在 JavaScript 中,我们常常会有对数字或者数字字符串的取整需求,要么向上取整Math.ceil(number)
,要么向下取整Math.floor(number)
,还有一种情况就是parseInt
,parseInt
是当数字大于 0 时,向下取整,小于 0 时,向上取整。但是在 es6 中我们有了新的方法Math.trunc(number)
。
es5 和 es6 取整操作
const number = 80.6
// Old Way
number < 0 ? Math.ceil(number) : Math.floor(number);
// or
const es5 = Number.parseInt(number);
// 80
// ✅ES6 Way
const es6 = Math.trunc(number);
// 80
现在我们来看一些特殊值的结果。
- Math.trunc
Math.trunc(80.1); // 80
Math.trunc(-80.1); // -80
Math.trunc('80.1'); // 80
Math.trunc('hello'); // NaN
Math.trunc(NaN); // NaN
Math.trunc(undefined); // NaN
Math.trunc(); // NaN
- parseInt
parseInt(80.1); // 80
parseInt(-80.1); // -80
parseInt('80.1'); // 80
parseInt('hello'); // NaN
parseInt(NaN); // NaN
parseInt(undefined); // NaN
parseInt(); // NaN
parseInt 和 Math.trunc 的区别
parseInt 常常被接收一个字符串的参数,而 Math.trunc 则可以接收一个数字参数,所以如果要对数字取整,还是建议使用 Math.trunc。
parseInt 使用中的问题
当你使用 parseInt 的时候,如果你传入的不是字符串,比如传入一个数字,parseInt 会先调用数字的 toString() 方法,大多数情况下,parseInt 是没有问题的,但是下面的情况就会出现问题:
const number = 11111111111111111111111111111;
const result = parseInt(number);
console.log(result); // 1 <--
这是什么原因呢,因为你传的参数不是 String,所以 parseInt 会先调用 toString() 方法。
const number = 11111111111111111111111111111;
const result = number.toString();
console.log(result); // "1.1111111111111112e+28"
const last = parseInt(result); // 1 <--
所以当我们对 1.1111111111111112e+28
取整时,就只能取到 1,由于这种潜在的问题,你就需要使用 Math 的方法。
浏览器支持情况
大多数现代浏览器都是支持的,除了 ie。
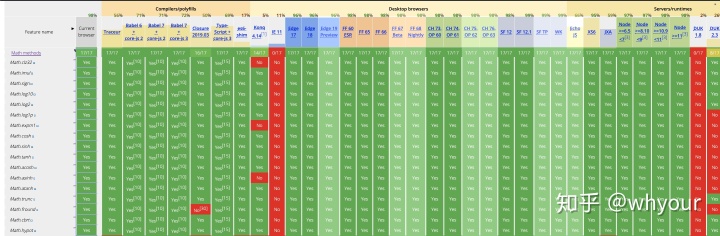
其他取整方法
位操作符 NOT
console.log(~~80.6); // 80
位操作符 OR
console.log(80.6 | 0); // 80
reference
- MDN Web Docs: Math.trunc
- MDN Web Docs: parseInt
- MDN Web Docs: Bitwise operators
- JS Tip: Use parseInt for strings, NOT for numbers
- 2ality: parseInt doesn’t always correctly convert to integer
- Number Truncation in JavaScript