1)派生类继承基类构造的简化
c++98的使用基类有参构造的通用写法:
基类A构造函数有参数,派生类D就必须定义对于的有参构造,再传递给A。
#include "stdafx.h"
#include <iostream>
using namespace std;
struct A
{
A(int i) {
cout << "A construction" << endl;
};
};
struct D:A
{
D(int i) :A(i) {
cout << "D construction" << endl;
}
};
void main() {
D d(1);
}
c++11语法:
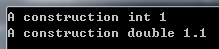
通过using A::A的声明,把基类的构造函数悉数继承到派生类中。可以理解为,使用后把对于的基类的构造函数给隐式声明了。
2) 继承构造函数冲突的处理方法
类的构造函数继承是要求有唯一性的,比如
#include "stdafx.h"
#include <iostream>
using namespace std;
struct A
{
A(int i) {
cout << "A construction int " <<i<< endl;
};
};
struct B
{
B(int i) {
cout << "B construction int " << i << endl;
};
};
struct D : A,B
{
using A::A;
using B::B;
};
void main() {
D d(1);
}
会出现下图错误,意思是已经继承了A的构造就不能再继承B的构造函数。
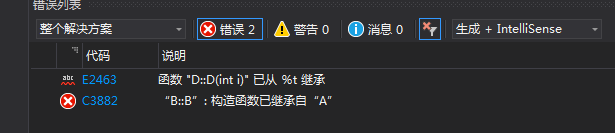
处理办法:添加显式声明,我在VS2017下测试不成功,在g++下面没有验证。
struct D : A,B
{
using A::A;
using B::B;
D(int){};
};
3)使用继承构造函数后,派生类的构造函数没有调用问题
class Base
{
public:
Base() {};
Base(int a) {};
~Base() {};
private:
};
class Drived : Base
{
using Base::Base; //继承base的所有构造函数
public:
Drived() {}; //程序运行后没有执行
~Drived() {};
private:
};
void main() {
Drived * c = new Drived(1);
}
后来在派生类中添加同样参数的构造函数才能执行。
class Base
{
public:
Base() {};
Base(int a) {};
~Base() {};
private:
};
class Drived : Base
{
using Base::Base; //继承base的所有构造函数
public:
Drived(int a) {}; //添加了这句,并且执行了这条
Drived() {}; //程序运行后没有执行
~Drived() {};
private:
};
void main() {
Drived * c = new Drived(1);
}
从上面的例子看出,在使用了继承构造函数后,
如果派生类构造中没有匹配的构造函数,那么就不会被调用,而是直接调用了基类的构造函数,并且把参数传递给了基类。
如果派生类构造函数中有匹配的构造函数,那么当然会调用,并且会自动调用基类同参数构造函数。