新浪微博OAuth2.0授权登陆
注册应用:http://open.weibo.com/
1. 申请APP Key和App Secret
想要使用新浪微博的账户接入服务,必须首先在新浪微博开发平台创建一个应用,并申请得到APP Key和App Secret,还要设置CALLBACK_URL
Key和Secret在创建完应用之后,就可以看到。
CALLBACK_URL设置的地方比较隐蔽:
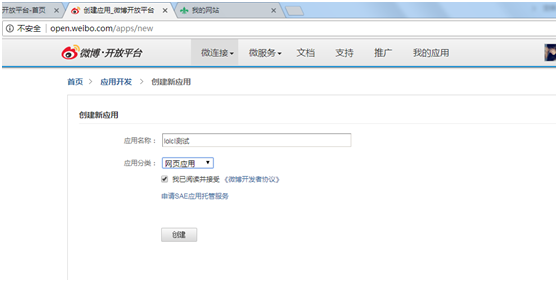
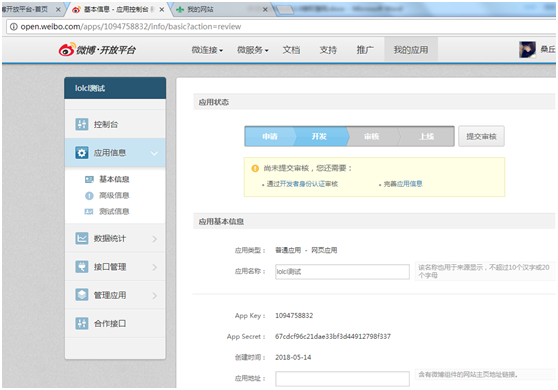
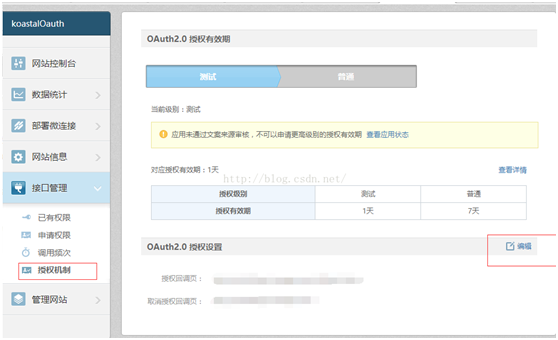
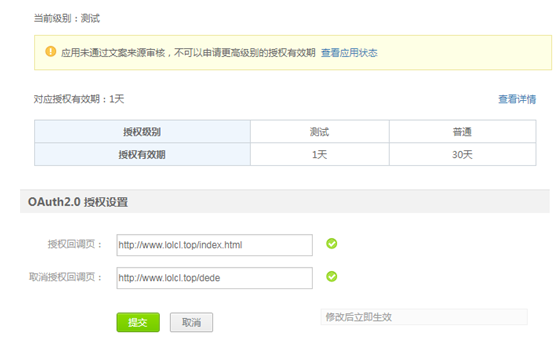
重定向地址:http://www.lolcl.top/index.html
获取到的信息
App Key: 1094758832 App Secret: 67cdcf96c21dae33bf3d44912798f337
|
2. 请求OAuth登录页
授权地址:
https://api.weibo.com/oauth2/authorize
使用GET方式传入以下参数:
client_id=YOUR_CLIENT_ID(APP Key)
response_type=code(验证码模式,共4种模式)
redirect_uri=YOUR_REGISTERED_REDIRECT_URI(重定向URL)
获取code完整地址:
3. OAuth服务器返回授权结果
(1)如果用户放弃登录,则会跳转到取消授权回调页
(2)如果用户登录成功,则会跳转到授权回调页,并且会返回一个code参数,供我们进一步获取Access Token使用。
4. 使用code参数,向OAuth服务器换取Access Token
授权地址:
https://api.weibo.com/oauth2/access_token
传入参数:
client_id=YOUR_CLIENT_ID
client_secret=YOUR_CLIENT_SECRET
grant_type=authorization_code //该参数是固定值
redirect_uri=YOUR_REGISTERED_REDIRECT_URI
code=CODE //这就是上一步获取的code值
http://www.lolcl.top/index.html?code=58fdd97931b3f2aab5e7d6be93773c87
完整地址:
https://api.weibo.com/oauth2/access_token?client_id=1094758832&client_secret=67cdcf96c21dae33bf3d44912798f337&grant_type=authorization_code&redirect_uri=http://www.lolcl.top/index.html&code=58fdd97931b3f2aab5e7d6be93773c87
5. 获取Access Token
使用java post请求http 获取返回值中的token
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.URL;
import java.net.URLConnection;
public class HttpRequest {
/**
* 向指定 URL 发送POST方法的请求
*
* @param url
* 发送请求的 URL
* @param param
* 请求参数,请求参数应该是 name1=value1&name2=value2 的形式。
* @return 所代表远程资源的响应结果
*/
public static String sendPost(String url, String param) {
//设置代理 如果本地没使用代理可不写该2行
System.getProperties().setProperty("http.proxyHost", PropertiesConfig.getValue("http.proxyHost"));//代理服务器IP
System.getProperties().setProperty("http.proxyPort", PropertiesConfig.getValue("http.proxyPort")); //使用端口号
PrintWriter out = null;
BufferedReader in = null;
String result = "";
try {
URL realUrl = new URL(url);
// 打开和URL之间的连接
URLConnection conn = realUrl.openConnection();
// 设置通用的请求属性
conn.setRequestProperty("accept", "*/*");
conn.setRequestProperty("connection", "Keep-Alive");
conn.setRequestProperty("user-agent",
"Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1;SV1)");
// 发送POST请求必须设置如下两行
conn.setDoOutput(true);
conn.setDoInput(true);
// 获取URLConnection对象对应的输出流
out = new PrintWriter(conn.getOutputStream());
// 发送请求参数
out.print(param);
// flush输出流的缓冲
out.flush();
// 定义BufferedReader输入流来读取URL的响应
in = new BufferedReader(
new InputStreamReader(conn.getInputStream()));
String line;
while ((line = in.readLine()) != null) {
result += line;
}
} catch (Exception e) {
System.out.println("发送 POST 请求出现异常!"+e);
e.printStackTrace();
}
//使用finally块来关闭输出流、输入流
finally{
try{
if(out!=null){
out.close();
}
if(in!=null){
in.close();
}
}
catch(IOException ex){
ex.printStackTrace();
}
}
return result;
}
}
6. 通过Access Token,调用微博可用API
我们在上一步已经将Access Token存到SESSION中,然后可以调用微博系统允许我们调用的API了。
// 新浪获取token 是dopost方式 且两个小时token失效
String result = HttpRequest.sendPost(accessTokenURL, params);
System.out.println("发送post 请求到服务器 获取access_token---------------result:"+result);
// {"access_token":"2.00f7DYzCWXsADDaf09250ca308lmdr","remind_in":"157679999","expires_in":157679999,"uid":"2741738497","isRealName":"true"}
Map<String , String> token = new HashMap<String, String>();
JSONObject json = JSONObject.parseObject(result);
token.put("accessToken", (String)json.get("access_token"));
token.put("uid", json.getString("uid"));
String accessToken = token.get("accessToken");
String uid = token.get("uid");
System.out.println(accessToken+"------"+uid);
获取用户信息
/**
* 获取用户信息
* @param token
* @return
*/
private Map<String , String> getUserWeiBoInfo(Map<String , String> token){
Map<String , String> userData = new HashMap<String, String>();
String UserInfo = "";
String url = PropertiesConfig.getValue("userInfoURL")+"?access_token="+token.get("accessToken")+"&uid="+token.get("uid");
System.out.println( url);
GetMethod getMethod = new GetMethod(url);
HttpClient client = new HttpClient();
try {
client.executeMethod(getMethod);
UserInfo = getMethod.getResponseBodyAsString();
System.out.println("UserInfo"+UserInfo);
JSONObject jsonData = JSONObject.parseObject(UserInfo);
userData.put("name",jsonData.getString("name").toString() );
userData.put("headImg", jsonData.getString("profile_image_url"));
} catch (Exception e) {
e.printStackTrace();
}
return userData;
}
Map<String, String> userWeiBoInfo = getUserWeiBoInfo(token);
for(Entry<String, String> entry : userWeiBoInfo.entrySet()){
System.out.println(entry.getKey()+"----"+entry.getValue());
}
资源下载地址:https://download.csdn.net/download/weixin_39209728/10420502