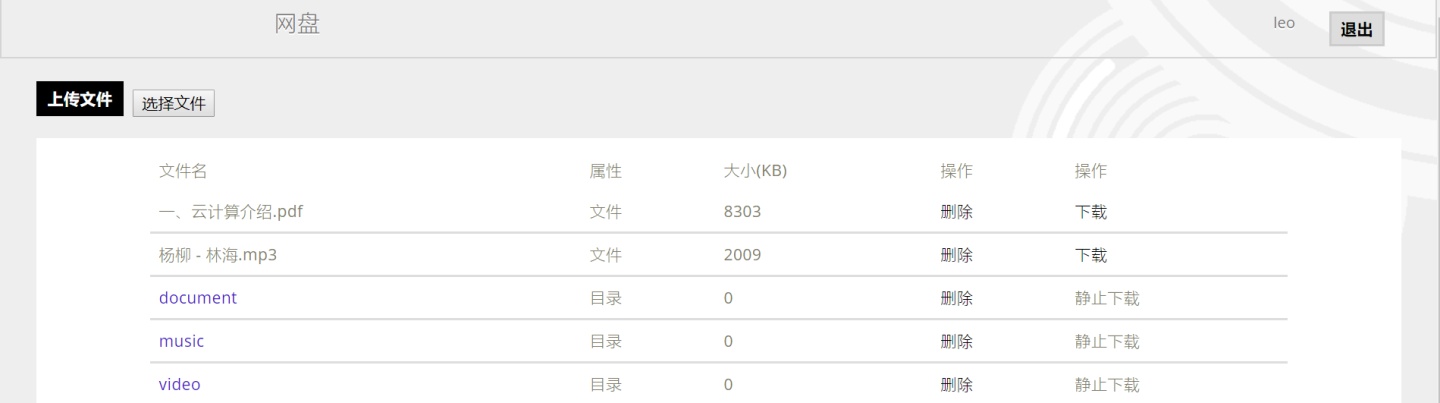
****************************************【展示】***************************************
①主页展示:
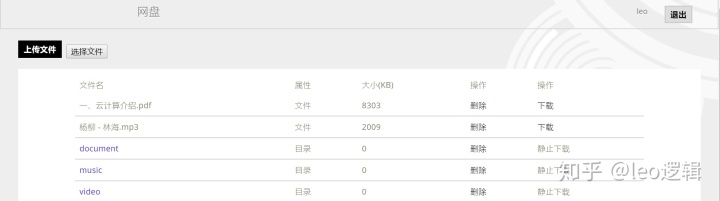
②二级文件夹_music展示

③二级文件夹_video展示

④删除文件后的效果
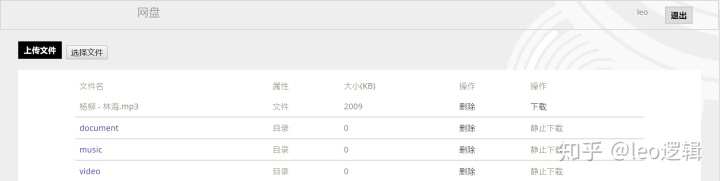
⑤下载文件的效果图
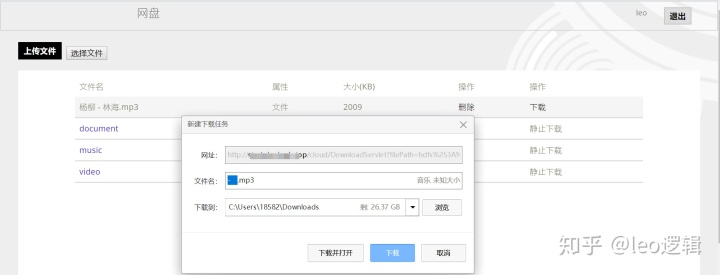
⑥登录界面
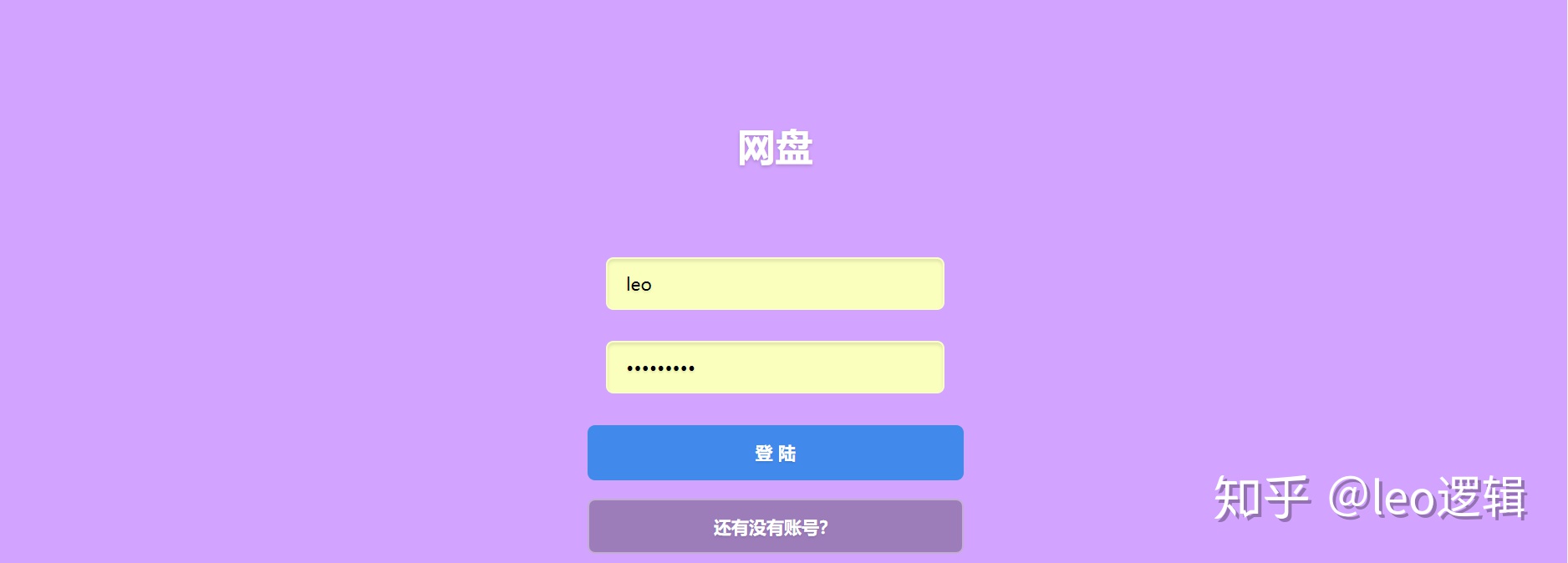
⑦注册页面
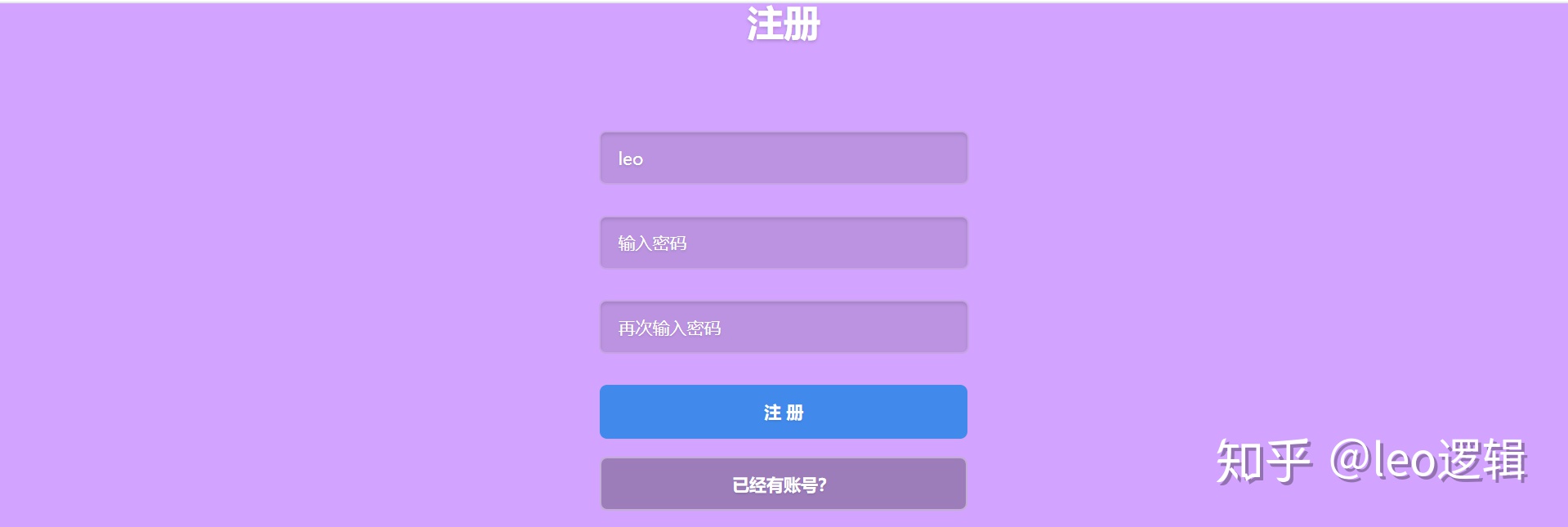
************************************【项目结构图】************************************
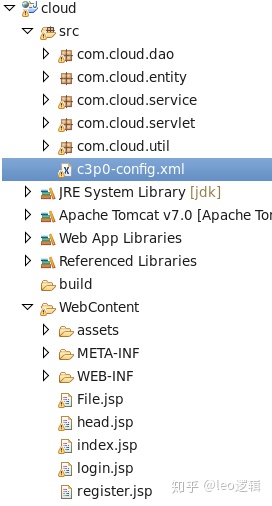
免密部分:
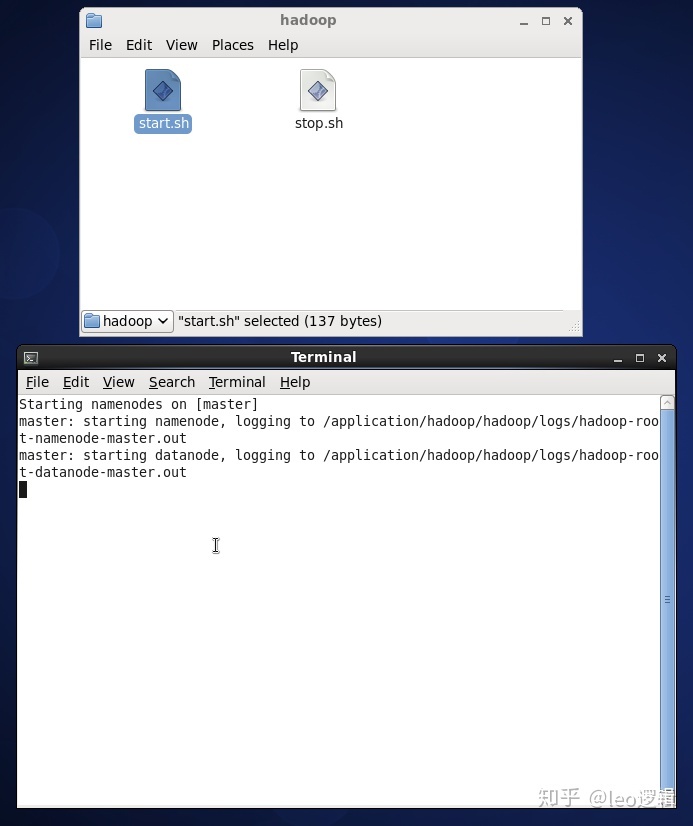
******************************【包下面的文件部分】**********************************
dao层:
①HDFSdao
package com.cloud.dao;
import java.io.IOException;
import java.net.URI;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileStatus;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.mapred.JobConf;
public class HdfsDAO {
//HDFS访问地址
private static final String HDFS = "hdfs://127.0.0.1:9000";
public static String getHdfs() {
return HDFS;
}
public HdfsDAO(Configuration conf) {
this(HDFS, conf);
}
public HdfsDAO(String hdfs, Configuration conf) {
this.hdfsPath = hdfs;
this.conf = conf;
}
//hdfs路径
private String hdfsPath;
//Hadoop系统配置
private Configuration conf;
//启动函数
public static void main(String[] args) throws IOException {
JobConf conf = config();
HdfsDAO hdfs = new HdfsDAO(conf);
hdfs.mkdirs("/Tom");
//hdfs.copyFile("C:files", "/wgc/");
//hdfs.ls("hdfs://192.168.1.104:9000/wgc/files");
//hdfs.rmr("/wgc/files");
//hdfs.download("/wgc/(3)windows下hadoop+eclipse环境搭建.docx", "c:");
//System.out.println("success!");
}
//加载Hadoop配置文件
public static JobConf config(){
JobConf conf = new JobConf(HdfsDAO.class);
conf.setJobName("HdfsDAO");
conf.addResource("classpath:/home/leo/software/hadoop/hadoop/etc/hadoop/core-site.xml");
conf.addResource("classpath:/home/leo/software/hadoop/hadoop/etc/hadoop/hdfs-site.xml");
conf.addResource("classpath:/home/leo/software/hadoop/hadoop/etc/hadoop/mapred-site.xml");
return conf;
}
//在根目录下创建文件夹
public void mkdirs(String folder) throws IOException {
Path path = new Path(folder);
FileSystem fs = FileSystem.get(URI.create(hdfsPath), conf);
if (!fs.exists(path)) {
fs.mkdirs(path);
//System.out.println("Create: " + folder);
}
fs.close();
}
//某个文件夹的文件列表
public FileStatus[] ls(String folder) throws IOException {
Path path = new Path(folder);
FileSystem fs = FileSystem.get(URI.create(hdfsPath), conf);
FileStatus[] list = fs.listStatus(path);
// System.out.println("ls: " + folder);
//System.out.println("==========================================================");
if(list != null)
for (FileStatus f : list) {
//System.out.printf("name: %s, folder: %s, size: %dn", f.getPath(), f.isDir(), f.getLen());
System.out.printf("%s, folder: %s, 大小: %dKn", f.getPath().getName(), (f.isDir()?"目录":"文件"), f.getLen()/1024);
}
// System.out.println("===========================================");
fs.close();
return list;
}
public void copyFile(String local, String remote) throws IOException {
FileSystem fs = FileSystem.get(URI.create(hdfsPath), conf);
//remote---/用户/用户下的文件或文件夹
fs.copyFromLocalFile(new Path(local), new Path(remote));
//System.out.println("copy from: " + local + " to " + remote);
fs.close();
}
//删除文件或文件夹
public void rmr(String folder) throws IOException {
Path path = new Path(folder);
FileSystem fs = FileSystem.get(URI.create(hdfsPath), conf);
fs.deleteOnExit(path);
//System.out.println("Delete: " + folder);
fs.close();
}
//下载文件到本地系统
public void download(String remote, String local) throws IOException {
Path path = new Path(remote);
FileSystem fs = FileSystem.get(URI.create(hdfsPath), conf);
fs.copyToLocalFile(path, new Path(local));
System.out.println("download: from" + remote + " to " + local);
fs.close();
}
}
②UserDao
package com.cloud.dao;
import java.sql.SQLException;
import java.util.List;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import com.cloud.entity.User;
import com.cloud.util.C3p0UtilsConfig;
public class UserDao {
//登录判断
public User findByNameAndPassWord(User u) throws SQLException {
QueryRunner qr = new QueryRunner(C3p0UtilsConfig.getDataSource());
String sql="select * from user where uname=? and upassword=?";
return qr.query(sql,
new BeanHandler<User>(User.class),
u.getUname(),
u.getUpassword()
);
}
//用户注册
public int registerByNameAndPassWord(User u) throws SQLException {
QueryRunner qr = new QueryRunner(C3p0UtilsConfig.getDataSource());
int i = qr.update(
"insert into user values(null,?,?)",
u.getUname(),
u.getUpassword()
);
return i;
}
}
util层:
package com.cloud.util;
import java.sql.Connection;
import java.sql.SQLException;
import org.apache.commons.dbutils.QueryRunner;
import com.mchange.v2.c3p0.ComboPooledDataSource;
/**
* @author xican.leo
* @date 2019年4月26日
* @version 1.0
*/
public class C3p0UtilsConfig {
//这个类是自己写的工具类在这里创建了一个连接池对象所有的连接都存在对象里这个对象就是连接池
private static ComboPooledDataSource dataSource = null;
static {
//创建一个连接池这里的创建的时候在构造函数里传入一个连接池名字它会自动的根据名字去找配置文件
dataSource = new ComboPooledDataSource("c3p0-config");
}
public static ComboPooledDataSource getDataSource(){
//返回这个连接池
return dataSource;
}
/**
*
* @return 返回一个从数据库里拿出来的连接
* @throws SQLException
*/
public static Connection getConnection() throws SQLException {
//返回一条连接
return dataSource.getConnection();
}
// public static void main(String[] args) throws SQLException {
// //测试数据库连接
// QueryRunner qr = new QueryRunner(C3p0UtilsConfig.getDataSource());
// qr.update("update stu set sname = ? where sid = 1","张苏");
// }
}
使用c3p0-config.xml
<?xml version="1.0" encoding="UTF-8"?>
<c3p0-config>
<named-config name = "c3p0-config" >
<property name="driverClass">com.mysql.jdbc.Driver</property>
<property name="jdbcUrl">jdbc:mysql://127.0.0.1:3306/cloud?useUnicode=true&characterEncoding=utf-8</property>
<property name="user">root</property>
<property name="password">123456</property>
<property name="checkoutTimeout">30000</property>
<property name="idleConnectionTestPeriod">30</property>
<property name="initialPoolSize">10</property>
<property name="maxIdleTime">30</property>
<property name="maxPoolSize">100</property>
<property name="minPoolSize">10</property>
<property name="maxStatements">200</property>
</named-config>
</c3p0-config>
entity层:
package com.cloud.entity;
//建立一个用户实体
public class User {
private int uid;
private String uname;
private String upassword;
public int getUid() {
return uid;
}
public void setUid(int uid) {
this.uid = uid;
}
public String getUname() {
return uname;
}
public void setUname(String uname) {
this.uname = uname;
}
public String getUpassword() {
return upassword;
}
public void setUpassword(String upassword) {
this.upassword = upassword;
}
@Override
public String toString() {
return "User [uid=" + uid + ", uname=" + uname + ", upassword=" + upassword + "]";
}
}
servlet层:
①UserServlet
package com.cloud.servlet;
import java.io.IOException;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import com.cloud.entity.User;
import com.cloud.service.UserService;
@WebServlet("/UserServlet")
public class UserServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String type = request.getParameter("type");
if(type != null && type.equals("login")){
try {
String username = request.getParameter("username");
String password = request.getParameter("password");
//将数据封装到实体中
User u =new User();
u.setUname(username);
u.setUpassword(password);
//向service层拿数据
UserService us = new UserService();
boolean flog=us.findByNameAndPassWord(u);
if(flog) {
//建立一个session对象
HttpSession session = request.getSession();
//保存用户名到session
session.setAttribute("user",u);
//请求转发到index.jsp
request.getRequestDispatcher("ShowIndexServlet").forward(request, response);
}else {
//1.将User保存在域对象
request.setAttribute("msg", "账号或密码错误请重新登录");
//2.请求转发到index.jsp
request.getRequestDispatcher("/login.jsp").forward(request, response);
}
} catch (SQLException e) {
e.printStackTrace();
}
}else {
try {
String username = request.getParameter("username");
String password = request.getParameter("password");
//将数据封装到实体中
User u =new User();
u.setUname(username);
u.setUpassword(password);
//向service层拿数据
UserService us = new UserService();
boolean flog=us.registerByNameAndPassWord(u);
if(flog){
//1.将msg保存在域对象
request.setAttribute("msg", username+",您已注册成功,请登录!");
//2.请求转发到index.jsp
request.getRequestDispatcher("/login.jsp").forward(request, response);
}else {
//1.将msg保存在域对象
request.setAttribute("msg", "注册失败,请重新注册");
//2.请求转发到index.jsp
request.getRequestDispatcher("/register.jsp").forward(request, response);
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
②UploadServlet
package com.cloud.servlet;
import java.io.File;
import java.io.IOException;
import java.util.Iterator;
import java.util.List;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
import org.apache.hadoop.fs.FileStatus;
import org.apache.hadoop.mapred.JobConf;
import com.cloud.dao.HdfsDAO;
@WebServlet("/UploadServlet")
public class UploadServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doPost(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String url = request.getParameter("url"); //获取文件夹路径
File file;
int maxFileSize = 50 * 1024 *1024; //50M
int maxMemSize = 50 * 1024 *1024; //50M
ServletContext context = getServletContext();
String filePath = context.getInitParameter("file-upload");
System.out.println("source file path:"+filePath+"");
// 验证上传内容了类型
String contentType = request.getContentType();
if ((contentType.indexOf("multipart/form-data") >= 0)) {
DiskFileItemFactory factory = new DiskFileItemFactory();
// 设置内存中存储文件的最大值
factory.setSizeThreshold(maxMemSize);
// 本地存储的数据大于 maxMemSize.
factory.setRepository(new File("c:temp"));
// 创建一个新的文件上传处理程序
ServletFileUpload upload = new ServletFileUpload(factory);
// 设置最大上传的文件大小
upload.setSizeMax( maxFileSize );
try{
// 解析获取的文件
List fileItems = upload.parseRequest(request);
// 处理上传的文件
Iterator i = fileItems.iterator();
System.out.println("begin to upload file to tomcat server</p>");
while ( i.hasNext () )
{
FileItem fi = (FileItem)i.next();
if ( !fi.isFormField () )
{
// 获取上传文件的参数
String fieldName = fi.getFieldName();
System.out.println(fieldName+"11111111111111");
String fileName = fi.getName();
System.out.println(filePath+"333333333333");
System.out.println(fileName+"222222222222222");
String fn = fileName.substring(fileName.lastIndexOf("")+1);
System.out.println("<br>"+fn+"<br>");
boolean isInMemory = fi.isInMemory();
long sizeInBytes = fi.getSize();
// 写入文件
if( fileName.lastIndexOf("") >= 0 ){
file = new File( filePath ,
fileName.substring( fileName.lastIndexOf(""))) ;
//out.println("filename"+fileName.substring( fileName.lastIndexOf(""))+"||||||");
}else{
file = new File( filePath ,
fileName.substring(fileName.lastIndexOf("")+1)) ;
}
fi.write( file ) ;
System.out.println("upload file to tomcat server success!");
System.out.println("begin to upload file to hadoop hdfs</p>");
String name = filePath + File.separator + fileName;
System.out.println(name+"55555555555");
//将tomcat上的文件上传到hadoop上
JobConf conf = HdfsDAO.config();
HdfsDAO hdfs = new HdfsDAO(conf);
String HDFSLoc="/";
if(url != null) {
HDFSLoc=HDFSLoc+url;
}
hdfs.copyFile(name, HDFSLoc);
System.out.println("upload file to hadoop hdfs success!");
if(url != null) {//获取hdfs文件夹路径,转跳上传所在页面提升用户体验
String filePathFile=HdfsDAO.getHdfs()+"/"+url;
request.setAttribute("fileP", filePathFile);
request.getRequestDispatcher("FileServlet").forward(request, response);
}else {
request.getRequestDispatcher("ShowIndexServlet").forward(request, response);
}
}
}
}catch(Exception ex) {
System.out.println(ex);
}
}else{
System.out.println("<p>No file uploaded</p>");
}
}
}
③ShowIndexServlet
package com.cloud.servlet;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.hadoop.fs.FileStatus;
import org.apache.hadoop.mapred.JobConf;
import com.cloud.dao.HdfsDAO;
/**
* Servlet implementation class ShowIndexServlet
*/
@WebServlet("/ShowIndexServlet")
public class ShowIndexServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
JobConf conf = HdfsDAO.config();
HdfsDAO hdfs = new HdfsDAO(conf);
FileStatus[] documentList = hdfs.ls("/");
request.setAttribute("documentList",documentList);
System.out.println("得到list数据"+documentList);
request.getRequestDispatcher("index.jsp").forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
④FileServlet
package com.cloud.servlet;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.hadoop.fs.FileStatus;
import org.apache.hadoop.mapred.JobConf;
import com.cloud.dao.HdfsDAO;
@WebServlet("/FileServlet")
public class FileServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String filePath=request.getParameter("filePath");
if (filePath !=null){
filePath = new String(request.getParameter("filePath").getBytes("ISO-8859-1"),"GB2312");
}else {
filePath = request.getAttribute("fileP").toString();
}
JobConf conf = HdfsDAO.config();
HdfsDAO hdfs = new HdfsDAO(conf);
FileStatus[] documentList = hdfs.ls(filePath);
int index = filePath.lastIndexOf("/");
String after = filePath.substring(index + 1);
request.setAttribute("urlNOW",after);
request.setAttribute("documentList",documentList);
request.getRequestDispatcher("File.jsp").forward(request,response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doGet(request, response);
}
}
⑤DownloadServlet
package com.cloud.servlet;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.hadoop.mapred.JobConf;
import org.apache.tomcat.util.http.fileupload.IOUtils;
import java.net.*;
import com.cloud.dao.HdfsDAO;
/**
* Servlet implementation class DownloadServlet
*/
@WebServlet("/DownloadServlet")
public class DownloadServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String filePath =request.getParameter("filePath");
String filename =request.getParameter("filename");//获取文件名
filePath = java.net.URLDecoder.decode(filePath, "UTF-8");
filePath =java.net.URLDecoder.decode(filePath,"UTF-8");
System.out.println(filePath);
String locPath = "/home/leo/Downloads";
JobConf conf = HdfsDAO.config();
HdfsDAO hdfs = new HdfsDAO(conf);
hdfs.download(filePath, locPath);
//---------------------------------------------------------------------
//获取服务器文件
locPath=locPath+"/"+filename;
File file = new File(locPath);
InputStream ins = new FileInputStream(file);
/* 设置文件ContentType类型,这样设置,会自动判断下载文件类型 */
response.setContentType("multipart/form-data");
/* 设置文件头:最后一个参数是设置下载文件名 */
response.setHeader("Content-Disposition", "attachment;filename="+file.getName());
try{
OutputStream os = response.getOutputStream();
byte[] b = new byte[1024];
int len;
while((len = ins.read(b)) > 0){
os.write(b,0,len);
}
os.flush();
os.close();
ins.close();
}catch (IOException ioe){
ioe.printStackTrace();
}
// request.getRequestDispatcher("ShowIndexServlet").forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
⑥DelFileServlet
package com.cloud.servlet;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.URLDecoder;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.hadoop.mapred.JobConf;
import com.cloud.dao.HdfsDAO;
/**
* Servlet implementation class DelServlet
*/
@WebServlet("/DelFileServlet")
public class DelFileServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String url = request.getParameter("url"); //获取文件夹路径
String filePath =request.getParameter("filePath");
filePath = java.net.URLDecoder.decode(filePath, "UTF-8");
filePath =java.net.URLDecoder.decode(filePath,"UTF-8");
System.out.println("文件名为!!!!!!!!!!"+filePath);
JobConf conf = HdfsDAO.config();
HdfsDAO hdfs = new HdfsDAO(conf);
hdfs.rmr(filePath);
System.out.println("del file to hadoop hdfs success!");
if(url != null) {//获取hdfs文件夹路径,转跳上传所在页面提升用户体验
String filePathFile=HdfsDAO.getHdfs()+"/"+url;
request.setAttribute("fileP", filePathFile);
request.getRequestDispatcher("FileServlet").forward(request, response);
}else {
request.getRequestDispatcher("ShowIndexServlet").forward(request, response);
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
service层
package com.cloud.service;
import java.sql.SQLException;
import java.util.List;
import com.cloud.dao.UserDao;
import com.cloud.entity.User;
public class UserService{
public boolean findByNameAndPassWord(User u) throws SQLException {
UserDao ud = new UserDao();
User user = ud.findByNameAndPassWord(u);
if(user != null) {
return true;
}else {
return false;
}
}
public boolean registerByNameAndPassWord(User u) throws SQLException {
UserDao ud = new UserDao();
int i = ud.registerByNameAndPassWord(u);
if(i != 0) {
return true;
}else {
return false;
}
}
}
******************************【jsp部分】**********************************
①register.jsp
<%@ page contentType="text/html; charset=utf-8"%>
<%
String basePath = request.getScheme()+":"+"//"+request.getServerName()+":"+request.getServerPort()
+request.getServletContext().getContextPath()+"/";
%>
<!DOCTYPE html>
<html>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<script type="text/javascript">
function checkRegister() {
if (document.register.inputname.value == "") {
alert("用户名不能为空.");
return false;
}else{
if (document.register.inputpass.value == "") {
alert("密码不能为空.");
return false;
}else {
if (document.register.surepass.value == "") {
alert("确认密码不能为空.");
return false;
}else {
if(document.register.inputpass.value != document.register.surepass.value){
alert("两次密码不一致。");
return false;
}
}
}
}
return true;
}
</script>
<title>注册</title>
<link rel="stylesheet" href="assets/css/login.css">
<body>
<div>
<h1>注册</h1>
<div class="connect">
<p>信息注册</p>
</div>
<form action="<%=basePath%>UserServlet" method="post" id="registerForm" onsubmit="return checkRegister()" name="register">
<div>
<input type="text" name="username" id="inputname" class="username" placeholder="您的用户名" autocomplete="off"/>
</div>
<div>
<input type="password" name="password" id="inputpass" class="password" placeholder="输入密码" />
</div>
<div>
<input type="password" name="surepass" id="surepass" class="surepass" placeholder="再次输入密码" />
</div>
<button id="submit" type="submit">注 册</button>
</form>
<a href="login.jsp">
<button type="button" class="register-tis">已经有账号?</button>
</a>
</div>
</body>
</html>
②login.jsp
<%@ page contentType="text/html; charset=utf-8"%>
<%
String basePath = request.getScheme()+":"+"//"+request.getServerName()+":"+request.getServerPort()
+request.getServletContext().getContextPath()+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<script type="text/javascript">
function checkUser() {
if (document.login.inputname.value == "") {
alert("用户名不能为空.");
return false;
}
if (document.login.inputpass.value == "") {
alert("密码不能为空.");
return false;
}
return true;
}
</script>
<title>云盘</title>
<link rel="stylesheet" href="assets/css/style.css">
<body
style="background-color: #D3A4FF; background-position: center; background-repeat: repeat-y">
<div class="login-container">
<h1>网盘</h1>
<div class="connect">
<p>www.shiyanbar.com</p>
</div>
<div>${msg}</div>
<form action="<%=basePath%>UserServlet?type=login" method="post" id="loginForm" name="login"
onsubmit="return checkUser()">
<div>
<input type="text" id="inputname" name="username" class="username"
placeholder="用户名" autocomplete="off" />
</div>
<div>
<input type="password" id="inputpass" name="password"
class="password" placeholder="密码" oncontextmenu="return false"
onpaste="return false"/>
</div>
<button id="submit" type="submit">登 陆</button>
</form>
<a href="register.jsp">
<button type="button" class="register-tis">还有没有账号?</button>
</a>
</div>
<div
style="text-align: center; margin: 50px 0; font: normal 14px/24px 'MicroSoft YaHei';">
<p>适用浏览器:360、FireFox、Chrome、Safari、Opera、傲游、搜狗、世界之窗. 不支持IE8及以下浏览器。</p>
<p>
来源:<a href="http://sc.chinaz.com/" target="_blank">教育</a>
</p>
</div>
</body>
</html>
③index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<%@ include file="head.jsp"%>
<%@page import="org.apache.hadoop.fs.FileStatus"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
String basePath = request.getScheme()+":"+"//"+request.getServerName()+":"+request.getServerPort()
+request.getServletContext().getContextPath()+"/";
%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<body style="text-align: center; margin-bottom: 100px;">
<div class="navbar">
<div class="navbar-inner">
<a class="brand" href="#" style="margin-left: 200px;" style="color:#000000">网盘</a>
<ul class="nav" style="line-height: 50px; float: right;">
<c:choose>
<c:when test="${empty user}">
<script type="text/javascript" language="javascript">
alert("您还没有登录,请登录...");
top.location.href="<%=basePath%>login.jsp";
</script>
</c:when>
<c:otherwise>
<li><a style="color:#000000 size=40">${user.uname}</a></li>
<li><a href="login.jsp"><input type="button" value="退出"></a></li>
</c:otherwise>
</c:choose>
</ul>
</div>
</div>
<div
style="margin: 0px auto; text-align: left; width: 1200px; height: 50px;">
<form class="form-inline" method="POST" enctype="MULTIPART/FORM-DATA"
action="UploadServlet">
<div style="line-height: 50px; float: left;">
<input style="background-color:#000000" type="submit" name="submit" value="上传文件">
</div>
<div style="line-height: 50px; float: left;">
<input type="file" name="file1" size="30"/>
</div>
</form>
</div>
<div
style="margin: 0px auto; width: 1200px; height: 500px; background: #fff">
<table class="table table-hover"
style="width: 1000px; margin-left: 100px;">
<tr>
<td>文件名</td>
<td>属性</td>
<td>大小(KB)</td>
<td>操作</td>
<td>操作</td>
</tr>
<%
FileStatus[] list = (FileStatus[])request.getAttribute("documentList");
String name = (String)request.getAttribute("username");
if(list != null)
for (int i=0; i<list.length; i++) {
%>
<tr style="border-bottom: 2px solid #ddd">
<%
if(list[i].isDir())//DocumentServlet
{
out.print("<td><a href="FileServlet?filePath="+list[i].getPath()+"">"+list[i].getPath().getName()+"</a></td>");
}else{
out.print("<td>"+list[i].getPath().getName()+"</td>");
}
%>
<td><%= (list[i].isDir()?"目录":"文件") %></td>
<td><%= list[i].getLen()/1024%></td>
<td ><a style="color:#000000"
href="DelFileServlet?filePath=<%=java.net.URLEncoder.encode(java.net.URLEncoder.encode(list[i].getPath().toString(),"UTF-8"),"UTF-8")%>">删除</a>
</td>
<c:choose>
<c:when test="<%=list[i].isDir()%>">
<td >
静止下载
</td>
</c:when>
<c:otherwise>
<td ><a style="color:#000000"
href="DownloadServlet?filePath=<%=java.net.URLEncoder.encode(java.net.URLEncoder.encode(list[i].getPath().toString(),"UTF-8"),"UTF-8")%>&filename=<%=list[i].getPath().getName()%>">下载</a>
</td>
</c:otherwise>
</c:choose>
</tr>
<%
}
%>
</table>
</div>
</body>
</body>
</html>
④head.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<link rel="stylesheet" type="text/css" href="assets/css/bootmetro.css">
<link rel="stylesheet" type="text/css" href="assets/css/bootmetro-responsive.css">
<link rel="stylesheet" type="text/css" href="assets/css/bootmetro-icons.css">
<link rel="stylesheet" type="text/css" href="assets/css/bootmetro-ui-light.css">
<link rel="stylesheet" type="text/css" href="assets/css/datepicker.css">
<!-- these two css are to use only for documentation -->
<link rel="stylesheet" type="text/css" href="assets/css/site.css">
<!-- Le fav and touch icons -->
<link rel="shortcut icon" href="assets/ico/favicon.ico">
<link rel="apple-touch-icon-precomposed" sizes="144x144" href="assets/ico/apple-touch-icon-144-precomposed.png">
<link rel="apple-touch-icon-precomposed" sizes="114x114" href="assets/ico/apple-touch-icon-114-precomposed.png">
<link rel="apple-touch-icon-precomposed" sizes="72x72" href="assets/ico/apple-touch-icon-72-precomposed.png">
<link rel="apple-touch-icon-precomposed" href="assets/ico/apple-touch-icon-57-precomposed.png">
<!-- All JavaScript at the bottom, except for Modernizr and Respond.
Modernizr enables HTML5 elements & feature detects; Respond is a polyfill for min/max-width CSS3 Media Queries
For optimal performance, use a custom Modernizr build: www.modernizr.com/download/ -->
<script src="assets/js/modernizr-2.6.2.min.js"></script>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
</html>
⑤File.jsp
<%@ include file="head.jsp"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@page import="org.apache.hadoop.fs.FileStatus"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
String basePath = request.getScheme()+":"+"//"+request.getServerName()+":"+request.getServerPort()
+request.getServletContext().getContextPath()+"/";
%>
<body style="text-align:center;margin-bottom:100px;">
<div class="navbar" >
<div class="navbar-inner">
<a class="brand" href="<%=basePath%>ShowIndexServlet" style="margin-left:200px;">网盘</a>
<ul class="nav">
<li class="active"><a href="">${urlNOW}</a></li>
</ul>
</div>
</div>
<div style="margin:0px auto; text-align:left;width:1200px; height:50px;">
<form class="form-inline" method="POST" enctype="MULTIPART/FORM-DATA" action="UploadServlet?url=${urlNOW}" >
<div style="line-height:50px;float:left;">
<input type="submit" name="submit" value="上传文件" />
</div>
<div style="line-height:50px;float:left;">
<input type="file" name="file1" size="30"/>
</div>
</form>
</div>
<div style="margin:0px auto; width:1200px;height:500px; background:#fff">
<table class="table table-hover" style="width:1000px;margin-left:100px;">
<tr><td>文件名</td><td>属性</td><td>大小(KB)</td><td>操作</td><td>操作</td></tr>
<%
FileStatus[] list = (FileStatus[])request.getAttribute("documentList");
if(list != null)
for (int i=0; i<list.length; i++) {
%>
<tr style=" border-bottom:2px solid #ddd">
<%
if(list[i].isDir())
{
out.print("<td><a href="DocumentServlet?filePath="+list[i].getPath()+"">"+list[i].getPath().getName()+"</a></td>");
}else{
out.print("<td>"+list[i].getPath().getName()+"</td>");
}
%>
<td><%= (list[i].isDir()?"目录":"文件") %></td>
<td><%= list[i].getLen()/1024%></td>
<td ><a style="color:#000000"
href="DelFileServlet?&filePath=<%=java.net.URLEncoder.encode(java.net.URLEncoder.encode(list[i].getPath().toString(),"UTF-8"),"UTF-8")%>">删除</a>
</td>
<c:choose>
<c:when test="<%=list[i].isDir()%>">
<td >
静止下载
</td>
</c:when>
<c:otherwise>
<td ><a style="color:#000000"
href="DownloadServlet?filePath=<%=java.net.URLEncoder.encode(java.net.URLEncoder.encode(list[i].getPath().toString(),"UTF-8"),"UTF-8")%>&filename=<%=list[i].getPath().getName()%>">下载</a>
</td>
</c:otherwise>
</c:choose>
</tr>
<%
}
%>
</table>
</div>
</body>
</html>
⑥web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name>MyCloudPan</display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<context-param>
<param-name>file-upload</param-name>
<param-value>/home/leo/Desktop/file/input</param-value>
</context-param>
</web-app>