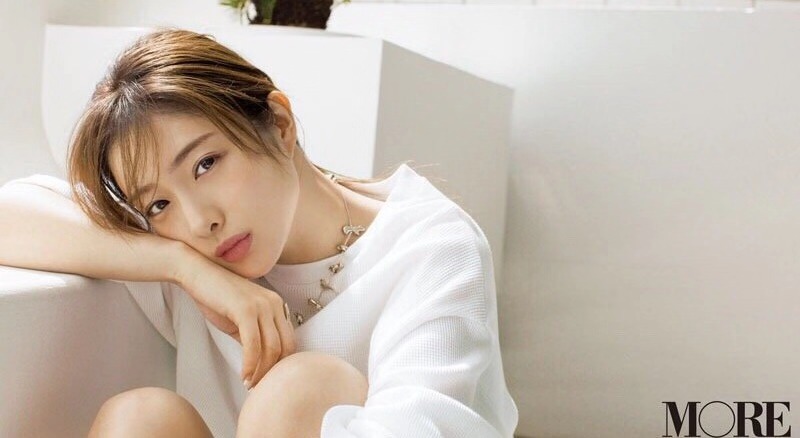
在leetcode 中, 快慢指针和双指针的related topics都是two pointers, 其实两种算法的应用场景还是有很大不同, 所以本篇文章单独把快慢指针列出来。
快慢指针方法也被称为 Hare & Tortoise 算法,该算法会使用两个在数组(或序列/链表)中以不同速度移动的指针。该方法在处理循环链表或数组时非常有用。
该算法的应用场景:
- 处理链表或数组中的循环的问题
- 找链表中点或需要知道特定元素的位置
何时应该优先选择这种方法,而不是上面提到的二指针方法?
- 有些情况不适合使用二指针方法,比如在不能反向移动的单链接链表中。使用快速和慢速模式的一个案例是当你想要确定一个链表是否为回文(palindrome)时。
例如:判断一个链表中是否有环, 迭代的方式如图:
fast 指针每次移动两格, slow指针每次移动一格, 如果存在环路, 则fast最终一定会和slow相遇。
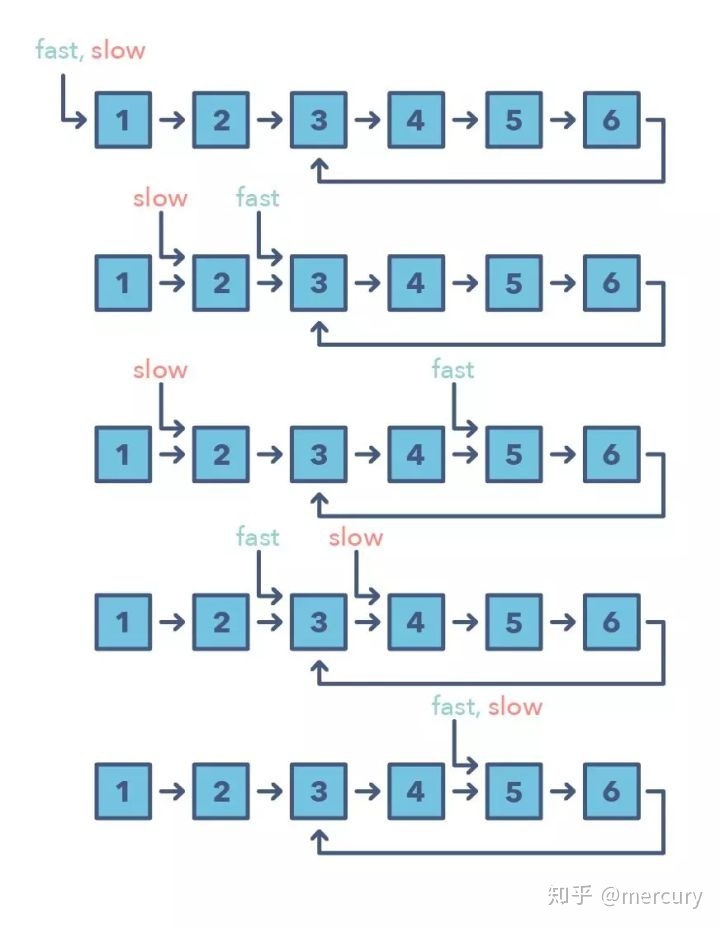
下面看几道例题:
一、 判断链表是否有环
leet code 第141题 - 难度 easy
public boolean hasCycle(ListNode head) {
if (head == null || head.next == null) {
return false;
}
ListNode slow = head;
ListNode fast = head.next;
while (slow != fast) {
if (fast == null || fast.next == null) {
return false;
}
slow = slow.next;
fast = fast.next.next;
}
return true;
}
二、返回一个存在环路的链表中环路开始的位置
来源: leet code - 142 题 - 难度 medium
描述: Given a linked list, return the node where the cycle begins. If there is no cycle, return null
. To represent a cycle in the given linked list, we use an integer pos
which represents the position (0-indexed) in the linked list where tail connects to. If pos
is -1
, then there is no cycle in the linked list.
Note: Do not modify the linked list.
Example 1:
Input: head = [3,2,0,-4], pos = 1
Output: tail connects to node index 1
Explanation: There is a cycle in the linked list, where tail connects to the second node.
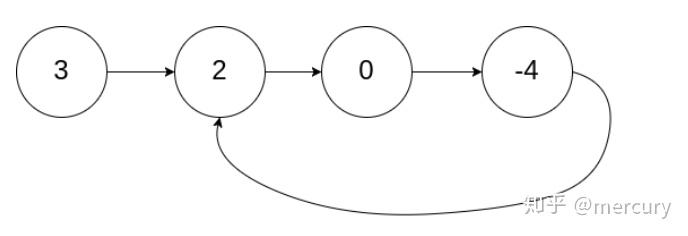
思路:
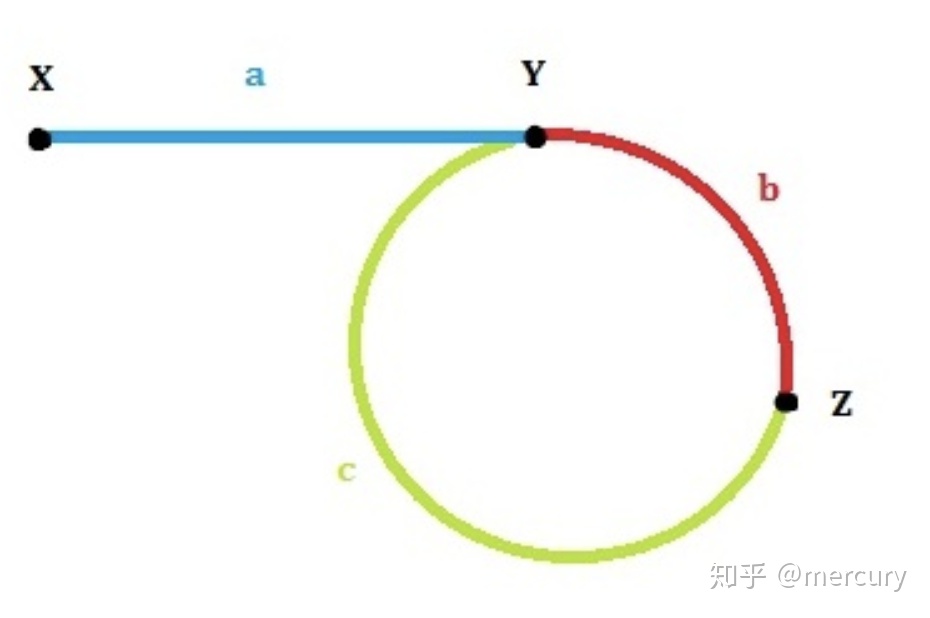
首先, 用快慢指针我们可以判断出链表中是否有环, 假设在z 点 fast指针和slow 指针相遇。x-y 长度为a, y-z 长度为 b , z-y 长度为c。
则:slow 指针走了 a+b 的距离。 fast 指针走了 a + n(b + c)+ b 的距离。(可能不仅绕了一圈而是绕环走了n圈)
fast指针的速度是slow 指针速度的2倍。 所以a + b + n(b + c) = 2 (a + b), 简化得 a+b = n(b + c)。 因为我们想要求 a 的距离, 所以我们移动方程 得到 a = (n-1)b + n c 。
仔细观察上面的等式。 考虑它的含义, 即c 总比b 多一次, 这就意味着, 我们再放两个指针, first放在x ,second放在z ,这次保持速度一致, first 跑到y , second 也会跑到y。所以,当它们相遇,就是cycle的起点。
public ListNode detectCycle(ListNode head) {
if(head == null || head.next == null ) {
return null;
}
ListNode fast = head;
ListNode slow = head;
// 找到相遇点z (对应上图)
while(true) {
if(fast == null || fast.next == null) {
return null;
}
fast = fast.next.next;
slow = slow.next;
if (fast == slow) {
break;
}
}
// 想到 cycle起点y (对应上图)
slow = head;
while(fast != slow) {
fast = fast.next;
slow = slow.next;
}
return fast;
}
三、 判断链表是否是回文链表
来源: leet code 第234 题 - 难度 easy
描述: Given a singly linked list, determine if it is a palindrome.
Example 1:
Input: 1->2
Output: false
Example 2:
Input: 1->2->2->1
Output: true
解题思路:用快慢指针找到链表的中点, 把后半部分链表逆序,再和前半部分链表做比较是否每个元素都相等。
public boolean isPalindrome(ListNode head) {
if(head == null || head.next == null) {
return true;
}
// 1. 找到链表中点。 比如length=4,找到的slow指针的index为1,length5,找到的slow指针index为2
ListNode fast = head.next;
ListNode slow = head;
while(fast != null && fast.next != null) {
fast = fast.next.next;
slow = slow.next;
}
// 2。从中点位置后的第一个元素开始反转后面的链表
slow = slow.next;
ListNode p = slow;
ListNode q = p.next;
while(q!= null) {
ListNode tmp = q.next;
q.next = p;
p = q;
q= tmp;
}
slow.next = null;
// 3。 只要后面的元素和前半部分都相等, 则链表是回文链表
while(p!= null){
if(p.val != head.val) {
return false;
}
p = p.next;
head = head.next;
}
return true;
}