1 数据库的创建
打开已经安装好的数据库,如下流程:
step 1:单击 “MySQL Command Line Client-Unicode”
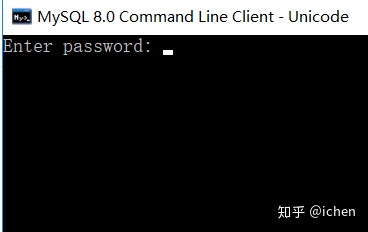
step 2:输入密码,进入数据库
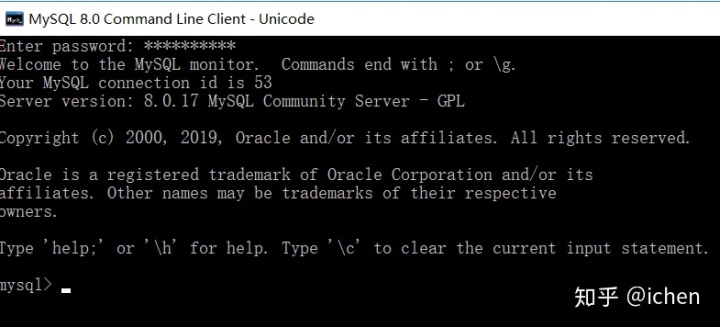
step 3:建立一个简单的数据库,在这里建立一个名称为“mysql_test”的数据库,如下所示:

不过注意此时的数据库里面还没有任何东西。
step 4:在数据库创建一个数据表,如下所示:
use mysql_test;
create table student(
id int not null auto_increment,
StuName varchar(5) not null,
StoNo varchar(14) not null,
Age varchar(3) not null,
primary id
)engine = InnoDB default charset utf=8;
describe student;
insert into student(StuName,StoNo,Age)
values
('张三',3120150802200,18),
('李四',3120150802201,18),
('王麻子',3120150802202,18),
('百度',3120150802203,19),
('阿里',3120150802204,19),
('腾讯',3120150802205,20);
select * from student;
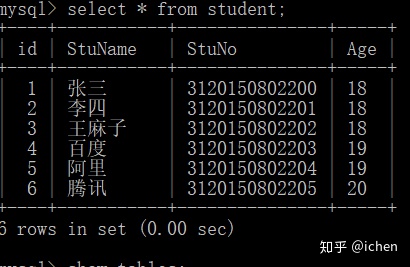
2 连接数据库
使用的是“窗体控件” 来实现连接数据库
step 1:新建一个“窗体控件”项目,从工具箱拖进“button ”公共控件
step 2:在解决方案资源管理器一栏,找到引用,并添加引用“MySql.Data.dll ”
step 3:双击控件,进入程序设计,添加:using MySql.Data.MySqlClient;
step 4:加接代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using MySql.Data.MySqlClient;
namespace mysql_connect
{
public partial class UserControl1: UserControl
{
public UserControl1()
{
InitializeComponent();
}
static string conStr = "server=localhost;port=3306;user=root;password=******; database=mysql_test;";//password 输入你所建立数据库的密码
private void Mysql_connect_Click(object sender, EventArgs e)
{
MySqlConnection connect = new MySqlConnection(conStr);
try
{
connect.Open();//建立连接,可能出现异常,使用try catch语句
MessageBox.Show("恭喜,已经建立连接!");
}
catch (MySqlException exe)
{
MessageBox.Show(exe.Message);//有错则报出错误
}
finally
{
connect.Close();//关闭通道
}
}
}
}
3 数据操作
数据库连接成功之后,就可以使用SQL语句对数据库进行命令操作了。command类提供了几个可执行的命令,下面分别介绍。
流程如下:
第一,使用SqlConnection对象连接数据库;
第二,建立SqlCommand对象,负责SQL语句的执行和存储过程的调用;
第三,对SQL或存储过程执行后返回的“结果”进行操作。
3.1 ExecuteNonQuery(): 执行一个命令,但不返回任何结果,就是执行非查询语句,如:Update:更新;Insert:插入;Delete:删除。
//Update更新代码
string SqlString = "Update ff "
+" Set username='李四',password='20191001'"
+" where id='2'";//SQL语句
MySqlCommand cmd = new MySqlCommand(SqlString,connect);//建立数据库命令,确定sql数据操作语句,和数据库连接。
int ret = cmd.ExecuteNonQuery();//执行SQL语句
MessageBox.Show("执行成功,影响了"+ ret.ToString() + "条数据!");
3.2 ExecuteReader():执行一个命令,返回一个类型化的IDataReader;执行查询语句的命令,也就是select语句。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using MySql.Data.MySqlClient;
namespace mysql_read1
{
public partial class UserControl1: UserControl
{
public UserControl1()
{
InitializeComponent();
}
static string conStr = "server=localhost;port=3306;user=root;password=11xxjlw520; database=mysql_test;";//password 输入你所建立数据库的密码
private void Button1_Click(object sender, EventArgs e)
{
MySqlConnection connect = new MySqlConnection(conStr);
try
{
connect.Open();//建立连接,可能出现异常,使用try catch语句
string SqlStr = "select StuName,StuNo,Age from student where StuNo='3120150802202'";
MySqlCommand cmd = new MySqlCommand(SqlStr, connect);
MySqlDataReader DataReader = cmd.ExecuteReader();
while (DataReader.Read())
{
Console.WriteLine(DataReader.GetString("StuName") + "t" + DataReader.GetString("StuNo") + "t"
+ "t" + DataReader.GetString("Age"));//"userid"是数据库对应的列名,推荐这种方式
}
}
catch (MySqlException exe)
{
MessageBox.Show(exe.Message);//有错则报出错误
}
finally
{
connect.Close();//关闭通道
}
}
}
}
3.3 ExecuteScalar:执行一个命令,返回一个值。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using MySql.Data.MySqlClient;
namespace mysql
{
public partial class UserControl1: UserControl
{
public UserControl1()
{
InitializeComponent();
}
static string conStr = "server=localhost;port=3306;user=root;password=11xxjlw520; database=mysql_test;";//password 输入你所建立数据库的密码
private void Button1_Click(object sender, EventArgs e)
{
MySqlConnection connect = new MySqlConnection(conStr);
try
{
connect.Open();//建立连接,可能出现异常,使用try catch语句
string SqlStr = "select now()";
MySqlCommand cmd = new MySqlCommand(SqlStr, connect);
object Ret = cmd.ExecuteScalar();
MessageBox.Show("数据库服务器当前系统时间是:"+Ret.ToString());
}
catch (MySqlException exe)
{
MessageBox.Show(exe.Message);//有错则报出错误
}
finally
{
connect.Close();//关闭通道
}
}
}
}
4.数据绑定:将我们需要的数据与显示的控件联系在一起。下面将实现使用DataGridView控件来显示连接数据源的详细数据。
step 1:创建Windows窗体程序
step 2:建立和数据库mysql_test的连接(向导法和程序法)