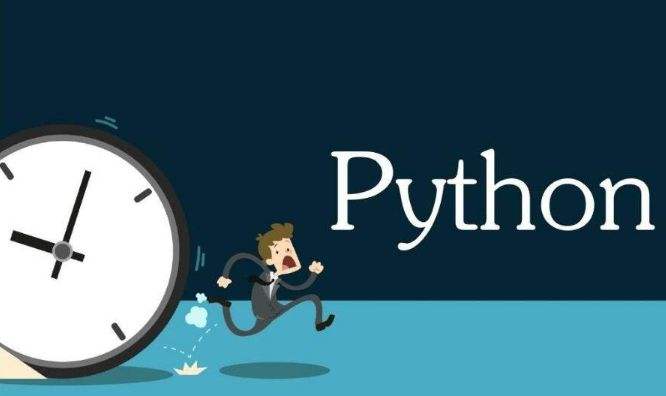
for循环在python中的重要性毋庸置疑,可是,我们真的把所有for循环的知识点都理解透了么?试试看以下内容:
- for 循环的基本格式
for
iterable是可迭代对象,包括字符串,列表,元组,字典,集合,迭代器,列表生成式,生成器。itervar是可迭代对象每次调用__next__方法返回的一个元素。else子句是可选。
2. 迭代字符串
output_list = []
# 迭代字符串 - 最简单最常用版本
this_str = 'it is a string'
for char in this_str:
output_list.append(char)
print(output_list) #out:['i', 't', ' ', 'i', 's', ' ', 'a', ' ', 's', 't', 'r', 'i', 'n', 'g']
#使用分片和下标迭代字符串
output_list.clear()
for index in range(len(this_str)):
char = this_str[index:index+1:1]
output_list.append(char)
print(output_list) #out:['i', 't', ' ', 'i', 's', ' ', 'a', ' ', 's', 't', 'r', 'i', 'n', 'g']
#改进版本,采用enumerate函数,生成下标
output_list.clear()
for index, char in enumerate(this_str):
output_list.append(this_str[index]) #等效:output_list.append(char)
print(output_list)
3. 迭代列表
# 迭代列表
output_list = []
this_list = ['a', 'b', 'c', 1, 2, 3]
for el in this_list:
output_list.append(el)
print(output_list) #out:['a', 'b', 'c', 1, 2, 3]
4. 迭代元组
# 迭代字元组
output_list = []
this_tuple = ('a', 'b', 'c', 1, 2, 3)
for el in this_tuple:
output_list.append(el)
print(output_list) #out:['a', 'b', 'c', 1, 2, 3]
5. 迭代字典
# 迭代字典。使用items方法返回key和value
this_dict = {0: 'a', 1: 'b', 2: 'c', 3: '1', 4: '2', 5: '3'}
for key, value in this_dict.items():
print('%s:%s' % (key, value))
#获取key 使用keys方法获取key
for key in this_dict.keys():
print('key is:%s' % key)
#获取value 使用values方法获取value
for value in this_dict.values():
print('value is:%s' % value)
6. 迭代集合
# 迭代集合
output_list = []
this_set = {'a', 'b', 'c', 1, 2, 3}
for el in this_set:
output_list.append(el)
print(output_list) #out:[1, 2, 3, 'b', 'c', 'a']
7. 迭代列表生成式,通常用于从一个列表推导出另外一个列表(筛选,操作)
# 迭代列表生成式 从一个列表推导到另外一个列表,所有元素都*2
this_list = ['a', 'b', 'c', 1, 2, 3]
output_list = []
for el in [x * 2 for x in this_list]:
output_list.append(el)
print(output_list) #out:'aa', 'bb', 'cc', 2, 4, 6]
# 迭代列表生成式 从一个列表筛选到另外一个列表,只要是字符串的元素
output_list.clear()
for el in [x for x in this_list if type(x) is str]:
output_list.append(el)
print(output_list) #out:['a', 'b', 'c']
8. 迭代生成器
# 迭代生成器 方法一 使用类似列表生成器的方式
output_list = []
for el in (num * 2 for num in range(10)):
output_list.append(el)
print(output_list) #out:[0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
# 迭代生成器 方法二 自定义一个函数并且用yield返回
def get_number(max_num: int):
for num in range(max_num):
yield num * 2
output_list = []
for el in get_number(10):
output_list.append(el)
print(output_list) # out:[0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
9. 如何在迭代过程中移除元素(列表和字典)
我们先看看python手册是怎么说在迭代中移除元素的:
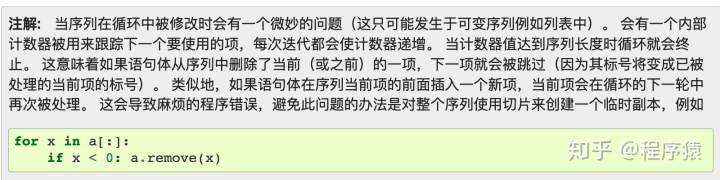
我们试试看:
this_list = ['a', 'b', 'c', 1, 2, 3]
#迭代中删除列表元素 和期待的输出结果不同!!
for el in this_list:
if type(el) is str:
this_list.remove(el) #out: ['b', 1, 2, 3]
在迭代列表的过程中,移除所有的字符串类型的元素,期望的输出结果是[1,2,3],为什么是 ['b', 1, 2, 3]?因为,移除了元素以后,就破坏了计数器,在列表中也是通过计数器来索引的。所以我们可以这么做:
this_list = ['a', 'b', 'c', 1, 2, 3]
#使用副本来进行迭代,用原本来进行删除
for el in this_list[:]: #this_list[:]是用切片生成this_list的一个副本
if type(el) is str:
this_list.remove(el) #out: [1, 2, 3]
再试试字典:
this_dict = {0: 'a', 1: 'b', 2: 'c', 3: '1', 4: '2', 5: '3', 6: 1, 7: 2}
for key, value in this_dict.items():
if type(value) is str:
del(this_dict[key]) #RuntimeError: dictionary changed size during iteration
可以这样写,遍历字典的key列表,同时根据key来删除元素
this_dict = {0: 'a', 1: 'b', 2: 'c', 3: '1', 4: '2', 5: '3', 6: 1, 7: 2}
for key in list(this_dict.keys()):
if type(this_dict[key]) is str:
del(this_dict[key]) #output: {6: 1, 7: 2}
希望这文章能帮到你。