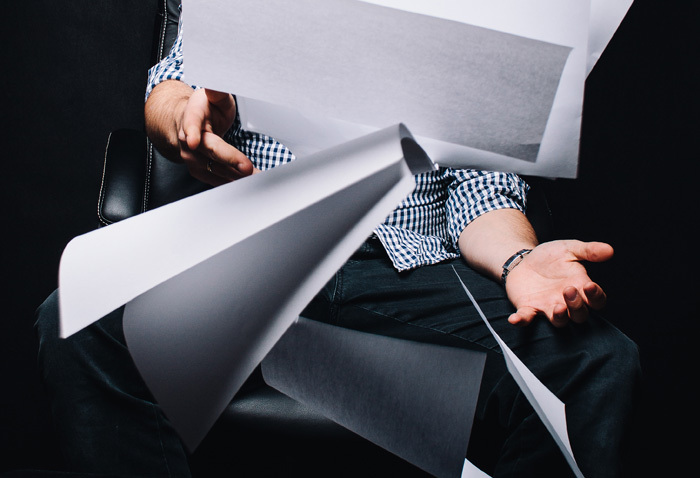
前言
要学习Vuex就要先知道这里的路由是什么?为什么我们不能像原来一样直接用标签编写链接哪?vue-router如何使用?常见路由操作有哪些?等等这些问题,就是本篇要探讨的主要问题。
Vuex是什么
Vuex 是一个专为 Vue.js 应用程序开发的状态管理模式。它采用集中式存储管理应用的所有组件的状态,并以相应的规则保证状态以一种可预测的方式发生变化。
State:类似与单组件中的data ()。
Getter:像计算属性一样,getter 的返回值会根据它的依赖被缓存起来,且只有当它的依赖值发生了改变才会被重新计算。
Mutation:状态只能通过mutation修改,mutation是同步的。
Action:Action 提交的是 mutation,而不是直接变更状态。Action是异步操作。
Module:Vuex 允许我们将 store 分割成模块(module)。每个模块拥有自己的 state、mutation、action、getter、甚至是嵌套子模块
如何使用Vuex
首先,安装Vuex
npm install Vuex
接下来,创建一个 store。创建过程直截了当——仅需要提供一个初始 state 对象和一些 mutation:
import Vue from 'vue'
import Vuex from 'vuex'
import axios from "axios";
Vue.use(Vuex)
const store = new Vuex.Store({
state: {
Todos: []
},
mutations: {
setTodos(state) {
const url = "https://localhost:44399/api/todo?pageIndex=1&pageSize=100";
axios.get(url)
.then((response) => {
console.log(response);
if (response.data.Code === 0) {
state.Todos = response.data.Result;
}
});
},
createTodo(state, item) {
const url = "https://localhost:44399/api/todo";
axios.post(url, item)
.then((response) => {
console.log(response);
if (response.data.Code === 0) {
this.commit("setTodos");
}
});
},
updateTodo(state, item) {
const url = `https://localhost:44399/api/todo/${item.Id}`;
axios.put(url, item)
.then((response) => {
console.log(response);
if (response.data.Code === 0) {
this.commit("setTodos");
}
});
},
deleteTodo(state, item) {
const url = `https://localhost:44399/api/todo/${item.Id}`;
axios.delete(url)
.then((response) => {
console.log(response);
if (response.data.Code === 0) {
this.commit("setTodos");
}
});
},
}
})
export default store //用export default 封装代码,让外部可以引用
接下来,在main.js文件中引入store/index.js文件:
import store from './store/index.js'
new Vue({
router,
store,//注册上vuex的store: 所有组件对象都多一个属性$store
render: h => h(App),
}).$mount('#app')
最后,新建一个组件TodoListWithVuex.vue
<template>
<div style="text-align: left">
<el-button type="text" @click="addTodo()">新增</el-button>
<el-table :data="Todos" style="width: 100%">
<el-table-column type="index" width="50"> </el-table-column>
<el-table-column prop="Name" label="名称"> </el-table-column>
<el-table-column fixed="right" label="操作" width="100">
<template slot-scope="scope">
<el-button @click="editTodo(scope.$index)" type="text" size="small">编辑</el-button>
<el-button @click="deleteTodo(scope.$index)" type="text" size="small">删除</el-button>
</template>
</el-table-column>
</el-table>
<TodoAddWithUI :dialogVisible="addDialogVisible" :selectedItem="selectedItem" @save="createTodo" @cancel="cancel"></TodoAddWithUI>
<TodoEditWithUI :dialogVisible="editDialogVisible" :selectedItem="selectedItem" @save="updateTodo" @cancel="cancel"></TodoEditWithUI>
</div>
</template>
<script>
import TodoAddWithUI from "./TodoAddWithUI.vue";
import TodoEditWithUI from "./TodoEditWithUI.vue";
export default {
components: {
TodoAddWithUI,
TodoEditWithUI,
},
data() {
return {
selectedIndex: -1, // 选择了哪条记录
selectedItem: {}, // 选中的信息
addDialogVisible: false,
editDialogVisible: false,
};
},
computed: {
Todos() {
return this.$store.state.Todos;
}
},
created: function () {
this.setTodos()
},
methods: {
setTodos() {
this.$store.commit("setTodos");
},
addTodo() {
this.addDialogVisible = true;
},
createTodo(item) {
this.$store.commit("createTodo",item);
this.selectedIndex = -1;
this.selectedItem = {};
this.addDialogVisible = false;
},
editTodo(index) {
this.selectedIndex = index;
this.selectedItem = JSON.parse(JSON.stringify(this.Todos[index]));
this.editDialogVisible = true;
},
updateTodo(item) {
this.$store.commit("updateTodo",item);
this.selectedIndex = -1;
this.selectedItem = {};
this.editDialogVisible = false;
},
deleteTodo(index) {
this.$store.commit("deleteTodo",this.Todos[index]);
this.selectedIndex = -1;
this.selectedItem = {};
},
cancel() {
this.addDialogVisible = false;
this.editDialogVisible = false;
},
},
};
</script>
小结
目前为止,我们完成了Vuex的基本用法,Vuex主要管理多组件状态的共享,如果只是单个组件交互及父子组件交互应该是没有必要使用的。
文中用到的代码我们放在:https://github.com/zcqiand/miscellaneous/tree/master/vue