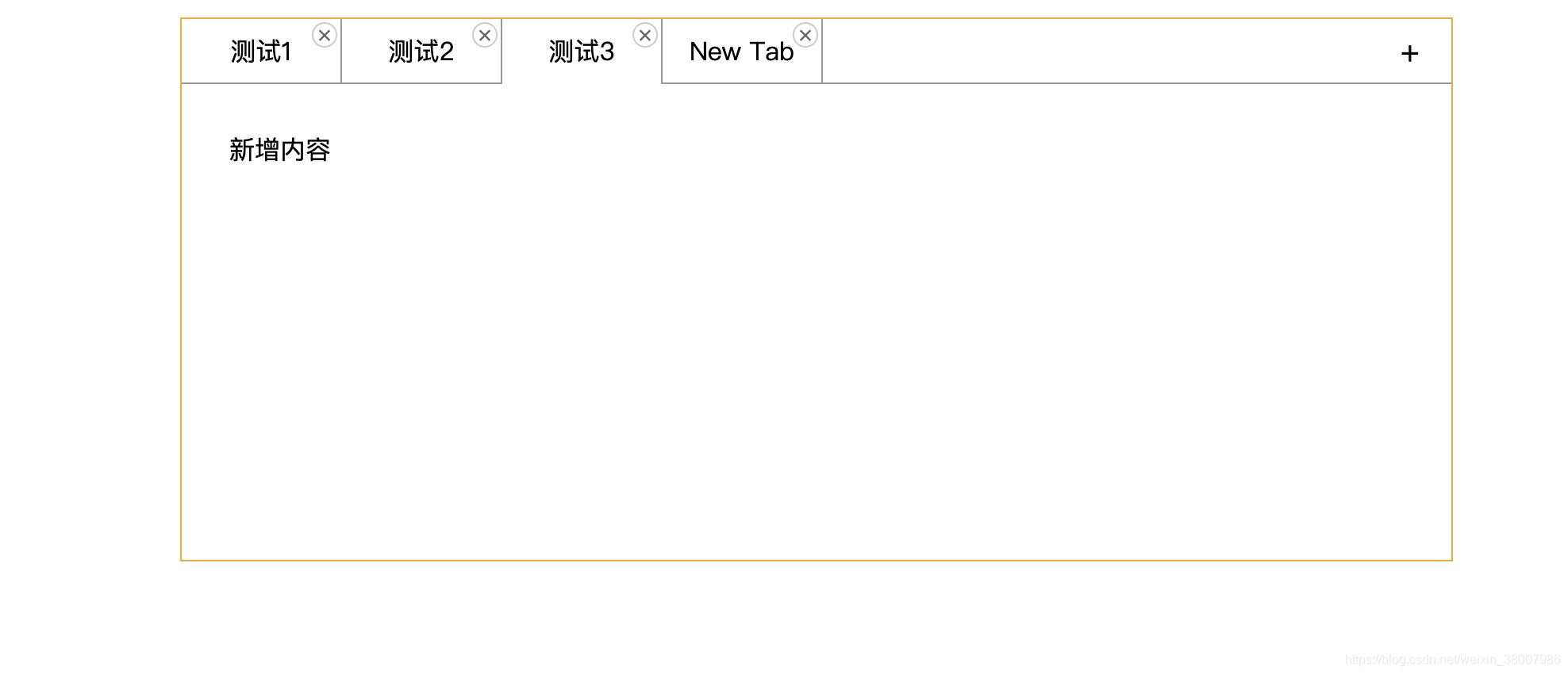