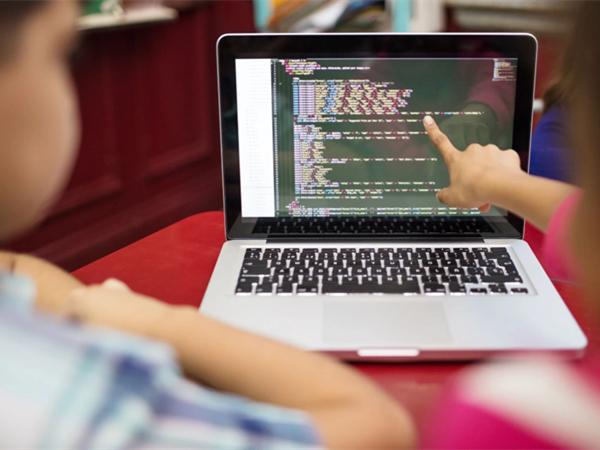
本文旨在提供对Python关键字的详细了解。
1. True:此关键字用于表示布尔值true。如果条件为真,则打印“True”。
2. False:此关键字用于表示布尔值false。如果语句为假,则打印“False”。
python中的True和False与1和0相同。示例:
print False == 0print True == 1 print True + True + Trueprint True + False + False
3. None:这是一个特殊常量,用于表示空值。重要的是要记住,0,任何空容器(例如,空列表)都不会计算为None。
它是一个自己数据类型的对象,NoneType。无法创建多个None对象并将其分配给变量。
4. and:这是python中的逻辑运算符。“and”的真值表如下所示。
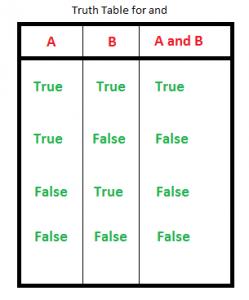
3 and 0返回03 and 10返回1010 and 20 and 30 and 10 and 70返回70
5. or:这是python中的逻辑运算符。“or”返回第一个True值。如果找不到,则返回最后一个。“or”的真值表如下所示。
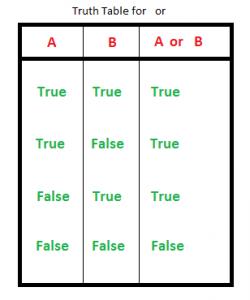
3 or 0返回33 or 10返回30 or 0 or 3 or 10 or 0返回3
6. not:此逻辑运算符求真值的取反。“not”的真值表如下所示。
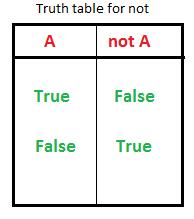
# Python code to demonstrate # True, False, None, and, or , not # showing that None is not equal to 0 # prints False as its false. print (None == 0) # showing objective of None # two None value equated to None # here x and y both are null # hence true x = Noney = Noneprint (x == y) # showing logical operation # or (returns True) print (True or False) # showing logical operation # and (returns False) print (False and True) # showing logical operation # not (returns False) print (not True)
输出:
FalseTrueTrueFalseFalse
7. assert:此函数用于调试目的。通常用于检查代码的正确性。如果一条语句的评估结果为true,则什么也不会发生,但是当它为false时,将引发“ AssertionError”。也可以打印带有错误的消息,并用逗号分隔。
8. break:“break”用于控制循环流。该语句用于中断循环,并在循环后立即将控制权传递给该语句。
9. continue:“continue”也用于控制代码流。关键字跳过循环的当前迭代,但不会结束循环。
10. class:此关键字用于声明用户定义的类。
11. def:此关键字用于声明用户定义的函数。
12. if:是用于决策的控制声明。Truth表达式强制控制进入“if”语句块。
13. else:这是决策的控制声明。False表达式会强制控制进入“else”语句块。
14. elif:这是用于决策的控制声明。这是“ else if”的缩写。
15. del:del用于删除对对象的引用。可以使用del删除任何变量或列表值。
# Python code to demonstrate # del and assert # initialising list a = [1, 2, 3] # printing list before deleting any value print ("The list before deleting any value") print (a) # using del to delete 2nd element of list del a[1] # printing list after deleting 2nd element print ("The list after deleting 2nd element") print (a) # demonstrating use of assert # prints AssertionError assert 5 < 3, "5 is not smaller than 3"
输出:
The list before deleting any value[1, 2, 3]The list after deleting 2nd element[1, 3]
运行时错误:
Traceback (most recent call last): File "9e957ae60b718765ec2376b8ab4225ab.py", line 19, in assert 5<3, "5 is not smaller than 3"AssertionError: 5 is not smaller than 3
16. try:此关键字用于异常处理,用于使用关键字except捕获代码中的错误。除执行块外,是否有其他类型的错误,将检查“ try”块中的代码。
17. except:如上所述,这与“try”一起捕获异常。
18. raise:也用于异常处理以显式引发异常。
19. finally:无论“try”块产生什么结果,始终执行称为“finally”的块。
20. for:此关键字用于控制流和循环。
21. while:具有类似“for”的作用,用于控制流量和循环。
22. pass:它是python中的null语句。遇到这种情况时,不会发生任何事情。这用于防止缩进错误并用作占位符。
23. import:此语句用于将特定模块包含到当前程序中。
24. from:通常与import一起使用,from用于从导入的模块中导入特定功能。
25. as:此关键字用于为导入的模块创建别名。即为导入的模块重新命名。例如,import math as mymath。
26. lambda:此关键字用于生成不允许内部使用语句的内联返回函数。
27. return:此关键字用于从函数返回。
28. yield:该关键字的用法与return语句相同,但用于返回生成器。
29. with:此关键字用于在上下文管理器定义的方法中包装代码块的执行。此关键字在日常编程中不常用。
30. in:此关键字用于检查容器是否包含值。此关键字还用于遍历容器。
31. is:此关键字用于测试对象身份,即检查两个对象是否位于相同的内存位置。
# Python code to demonstrate working of # in and is # using "in" to check if 's' in 'geeksforgeeks': print ("s is part of geeksforgeeks") else : print ("s is not part of geeksforgeeks") # using "in" to loop through for i in 'geeksforgeeks': print (i,end=" ") print ("") # using is to check object identity # string is immutable( cannot be changed once alloted) # hence occupy same memory location print (' ' is ' ') # using is to check object identity # dictionary is mutable( can be changed once alloted) # hence occupy different memory location print ({} is {})
输出:
s is part of geeksforgeeksg e e k s f o r g e e k s TrueFalse
32. global:此关键字用于将函数内部的变量定义为全局范围。
33. non-local:此关键字的工作方式类似于global,但不是全局的,此关键字声明一个变量,以在嵌套函数的情况下指向外部封闭函数的变量。
# Python code to demonstrate working of # global and non local #initializing variable globally a = 10 # used to read the variable def read(): print (a) # changing the value of globally defined variable def mod1(): global a a = 5 # changing value of only local variable def mod2(): a = 15 # reading initial value of a # prints 10 read() # calling mod 1 function to modify value # modifies value of global a to 5 mod1() # reading modified value # prints 5 read() # calling mod 2 function to modify value # modifies value of local a to 15, doesn't effect global value mod2() # reading modified value # again prints 5 read() # demonstrating non local # inner loop changing the value of outer a # prints 10 print ("Value of a using nonlocal is : ",end="") def outer(): a = 5 def inner(): nonlocal a a = 10 inner() print (a) outer() # demonstrating without non local # inner loop not changing the value of outer a # prints 5 print ("Value of a without using nonlocal is : ",end="") def outer(): a = 5 def inner(): a = 10 inner() print (a) outer()
输出:
1055Value of a using nonlocal is : 10Value of a without using nonlocal is : 5