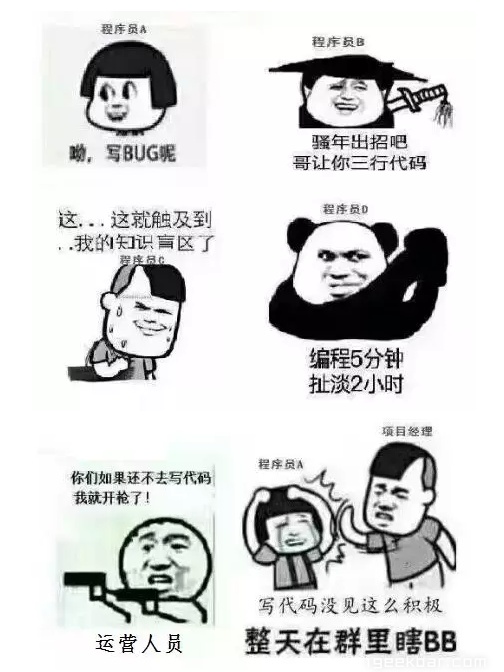
PageHelper 说明
Mybatis通用分页插件
PageHelper资源地址
https://github.com/pagehelper/Mybatis-PageHelper
PageHelper实现原理 PageHelper配置
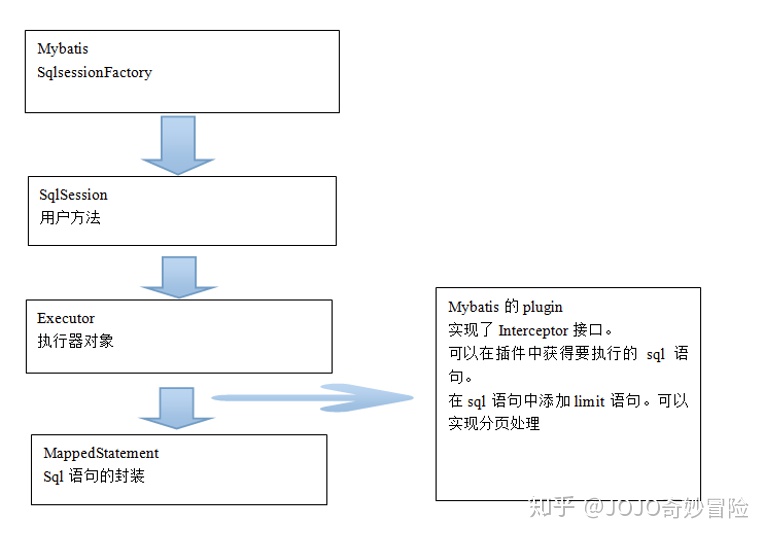
PageHelper配置
<plugins>
<plugin interceptor= "com.github.pagehelper.PageHelper" >
<!-- 设置数据库类型 Oracle,Mysql,MariaDB,SQLite,Hsqldb,PostgreSQL六 种数据库 -->
<property name= "dialect" value= "mysql" />
</plugin>
</plugins>
ego-common创建 PageResult 类
package com.bjsxt.ego.beans;
import java.io.Serializable; import java.util.List;
/** * 封装datagrid控件需要的数据模型
*
**/
public class PageResult<T> implements Serializable{
private List<T> rows;
private Long total;
public List<T> getRows() {
return rows;
}
public void setRows(List<T> rows) { this.rows = rows; }
public Long getTotal()
{
return total;
}
public void setTotal(Long total) {
this.total = total;
} }
创建 ItemService 接口
package com.bjsxt.ego.rpc.service;
import com.bjsxt.ego.beans.PageResult;
import com.bjsxt.ego.rpc.pojo.TbItem;
public interface ItemService {
/**
* 实现商品信息的分页查询
* **/
public PageResult<TbItem> selectItemList(Integer page,Integer rows);
}
创建 ItemServiceImpl 实现类
package com.bjsxt.ego.rpc.service.impl;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.bjsxt.ego.beans.PageResult;
import com.bjsxt.ego.rpc.mapper.TbItemMapper;
import com.bjsxt.ego.rpc.pojo.TbItem;
import com.bjsxt.ego.rpc.pojo.TbItemExample;
import com.bjsxt.ego.rpc.service.ItemService;
import com.github.pagehelper.Page;
import com.github.pagehelper.PageHelper;
@Service public class ItemServiceImpl implements ItemService {
//注入mapper接口代理对象
@Autowired
private TbItemMapper tbItemMapper;
@Override
public PageResult<TbItem> selectItemList(Integer page, Integer rows) {
// TODO Auto-generated method stub
//执行分页操作
Page ps = PageHelper. startPage (page, rows);
TbItemExample example=new TbItemExample();
//执行数据库查询操作
List<TbItem> list = tbItemMapper.selectByExample(example);
PageResult<TbItem> result = new PageResult<TbItem>();
result.setRows(list);
result.setTotal(ps.getTotal());
return result;
}
}
配置 applicationContext-dubbo.xml
<dubbo:service interface= "com.bjsxt.ego.rpc.service.ItemService"
ref= "itemServiceImpl" >
</dubbo:service>
启动 ego-rpc-service-impl 发布 RPC 服务
package com.bjsxt.ego.test;
import java.io.IOException;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class ProviderTest {
public static void main(String[] args) {
/**
* 加载spring容器,完成服务发布
*
**/ ClassPathXmlApplicationContext ac=
new ClassPathXmlApplicationContext("spring/applicationContext-dao.xml",
"spring/applicationContext-service.xml",
"spring/applicationContext-tx.xml",
"spring/applicationContext-dubbo.xml");
ac.start();
//阻塞程序的运行
try { System. in .read(); }
catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace(); }
ac.stop();
} }
商品分页查询 ego-manager-web 实现
配置 applicationContext-dubbo.xml
<dubbo:reference interface= "com.bjsxt.ego.rpc.service.ItemService"
id= "itemServiceProxy" ></dubbo:reference>
创建 ManagerItemService 接口
package com.bjsxt.ego.manager.service;
import com.bjsxt.ego.beans.PageResult; import com.bjsxt.ego.rpc.pojo.TbItem;
public interface ManagerItemService {
/**
* 完成商品信息的分页查询
*
**/
public PageResult<TbItem> selectItemListService(Integer page ,Integer rows);
}
创建 ManagerItemServiceImpl 实现类
package com.bjsxt.ego.manager.service.impl;
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service;
import com.bjsxt.ego.beans.PageResult;
import com.bjsxt.ego.manager.service.ManagerItemService;
import com.bjsxt.ego.rpc.pojo.TbItem;
import com.bjsxt.ego.rpc.service.ItemService;
@Service
public class ManagerItemServiceImpl implements ManagerItemService {
//注入的是远程服务的代理对象
@Autowired
private ItemService itemServiceProxy;
@Override
public PageResult<TbItem> selectItemListService(Integer page, Integer rows) {
// TODO Auto-generated method stub
return itemServiceProxy.selectItemList(page, rows);
}
}
创建 ItemController 类
package com.bjsxt.ego.manager.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.bjsxt.ego.beans.PageResult;
import com.bjsxt.ego.manager.service.ManagerItemService;
import com.bjsxt.ego.rpc.pojo.TbItem;
@Controller
public class ItemController {
//注入service对象
@Autowired
private ManagerItemService managerItemService;
/*** * 处理商品信息分页查询的请求
* **/
@RequestMapping(value="item/list",produces=MediaType. APPLICATION_JSON_VALU E +";charset=UTF-8")
@ResponseBody
public PageResult<TbItem> itemList(@RequestParam(defaultValue="1")Integer page,
@RequestParam(defaultValue="30")Integer rows){
return managerItemService.selectItemListService(page, rows);
} }